BaseGameFeature::CategoryManager Class Reference
#include <categorymanager.h>
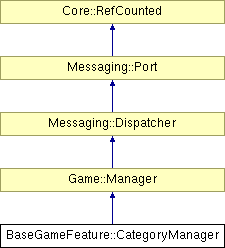
Detailed Description
Wraps entity categories and provides access to category template and instance tables.(C) 2007 Radon Labs GmbH
Public Member Functions | |
CategoryManager () | |
constructor | |
virtual | ~CategoryManager () |
destructor | |
virtual void | OnActivate () |
called when attached to game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
void | CommitChangesToDatabase () |
commit changes back into database | |
void | LoadInstances (const Util::String &levelName) |
load all instances with the given level attribute | |
Util::Array< Ptr < Db::Dataset > > | FindInstances (const Util::String &levelName) |
find all instances with the given level attribute | |
Entry | CreateDummyInstance () |
create a dummy instance which will never be saved to the database | |
Entry | CreateInstanceFromTemplate (const Util::String &categoryName, const Util::String &id) |
create a new instance from a template | |
Entry | CreateInstanceFromTemplateAsCategory (const Util::String &categoryName, const Util::String &id, const Util::String &targetCategory) |
create a new instance from a template as a different category | |
Entry | CreateInstanceFromAttrs (const Util::String &categoryName, const Util::Array< Attr::Attribute > &attrs) |
create a new instance from a template and override attributes | |
Entry | CreateInstanceFromInstance (const Entry &source) |
create a new instance as a copy from another instance of the same category | |
Entry | CreateInstanceFromInstanceAsCategory (const Entry &source, const Util::String &targetCategory, bool createMissingAttributes=false) |
create a new instance as a copy from another instance, but in a different category | |
Util::Array< Entry > | GetInstancesByAttr (const Attr::Attribute &attrs, bool loadedOnly, bool firstMatchOnly) |
find and/or create new instance by matching a key attribute, this method can be quite slow! | |
Util::Array< Entry > | GetInstancesByAttrs (const Util::Array< Attr::Attribute > &attrs, bool loadedOnly, bool firstMatchOnly) |
find and/or create new instance by matching a key attribute, this method can be quite slow! | |
void | DeleteInstance (const Entry &source) |
delete an instance identified by GUID | |
int | GetNumInstances () const |
get current overall number of instances | |
void | SetInstanceEntity (const Entry &instance, const Ptr< Game::Entity > &entity) |
bind entity pointer to instance | |
Ptr< Game::Entity > | GetInstanceEntity (const Entry &instance) const |
get instance entity pointer (can be 0!) | |
bool | HasCategory (const Util::String &categoryName) const |
return true if a category exists | |
SizeT | GetNumCategories () const |
get number of categories | |
const Category & | GetCategoryByIndex (IndexT index) const |
access to category attributes by index | |
const Category & | GetCategoryByName (const Util::String &categoryName) const |
access to category attributes by name | |
void | BeginAddCategoryAttrs (const Util::String &categoryName) |
begin adding category attributes | |
void | AddCategoryAttr (const Attr::AttrId &attrId) |
add a category attribute | |
void | EndAddCategoryAttrs () |
end adding category attributes | |
bool | HasTemplateTable (const Util::String &categoryName) const |
return true if a template table for a category exists | |
const Ptr < Db::ValueTable > & | GetTemplateTable (const Util::String &categoryName) const |
get template value table by category name | |
Entry | FindTemplate (const Util::String &categoryName, const Util::String &id) const |
find a template entry by category name and id | |
Entry | FindTemplateByAttr (const Attr::Attribute &attr, const Util::String &categoryName="") const |
find a single template by attribute | |
bool | HasInstanceTable (const Util::String &categoryName) const |
return true if an instance table for a category exists | |
const Ptr < Db::ValueTable > & | GetInstanceTable (const Util::String &categoryName) const |
get instance value table by category name | |
void | DuplicateLevel (const Util::String &levelName, const Util::String &duplicateName) |
duplicates a complete level in the databes | |
void | DeleteLevel (const Util::String &levelName) |
deletes a complete level from database | |
void | RenameLevel (const Util::String &levelName, const Util::String &newLevelName) |
renames a complete level in the database | |
bool | LevelExists (const Util::String &levelName) |
return true if level is found in _Instance_Levels table | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array < const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message | |
Data Structures | |
class | Category |
describes a category More... | |
class | Entry |
describes an entry in a value table More... |
Member Function Documentation
void BaseGameFeature::CategoryManager::OnActivate | ( | ) | [virtual] |
called when attached to game server
NOTE: The category manager must be re-activated when the world database is opened so that it may read the categories from the world database.
