Graphics::ActorEntity Class Reference
#include <actorentity.h>
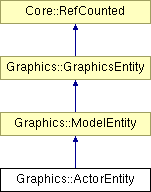
Detailed Description
An actor graphics entity. NOTE: The current ActorEntity class is just a wrapper around the legacy Nebula2 Character3 system and will be replaced with a "proper" Nebula3 character system in the future.(C) 2007 Radon Labs GmbH
Public Types | |
enum | Type |
entity types | |
enum | LinkType |
visibility link types | |
Public Member Functions | |
ActorEntity () | |
constructor | |
virtual | ~ActorEntity () |
destructor | |
virtual void | OnActivate () |
called when attached to game entity | |
virtual void | OnDeactivate () |
called when removed from game entity | |
void | SetAnimationMapping (const Util::String &n) |
set animation mapping to use | |
const Util::String & | GetAnimationMapping () const |
get animation mapping to use | |
void | SetSkinList (const Util::String &s) |
set optional skin lists for character3's | |
const Util::String & | GetSkinList () const |
get skin list | |
void | SetBaseAnimation (const Util::String &anim, Timing::Time fadeIn=0.0, Timing::Time timeOffset=0.0, bool onlyIfInactive=true, bool waitForOverlayAnim=false, float randomValue=0.0f) |
set the current base animation (usually loop anims) - OBSOLETE, use FadeAnimation(), ClearAnimations() | |
Util::String | GetBaseAnimation () const |
get current base animation (first of mix) | |
Util::String | GetBaseClip () const |
get current base clip (first of mix) | |
Timing::Time | GetBaseAnimationDuration () const |
get base animation duration | |
void | SetOverlayAnimation (const Util::String &anim, Timing::Time fadeIn=0.0, Timing::Time overrideDuration=0.0, bool onlyIfInactive=true, float randomValue=0.0f) |
set the current overlay animation (usually oneshot anims) - OBSOLETE, use FadeAnimation(), ClearAnimations() | |
Util::String | GetOverlayAnimation () const |
get name of currently active overlay animation (first of mix) | |
Util::String | GetOverlayClip () const |
get name of currently active overlay clip (first of mix) | |
Timing::Time | GetOverlayAnimationDuration () const |
get overlay animation duration | |
void | SetFadeAnimationMix (const Util::Array< Util::String > &anims, const Util::Array< float > &weights, Timing::Time fadeIn, Timing::Time overrideDuration, float randomValue) |
set the current fade animations - OBSOLETE, use FadeAnimation(), ClearAnimations() | |
void | StopOverlayAnimation (Timing::Time fadeIn) |
stop current overlay animation | |
bool | IsOverlayAnimationActive () const |
return true if overlay animation is currently active | |
virtual void | OnUpdate () |
called before rendering happens | |
Char::Character * | GetCharacterPointer () const |
get pointer to our nCharacter2 object | |
void | EvaluateSkeleton (bool enforceEvaluation=false) |
bring the character's skeleton uptodate | |
bool | HasCharacter3Set () const |
is this a character3 ? | |
Char::CharacterSet * | GetCharacter3Set () const |
get the character3 set, fail if no character3 set exists | |
int | GetJointIndexByName (const Util::String &name) |
return joint index by name, return InvalidIndex if joint doesn't exist | |
Char::CharJoint * | GetJoint (int jointIndex) const |
return joint at index | |
const Math::matrix44 & | GetLocalJointMatrix (int jointIndex) const |
return a joint's current matrix in model space | |
Math::matrix44 | GetJointMatrixByIndex (IndexT jointIndex) |
get joint matrix by index in global space | |
Math::matrix44 | GetJointMatrixByName (const Util::String &jointName) |
get joint matrix by name in global space, throws assert if joint doesn't exist | |
Anim::AnimClipScheduler * | GetAnimClipScheduler () const |
return the animation clip scheduler | |
void | FadeAnimation (const Util::String &anim, float targetWeight=0.0f, Timing::Time fadeInTime=0.0, Timing::Time fadeOutTime=0.0, float randomValue=0.0f, float runTime=0.0f, float timeFactor=1.0f, Timing::Time sampleTime=0.0, bool animRestart=false, bool fadeOutRunningAnims=true) |
fade an animation to a weight | |
void | FadeAnimationMix (const Util::Array< Util::String > &anims, float targetWeight=0.0f, Timing::Time fadeInTime=0.0, Timing::Time fadeOutTime=0.0, float randomValue=0.0f, float runTime=0.0f, float timeFactor=1.0f, Timing::Time sampleTime=0.0, bool animRestart=false, bool fadeOutRunningAnims=true) |
