Models::ParticleSystemNode Class Reference
#include <particlesystemnode.h>
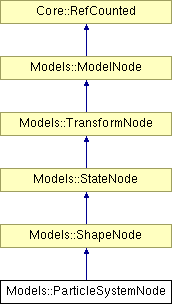
Detailed Description
A model node which holds particle system information and applies a shared dynamiceMeshes for its set of ParticleSystemNodeInstances.(C) 2008 Radon Labs GmbH
Public Member Functions | |
ParticleSystemNode () | |
constructor | |
virtual | ~ParticleSystemNode () |
destructor | |
virtual void | LoadResources () |
called when resources should be loaded | |
virtual void | UnloadResources () |
called when resources should be unloaded | |
Ptr < ParticleSystem::DynamicMesh > | GetParticleMesh () const |
get the particle mesh | |
void | SetInvisible (bool value) |
set if invisible or not | |
void | SetEmissionDuration (float time) |
set the end time | |
float | GetEmissionDuration () const |
get the emission duration | |
void | SetLoop (bool b) |
set if loop emitter or not | |
bool | GetLoop () const |
is loop emitter ? | |
void | SetActivityDistance (float activityDistance) |
set the activity distance | |
float | GetActivityDistance () const |
get the activity distance | |
void | SetStartRotationMin (float rotMin) |
set the maximum start rotation angle | |
void | SetStartRotationMax (float rotMax) |
set the maximum start rotation angle | |
void | SetGravity (float gravity) |
set gravity | |
float | GetGravity () const |
get gravity | |
void | SetParticleStretch (float particleStretch) |
set amount of stretching | |
void | SetParticleVelocityRandomize (float velRandom) |
set velocity randomize value | |
void | SetParticleRotationRandomize (float rotRandom) |
set rotation randomize value | |
void | SetParticleSizeRandomize (float sizeRandom) |
set size randomize value | |
void | SetRandomRotDir (bool isRandom) |
randomize rotation direction on/off | |
void | SetTileTexture (int tileTexture) |
set texture tiling parts | |
void | SetStretchToStart (bool isStretchToStart) |
set if texture should be stretched to the emission start point | |
void | SetPrecalcTime (float preClacTime) |
set precalc time | |
void | SetRenderOldestFirst (bool isOldestFirst) |
set wether to render oldest or youngest particles first | |
void | SetStretchDetail (int stretchDetail) |
set the stretch detail | |
void | SetViewAngleFade (bool viewAngelFade) |
set wether to render oldest or youngest particles first | |
bool | GetRenderOldestFirst () const |
get wether to render oldest or youngest particles first | |
void | SetStartDelay (float startDelay) |
set start delay | |
void | SetCurve (ParticleSystem::ParticleEmitter::CurveType curveType, const ParticleSystem::EnvelopeCurve &curve) |
set one of the envelope curves (not the color) | |
const ParticleSystem::EnvelopeCurve & | GetCurve (ParticleSystem::ParticleEmitter::CurveType curveType) const |
get one of the envelope curves | |
void | SetRGBCurve (const ParticleSystem::Vector3EnvelopeCurve &curve) |
set the particle rgb curv | |
const ParticleSystem::Vector3EnvelopeCurve & | GetRGBCurve () const |
get the particle rgb curve | |
void | SetBillboardOrientation (bool b) |
sets orientation to billboard | |
bool | GetBillboardOrientation () |
gets billboard flag | |
virtual Resources::Resource::State | GetResourceState () const |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled) | |
virtual void | ApplySharedState () |
apply state shared by all my ModelNodeInstances | |
const Ptr < Resources::ManagedMesh > & | GetManagedMesh () const |
get managed mesh | |
const Ptr < CoreGraphics::ShaderInstance > & | GetShaderInstance () const |
get pointer to contained shader instance | |
void | SetPosition (const Math::point &p) |
set position | |
const Math::point & | GetPosition () const |
get position | |
void | SetRotate (const Math::quaternion &r) |
set rotate quaternion | |
const Math::quaternion & | GetRotate () const |
get rotate quaternion | |
void | SetScale (const Math::vector &s) |
set scale | |
const Math::vector & | GetScale () const |
get scale | |
void | SetRotatePivot (const Math::point &p) |
set rotate pivot | |
const Math::point & | GetRotatePivot () const |
get rotate pivot | |
void | SetScalePivot (const Math::point &p) |
set scale pivot | |
const Math::point & | GetScalePivot () const |
get scale pivot | |
const Util::Atom < Util::String > & | GetName () const |
get model node name | |
ModelNodeType::Code | GetType () const |
