Graphics::Cell Class Reference
#include <cell.h>
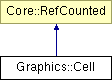
Detailed Description
Hierarchies of Cell objects group graphics entities by spatial relationship. They are the key class for efficient visibility queries. An application may derive specialized subclasses of Cell which must only adhere to the following 2 simple rules:
- if the Cell object is fully visible, all child Cells and all Entities attached to the Cell are guaranteed to be visible
- if the Cell object is fully invisible, all child Cells and all Entities attached to the Cell are guaranteed to be invisible
- Todo:
- : need to handle extra shadow bounding box
: statistics and profiling
: need to add visibility depending on LOD
: multithreaded visibility link update?
: add dirty handling to visibility links (e.g. don't need to update links between static lights and static objects)
Public Member Functions | |
Cell () | |
constructor | |
virtual | ~Cell () |
destructor | |
virtual void | OnAttachToStage (const Ptr< Stage > &stage) |
called when attached to Stage | |
virtual void | OnRemoveFromStage () |
called when removed from Stage | |
bool | IsAttachedToStage () const |
return true if currently attached to a stage | |
const Ptr< Stage > & | GetStage () const |
get stage we are attached to | |
void | SetBoundingBox (const Math::bbox &box) |
set the Cell's world space bounding box | |
const Math::bbox & | GetBoundingBox () const |
get the Cell's world space transform | |
void | AttachChildCell (const Ptr< Cell > &cell) |
add a child cell (only during setup phase) | |
const Ptr< Cell > & | GetParentCell () const |
get pointer to parent cell (returns invalid pointer if this is root cell) | |
const Util::Array < Ptr< Cell > > & | GetChildCells () const |
get current child cells | |
void | AttachEntity (const Ptr< GraphicsEntity > &entity) |
attach a graphics entity to this Cell | |
void | RemoveEntity (const Ptr< GraphicsEntity > &entity) |
remove a graphics entity from this Cell | |
void | InsertEntity (const Ptr< GraphicsEntity > &entity) |
insert an entity into the Cell hierarchy | |
const Util::Array < Ptr < GraphicsEntity > > & | GetEntities () const |
get all entities | |
const Util::Array < Ptr < GraphicsEntity > > & | GetEntitiesByType (GraphicsEntity::Type type) const |
get entities by entity type | |
SizeT | GetNumEntitiesInHierarchy () const |
get the number of entities in hierarchy | |
SizeT | GetNumEntitiesInHierarchyByType (GraphicsEntity::Type t) const |
get the number of entities in hierarchy by type | |
SizeT | GetNumEntitiesInHierarchyByTypeMask (uint entityTypeMask) const |
get the number of entities in hierarchy by entity type mask | |
void | UpdateLinks (const Ptr< GraphicsEntity > &observerEntity, uint entityTypeMask, GraphicsEntity::LinkType linkType) |
recursively create visibility links between observers and observed entities | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
add a child cell (only during setup phase)
NOTE: the cell hierarchy may only be built during the setup phase while the cell hierarchy haven't been added to the stage yet.
void Graphics::Cell::AttachEntity | ( | const Ptr< GraphicsEntity > & | entity | ) |
void Graphics::Cell::InsertEntity | ( | const Ptr< GraphicsEntity > & | entity | ) |
insert an entity into the Cell hierarchy
Insert a dynamic graphics entity into the cell tree. The entity will correctly be inserted into the smallest enclosing cell in the tree. The cell may not be currently attached to a cell, the refcount of the entity will be incremented.
- Parameters:
-
entity pointer to a graphics entity
void Graphics::Cell::UpdateLinks | ( | const Ptr< GraphicsEntity > & | observerEntity, | |
uint | observedEntityTypeMask, | |||
GraphicsEntity::LinkType | linkType | |||
) |
recursively create visibility links between observers and observed entities
Frontend method for updating visibility links. This method simply calls RecurseUpdateLinks() which recurses into child cells if necessary.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.