Lighting::GlobalLightEntity Class Reference
#include <globallightentity.h>
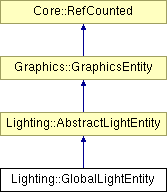
Detailed Description
Implements a global directional light intended for outdoor areas or as the basis for indoor rendering. Besides the primary directional light component, a global light also usually provides some sort of ambient component for areas in shadow. There should only be one GlobalLight active during rendering a frame. The primary light is directed along the negative z-axis of the light's transform matrix. Scaling and position has no effect on the global light.(C) 2007 Radon Labs GmbH
Public Types | |
enum | Type |
entity types | |
enum | LinkType |
visibility link types | |
Public Member Functions | |
GlobalLightEntity () | |
constructor | |
virtual Math::ClipStatus::Type | ComputeClipStatus (const Math::bbox &box) |
compute clip status against bounding box | |
void | SetBackLightColor (const Math::float4 &c) |
set the backlight color | |
const Math::float4 & | GetBackLightColor () const |
get the backlight color | |
const Math::vector & | GetLightDirection () const |
get the light direction (extracted from negative z-axis of transformation matrix) | |
LightType::Code | GetLightType () const |
get the light type | |
void | SetColor (const Math::float4 &c) |
set primary light color | |
const Math::float4 & | GetColor () const |
get primary light color | |
void | SetProjMapUvOffsetAndScale (const Math::float4 &v) |
set projection map UV offset and scale (xy->offset, zw->scale) | |
const Math::float4 & | GetProjMapUvOffsetAndScale () const |
get projection map UV offset and scale | |
void | SetCastShadows (bool b) |
enable/disable shadow casting | |
bool | GetCastShadows () const |
get shadow casting flag | |
const Math::matrix44 & | GetInvTransform () const |
get inverse transform (transforms from world to light space) | |
const Math::matrix44 & | GetProjTransform () const |
get light-projection matrix (transforms from light space to light projection space) | |
const Math::matrix44 & | GetInvLightProjTransform () const |
get world-to-light-projection transform (transform from world to light projection space) | |
void | SetShadowBufferUvOffsetAndScale (const Math::float4 &uvOffset) |
set shadow buffer uv rectangle (optionally set by light/shadow servers) | |
const Math::float4 & | GetShadowBufferUvOffsetAndScale () const |
get shadow buffer uv rectangle | |
bool | IsActive () const |
return true if entity is currently active (is between OnActivate()/OnDeactivate() | |
bool | IsValid () const |
return true if entity is current valid (ready for rendering) | |
Type | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< Stage > & | GetStage () const |
get the stage this entity is attached to | |
bool | IsAttachedToStage () const |
return true if entity is attached to stage | |
const Ptr< Cell > & | GetCell () const |
get the cell this entity is attached to | |
bool | IsAttachedToCell () const |
return true if entity is attached to cell | |
const Math::bbox & | GetLocalBoundingBox () const |
get the local space bounding box | |
const Math::bbox & | GetGlobalBoundingBox () |
get bounding box in global space | |
void | ClearLinks (LinkType linkType) |
clear all visibility links | |
void | AddLink (LinkType linkType, const Ptr< GraphicsEntity > &entity) |
add visibility link | |
const Util::Array < Ptr < GraphicsEntity > > & | GetLinks (LinkType type) const |
get visibility links by type | |
void | SetTime (Timing::Time t) |
set graphics time | |
Timing::Time | GetTime () const |
get graphics time | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | OnTransformChanged () |
called when transform matrix changed | |
void | SetLightType (LightType::Code c) |
set the light type (must be called from sub-classes constructor | |
void | SetType (Type t) |
set entity type, call in constructor of derived class! | |
void | SetLocalBoundingBox (const Math::bbox &b) |
set the local space bounding box | |
void | SetValid (bool b) |
set to valid state (when the entity becomes ready for rendering) | |
virtual Ptr < GraphicsEntity > | CreateClone () const |
create a clone of the entity | |
virtual void | OnActivate () |
called when entity is created | |
virtual void | OnDeactivate () |
called before entity is destroyed | |
virtual void | OnAttachToStage (const Ptr< Stage > &stage) |
called when attached to Stage | |
virtual void | OnRemoveFromStage () |
called when removed from Stage | |
virtual void | OnAttachToCell (const Ptr< Cell > &cell) |
called when attached to a Cell | |
virtual void | OnRemoveFromCell () |
called when removed from a Cell | |
virtual void | OnShow () |
called when the entity becomes visible | |
virtual void | OnHide () |
called when the entity becomes invisible | |
virtual void | OnUpdate () |
called to update the entity before rendering | |
virtual void | OnRender () |
called before the entity is rendered | |
virtual void | OnRenderDebug () |
called to render a debug visualization of the entity | |
void | UpdatePositionInCellTree () |
update our position in the cell hierarchy | |
void | UpdateGlobalBoundingBox () |
update the global bounding box from the transform and local box |
Member Function Documentation
Ptr< GraphicsEntity > Graphics::GraphicsEntity::CreateClone | ( | ) | const [protected, virtual, inherited] |
create a clone of the entity
This method is called to create an exact clone of this graphics entity. The new entity will not be attached to a stage.