Reimplemented from Game::Manager.
void BaseGameFeature::CategoryManager::OnDeactivate | ( | ) | [virtual] |
void BaseGameFeature::CategoryManager::CommitChangesToDatabase | ( | ) |
commit changes back into database
This commits changes to the instance data back into the database. Call this method right before creating a save game.
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateDummyInstance | ( | ) |
create a dummy instance which will never be saved to the database
Create an instance in the dummy category, this is for entities which are created as helper entities and should never show up in the database (i.e. the global environment entity).
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateInstanceFromTemplate | ( | const Util::String & | categoryName, | |
const Util::String & | id | |||
) |
create a new instance from a template
This creates a new instance from a category name and id and returns its location.
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateInstanceFromTemplateAsCategory | ( | const Util::String & | categoryName, | |
const Util::String & | id, | |||
const Util::String & | targetCategory | |||
) |
create a new instance from a template as a different category
This creates a new instance from a category name and id, but puts the instance into a different category's instance table.
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateInstanceFromAttrs | ( | const Util::String & | categoryName, | |
const Util::Array< Attr::Attribute > & | attrs | |||
) |
create a new instance from a template and override attributes
This creates a new instance from scratch and fills it with the provided attributes.
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateInstanceFromInstance | ( | const Entry & | source | ) |
create a new instance as a copy from another instance of the same category
This creates a new instance as a copy of another instance of the same category.
CategoryManager::Entry BaseGameFeature::CategoryManager::CreateInstanceFromInstanceAsCategory | ( | const Entry & | source, | |
const Util::String & | targetCategory, | |||
bool | createMissingAttributes = false | |||
) |
create a new instance as a copy from another instance, but in a different category
This creates a new instance in a different category as a copy of another instance.
Util::Array< CategoryManager::Entry > BaseGameFeature::CategoryManager::GetInstancesByAttr | ( | const Attr::Attribute & | attrs, | |
bool | loadedOnly, | |||
bool | firstMatchOnly | |||
) |
find and/or create new instance by matching a key attribute, this method can be quite slow!
Create new instances by a matching attribute. This method can do several database access, and thus can be slow if the requested attribute doesn't map to an indexed database column.
If you know that there is only one instance in the database, set the firstMatchOnly parameter to true to avoid unessecary database accesses.
Matching instances which already loaded will not be correctly ignored, this is done by checking against the primary attribute (Attr::Guid) of the table.
Util::Array< CategoryManager::Entry > BaseGameFeature::CategoryManager::GetInstancesByAttrs | ( | const Util::Array< Attr::Attribute > & | attrs, | |
bool | loadedOnly, | |||
bool | firstMatchOnly | |||
) |
find and/or create new instance by matching a key attribute, this method can be quite slow!
Create new instances by a multiple matching attributes.
void BaseGameFeature::CategoryManager::DeleteInstance | ( | const Entry & | entry | ) |
delete an instance identified by GUID
This deletes an instance from the database.
int BaseGameFeature::CategoryManager::GetNumInstances | ( | ) | const |
get current overall number of instances
This returns the overall number of loaded instances.
void BaseGameFeature::CategoryManager::SetInstanceEntity | ( | const Entry & | instance, | |
const Ptr< Game::Entity > & | entity | |||
) |
bind entity pointer to instance
Associate a game entity pointer with an instance. This uses the ValueTable's per-row user data field to store the pointer.
Ptr< Game::Entity > BaseGameFeature::CategoryManager::GetInstanceEntity | ( | const Entry & | instance | ) | const |
get instance entity pointer (can be 0!)
Get the game entity pointer associated with an instance.
void BaseGameFeature::CategoryManager::BeginAddCategoryAttrs | ( | const Util::String & | categoryName | ) |
begin adding category attributes
Begin adding category attributes.
void BaseGameFeature::CategoryManager::AddCategoryAttr | ( | const Attr::AttrId & | attrId | ) |
add a category attribute
This adds an attribute to a category. Will extend the template and instance table of the category by the new attribute and update the attr/category mapping table.
void BaseGameFeature::CategoryManager::EndAddCategoryAttrs | ( | ) |
end adding category attributes
End adding attributes to a category.
CategoryManager::Entry BaseGameFeature::CategoryManager::FindTemplateByAttr | ( | const Attr::Attribute & | attr, | |
const Util::String & | categoryName = "" | |||
) | const |
find a single template by attribute
Find the first template which has the matching attribute. If categoryName is given, only search in this category, otherwise in all categories which have the attribute.
WARNING: this method does linear searches on the value tables and thus can be slow.
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.