Win32::Win32DisplayDevice Class Reference
#include <win32displaydevice.h>
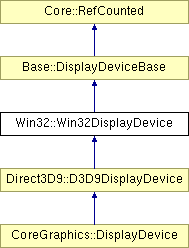
Detailed Description
Win32 implementation of DisplayDevice class. Manages the application window.(C) 2006 Radon Labs GmbH
Public Member Functions | |
Win32DisplayDevice () | |
constructor | |
virtual | ~Win32DisplayDevice () |
destructor | |
virtual bool | Open () |
open the display | |
virtual void | Close () |
close the display | |
virtual void | ProcessWindowMessages () |
process window system messages, call this method once per frame | |
HWND | GetHwnd () const |
get the application window HWND | |
bool | AdapterExists (CoreGraphics::Adapter::Code adapter) |
return true if adapter exists | |
Util::Array < CoreGraphics::DisplayMode > | GetAvailableDisplayModes (CoreGraphics::Adapter::Code adapter, CoreGraphics::PixelFormat::Code pixelFormat) |
get available display modes on given adapter | |
bool | SupportsDisplayMode (CoreGraphics::Adapter::Code adapter, const CoreGraphics::DisplayMode &requestedMode) |
return true if a given display mode is supported | |
CoreGraphics::DisplayMode | GetCurrentAdapterDisplayMode (CoreGraphics::Adapter::Code adapter) |
get current adapter display mode (i.e. the desktop display mode) | |
CoreGraphics::AdapterInfo | GetAdapterInfo (CoreGraphics::Adapter::Code adapter) |
get general info about display adapter | |
void | SetAdapter (CoreGraphics::Adapter::Code a) |
set display adapter (make sure adapter exists!) | |
CoreGraphics::Adapter::Code | GetAdapter () const |
get display adapter | |
void | SetDisplayMode (const CoreGraphics::DisplayMode &m) |
set display mode (make sure the display mode is supported!) | |
const CoreGraphics::DisplayMode & | GetDisplayMode () const |
get display mode | |
void | SetAntiAliasQuality (CoreGraphics::AntiAliasQuality::Code aa) |
set antialias quality | |
CoreGraphics::AntiAliasQuality::Code | GetAntiAliasQuality () const |
get antialias quality | |
void | SetFullscreen (bool b) |
set windowed/fullscreen mode | |
bool | IsFullscreen () const |
get windowed/fullscreen mode | |
void | SetDisplayModeSwitchEnabled (bool b) |
enable display mode switch when running fullscreen (default is true); | |
bool | IsDisplayModeSwitchEnabled () const |
is display mode switch enabled for fullscreen? | |
void | SetTripleBufferingEnabled (bool b) |
enable triple buffer for fullscreen (default is double buffering) | |
bool | IsTripleBufferingEnabled () const |
is triple buffer enabled for fullscreen? | |
void | SetAlwaysOnTop (bool b) |
set always-on-top behaviour | |
bool | IsAlwaysOnTop () const |
get always-on-top behaviour | |
void | SetVerticalSyncEnabled (bool b) |
turn vertical sync on/off | |
bool | IsVerticalSyncEnabled () const |
get vertical sync flag | |
void | SetIconName (const Util::String &s) |
set optional window icon resource name | |
const Util::String & | GetIconName () const |
get optional window icon resource name | |
void | SetWindowTitle (const Util::String &t) |
set window title string (can be changed anytime) | |
const Util::String & | GetWindowTitle () const |
get window title string | |
bool | IsOpen () const |
return true if display is currently open | |
void | AttachEventHandler (const Ptr< CoreGraphics::DisplayEventHandler > &h) |
attach a display event handler | |
void | RemoveEventHandler (const Ptr< CoreGraphics::DisplayEventHandler > &h) |
remove a display event handler | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual bool | OpenWindow () |
open the application window | |
virtual void | CloseWindow () |
close the application window | |
virtual void | OnMinimized () |
called on WM_SIZE when window is minimized | |
virtual void | OnRestored () |
called on WM_SIZE when window is restored | |
virtual bool | OnSetCursor () |
called on WM_SETCURSOR | |
virtual void | OnPaint () |
called on WM_PAINT | |
virtual void | OnSetFocus () |
called on WM_SETFOCUS | |
virtual void | OnKillFocus () |
called on WM_KILLFOCUS | |
virtual void | OnCloseRequested () |
called on WM_CLOSE to request if window should be closed | |
virtual void | OnToggleFullscreenWindowed () |
called when Alt-Enter is pressed | |
virtual void | OnKeyDown (WPARAM wParam) |
called on WM_KEYDOWN | |
virtual void | OnKeyUp (WPARAM wParam) |
called on WM_KEYUP | |
virtual void | OnChar (WPARAM wParam) |
called on WM_CHAR | |
virtual void | OnMouseButton (UINT uMsg, LPARAM lParam) |
called on mouse button event | |
virtual void | OnMouseMove (LPARAM lParam) |
called on WM_MOUSEMOVE | |
virtual void | OnMouseWheel (WPARAM wParam) |
called on WM_MOUSEWHEEL | |
Input::Key::Code | TranslateKeyCode (WPARAM wParam) const |
translate a Windows virtual key code into a Nebula3 key code | |
virtual CoreGraphics::DisplayMode | ComputeAdjustedWindowRect () |
adjust window size taking client area into account | |
Math::float2 | ComputeAbsMousePos (LPARAM lParam) const |
compute absolute mouse position from lParam | |
Math::float2 | ComputeNormMousePos (const Math::float2 &absMousePos) const |
compute normalized mouse position from absolute mouse pos | |
bool | NotifyEventHandlers (const CoreGraphics::DisplayEvent &e) |
notify event handlers about an event | |
Static Protected Member Functions | |
static LRESULT CALLBACK | WinProc (HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam) |
the WinProc |
Member Function Documentation
void Win32::Win32DisplayDevice::ProcessWindowMessages | ( | ) | [virtual] |
process window system messages, call this method once per frame
Polls for and processes window messages. Call this message once per frame in your render loop. If the user clicks the window close button, or hits Alt-F4, a CloseRequested input event will be sent.
