Attr::AttributeTable Class Reference
#include <attributetable.h>
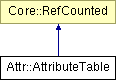
Detailed Description
A table of attributes with a compact memory footprint and fast random access. Table columns are defined by attribute ids which associate a name, a fourcc code, a datatype and an access mode (ReadWrite, ReadOnly) to the table. Attribute values are stored in one big chunk of memory without additional overhead. Table cells can have the NULL status, which means the cell contains no value.The table's value buffer consists of 4-byte aligned rows, each row consists of a bitfield with 2 bits per row (one bit is set if a column/row value is valid, the other is used as modified-marker).
The header-bitfield is padded to 4-byte. After the header field follow the value fields, one field for each column. The size of the field depends on the datatype of the column, the minimum field size of 4 bytes for data alignment reasons:
Bool: sizeof(int) usually 4 bytes Int: sizeof(int) usually 4 bytes Float: sizeof(float) usually 4 bytes Float4: sizeof(float4) usually 16 bytes Matrix44: sizeof(matrix44) usually 48 bytes String: sizeof(char*) usually 4 bytes
The AttributeTable object keeps track of all changes (added columns, added rows, modified rows, modified values).
(C) 2006 Radon Labs GmbH
Public Member Functions | |
AttributeTable () | |
constructor | |
virtual | ~AttributeTable () |
destructor | |
void | SetModifiedTracking (bool b) |
enable/disable modified tracking (default is on) | |
bool | GetModifiedTracking () const |
get modified tracking flag | |
bool | IsModified () const |
return true if the object has been modified since the last ResetModifiedState() | |
void | ResetModifiedState () |
reset all the modified bits in the table | |
void | Clear () |
clear the table object | |
void | PrintDebug () |
print the contents of the table for debugging reasons | |
void | BeginAddColumns (bool recordNewColumns=true) |
optional: call before adding columns, speeds up adding many columns at once | |
void | AddColumn (const AttrId &id, bool recordNewColumn=true) |
add a column | |
void | EndAddColumns () |
optional: call after adding columns, speeds up adding many columns at once | |
bool | HasColumn (const AttrId &id) const |
return true if a column exists | |
IndexT | GetColumnIndex (const AttrId &id) const |
return index of column by id | |
SizeT | GetNumColumns () const |
get number of columns | |
const AttrId & | GetColumnId (IndexT colIndex) const |
get column definition at index | |
const Util::String & | GetColumnName (IndexT colIndex) const |
get a column's name | |
const Util::FourCC & | GetColumnFourCC (IndexT colIndex) const |
get column FourCC | |
AccessMode | GetColumnAccessMode (IndexT colIndex) const |
get a column's access mode | |
ValueType | GetColumnValueType (IndexT colIndex) const |
get a column's value type | |
const Util::Array < IndexT > & | GetNewColumnIndices () const |
return indices of columns added since the last ResetModifiedState() | |
const Util::Array < IndexT > & | GetReadWriteColumnIndices () const |
return indices of all ReadWrite columns | |
IndexT | AddRow () |
add a row to the table, returns index of new row | |
void | ClearNewRowFlags () |
clear the new row flags, so that new rows are treated like updated rows | |
void | ClearDeletedRowsFlags () |
clear deleted rows flags | |
void | DeleteRow (IndexT rowIndex) |
mark a row as deleted from the table | |
void | DeleteAllRows () |
mark all rows as deleted | |
bool | IsRowDeleted (IndexT rowIndex) const |
return true if row has been marked as deleted | |
IndexT | CopyRow (IndexT rowIndex) |
create a new row as copy of another row | |
IndexT | CopyExtRow (AttributeTable *other, IndexT otherRowIndex, bool createMissingRows=false) |
create a new row as copy of a row from another value table | |
SizeT | GetNumRows () const |
get number of rows in table | |
bool | HasNewRows () const |
return true if table has new rows | |
bool | HasModifiedRows () const |
return true if table has modified rows | |
bool | HasDeletedRows () const |
return true if table has deleted rows | |
bool | IsRowModified (IndexT rowIndex) const |
return true if a row has been modified since the last ResetModifiedState() | |
const Util::Array < IndexT > & | GetNewRowIndices () const |
return indices of rows added since the last ResetModfiedState() | |
const Util::Array < IndexT > & | GetDeletedRowIndices () const |
return indices of rows deleted since the last ResetModifiedState() | |
Util::Array< IndexT > | GetModifiedRowsExcludeNewAndDeletedRows () const |
return array of modified rows, exclude rows marked as rows | |
void | ReserveRows (SizeT numRows) |
reserve rows to reduce re-allocation overhead | |