void Graphics::GraphicsEntity::OnActivate | ( | ) | [protected, virtual, inherited] |
called when entity is created
Activate the entity. This method is called when the entity is created an attached to the graphics server. During OnActivate() the entity should perform any one-time initializations.
Reimplemented in Graphics::ActorEntity, and Graphics::ModelEntity.
void Graphics::GraphicsEntity::OnDeactivate | ( | ) | [protected, virtual, inherited] |
called before entity is destroyed
Deactivate the entity, this method is called when the entity is removed from the graphics server. Any initialization done in OnActivate() should be undone here.
Reimplemented in Graphics::ActorEntity, Graphics::CameraEntity, and Graphics::ModelEntity.
void Graphics::GraphicsEntity::OnAttachToStage | ( | const Ptr< Stage > & | s | ) | [protected, virtual, inherited] |
called when attached to Stage
This method is called when the graphics entity is attached to a stage. An entity may only be attached to one stage at any time, but can be attached to different stages during its lifetime. Attachging an entity to a stage may be relatively slow because the entity must be inserted into the cell hierarchy.
void Graphics::GraphicsEntity::OnRemoveFromStage | ( | ) | [protected, virtual, inherited] |
called when removed from Stage
This method is called when the graphics entity is removed from a stage.
void Graphics::GraphicsEntity::OnRemoveFromCell | ( | ) | [protected, virtual, inherited] |
called when removed from a Cell
Called when the graphics entity is removed from a cell inside a stage.
void Graphics::GraphicsEntity::OnShow | ( | ) | [protected, virtual, inherited] |
called when the entity becomes visible
This method is called from the SetVisible() method when the entity changes from invisible to visible state.
void Graphics::GraphicsEntity::OnHide | ( | ) | [protected, virtual, inherited] |
called when the entity becomes invisible
This method is called from the SetVisible() method when the entity changes from visible to invisible state.
void Graphics::GraphicsEntity::OnUpdate | ( | ) | [protected, virtual, inherited] |
called to update the entity before rendering
This method is called on the graphics entity to update itself, for instance to implement hierarchy animation or particle updates.
Reimplemented in Graphics::ActorEntity, and Graphics::ModelEntity.
void Graphics::GraphicsEntity::OnRender | ( | ) | [protected, virtual, inherited] |
called before the entity is rendered
This method is called on the graphics entity to render itself. This method will only be called if the entity is visible through the camera of the currently rendered View.
void Graphics::GraphicsEntity::OnRenderDebug | ( | ) | [protected, virtual, inherited] |
called to render a debug visualization of the entity
This method is called on the graphics entity to render a debug visualization of itself.
Reimplemented in Graphics::ModelEntity.
void Graphics::GraphicsEntity::UpdatePositionInCellTree | ( | ) | [protected, inherited] |
update our position in the cell hierarchy
This method is called from OnUpdate() when either the entity's transformation or bounding box has changed. It checks if the entity still fits into its current cell, and if not, moves the entity in a new cell.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.