Game::Entity Class Reference
#include <entity.h>
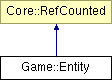
Detailed Description
The game entity class. A game entity represents one game object, such as an actor, an item, etc...The entity class itself doesn't have any game specific attributes or functionality. Instead it's configured by attaching properties to the entity. Properties add functionality to a game entity, for instance, if an entity should be visible, add a GraphicsProperty, if it should be audible, add an AudioProperty, and so forth...
Any data used for initilizing any property or holding any information about states etc. is stored in the attributeTable.
(C) 2007 RadonLabs GmbH
Public Types | |
typedef unsigned int | EntityId |
an Id type, used to identify entities | |
Public Member Functions | |
Entity () | |
constructor | |
virtual | ~Entity () |
destructor | |
const Util::String & | GetCategory () const |
get the entity's category | |
EntityId | GetUniqueId () const |
get unique id of entity | |
bool | AcceptsMessage (const Messaging::Id &msgId) const |
return true if any property accepts/processes message | |
void | SendSync (const Ptr< Messaging::Message > &msg) |
send a synchronous message to the entity | |
bool | IsActive () const |
return true if the entity is currently active (between OnActivate/OnDeactivate) | |
const Ptr < Db::ValueTable > & | GetAttrTable () const |
get the instance attribute table for the entity | |
IndexT | GetAttrTableRowIndex () const |
get the instance attribute table row index for the entity | |
Ptr< Property > | FindProperty (const Core::Rtti &rtti) const |
find property by RTTI (performs IsA() check), returns invalid pointer if not exists, slow! | |
void | RegisterPropertyCallback (const Ptr< Property > &prop, Property::CallbackType callback) |
register a property callback, called by Property::SetupCallback() method | |
void | OnActivate () |
called when attached to world | |
void | OnDeactivate () |
called when removed from world | |
void | OnBeginFrame () |
called at the beginning of the frame | |
void | OnMoveBefore () |
called before movement | |
void | OnMoveAfter () |
called after movement | |
void | OnRender () |
called before rendering | |
void | OnRenderDebug () |
debug rendering called before rendering | |
void | OnLoseActivity () |
called if entity loses activity | |
void | OnGainActivity () |
called if entity gains activity | |
void | OnLoad () |
called after loading from database has happened | |
void | OnStart () |
called when the entity starts to live in the complete world | |
void | OnSave () |
called before saving from database happens | |
bool | HasAttr (const Attr::AttrId &attrId) const |
return true if entity has an attribute | |
void | SetAttr (const Attr::Attribute &attr) |
generic attribut setter (slow!) | |
Attr::Attribute | GetAttr (const Attr::AttrId &attr) const |
generic attribute getter (slow!) | |
void | SetString (const Attr::StringAttrId &attrId, const Util::String &s) |
set string attribute on the entity | |
const Util::String & | GetString (const Attr::StringAttrId &attrId) const |
get string attribute from the entity | |
void | SetInt (const Attr::IntAttrId &attrId, int i) |
set int attribute on the entity | |
int | GetInt (const Attr::IntAttrId &attrId) const |
get int attribute from the entity | |
void | SetFloat (const Attr::FloatAttrId &attrId, float f) |
set float attribute on the entity | |
float | GetFloat (const Attr::FloatAttrId &attrId) const |
get float attribute from the entity | |
void | SetBool (const Attr::BoolAttrId &attrId, bool b) |
set bool attribute on the entity | |
bool | GetBool (const Attr::BoolAttrId &attrId) const |
get bool attribute from the entity | |
void | SetFloat4 (const Attr::Float4AttrId &attrId, const Math::float4 &v) |
set float4 attribute on the entity | |
const Math::float4 | GetFloat4 (const Attr::Float4AttrId &attrId) const |
get float4 attribute from the entity | |
void | SetMatrix44 (const Attr::Matrix44AttrId &attrId, const Math::matrix44 &m) |
set matrix44 attribute on the entity | |
const Math::matrix44 | GetMatrix44 (const Attr::Matrix44AttrId &attrid) const |
get matrix44 attribute from the entity | |
void | SetGuid (const Attr::GuidAttrId &attrId, const Util::Guid &guid) |
set guid attribute on the entity | |
const Util::Guid & | GetGuid (const Attr::GuidAttrId &attrId) const |
get guid attribute on the entity | |
void | SetBlob (const Attr::BlobAttrId &attrId, const Util::Blob &blob) |