Util::Array< IndexT > | FindRowIndicesByAttr (const Attribute &attr, bool firstMatchOnly) const |
find all matching row indices by attribute value | |
Util::Array< IndexT > | FindRowIndicesByAttrs (const Util::Array< Attribute > &attrs, bool firstMatchOnly) const |
find all matching row indices by multiple attribute values | |
IndexT | FindRowIndexByAttr (const Attribute &attr) const |
find all matching row indices by attribute value | |
IndexT | FindRowIndexByAttrs (const Util::Array< Attribute > &attrs) const |
find all matching row indices by multiple attribute values | |
void | SetRowUserData (IndexT rowIndex, void *p) |
set an optional row user data pointer | |
void * | GetRowUserData (IndexT rowIndex) const |
get optional row user data pointer | |
void | SetAttr (const Attr::Attribute &attr, IndexT rowIndex) |
set a generic attribute (slow!) | |
void | SetBool (const BoolAttrId &colAttrId, IndexT rowIndex, bool val) |
set bool value | |
bool | GetBool (const BoolAttrId &colAttrId, IndexT rowIndex) const |
get bool value | |
void | SetFloat (const FloatAttrId &colAttrId, IndexT rowIndex, float val) |
set float value | |
float | GetFloat (const FloatAttrId &colAttrId, IndexT rowIndex) const |
get float value | |
void | SetInt (const IntAttrId &colAttrId, IndexT rowIndex, int val) |
set int value | |
int | GetInt (const IntAttrId &colAttrId, IndexT rowIndex) const |
get int value | |
void | SetString (const StringAttrId &colAttrId, IndexT rowIndex, const Util::String &val) |
set string value | |
const Util::String & | GetString (const StringAttrId &colAttrId, IndexT rowIndex) const |
get string value | |
void | SetFloat4 (const Float4AttrId &colAttrId, IndexT rowIndex, const Math::float4 &val) |
set float4 value | |
Math::float4 | GetFloat4 (const Float4AttrId &colAttrId, IndexT rowIndex) const |
get float4 value | |
void | SetMatrix44 (const Matrix44AttrId &colAttrId, IndexT rowIndex, const Math::matrix44 &val) |
set matrix44 value | |
Math::matrix44 | GetMatrix44 (const Matrix44AttrId &colAttrId, IndexT rowIndex) const |
get matrix44 value | |
void | SetGuid (const GuidAttrId &colAttrId, IndexT rowIndex, const Util::Guid &guid) |
set guid value | |
const Util::Guid & | GetGuid (const GuidAttrId &colAttrId, IndexT rowIndex) const |
get guid value | |
void | SetBlob (const BlobAttrId &colAttrId, IndexT rowIndex, const Util::Blob &blob) |
set blob value | |
const Util::Blob & | GetBlob (const BlobAttrId &colAttrId, IndexT rowIndex) const |
get blob value | |
void | SetBool (IndexT colIndex, IndexT rowIndex, bool val) |
set bool value by column index | |
bool | GetBool (IndexT colIndex, IndexT rowIndex) const |
get bool value by column index | |
void | SetFloat (IndexT colIndex, IndexT rowIndex, float val) |
set float value by column index | |
float | GetFloat (IndexT colIndex, IndexT rowIndex) const |
get float value by column index | |
void | SetInt (IndexT colIndex, IndexT rowIndex, int val) |
set int value by column index | |
int | GetInt (IndexT colIndex, IndexT rowIndex) const |
get int value by column index | |
void | SetString (IndexT colIndex, IndexT rowIndex, const Util::String &val) |
set string value by column index | |
const Util::String & | GetString (IndexT colIndex, IndexT rowIndex) const |
get string value by column index | |
void | SetFloat4 (IndexT colIndex, IndexT rowIndex, const Math::float4 &val) |
set float4 value by column index | |
Math::float4 | GetFloat4 (IndexT colIndex, IndexT rowIndex) const |
get float4 value by column index | |
void | SetMatrix44 (IndexT colIndex, IndexT rowIndex, const Math::matrix44 &val) |
set matrix44 value by column index | |
Math::matrix44 | GetMatrix44 (IndexT colIndex, IndexT rowIndex) const |
get matrix44 value by column index | |
void | SetGuid (IndexT colIndex, IndexT rowIndex, const Util::Guid &guid) |
set guid value by column index | |
const Util::Guid & | GetGuid (IndexT colIndex, IndexT rowIndex) const |
get guid value by column index | |
void | SetBlob (IndexT colIndex, IndexT rowIndex, const Util::Blob &blob) |
set blob value by column index | |
const Util::Blob & | GetBlob (IndexT colIndex, IndexT rowIndex) const |
get blob value by column index | |
bool | LoadXmlTable (const Util::String &fileName) |
load xml table | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void Attr::AttributeTable::ResetModifiedState | ( | ) |
reset all the modified bits in the table
This method resets the object to the unmodified state, which means the new-row and new-column index arrays are reset, and all modified bits are cleared.
void Attr::AttributeTable::Clear | ( | ) |
clear the table object
Clear all rows data and reset the number of rows.
void Attr::AttributeTable::PrintDebug | ( | ) |
print the contents of the table for debugging reasons
Print contents of the table for debugging.
