Attr::Attribute Class Reference
#include <attribute.h>
Inheritance diagram for Attr::Attribute:
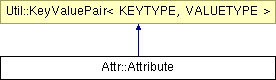
Detailed Description
A compiletime-typesafe key/value pair.(C) 2006 Radon Labs GmbH
Public Member Functions | |
Attribute () | |
default constructor | |
Attribute (const Attribute &rhs) | |
copy constructor | |
Attribute (const AttrId &id) | |
construct from typeless attribute id | |
Attribute (const BoolAttrId &id, bool val) | |
construct from bool | |
Attribute (const FloatAttrId &id, float val) | |
construct from float | |
Attribute (const IntAttrId &id, int val) | |
construct from int | |
Attribute (const Matrix44AttrId &id, const Math::matrix44 &val) | |
construct from matrix44 | |
Attribute (const StringAttrId &id, const Util::String &val) | |
construct from string | |
Attribute (const Float4AttrId &id, const Math::float4 &val) | |
construct from float4 | |
Attribute (const GuidAttrId &id, const Util::Guid &val) | |
construct from guid | |
Attribute (const BlobAttrId &id, const Util::Blob &val) | |
construct from blob | |
void | SetAttrId (const AttrId &id) |
set attribute id | |
const AttrId & | GetAttrId () const |
get attribute id | |
const Util::String & | GetName () const |
get name of attribute | |
const Util::FourCC & | GetFourCC () const |
get fourcc of attribute | |
ValueType | GetValueType () const |
get value type of attribute | |
AccessMode | GetAccessMode () const |
get access mode of attribute | |
void | Clear () |
clear the attribute's value | |
void | operator= (const Attribute &rhs) |
assignment operator | |
void | operator= (bool rhs) |
bool assignment operator | |
void | operator= (float rhs) |
float assignment operator | |
void | operator= (int rhs) |
int assignment operator | |
void | operator= (const Math::matrix44 &rhs) |
matrix44 assignment operator | |
void | operator= (const Util::String &rhs) |
string assignment operator | |
void | operator= (const Math::float4 &rhs) |
float4 assignment operator | |
void | operator= (const Util::Guid &rhs) |
guid assignment operator | |
void | operator= (const Util::Blob &rhs) |
blob assignment operator | |
bool | operator== (const Attribute &rhs) const |
equality operator | |
bool | operator== (const Util::String &rhs) const |
string equality operator | |
bool | operator== (int rhs) const |
int equality operator | |
bool | operator== (float rhs) const |
float equality operator | |
bool | operator== (bool rhs) const |
bool equality operator | |
bool | operator== (const Math::float4 &rhs) const |
float4 equality operator | |
bool | operator== (const Util::Guid &rhs) const |
guid equality operator | |
bool | operator!= (const Attribute &rhs) const |
equality operator | |
bool | operator!= (const Util::String &rhs) const |
string equality operator | |
bool | operator!= (int rhs) const |
int equality operator | |
bool | operator!= (float rhs) const |
float equality operator | |
bool | operator!= (bool rhs) const |
bool equality operator | |
bool | operator!= (const Math::float4 &rhs) const |
float4 equality operator | |
bool | operator!= (const Util::Guid &rhs) const |
guid equality operator | |
void | SetBool (bool val) |
set bool content | |
bool | GetBool () const |
get bool content | |
void | SetInt (int val) |
set int content | |
int | GetInt () const |
get int content | |
void | SetFloat (float val) |
set float content | |
float | GetFloat () const |
get float content | |
void | SetString (const Util::String &val) |
set string content | |
const Util::String & | GetString () const |
get string content | |
void | SetMatrix44 (const Math::matrix44 &val) |
set matrix44 value | |
const Math::matrix44 & | GetMatrix44 () const |
get matrix44 value | |
void | SetFloat4 (const Math::float4 &val) |
set float4 value | |
Math::float4 | GetFloat4 () const |
get float4 value | |
void | SetGuid (const Util::Guid &val) |
set guid value | |
const Util::Guid & | GetGuid () const |
get guid value | |
void | SetBlob (const Util::Blob &val) |
set blob value | |
const Util::Blob & | GetBlob () const |
get blob value | |
void | SetValue (const Util::Variant &val) |
set generic value | |
const Util::Variant & | GetValue () const |
get generic value | |
void | SetValueFromString (const Util::String &str) |
set value from string (convert as necessary) | |
Util::String | ValueAsString () const |
convert the content to a string | |
bool | operator== (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
equality operator | |
bool | operator!= (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
inequality operator | |
bool | operator> (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
greater operator | |
bool | operator>= (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
greater-or-equal operator | |
bool | operator< (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
lesser operator | |
bool | operator<= (const KeyValuePair< KEYTYPE, VALUETYPE > &rhs) const |
lesser-or-equal operator | |
VALUETYPE & | Value () |
read/write access to value | |
const VALUETYPE & | Value () const |
read access to key | |
const KEYTYPE & | Key () const |
read access to key | |
Static Public Member Functions | |
static ValueType | StringToValueType (const Util::String &s) |
convert string to type | |
static Util::String | ValueTypeToString (ValueType t) |
convert type to string |