BaseGameFeature::EnvQueryManager Class Reference
#include <envquerymanager.h>
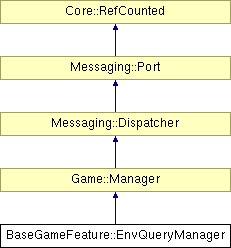
Detailed Description
The EnvQueryManager implements environment queries into the game world, like stabbing queries, line-of-sight checks, etc...(C) 2005 Radon Labs GmbH
Public Member Functions | |
EnvQueryManager () | |
constructor | |
virtual | ~EnvQueryManager () |
destructor | |
virtual void | OnActivate () |
called when attached to game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
virtual void | OnFrame () |
called per-frame by game server | |
const Physics::FilterSet & | GetMouseExcludeSet () const |
get a const Ref on the MouseExcludeSet | |
void | SetMouseExcludeSet (const Physics::FilterSet &mouseExcludeSet) |
set a new MouseExcludeSet | |
Game::Entity * | GetEntityUnderMouse () const |
get the entity under the mouse cursor | |
Physics::MaterialType | GetMaterialUnderMouse () const |
get the material under the mouse cursor | |
const Math::point & | GetMousePos3d () const |
get the mouse position in the 3d world | |
Math::line | ComputeMouseWorldRay (const Math::float2 &mousePos, float length, const Ptr< Graphics::View > &view) const |
compute 3d ray thru mouse pos | |
const Math::vector & | GetUpVector () const |
get the upVector of the face under the mouse cursor | |
bool | HasMouseIntersection () const |
return true if mouse is over "something" | |
Util::Array< Ptr < Game::Entity > > | GetEntitiesInSphere (const Math::point &midPoint, float radius) |
get all entities in a given spherical area | |
Util::Array< Ptr < Game::Entity > > | GetEntitiesInBox (const Math::vector &scale, const Math::matrix44 &m) |
get all entities in a given box shaped area | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array < const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void BaseGameFeature::EnvQueryManager::OnFrame | ( | ) | [virtual] |
called per-frame by game server
This method is called per-frame by the game server and updates the current values, like entity-under-mouse, 3d mouse position, etc...
Reimplemented from Game::Manager.
const Physics::FilterSet & BaseGameFeature::EnvQueryManager::GetMouseExcludeSet | ( | ) | const |
get a const Ref on the MouseExcludeSet
get a const Ref on the MouseExcludeSet
void BaseGameFeature::EnvQueryManager::SetMouseExcludeSet | ( | const Physics::FilterSet & | mouseExcludeSet | ) |
set a new MouseExcludeSet
set a new MouseExcludeSet
Entity * BaseGameFeature::EnvQueryManager::GetEntityUnderMouse | ( | ) | const |
get the entity under the mouse cursor
This returns a pointer to the entity under the mouse, or 0 if mouse is not over an entity.
Physics::MaterialType BaseGameFeature::EnvQueryManager::GetMaterialUnderMouse | ( | ) | const |
get the material under the mouse cursor
This returns the MaterialType of the entity under the mouse, or InvalidMaterial if mouse is not over an entity.
const point & BaseGameFeature::EnvQueryManager::GetMousePos3d | ( | ) | const |
get the mouse position in the 3d world
This returns the position where a vector through the mouse position intersects the 3d world (or the nearest entity). If the mouse doesn't intersect, the result will be undefined, and the method HasMouseIntersection() returns false.
const vector & BaseGameFeature::EnvQueryManager::GetUpVector | ( | ) | const |
get the upVector of the face under the mouse cursor
This returns the upvector of the face under the mousecursor. If the mouse doesn't intersect, the result will be undefined, and the method HasMouseIntersection() returns false.
bool BaseGameFeature::EnvQueryManager::HasMouseIntersection | ( | ) | const |
return true if mouse is over "something"
Returns true if the vector through the current mouse position intersects the world, or an entity, false if no intersection exists.
Util::Array< Ptr< Game::Entity > > BaseGameFeature::EnvQueryManager::GetEntitiesInSphere | ( | const Math::point & | midPoint, | |
float | radius | |||
) |
get all entities in a given spherical area
Returns all game entities which intersect the given sphere. Uses the physics subsystem to do the query.
Util::Array< Ptr< Game::Entity > > BaseGameFeature::EnvQueryManager::GetEntitiesInBox | ( | const Math::vector & | scale, | |
const Math::matrix44 & | m | |||
) |
get all entities in a given box shaped area
Returns all game entities which intersect the given box. Uses the physics subsystem to do the query.
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.