Models::ModelInstance Class Reference
#include <modelinstance.h>
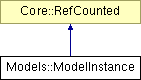
Detailed Description
A ModelInstance contains the per-instance data for rendering a Model. Usually there is one ModelInstance created per game object.A ModelInstance is roughly comparable to a Nebula2 nRenderContext.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
ModelInstance () | |
constructor | |
virtual | ~ModelInstance () |
destructor | |
void | Discard () |
cleanup and unlink from model | |
bool | IsAttachedToModel () const |
return true if currently attached to Model | |
const Ptr< Model > & | GetModel () const |
get the Model this instance was created from | |
const Ptr < Graphics::ModelEntity > & | GetModelEntity () const |
get the ModelEntity which owns this instance | |
void | SetTransform (const Math::matrix44 &m) |
set world space transform of the instance | |
const Math::matrix44 & | GetTransform () const |
get world space transform | |
void | SetTime (Timing::Time t) |
set instance time | |
Timing::Time | GetTime () const |
get instance time | |
bool | HasNodeInstance (const Util::Atom< Util::String > &name) const |
return true if a model instance node exists | |
const Ptr < ModelNodeInstance > & | LookupNodeInstance (const Util::Atom< Util::String > &name) const |
lookup an instance node | |
const Util::Array < Ptr < ModelNodeInstance > > & | GetNodeInstances () const |
get all instance nodes | |
const Ptr < ModelNodeInstance > & | LookupNodeInstanceByRTTI (const Core::Rtti &rtti) const |
lookup an instance node by rtti type | |
void | Update () |
perform per-frame update (after setting transform, visibility, time, etc) | |
void | RenderDebug () |
render node specific debug shape | |
void | SetAllNodeInstancesVisible (bool b) |
set all modelnode instances visible | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetModelEntity (const Ptr< Graphics::ModelEntity > &mdlEntity) |
set pointer to ModelEntity which owns this instance | |
void | NotifyVisible (IndexT frameIndex) |
notify the model instance that it is currently visible | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to Model | |
virtual void | OnRemoveFromModel () |
called when removed from Model |
Member Function Documentation
bool Models::ModelInstance::HasNodeInstance | ( | const Util::Atom< Util::String > & | name | ) | const |
return true if a model instance node exists
Returns true if a ModelInstanceNode exists by name. ModelInstanceNodes are associated 1:1 with the ModelNodes of the Model which created this instance. Because of this we can just use the Model's node map.
void Models::ModelInstance::Update | ( | ) |
perform per-frame update (after setting transform, visibility, time, etc)
The Update() method is called once per frame on potentially visible ModelInstances. The method must be called AFTER transformation, visibility, time or other parameters have been set. The Update() method will be propagated to all instance nodes.
void Models::ModelInstance::RenderDebug | ( | ) |
render node specific debug shape
This method is called from the RenderDebug of Graphics::ModelEntity.
void Models::ModelInstance::NotifyVisible | ( | IndexT | frameIndex | ) | [protected] |
notify the model instance that it is currently visible
This method is called by the Graphics subsystem when we are currently visible. Once all visible model instances are notified, the Graphics subsystem can get a render-order-optimized list of all visible model-node-instances through the ModelServer.
void Models::ModelInstance::OnRemoveFromModel | ( | ) | [protected, virtual] |
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.