BaseGameFeature::EntityManager Class Reference
#include <entitymanager.h>
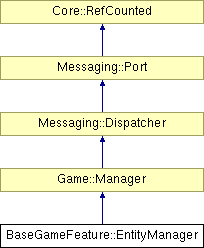
Detailed Description
The entity manager object keeps track of all active game entities and calls their per-frame-update methods to keep them alive. It also contains methods to iterate through existing entities. Derive from this class if your application needs different or more advanced game entity management, but make sure that all methods which are defined in entity manager still do the expected thing in your derived class.(C) 2007 Radon Labs GmbH
Public Member Functions | |
EntityManager () | |
constructor | |
virtual | ~EntityManager () |
destructor | |
void | SetMaxTriggerDistance (float d) |
TEMP HACK: set the maximum-trigger-distance (default is 100 meters). | |
float | GetMaxTriggerDistance () const |
TEMP HACK: get the maximum-trigger-distance. | |
virtual void | OnDeactivate () |
called when removed from game server | |
void | OnStart () |
called on start by game server | |
virtual void | OnBeginFrame () |
called per-frame by game server | |
virtual void | OnEndFrame () |
called per-frame by game server | |
virtual void | OnSave () |
called before saving game state | |
void | OnRenderDebug () |
called if a render debug visulalization is requested | |
void | Cleanup () |
cleanup the entity manager, removes/deactivates all entities | |
bool | HasActiveEntities () const |
return true if at least one active entity exists in the world | |
const Util::Array < Ptr< Game::Entity > > & | GetEntities () const |
get currently active entities | |
void | AttachEntity (const Ptr< Game::Entity > &entity) |
immediately attach an entity to the world | |
void | RemoveEntity (const Ptr< Game::Entity > &entity) |
remove an entity from the world, delayed until end of frame | |
void | DeleteEntity (const Ptr< Game::Entity > &entity) |
delete an entity from the world (also deletes the entity from DB!), delayed until end of frame | |
void | RemoveEntityImmediate (const Ptr< Game::Entity > &entity) |
remove an entity from the world, calling restrictions apply | |
void | DeleteEntityImmediate (const Ptr< Game::Entity > &entity) |
delete an entity from the world (also deletes the entity from DB!), calling restrictions apply | |
bool | ExistsEntityByUniqueId (Game::Entity::EntityId id) const |
return true if a entity with the given unique id exists | |
Ptr< Game::Entity > | GetEntityByUniqueId (Game::Entity::EntityId id) const |
get the entity for the given unique id | |
bool | ExistsEntityByAttr (const Attr::Attribute &attr) const |
return true if at least one entity exists with the given attribute | |
Util::Array< Ptr < Game::Entity > > | GetEntitiesByAttr (const Attr::Attribute &attr, bool onlyFirstEntity=false) |
get the entities for the given attribute | |
Util::Array< Ptr < Game::Entity > > | GetEntitiesByAttrs (const Util::Array< Attr::Attribute > &attr, bool onlyFirstEntity=false) |
get a single entity by multiple matching attributes | |
Ptr< Game::Entity > | GetEntityByAttr (const Attr::Attribute &attr, bool failOnError=true) |
get a single entity by a single attribute | |
Ptr< Game::Entity > | GetEntityByAttrs (const Util::Array< Attr::Attribute > &attr, bool failOnError=true) |
get a single entity by multiple matching attributes | |
void | GetEntitiesInActivityBubble (Util::Array< Ptr< Game::Entity > > &outEntities) |
fill provided array with all entities inside the activity bubble | |
virtual void | OnActivate () |
called when attached to game server | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array < const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Types | |
enum | DelayedJobType |
delayed job types | |
Protected Member Functions | |
void | StartEntities () |
call OnStart() on all entities | |
void | ActivateEntity (const Ptr< Game::Entity > &entity) |
activate an entity | |
void | DeactivateEntity (const Ptr< Game::Entity > &entity) |
deactivate an entity | |
void | AddDelayedJob (DelayedJobType type, const Ptr< Game::Entity > &entity) |
