Scripting::Command Class Reference
#include <command.h>
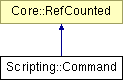
Detailed Description
Base class for script commands. A script command object implements a new scripting command in a language independent fashion. New script commands are added by deriving a new class from Scripting::Command and registering it with the script server.(C) 2006 Radon Labs GmbH
Public Member Functions | |
Command () | |
constructor | |
virtual | ~Command () |
destructor | |
virtual void | OnRegister () |
called when the scripting command is registered | |
virtual void | OnUnregister () |
called when the scripting command is unregistered | |
bool | IsRegistered () const |
return true if currently registered | |
virtual bool | OnExecute () |
called when the script command is executed | |
virtual Util::String | GetHelp () const |
return a short help string, describing the command | |
const Util::String & | GetName () const |
get the name under which the command has been registered | |
Util::String | GetSyntax () const |
get syntax as string | |
const ArgsBlock & | GetArguments () const |
read-only access to command args | |
ArgsBlock & | Arguments () |
read/write access to command arguments | |
const ArgsBlock & | GetResults () const |
access to result arguments | |
const Util::String & | GetError () const |
get error string | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void __cdecl | SetError (const char *fmt,...) |
set error |
Member Function Documentation
void Scripting::Command::OnRegister | ( | ) | [virtual] |
called when the scripting command is registered
Called when the command is registered with the script server. Derive this method and setup the arguments the command requires and the block of results the command returns.
void Scripting::Command::OnUnregister | ( | ) | [virtual] |
called when the scripting command is unregistered
Called when the command is unregistered from the script server. This method must undo everything done in the OnRegister() method.
bool Scripting::Command::OnExecute | ( | ) | [virtual] |
called when the script command is executed
Called when the script command should be executed. The command arguments will be filled with the args from the script function call, after the command functionality has been executed the command should provide any results in its results block. If there was an error executing the command the command should return false and have an error string set.
String Scripting::Command::GetSyntax | ( | ) | const |
get syntax as string
Returns a string describing the command syntax in the form "type name = cmd(type name, type name, ...)"
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.