Reimplemented from Base::DisplayDeviceBase.
LRESULT CALLBACK Win32::Win32DisplayDevice::WinProc | ( | HWND | hWnd, | |
UINT | uMsg, | |||
WPARAM | wParam, | |||
LPARAM | lParam | |||
) | [static, protected] |
the WinProc
The Nebula3 WinProc.
bool Win32::Win32DisplayDevice::OpenWindow | ( | ) | [protected, virtual] |
open the application window
Open the application window.
void Win32::Win32DisplayDevice::CloseWindow | ( | ) | [protected, virtual] |
close the application window
Close the application window.
Input::Key::Code Win32::Win32DisplayDevice::TranslateKeyCode | ( | WPARAM | wParam | ) | const [protected] |
translate a Windows virtual key code into a Nebula3 key code
Helper method which translates a Win32 virtual key code into a Nebula key code.
DisplayMode Win32::Win32DisplayDevice::ComputeAdjustedWindowRect | ( | ) | [protected, virtual] |
adjust window size taking client area into account
This will return an adjusted window size which takes the client area of the window into account. This is only relevant for windowed mode.
bool Base::DisplayDeviceBase::AdapterExists | ( | CoreGraphics::Adapter::Code | adapter | ) | [inherited] |
return true if adapter exists
Checks if the given adapter exists.
Reimplemented in Direct3D9::D3D9DisplayDevice.
Util::Array< DisplayMode > Base::DisplayDeviceBase::GetAvailableDisplayModes | ( | CoreGraphics::Adapter::Code | adapter, | |
CoreGraphics::PixelFormat::Code | pixelFormat | |||
) | [inherited] |
get available display modes on given adapter
Returns the display modes on the given adapter in the given pixel format.
Reimplemented in Direct3D9::D3D9DisplayDevice.
bool Base::DisplayDeviceBase::SupportsDisplayMode | ( | CoreGraphics::Adapter::Code | adapter, | |
const CoreGraphics::DisplayMode & | requestedMode | |||
) | [inherited] |
return true if a given display mode is supported
This method checks the available display modes on the given adapter against the requested display modes and returns true if the display mode exists.
Reimplemented in Direct3D9::D3D9DisplayDevice.
DisplayMode Base::DisplayDeviceBase::GetCurrentAdapterDisplayMode | ( | CoreGraphics::Adapter::Code | adapter | ) | [inherited] |
get current adapter display mode (i.e. the desktop display mode)
This method returns the current adapter display mode. It can be used to get the current desktop display mode.
Reimplemented in Direct3D9::D3D9DisplayDevice.
AdapterInfo Base::DisplayDeviceBase::GetAdapterInfo | ( | CoreGraphics::Adapter::Code | adapter | ) | [inherited] |
get general info about display adapter
Returns information about the provided adapter.
Reimplemented in Direct3D9::D3D9DisplayDevice.
void Base::DisplayDeviceBase::SetWindowTitle | ( | const Util::String & | str | ) | [inherited] |
set window title string (can be changed anytime)
Set the window title. An application should be able to change the window title at any time, that's why this is a virtual method, so that a subclass may override it.
void Base::DisplayDeviceBase::AttachEventHandler | ( | const Ptr< CoreGraphics::DisplayEventHandler > & | h | ) | [inherited] |
attach a display event handler
Attach an event handler to the display device.
void Base::DisplayDeviceBase::RemoveEventHandler | ( | const Ptr< CoreGraphics::DisplayEventHandler > & | h | ) | [inherited] |
remove a display event handler
Remove an event handler from the display device.
bool Base::DisplayDeviceBase::NotifyEventHandlers | ( | const CoreGraphics::DisplayEvent & | e | ) | [protected, inherited] |
notify event handlers about an event
Notify all event handlers about an event.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.