fade a mix of animations to a weight | |
void | FadeAnimationMix (const Util::Array< Util::String > &anims, const Util::Array< float > &targetWeights, Timing::Time fadeInTime=0.0, Timing::Time fadeOutTime=0.0, float randomValue=0.0f, float runTime=0.0f, float timeFactor=1.0f, Timing::Time sampleTime=0.0, bool animRestart=false, bool fadeOutRunningAnims=true) |
fade a mix of animations to various weights | |
void | FadeRunningAnimationsOut () |
fade all running animations out | |
void | FadeRunningAnimationsOut (AnimationType type, const Util::Array< int > *clipFilter=0) |
fades running clips of the given type out | |
const Ptr < Models::CharacterNode > & | GetCharacterNode () const |
get character node | |
void | SetResourceId (const Resources::ResourceId &resId) |
set the model's resource id | |
const Resources::ResourceId & | GetResourceId () const |
get the model's resource id | |
Resources::Resource::State | GetModelResourceState () const |
get the state of the contained managed model resource | |
const Ptr < Models::ModelInstance > & | GetModelInstance () const |
get pointer to model instance (only valid if already loaded) | |
bool | IsActive () const |
return true if entity is currently active (is between OnActivate()/OnDeactivate() | |
bool | IsValid () const |
return true if entity is current valid (ready for rendering) | |
Type | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< Stage > & | GetStage () const |
get the stage this entity is attached to | |
bool | IsAttachedToStage () const |
return true if entity is attached to stage | |
const Ptr< Cell > & | GetCell () const |
get the cell this entity is attached to | |
bool | IsAttachedToCell () const |
return true if entity is attached to cell | |
const Math::bbox & | GetLocalBoundingBox () const |
get the local space bounding box | |
const Math::bbox & | GetGlobalBoundingBox () |
get bounding box in global space | |
void | ClearLinks (LinkType linkType) |
clear all visibility links | |
void | AddLink (LinkType linkType, const Ptr< GraphicsEntity > &entity) |
add visibility link | |
const Util::Array < Ptr < GraphicsEntity > > & | GetLinks (LinkType type) const |
get visibility links by type | |
virtual Math::ClipStatus::Type | ComputeClipStatus (const Math::bbox &box) |
compute clip status against bounding box | |
void | SetTime (Timing::Time t) |
set graphics time | |
Timing::Time | GetTime () const |
get graphics time | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | OnTransformChanged () |
called when transform matrix changed | |
virtual void | OnRenderDebug () |
called to render a debug visualization of the entity | |
void | ValidateModelInstance () |
validate the ModelInstance | |
void | SetType (Type t) |
set entity type, call in constructor of derived class! | |
void | SetLocalBoundingBox (const Math::bbox &b) |
set the local space bounding box | |
void | SetValid (bool b) |
set to valid state (when the entity becomes ready for rendering) | |
virtual Ptr < GraphicsEntity > | CreateClone () const |
create a clone of the entity | |
virtual void | OnAttachToStage (const Ptr< Stage > &stage) |
called when attached to Stage | |
virtual void | OnRemoveFromStage () |
called when removed from Stage | |
virtual void | OnAttachToCell (const Ptr< Cell > &cell) |
called when attached to a Cell | |
virtual void | OnRemoveFromCell () |
called when removed from a Cell | |
virtual void | OnShow () |
called when the entity becomes visible | |
virtual void | OnHide () |
called when the entity becomes invisible | |
virtual void | OnRender () |
called before the entity is rendered | |
void | UpdatePositionInCellTree () |
update our position in the cell hierarchy | |
void | UpdateGlobalBoundingBox () |
update the global bounding box from the transform and local box |
Member Function Documentation
void Graphics::ActorEntity::SetBaseAnimation | ( | const Util::String & | anim, | |
Timing::Time | fadeIn = 0.0 , |
|||
Timing::Time | timeOffset = 0.0 , |
|||
bool | onlyIfInactive = true , |
|||
bool | waitForOverlayAnim = false , |
|||
float | randomValue = 0.0f | |||
) |
set the current base animation (usually loop anims) - OBSOLETE, use FadeAnimation(), ClearAnimations()
Set a new base animation. This is usually a looping animation, like Idle, Walking, Running, etc...