set blob attribute on the entity | |
const Util::Blob & | GetBlob (const Attr::BlobAttrId &attrId) const |
get blob attribute on the entity | |
void | AddString (const Attr::StringAttrId &attrId) |
add string attribute if not exists | |
void | AddInt (const Attr::IntAttrId &attrId) |
add int attribute if not exists | |
void | AddFloat (const Attr::FloatAttrId &attrId) |
add float attribute if not exists | |
void | AddBool (const Attr::BoolAttrId &attrId) |
add bool attribute if not exists | |
void | AddFloat4 (const Attr::Float4AttrId &attrId) |
add float4 attribute if not exists | |
void | AddMatrix44 (const Attr::Matrix44AttrId &attrId) |
add matrix44 attribute if not exists | |
void | AddGuid (const Attr::GuidAttrId &attrId) |
add guid attribute if not exists | |
void | AddBlob (const Attr::BlobAttrId &attrId) |
add blob attribute if not exists | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void Game::Entity::RegisterPropertyCallback | ( | const Ptr< Property > & | prop, | |
Property::CallbackType | callbackType | |||
) |
register a property callback, called by Property::SetupCallback() method
This method is called from within Property::SetupCallbacks() to register per-frame callback methods with the entity.
void Game::Entity::OnActivate | ( | ) |
called when attached to world
Called when the game entity has been attached to a game level object. This will attach contained subsystem entities to their respective subsystems.
- Parameters:
-
l pointer to a level object to which the entity was attached
- 01-Apr-05 floh graphics entity now created after physics entity
void Game::Entity::OnDeactivate | ( | ) |
called when removed from world
Called when the game entity has been removed from the game level object. This will remove any contained subsystem entities from their subsystems.
void Game::Entity::OnBeginFrame | ( | ) |
called at the beginning of the frame
Called on game entities at the begin of the frame.
void Game::Entity::OnMoveBefore | ( | ) |
called before movement
Called on game entities before movement.
void Game::Entity::OnMoveAfter | ( | ) |
called after movement
Called on game entities after movement.
void Game::Entity::OnRender | ( | ) |
called before rendering
Called on game entities before rendering.
void Game::Entity::OnRenderDebug | ( | ) |
debug rendering called before rendering
Called on game entities before rendering.
void Game::Entity::OnLoseActivity | ( | ) |
called if entity loses activity
Called on game entities at the begin of the frame.
void Game::Entity::OnGainActivity | ( | ) |
called if entity gains activity
Called on game entities if gaining/regaining activity
void Game::Entity::OnLoad | ( | ) |
called after loading from database has happened
This method is called after the game world has been loaded from the database. At the time when this method is called all entities in the world have already been created and their attributes have been loaded from the database.
This method reads the entity attributes from the world database. A valid GUID attribute must exist on the entity for identification in the database. After the attributes are loaded from the world database, the Property::OnLoad() method will be called on all attached properties.
void Game::Entity::OnStart | ( | ) |
called when the entity starts to live in the complete world
This method is called in 2 cases:
When a level is loaded it is called on all entities after OnLoad when the complete world already exist.
When a entity is created at ruTime (while a level is active) OnStart is called after the entity is attached to level.
void Game::Entity::OnSave | ( | ) |
called before saving from database happens
This method writes the current entity attributes back to the world database. At least a valid GUID attribute must exist on the entity for identification. If no entry exists yet in the database for the entity, a new one will be created. Before the attributes are written back to the database, the method Property::OnSave() will be called on each attached property.
void Game::Entity::SetAttr | ( | const Attr::Attribute & | attr | ) |
generic attribut setter (slow!)
Generic attribute setter method. This is slower then the specialied attribute setter methods!
Attr::Attribute Game::Entity::GetAttr | ( | const Attr::AttrId & | attrId | ) | const |
generic attribute getter (slow!)
Generic attribute getter method. This is slower then the specialied attribute getter methods!
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.