IO::ZipFileSystem Class Reference
#include <zipfilesystem.h>
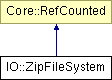
Detailed Description
A filesystem wrapper for ZIP files. It is recommended to use the ZipStreamClass to access Zip files, and only fall back to the ZipFileSystem class if advanced functionality is needed which is not provided by the ZipStreamClass.Uses the zlib and the minizip package under the hood.
Limitations: No write access. No seek on compressed data, the ZipFileSystem will generally decompress an entire file into memory at once, so that the ZipStreamClass can provide random access on the decompressed data. Thus the typical "audio streaming scenario" is not possible from zip files (that's what XACT's sound banks is there for anyway ;)
How to fix the no-seek problem: zlib processes datas in chunks, and cannot seek randomly within a chunk, and the chunk size is dependent on the compress application being used to create the zip file(?), if those internals are known, it would be possible to write a chunked filesystem which keeps buffered chunks around for each client, probably not worth the effort. Another appoach would be to split stream-files into "chunk-files" before compressing, and to read the next complete chunk files when new data is needed. This approach doesn't require changes to the strip filesystem.
(C) 2006 Radon Labs GmbH
Public Member Functions | |
ZipFileSystem () | |
constructor | |
virtual | ~ZipFileSystem () |
destructor | |
Ptr< ZipArchive > | Mount (const URI &uri) |
"mount" a zip archive | |
void | Unmount (const URI &uri) |
"unmount" a zip archive by its file name | |
void | Unmount (const Ptr< ZipArchive > &zipArchive) |
"unmount a zip archive by pointer | |
bool | IsMounted (const URI &uri) const |
return true if a zip archive is already mounted | |
Util::Array< Ptr < ZipArchive > > | GetMountedZipArchives () const |
get all mounted zip archives | |
const Ptr< ZipArchive > & | FindZipArchive (const URI &zipUri) |
find a zip archive by name, returns 0 if not exists | |
const Ptr< ZipArchive > & | FindZipArchiveWithFile (const URI &fileUri) |
find first zip archive which contains the file path | |
const Ptr< ZipArchive > & | FindZipArchiveWithDir (const URI &dirUri) |
find first zip archive which contains the directory path | |
URI | ConvertFileToZipURIIfExists (const URI &uri) |
transparently convert a URI pointing to a file into a matching zip URI | |
URI | ConvertDirToZipURIIfExists (const URI &uri) |
transparently convert a URI pointing to a directory into a matching zip URI | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
Ptr< ZipArchive > IO::ZipFileSystem::Mount | ( | const URI & | uri | ) |
"mount" a zip archive
This "mounts" a zip file of the given filename by creating a ZipArchive object and reading the zip file structure. If opening the zip archive fails, 0 will be returned.
void IO::ZipFileSystem::Unmount | ( | const URI & | uri | ) |
"unmount" a zip archive by its file name
Unmount a zip archive by zip filename.
void IO::ZipFileSystem::Unmount | ( | const Ptr< ZipArchive > & | zipArchive | ) |
"unmount a zip archive by pointer
Unmount a zip archive, this will close the ZipArchive object and remove it from the internal registry.
const Ptr< ZipArchive > & IO::ZipFileSystem::FindZipArchive | ( | const URI & | uri | ) |
find a zip archive by name, returns 0 if not exists
Resolve a zip archive path into a ZipArchive pointer. Returns 0 if no archive with that name exists. The filename will be resolved into an absolute path internally before the lookup happens.
const Ptr< ZipArchive > & IO::ZipFileSystem::FindZipArchiveWithFile | ( | const URI & | uri | ) |
find first zip archive which contains the file path
This method takes a normal file URI and checks if the local path of the URI is contained as file entry in any mounted zip archive. If yes the name of the zip archive is returned, otherwise a 0 pointer. NOTE: if the same path resides in several zip archives, it is currently not defined which one will be returned (the current implementation returns the first zip archive in alphabetical order which contains the file).
const Ptr< ZipArchive > & IO::ZipFileSystem::FindZipArchiveWithDir | ( | const URI & | uri | ) |
find first zip archive which contains the directory path
Same as FindZipArchiveWithFile(), but checks for a directory entry in a zip file.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.