Game::Property Class Reference
#include <property.h>
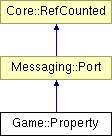
Detailed Description
Properties are attached to game entities to add specific functionality or behaviours to the entity. For instance, you add a GraphicsProperty if the entity should be able render itself, or you add an AudioProperty if the entity should be able to emit sound. Properties are derived from the Messaging::Port class and as such may receive and handle messages but they are not required to do so.(C) 2007 Radon Labs GmbH
Public Types | |
enum | CallbackType |
callback types | |
Public Member Functions | |
Property () | |
constructor | |
virtual | ~Property () |
destructor | |
const Ptr< Entity > & | GetEntity () const |
get entity this property is attached to | |
bool | HasEntity () const |
return true if entity pointer is valid | |
virtual void | SetupDefaultAttributes () |
setup the property's attributes to their default state | |
virtual void | SetupCallbacks () |
setup callbacks for this property, call by entity in OnActivate() | |
virtual void | OnActivate () |
called from Entity::ActivateProperties() | |
virtual void | OnDeactivate () |
called from Entity::DeactivateProperties() | |
bool | IsActive () const |
return true if property is currently active | |
virtual void | OnLoad () |
called from within Entity::Load() after attributes are loaded | |
virtual void | OnStart () |
called from within Entity::OnStart() after OnLoad when the complete world exist | |
virtual void | OnSave () |
called from within Entity::Save() before attributes are saved back to database | |
virtual void | OnBeginFrame () |
called on begin of frame | |
virtual void | OnMoveBefore () |
called before movement happens | |
virtual void | OnMoveAfter () |
called after movement has happened | |
virtual void | OnRender () |
called before rendering happens | |
virtual void | OnRenderDebug () |
called when game debug visualization is on | |
virtual void | OnLoseActivity () |
called when game debug visualization is on | |
virtual void | OnGainActivity () |
called when game debug visualization is on | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array < const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single accepted message | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetEntity (const Ptr< Entity > &v) |
Set entity, this is attached to, to `v'. | |
void | ClearEntity () |
Remove entity. | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void Game::Property::SetupDefaultAttributes | ( | ) | [virtual] |
setup the property's attributes to their default state
If a property adds attributes to an entity, override this method to setup their default state. This method is called before the entity is even initialized from the database. After this method, entity attributes may be overwritten from the database, and after that from a stream.
void Game::Property::SetupCallbacks | ( | ) | [virtual] |
setup callbacks for this property, call by entity in OnActivate()
Tells the entity what per-frame callback methods should be called for this property. The method is called after SetupDefaultAttributes() by the entity, and the property is expected to call the Entity::RegisterPropertyCallback() once for every callback method (OnBeginFrame(), OnMoveBefore(), ...) that should be called per-frame.
void Game::Property::OnActivate | ( | ) | [virtual] |
called from Entity::ActivateProperties()
This method is called by Game::Entity::ActivateProperties(). Use this method for one-time initializations of the property.
void Game::Property::OnDeactivate | ( | ) | [virtual] |
called from Entity::DeactivateProperties()
This method is called by Game::Entity::DeactivateProperties(). Use this method to cleanup stuff which has been initialized in OnActivate().
void Game::Property::OnLoad | ( | ) | [virtual] |
called from within Entity::Load() after attributes are loaded
This method is called from within Game::Entity::Load() after the entity attributes have been loaded from the database. You can override this method in a subclass if further initialization is needed for the property after attributes have been loaded from the database, but please be aware that this method may not be called if the entity is created directly.
void Game::Property::OnStart | ( | ) | [virtual] |
called from within Entity::OnStart() after OnLoad when the complete world exist
This method is called from within Game::Entity::OnStart(). This is the moment when the world is complete and the entity can establish connections to other entitys.
void Game::Property::OnSave | ( | ) | [virtual] |
called from within Entity::Save() before attributes are saved back to database
This method is called from within Game::Entity::Save() before the entity attributes will be saved to the database. You can override this method in a subclass if actions are needed before a save happens (this is usually the case if entity attributes need to be updated by the property before saving).
void Game::Property::OnBeginFrame | ( | ) | [virtual] |
called on begin of frame
This method is called from Game::Entity::OnBeginFrame() on all properties attached to an entity in the order of attachment. Override this method if your property has to do any work at the beginning of the frame.
void Game::Property::OnMoveBefore | ( | ) | [virtual] |
called before movement happens
This method is called from Game::Entity::OnMoveBefore() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do before the physics subsystem is triggered.
void Game::Property::OnMoveAfter | ( | ) | [virtual] |
called after movement has happened
This method is called from Game::Entity::OnMoveAfter() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do after the physics subsystem has been triggered.
void Game::Property::OnRender | ( | ) | [virtual] |
called before rendering happens
This method is called from Game::Entity::OnRender() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do before rendering happens.
void Game::Property::OnRenderDebug | ( | ) | [virtual] |
called when game debug visualization is on
This method is called from Game::Entity::OnRenderDebug() on all properties attached to an entity in the order of attachment. It's meant for debug issues. It will be called when debug mode is enabled.
void Game::Property::OnLoseActivity | ( | ) | [virtual] |
called when game debug visualization is on
This method is called from Game::Entity::OnLoseActivity() on all properties attached to an entity in the order of attachment. It indicates that the entity will no longer be trigger, due to leaving the "Activity Bubble", i.e. the area of interest (most probably around the active camera).
void Game::Property::OnGainActivity | ( | ) | [virtual] |
called when game debug visualization is on
This method is called from Game::Entity::OnRenderDebug() on all properties attached to an entity in the order of attachment. It indicates that the entity will be trigger from now on, due to entering the "Activity Bubble", i.e. the area of interest (most probably around the active camera).
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
void Messaging::Port::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single accepted message
Handle a specific message. Overwrite this method in a subclass. It is guaranteed that this method will only be called for messages which are accepted by AcceptMessage().
Reimplemented in Messaging::Dispatcher.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.