Input::Mouse Class Reference
#include <mouse.h>
Inheritance diagram for Input::Mouse:
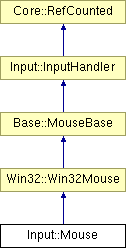
Detailed Description
An input handler which represents a mouse for polling.(C) 2007 Radon Labs GmbH
Public Member Functions | |
const Math::float2 & | GetMovement () const |
get mouse movement | |
virtual void | BeginCapture () |
capture input to this event handler | |
virtual void | EndCapture () |
end input capturing to this event handler | |
bool | ButtonPressed (Input::MouseButton::Code btn) const |
return true if button is currently pressed | |
bool | ButtonDown (Input::MouseButton::Code btn) const |
return true if button was down at least once in current frame | |
bool | ButtonUp (Input::MouseButton::Code btn) const |
return true if button was up at least once in current frame | |
bool | ButtonDoubleClicked (Input::MouseButton::Code btn) const |
return true if a button has been double clicked | |
bool | WheelForward () const |
return true if mouse wheel rotated forward | |
bool | WheelBackward () const |
return true if mouse wheel rotated backward | |
const Math::float2 & | GetPixelPosition () const |
get current absolute mouse position (in pixels) | |
const Math::float2 & | GetScreenPosition () const |
get current screen space mouse position (0.0 .. 1.0) | |
bool | IsAttached () const |
return true if the input handler is currently attached | |
bool | IsCapturing () const |
return true if this input handler captures input | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | OnAttach () |
called when the handler is attached to the input server | |
virtual void | OnBeginFrame () |
called on InputServer::BeginFrame() | |
virtual bool | OnEvent (const Input::InputEvent &inputEvent) |
called when an input event should be processed | |
virtual void | OnObtainCapture () |
called when input handler obtains capture | |
virtual void | OnReleaseCapture () |
called when input handler looses capture | |
virtual void | OnReset () |
reset the input handler | |
virtual void | OnRemove () |
called when the handler is removed from the input server | |
virtual void | OnEndFrame () |
called on InputServer::EndFrame(); |
Member Function Documentation
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.