add a delayed job (Remove or Delete entity) | |
void | HandleDelayedJobs () |
handle all queued up delayed jobs, called at beginning and end of frame | |
void | RemoveNullEntriesFromArrays () |
remove all null entries from the internal arrays | |
void | UpdateTriggeredEntities () |
update both arrays | |
bool | IsInFocus (const Ptr< Game::Entity > &entity, Math::point &focusEntityPos) |
check if entity is near focus entity | |
void | RemoveEntityFromTriggered (const Ptr< Game::Entity > &entity) |
remove entity from triggerd/untriggered arrays | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message | |
Data Structures | |
class | DelayedJob |
delayed jobs More... |
Member Function Documentation
void BaseGameFeature::EntityManager::OnBeginFrame | ( | ) | [virtual] |
called per-frame by game server
Handles delayed jobs, like removing entities. Calls OnBeginFrame and OnMoveBefore on all Entities. OnMoveAfter and OnRender is called in OnEndFrame of the EntityManager to allow any physics system to step its world.
Reimplemented from Game::Manager.
void BaseGameFeature::EntityManager::OnEndFrame | ( | ) | [virtual] |
called per-frame by game server
Calls OnMoveAfter and OnRender on all Entities.
Reimplemented from Game::Manager.
void BaseGameFeature::EntityManager::Cleanup | ( | ) |
cleanup the entity manager, removes/deactivates all entities
This immediately removes and deactivates all entities from the entity manager. This method is usually only called at the end of a level.
bool BaseGameFeature::EntityManager::HasActiveEntities | ( | ) | const |
return true if at least one active entity exists in the world
Return true if there is at least one active entity in the world.
const Util::Array< Ptr< Game::Entity > > & BaseGameFeature::EntityManager::GetEntities | ( | ) | const |
get currently active entities
Get the array of currently active entities.
void BaseGameFeature::EntityManager::AttachEntity | ( | const Ptr< Game::Entity > & | entity | ) |
immediately attach an entity to the world
Immediately attach (and activate) a game entity.
void BaseGameFeature::EntityManager::RemoveEntity | ( | const Ptr< Game::Entity > & | entity | ) |
remove an entity from the world, delayed until end of frame
Remove a game entity from the entity manager. This will just mark the entity as dismissed, deactivation will happen at the beginning of the next frame to prevent any data incosistencies. The entity will just function as usual for the rest of the frame.
void BaseGameFeature::EntityManager::DeleteEntity | ( | const Ptr< Game::Entity > & | entity | ) |
delete an entity from the world (also deletes the entity from DB!), delayed until end of frame
Delete an entity (remove it from the world and delete it from the database) at the beginning of the next frame.
void BaseGameFeature::EntityManager::RemoveEntityImmediate | ( | const Ptr< Game::Entity > & | entity | ) |
remove an entity from the world, calling restrictions apply
Immediately remove an entity from the entity manager. This method is more restrictive then RemoveEntity() because it must not be called from inside the game loop.
void BaseGameFeature::EntityManager::DeleteEntityImmediate | ( | const Ptr< Game::Entity > & | entity | ) |
delete an entity from the world (also deletes the entity from DB!), calling restrictions apply
Immediately delete an entity (remove from world and delete from database). This method is more restrictive then DeleteEntity() because it must not be called from inside the game loop.
Ptr< Game::Entity > BaseGameFeature::EntityManager::GetEntityByUniqueId | ( | Game::Entity::EntityId | uniqueId | ) | const |
get the entity for the given unique id
Return entity by unique id or an invalid Ptr<> if the entity doesn't exist.
bool BaseGameFeature::EntityManager::ExistsEntityByAttr | ( | const Attr::Attribute & | attr | ) | const |
return true if at least one entity exists with the given attribute
Returns true if at least one entity exists with a matching attribute.
