Models::SkinShapeNode Class Reference
#include <skinshapenode.h>
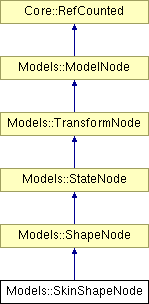
Detailed Description
A ModelNode which describes a skinned shape. Since the SkinShapeNode is derived from the ShapeNode (which in turn is derived from the TransformNode), a SkinShapeNode contains all the necessary information for rendering a skinned mesh.It supports the use of variations and skinlists for multi exchangeable animated skins and textures (all the nebula2 character3 functionalities).
(C) 2007 Radon Labs GmbH
Public Member Functions | |
SkinShapeNode () | |
constructor | |
virtual | ~SkinShapeNode () |
destructor | |
virtual Ptr < ModelNodeInstance > | CreateNodeInstance () const |
create a model node instance | |
virtual void | ApplySharedState () |
apply state shared by all my SkinShapeNodeInstances | |
void | BeginFragments (int num) |
begin defining mesh fragments | |
void | SetFragGroupIndex (int fragIndex, int primGroupIndex) |
set mesh group index of a skin fragment | |
int | GetFragGroupIndex (int fragIndex) const |
get mesh group index of a skin fragment | |
void | BeginJointPalette (int fragIndex, int numJoints) |
begin defining the joint palette of a fragment | |
void | SetJointIndices (int fragIndex, int paletteIndex, int ji0, int ji1, int ji2, int ji3, int ji4, int ji5, int ji6, int ji7) |
add up to 8 joint indices to the fragments joint palette | |
void | SetJointIndex (int fragIndex, int paletteIndex, int jointIndex) |
add a single joint index to the fragments joint palette | |
void | EndJointPalette (int fragIndex) |
finish adding joints to the joint palette | |
void | EndFragments () |
finish defining fragments | |
int | GetNumFragments () const |
get number of fragments | |
const Util::FixedArray < Char::CharFragment > & | GetFragmentArray () const |
get fragment array | |
int | GetJointPaletteSize (int fragIndex) const |
get number of joints in a fragment's joint palette | |
int | GetJointIndex (int fragIndex, int paletteIndex) const |
get a joint index from a fragment's joint palette | |
void | RequestLoadResources () |
request load resources, if not loaded yet | |
void | RequestUnloadResources () |
request to unload our resources | |
virtual Resources::Resource::State | GetResourceState () const |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled) | |
const Ptr < Resources::ManagedMesh > & | GetManagedMesh () const |
get managed mesh | |
const Ptr < CoreGraphics::ShaderInstance > & | GetShaderInstance () const |
get pointer to contained shader instance | |
void | SetPosition (const Math::point &p) |
set position | |
const Math::point & | GetPosition () const |
get position | |
void | SetRotate (const Math::quaternion &r) |
set rotate quaternion | |
const Math::quaternion & | GetRotate () const |
get rotate quaternion | |
void | SetScale (const Math::vector &s) |
set scale | |
const Math::vector & | GetScale () const |
get scale | |
void | SetRotatePivot (const Math::point &p) |
set rotate pivot | |
const Math::point & | GetRotatePivot () const |
get rotate pivot | |
void | SetScalePivot (const Math::point &p) |
set scale pivot | |
const Math::point & | GetScalePivot () const |
get scale pivot | |
const Util::Atom < Util::String > & | GetName () const |
get model node name | |
ModelNodeType::Code | GetType () const |
get the ModelNodeType | |
bool | HasParent () const |
return true if node has a parent | |
const Ptr< ModelNode > & | GetParent () const |
get parent node | |
const Util::Array < Ptr< ModelNode > > & | GetChildren () const |
get child nodes | |
bool | IsAttachedToModel () const |
return true if currently attached to a Model | |
const Ptr< Model > & | GetModel () const |
get model this node is attached to | |
const Math::bbox & | GetBoundingBox () const |
get bounding box of model node | |
const Attr::AttributeContainer & | GetAttrs () const |
read access to model node attributes | |
bool | HasAttr (const Attr::AttrId &attrId) const |
check if model node attribute exists | |
void | SetAttr (const Attr::Attribute &attr) |
set generic model node attribute | |
const Attr::Attribute & | GetAttr (const Attr::AttrId &attrId) const |
get generic model node attribute | |
void | SetBool (const Attr::BoolAttrId &attrId, bool val) |
set bool value | |
bool | GetBool (const Attr::BoolAttrId &attrId) const |
get bool value | |
void | SetFloat (const Attr::FloatAttrId &attrId, float val) |
set float value | |
float | GetFloat (const Attr::FloatAttrId &attrId) const |
get float value | |
void | SetInt (const Attr::IntAttrId &attrId, int val) |
set int value | |
int | GetInt (const Attr::IntAttrId &attrId) const |
get int value | |
void | SetString (const Attr::StringAttrId &attrId, const Util::String &val) |
set string value | |
const Util::String & | GetString (const Attr::StringAttrId &attrId) const |
get string value | |
void | SetFloat4 (const Attr::Float4AttrId &attrId, const Math::float4 &val) |
set float4 value | |
Math::float4 | GetFloat4 (const Attr::Float4AttrId &attrId) const |
get float4 value | |
void | SetMatrix44 (const Attr::Matrix44AttrId &attrId, const Math::matrix44 &val) |
set matrix44 value | |
const Math::matrix44 & | GetMatrix44 (const Attr::Matrix44AttrId &attrId) const |
get matrix44 value | |
void | SetGuid (const Attr::GuidAttrId &attrId, const Util::Guid &guid) |
set guid value | |
const Util::Guid & | GetGuid (const Attr::GuidAttrId &attrId) const |
get guid value | |
void | SetBlob (const Attr::BlobAttrId &attrId, const Util::Blob &blob) |