- Parameters:
-
anim new base animation fadeIn time to fade from current animation timeOffset optional animation time offset
void Graphics::ActorEntity::SetOverlayAnimation | ( | const Util::String & | anim, | |
Timing::Time | fadeIn = 0.0 , |
|||
Timing::Time | overrideDuration = 0.0 , |
|||
bool | onlyIfInactive = true , |
|||
float | randomValue = 0.0f | |||
) |
set the current overlay animation (usually oneshot anims) - OBSOLETE, use FadeAnimation(), ClearAnimations()
Set a new overlay animation. This is usually a looping animation, like Idle, Walking, Running, etc...
- Parameters:
-
animName new base animation fadeIn time to fade from current animation overrideDuration if != 0.0, override the animation's duration with this value
void Graphics::ActorEntity::SetFadeAnimationMix | ( | const Util::Array< Util::String > & | anims, | |
const Util::Array< float > & | weights, | |||
Timing::Time | fadeIn, | |||
Timing::Time | overrideDuration, | |||
float | randomValue | |||
) |
set the current fade animations - OBSOLETE, use FadeAnimation(), ClearAnimations()
- Parameters:
-
clipNames new fade animations weights new fade animation weights
void Graphics::ActorEntity::OnUpdate | ( | ) | [virtual] |
called before rendering happens
This method checks whether the current overlay animation is over and the base animation must be re-activated. This is the only place where animations are actually started to prevent stuttering when several animations are started in the same frame.
Reimplemented from Graphics::ModelEntity.
Char::Character * Graphics::ActorEntity::GetCharacterPointer | ( | ) | const [inline] |
get pointer to our nCharacter2 object
Returns the pointer to our nCharacter2 object.
void Graphics::ActorEntity::EvaluateSkeleton | ( | bool | enforceEvaluation = false |
) |
bring the character's skeleton uptodate
This brings the character's skeleton uptodate. Make sure the entity's time and animation state weights in the rendercontext are uptodate before calling this method, to avoid one-frame-latencies.
int Graphics::ActorEntity::GetJointIndexByName | ( | const Util::String & | name | ) |
return joint index by name, return InvalidIndex if joint doesn't exist
Returns a character joint index by its name. Returns -1 if a joint by that name doesn't exist in the character.
CharJoint * Graphics::ActorEntity::GetJoint | ( | int | jointIndex | ) | const |
return joint at index
Return pointer to joint or 0 if joint doesn't exist.
const matrix44 & Graphics::ActorEntity::GetLocalJointMatrix | ( | int | jointIndex | ) | const |
return a joint's current matrix in model space
Returns a joint's current matrix in model space. Make sure to call EvaluateSkeleton() before!
matrix44 Graphics::ActorEntity::GetJointMatrixByIndex | ( | IndexT | jointIndex | ) |
get joint matrix by index in global space
Find the joint matrix in world space by index. Careful, this method does an EvaluateSkeleton() per invocation!
matrix44 Graphics::ActorEntity::GetJointMatrixByName | ( | const Util::String & | jointName | ) |
get joint matrix by name in global space, throws assert if joint doesn't exist
Returns a joint matrix in world space by name. Careful, this method does an EvaluateSkeleton() per invocation!
void Graphics::ActorEntity::FadeAnimation | ( | const Util::String & | anim, | |
float | targetWeight = 0.0f , |
|||
Timing::Time | fadeInTime = 0.0 , |
|||
Timing::Time | fadeOutTime = 0.0 , |
|||
float | randomValue = 0.0f , |
|||
float | runTime = 0.0f , |
|||
float | timeFactor = 1.0f , |
|||
Timing::Time | sampleTime = 0.0 , |
|||
bool | animRestart = false , |
|||
bool | fadeOutRunningAnims = true | |||
) |
fade an animation to a weight
Fades an animation to the specified target weight
- Parameters:
-
animRestart enable this, if you want to restart the clip (if it runs already) before fading it to the target weight fadeOutRunningAnims enable this, if all currently running clips should fade out
void Graphics::ActorEntity::FadeAnimationMix | ( | const Util::Array< Util::String > & | anims, | |
float | targetWeight = 0.0f , |
|||
Timing::Time | fadeInTime = 0.0 , |
|||
Timing::Time | fadeOutTime = 0.0 , |
|||
float | randomValue = 0.0f , |
|||
float | runTime = 0.0f , |
|||
float | timeFactor = 1.0f , |
|||
Timing::Time | sampleTime = 0.0 , |
|||
bool | animRestart = false , |
|||
bool | fadeOutRunningAnims = true | |||
) |
fade a mix of animations to a weight
Fades a mix of animations to the specified target weight
- Parameters:
-
animRestart enable this, if you want to restart currently running clips before fading them to the target weight fadeOutRunningAnims enable this, if all currently running clips should fade out