Util::Array< Ptr< Game::Entity > > BaseGameFeature::EntityManager::GetEntitiesByAttr | ( | const Attr::Attribute & | attr, | |
bool | onlyFirstEntity = false | |||
) |
get the entities for the given attribute
Returns all entities which match a given attribute. If only the first entity is interesting (if you know that there will only one result) the onlyFirstEntity flag can be used to stop searching after the first match.
Util::Array< Ptr< Game::Entity > > BaseGameFeature::EntityManager::GetEntitiesByAttrs | ( | const Util::Array< Attr::Attribute > & | attr, | |
bool | onlyFirstEntity = false | |||
) |
get a single entity by multiple matching attributes
Returns all entities which match multiple attributes. If only the first entity is interesting (if you know that there will only one result) the onlyFirstEntity flag can be used to stop searching after the first match.
void BaseGameFeature::EntityManager::GetEntitiesInActivityBubble | ( | Util::Array< Ptr< Game::Entity > > & | outEntities | ) |
fill provided array with all entities inside the activity bubble
This updates updates the activityBubbleEntities array which contains all entities around the current viewer which should be triggered.
25-Jan-07 floh added optional per-entity trigger radius
void BaseGameFeature::EntityManager::StartEntities | ( | ) | [protected] |
void BaseGameFeature::EntityManager::DeactivateEntity | ( | const Ptr< Game::Entity > & | entity | ) | [protected] |
deactivate an entity
Deactivate an entity. This will remove the entity from the world and call its OnDeactivate() method. This is a private helper method and will be called from RemoveEntity() or RemoveDismissedEntities().
void BaseGameFeature::EntityManager::AddDelayedJob | ( | DelayedJobType | type, | |
const Ptr< Game::Entity > & | entity | |||
) | [protected] |
add a delayed job (Remove or Delete entity)
Add a new delayed job to the internal job queue. Delayed jobs are executed at the beginning of the next frame.
void BaseGameFeature::EntityManager::HandleDelayedJobs | ( | ) | [protected] |
handle all queued up delayed jobs, called at beginning and end of frame
This handles all delayed jobs. This method is executed once at the end of each frame.
void BaseGameFeature::EntityManager::RemoveNullEntriesFromArrays | ( | ) | [protected] |
remove all null entries from the internal arrays
This method should be called once at the end of the frame. It will check the internal arrays for null entries, and remove those entries. Null entries are written by the RemoveEntityImmediate() method because it may be dangerous to change array layouts while an iteration is running over the array.
void BaseGameFeature::EntityManager::UpdateTriggeredEntities | ( | ) | [protected] |
update both arrays
This updates updates the triggeredEntities/untriggeredEntities arrays that divide all entities around the current viewer in those who should be triggered and those who shouldnt be triggered.
bool BaseGameFeature::EntityManager::IsInFocus | ( | const Ptr< Game::Entity > & | curEntity, | |
Math::point & | focusEntityPos | |||
) | [protected] |
check if entity is near focus entity
Checks if an entity is "in reach" of the focus entity, i.e. within Attr::EntityTriggerRadius + this->maxTriggerDistance.
Note: will also return true on entities without transform attribute
void BaseGameFeature::EntityManager::RemoveEntityFromTriggered | ( | const Ptr< Game::Entity > & | entity | ) | [protected] |
remove entity from triggerd/untriggered arrays
Immediately remove an entity from the entity manager. This method is more restrictive then RemoveEntity() because it must not be called from inside the game loop.
void Game::Manager::OnActivate | ( | ) | [virtual, inherited] |
called when attached to game server
This method is called when the manager is attached to the game server. The manager base class will register its message port with the message server.
Reimplemented in BaseGameFeature::CategoryManager, BaseGameFeature::EnvQueryManager, and BaseGameFeature::GlobalAttrsManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.