set blob value | |
const Util::Blob & | GetBlob (const Attr::BlobAttrId &attrId) const |
get blob value | |
void | SetName (const Util::Atom< Util::String > &n) |
set model node name | |
void | SetType (ModelNodeType::Code t) |
set ModelNodeType | |
void | SetParent (const Ptr< ModelNode > &p) |
set parent node | |
void | AddChild (const Ptr< ModelNode > &c) |
add a child node | |
void | AddVisibleNodeInstance (IndexT frameIndex, const Ptr< ModelNodeInstance > &nodeInst) |
called by model node instance on NotifyVisible() | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | LoadResources () |
called when resources should be loaded | |
virtual void | UnloadResources () |
called when resources should be unloaded | |
void | SetupManagedTextureVariable (const Attr::AttrId &resAttrId, const Ptr< CoreGraphics::ShaderVariable > &var) |
setup a new managed texture variable | |
void | UpdateManagedTextureVariables () |
update managed texture variables | |
virtual void | LoadFromAttrs (const Attr::AttributeContainer &attrs) |
called to initialize from attributes | |
virtual void | SaveToAttrs (Attr::AttributeContainer &attrs) |
called to save state back to attributes | |
void | SetBoundingBox (const Math::bbox &b) |
set bounding box | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to model node | |
virtual void | OnRemoveFromModel () |
called when removed from model node | |
const Util::Array < Ptr < ModelNodeInstance > > & | GetVisibleModelNodeInstances (ModelNodeType::Code t) const |
get visible model node instances |
Member Function Documentation
void Models::SkinShapeNode::ApplySharedState | ( | ) | [virtual] |
apply state shared by all my SkinShapeNodeInstances
This method is called once before rendering the ModelNode's visible instance nodes through the ModelNodeInstance::ApplyState() and ModelNodeInstance::Render() methods. The method must apply the shader state that is shared across all instances. Since this state is constant across all instance nodes, this only happens once before rendering an instance set.
Reimplemented from Models::ShapeNode.
void Models::SkinShapeNode::BeginFragments | ( | int | num | ) |
begin defining mesh fragments
Begin defining mesh fragment. A skin mesh may be divided into several fragments to account for gfx hardware which an only render a skinned mesh with a limited number of influence objects (joints).
void Models::SkinShapeNode::SetFragGroupIndex | ( | int | fragIndex, | |
int | primGroupIndex | |||
) |
set mesh group index of a skin fragment
Set the mesh group index for a skin fragment.
int Models::SkinShapeNode::GetFragGroupIndex | ( | int | fragIndex | ) | const |
get mesh group index of a skin fragment
Get the mesh group index for a skin fragment.
void Models::SkinShapeNode::BeginJointPalette | ( | int | fragIndex, | |
int | numJoints | |||
) |
begin defining the joint palette of a fragment
Begin defining the joint palette of a skin fragment.
void Models::SkinShapeNode::SetJointIndices | ( | int | fragIndex, | |
int | paletteIndex, | |||
int | ji0, | |||
int | ji1, | |||
int | ji2, | |||
int | ji3, | |||
int | ji4, | |||
int | ji5, | |||
int | ji6, | |||
int | ji7 | |||
) |
add up to 8 joint indices to the fragments joint palette
Add up to 8 joints to a fragments joint palette starting at a given palette index.
void Models::SkinShapeNode::EndJointPalette | ( | int | fragIndex | ) |
finish adding joints to the joint palette
Finish defining the joint palette of a skin fragment.
void Models::SkinShapeNode::EndFragments | ( | ) |
finish defining fragments
Finish defining fragments.
int Models::SkinShapeNode::GetNumFragments | ( | ) | const [inline] |
get number of fragments
Get number of fragments.
const Util::FixedArray< Char::CharFragment > & Models::SkinShapeNode::GetFragmentArray | ( | ) | const [inline] |
get fragment array
Get fragments.
int Models::SkinShapeNode::GetJointPaletteSize | ( | int | fragIndex | ) | const [inline] |
get number of joints in a fragment's joint palette
Get joint palette size of a skin fragment.
int Models::SkinShapeNode::GetJointIndex | ( | int | fragIndex, | |
int | paletteIndex | |||
) | const [inline] |
get a joint index from a fragment's joint palette
Get a joint index from a fragment's joint index.
void Models::SkinShapeNode::RequestLoadResources | ( | ) |
request load resources, if not loaded yet
Is call by instances which are getting visible.
void Models::SkinShapeNode::RequestUnloadResources | ( | ) |
request to unload our resources
Request an unload of our resources, if no instance node references out resource, we try to unload it
void Models::SkinShapeNode::LoadResources | ( | ) | [protected, virtual] |
called when resources should be loaded
Do not load resources by default.
Reimplemented from Models::ShapeNode.
void Models::StateNode::SetupManagedTextureVariable | ( | const Attr::AttrId & | resAttrId, | |
const Ptr< CoreGraphics::ShaderVariable > & | var | |||
) | [protected, inherited] |
setup a new managed texture variable
Create a new managed texture resource and bind it to the provided shader variable.
void Models::StateNode::UpdateManagedTextureVariables | ( | ) | [protected, inherited] |
update managed texture variables
This method transfers texture from our managed texture objects into their associated shader variable. This is necessary since the actual texture of a managed texture may change from frame to frame because of resource management.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.