void Attr::AttributeTable::BeginAddColumns | ( | bool | recordAsNewColumns = true |
) |
optional: call before adding columns, speeds up adding many columns at once
Begin adding columns. Columns can be added at any time, but it will be much more efficient when called between BeginAddColumns() and EndAddColumns(), since this will save a lot of re-allocations.
void Attr::AttributeTable::AddColumn | ( | const AttrId & | id, | |
bool | recordAsNewColumn = true | |||
) |
add a column
Add a column to the attribute table. If the attribute table already contains data, this will reallocate the existing data buffer. The name, data type, access mode, etc of the column is all defined by the given attribute id. The new column will be filled with the attribute id's default value.
void Attr::AttributeTable::EndAddColumns | ( | ) |
optional: call after adding columns, speeds up adding many columns at once
End adding columns. This will do the actual work.
IndexT Attr::AttributeTable::AddRow | ( | ) |
add a row to the table, returns index of new row
Adds an empty row at the end of the value buffer. The row will be marked invalid until the first value is set in the row. This will re-allocate the existing value buffer. If you know beforehand how many rows will exist in the table it is more efficient to use one SetNumRows(N) instead of N times AddRow()! The method returns the index of the newly added row. The row will be filled with the row attribute's default values.
void Attr::AttributeTable::ClearNewRowFlags | ( | ) |
clear the new row flags, so that new rows are treated like updated rows
Clears the new row flags. After this, new rows are treated just like updated rows, which may be useful for some database operations (when an UPDATE is wanted instead of an INSERT).
void Attr::AttributeTable::ClearDeletedRowsFlags | ( | ) |
clear deleted rows flags
Clears the deleted row flags.
void Attr::AttributeTable::DeleteRow | ( | IndexT | rowIndex | ) |
mark a row as deleted from the table
This marks a row for deletion. Note that the row will only be marked for deletion, deleted row indices are returned with the GetDeletedRowIndices() call. The row will never be physically removed from memory!
void Attr::AttributeTable::DeleteAllRows | ( | ) |
mark all rows as deleted
Marks all rows in the table as deleted.
bool Attr::AttributeTable::IsRowDeleted | ( | IndexT | rowIndex | ) | const [inline] |
return true if row has been marked as deleted
Return true if a row has been marked as deleted.
IndexT Attr::AttributeTable::CopyRow | ( | IndexT | fromRowIndex | ) |
create a new row as copy of another row
This creates a new row as a copy of an existing row. Returns the index of the new row. NOTE: the user data will be initialized to 0 for the new row!
IndexT Attr::AttributeTable::CopyExtRow | ( | AttributeTable * | other, | |
IndexT | otherRowIndex, | |||
bool | createMissingRows = false | |||
) |
create a new row as copy of a row from another value table
Create a new row as a copy of a row in another value table. The layouts of the value tables must not match, since only matching columns will be considered. NOTE: the user data will be initialised to 0 for the new row.
bool Attr::AttributeTable::IsRowModified | ( | IndexT | rowIndex | ) | const [inline] |
return true if a row has been modified since the last ResetModifiedState()
Quickly check if a row is modified.
Util::Array< IndexT > Attr::AttributeTable::GetModifiedRowsExcludeNewAndDeletedRows | ( | ) | const |
return array of modified rows, exclude rows marked as rows
Returns an array of all modified rows, but excludes rows marked as new.
void Attr::AttributeTable::ReserveRows | ( | SizeT | num | ) |
reserve rows to reduce re-allocation overhead
Reserve N more rows beforehand to reduce re-allocation overhead during AddRow().
Util::Array< IndexT > Attr::AttributeTable::FindRowIndicesByAttr | ( | const Attribute & | attr, | |
bool | firstMatchOnly | |||
) | const |
find all matching row indices by attribute value
Finds multiple row indices by matching attribute. This method can be slow since it may search linearly (and vertically) through the table.
Util::Array< IndexT > Attr::AttributeTable::FindRowIndicesByAttrs | ( | const Util::Array< Attribute > & | attrs, | |
bool | firstMatchOnly | |||
) | const |
find all matching row indices by multiple attribute values
Finds multiple row indices by multiple matching attribute. This method can be slow since it may search linearly (and vertically) through the table.
IndexT Attr::AttributeTable::FindRowIndexByAttr | ( | const Attribute & | attr | ) | const |
find all matching row indices by attribute value
Finds single row index by matching attribute. This method can be slow since it may search linearly (and vertically) through the table.
IndexT Attr::AttributeTable::FindRowIndexByAttrs | ( | const Util::Array< Attribute > & | attrs | ) | const |
find all matching row indices by multiple attribute values
Finds single row index by multiple matching attributes. This method can be slow since it may search linearly (and vertically) through the table.
void Attr::AttributeTable::SetAttr | ( | const Attr::Attribute & | attr, | |
IndexT | rowIndex | |||
) |
set a generic attribute (slow!)
Set a generic attribute, this is a slow method!
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.