void Graphics::ActorEntity::FadeAnimationMix | ( | const Util::Array< Util::String > & | anims, | |
const Util::Array< float > & | targetWeights, | |||
Timing::Time | fadeInTime = 0.0 , |
|||
Timing::Time | fadeOutTime = 0.0 , |
|||
float | randomValue = 0.0f , |
|||
float | runTime = 0.0f , |
|||
float | timeFactor = 1.0f , |
|||
Timing::Time | sampleTime = 0.0 , |
|||
bool | animRestart = false , |
|||
bool | fadeOutRunningAnims = true | |||
) |
fade a mix of animations to various weights
Fades a mix of animations to the specified target weights
- Parameters:
-
animRestart enable this, if you want to restart currently running clips before fading them to the target weights fadeOutRunningAnims enable this, if all currently running clips should fade out
void Graphics::ActorEntity::FadeRunningAnimationsOut | ( | ) |
fade all running animations out
Fade out all animation clips with a weight > 0.0f
void Graphics::ActorEntity::FadeRunningAnimationsOut | ( | AnimationType | type, | |
const Util::Array< int > * | clipFilter = 0 | |||
) |
fades running clips of the given type out
Fade out all animation clips with a weight > 0.0f, if they are running as Base- or Overlay-Animation
- Parameters:
-
type specify which animation type should fade out (Base- or Overlay-Animations)
void Graphics::ModelEntity::OnTransformChanged | ( | ) | [protected, virtual, inherited] |
called when transform matrix changed
In OnTransformChanged() we need to update the transformation of our ModelInstance (if it has already been initialised).
Reimplemented from Graphics::GraphicsEntity.
void Graphics::ModelEntity::OnRenderDebug | ( | ) | [protected, virtual, inherited] |
called to render a debug visualization of the entity
Render our debug visualization (the bounding box).
Reimplemented from Graphics::GraphicsEntity.
void Graphics::ModelEntity::ValidateModelInstance | ( | ) | [protected, inherited] |
validate the ModelInstance
This creates and initializes our ModelInstance if needed. Since Model loading happens asynchronously this may happen at any time after the ModelEntity is activated.
ClipStatus::Type Graphics::GraphicsEntity::ComputeClipStatus | ( | const Math::bbox & | box | ) | [virtual, inherited] |
compute clip status against bounding box
Compute the clip status between this entity and a bounding box in global space. This method must be overwritten in a derived class.
Reimplemented in Graphics::CameraEntity, Lighting::GlobalLightEntity, and Lighting::SpotLightEntity.
Ptr< GraphicsEntity > Graphics::GraphicsEntity::CreateClone | ( | ) | const [protected, virtual, inherited] |
create a clone of the entity
This method is called to create an exact clone of this graphics entity. The new entity will not be attached to a stage.
void Graphics::GraphicsEntity::OnAttachToStage | ( | const Ptr< Stage > & | s | ) | [protected, virtual, inherited] |
called when attached to Stage
This method is called when the graphics entity is attached to a stage. An entity may only be attached to one stage at any time, but can be attached to different stages during its lifetime. Attachging an entity to a stage may be relatively slow because the entity must be inserted into the cell hierarchy.
void Graphics::GraphicsEntity::OnRemoveFromStage | ( | ) | [protected, virtual, inherited] |
called when removed from Stage
This method is called when the graphics entity is removed from a stage.
void Graphics::GraphicsEntity::OnRemoveFromCell | ( | ) | [protected, virtual, inherited] |
called when removed from a Cell
Called when the graphics entity is removed from a cell inside a stage.
void Graphics::GraphicsEntity::OnShow | ( | ) | [protected, virtual, inherited] |
called when the entity becomes visible
This method is called from the SetVisible() method when the entity changes from invisible to visible state.
void Graphics::GraphicsEntity::OnHide | ( | ) | [protected, virtual, inherited] |
called when the entity becomes invisible
This method is called from the SetVisible() method when the entity changes from visible to invisible state.
void Graphics::GraphicsEntity::OnRender | ( | ) | [protected, virtual, inherited] |
called before the entity is rendered
This method is called on the graphics entity to render itself. This method will only be called if the entity is visible through the camera of the currently rendered View.
void Graphics::GraphicsEntity::UpdatePositionInCellTree | ( | ) | [protected, inherited] |
update our position in the cell hierarchy
This method is called from OnUpdate() when either the entity's transformation or bounding box has changed. It checks if the entity still fits into its current cell, and if not, moves the entity in a new cell.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.