get the ModelNodeType | |
bool | HasParent () const |
return true if node has a parent | |
const Ptr< ModelNode > & | GetParent () const |
get parent node | |
const Util::Array < Ptr< ModelNode > > & | GetChildren () const |
get child nodes | |
bool | IsAttachedToModel () const |
return true if currently attached to a Model | |
const Ptr< Model > & | GetModel () const |
get model this node is attached to | |
const Math::bbox & | GetBoundingBox () const |
get bounding box of model node | |
const Attr::AttributeContainer & | GetAttrs () const |
read access to model node attributes | |
bool | HasAttr (const Attr::AttrId &attrId) const |
check if model node attribute exists | |
void | SetAttr (const Attr::Attribute &attr) |
set generic model node attribute | |
const Attr::Attribute & | GetAttr (const Attr::AttrId &attrId) const |
get generic model node attribute | |
void | SetBool (const Attr::BoolAttrId &attrId, bool val) |
set bool value | |
bool | GetBool (const Attr::BoolAttrId &attrId) const |
get bool value | |
void | SetFloat (const Attr::FloatAttrId &attrId, float val) |
set float value | |
float | GetFloat (const Attr::FloatAttrId &attrId) const |
get float value | |
void | SetInt (const Attr::IntAttrId &attrId, int val) |
set int value | |
int | GetInt (const Attr::IntAttrId &attrId) const |
get int value | |
void | SetString (const Attr::StringAttrId &attrId, const Util::String &val) |
set string value | |
const Util::String & | GetString (const Attr::StringAttrId &attrId) const |
get string value | |
void | SetFloat4 (const Attr::Float4AttrId &attrId, const Math::float4 &val) |
set float4 value | |
Math::float4 | GetFloat4 (const Attr::Float4AttrId &attrId) const |
get float4 value | |
void | SetMatrix44 (const Attr::Matrix44AttrId &attrId, const Math::matrix44 &val) |
set matrix44 value | |
const Math::matrix44 & | GetMatrix44 (const Attr::Matrix44AttrId &attrId) const |
get matrix44 value | |
void | SetGuid (const Attr::GuidAttrId &attrId, const Util::Guid &guid) |
set guid value | |
const Util::Guid & | GetGuid (const Attr::GuidAttrId &attrId) const |
get guid value | |
void | SetBlob (const Attr::BlobAttrId &attrId, const Util::Blob &blob) |
set blob value | |
const Util::Blob & | GetBlob (const Attr::BlobAttrId &attrId) const |
get blob value | |
void | SetName (const Util::Atom< Util::String > &n) |
set model node name | |
void | SetType (ModelNodeType::Code t) |
set ModelNodeType | |
void | SetParent (const Ptr< ModelNode > &p) |
set parent node | |
void | AddChild (const Ptr< ModelNode > &c) |
add a child node | |
void | AddVisibleNodeInstance (IndexT frameIndex, const Ptr< ModelNodeInstance > &nodeInst) |
called by model node instance on NotifyVisible() | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual Ptr < ModelNodeInstance > | CreateNodeInstance () const |
gets the particle system mesh; | |
void | SetupManagedTextureVariable (const Attr::AttrId &resAttrId, const Ptr< CoreGraphics::ShaderVariable > &var) |
setup a new managed texture variable | |
void | UpdateManagedTextureVariables () |
update managed texture variables | |
virtual void | LoadFromAttrs (const Attr::AttributeContainer &attrs) |
called to initialize from attributes | |
virtual void | SaveToAttrs (Attr::AttributeContainer &attrs) |
called to save state back to attributes | |
void | SetBoundingBox (const Math::bbox &b) |
set bounding box | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to model node | |
virtual void | OnRemoveFromModel () |
called when removed from model node | |
const Util::Array < Ptr < ModelNodeInstance > > & | GetVisibleModelNodeInstances (ModelNodeType::Code t) const |
get visible model node instances | |
Protected Attributes | |
float | sampledCurves [ParticleSystem::ParticleEmitter::ParticleTimeDetail][ParticleSystem::ParticleEmitter::CurveTypeCount] |
a mesh for storing and rendering dynamic data. In this case particle data. |
Member Function Documentation
Ptr< ModelNodeInstance > Models::ParticleSystemNode::CreateNodeInstance | ( | ) | const [protected, virtual] |
void Models::StateNode::SetupManagedTextureVariable | ( | const Attr::AttrId & | resAttrId, | |
const Ptr< CoreGraphics::ShaderVariable > & | var | |||
) | [protected, inherited] |
setup a new managed texture variable
Create a new managed texture resource and bind it to the provided shader variable.
void Models::StateNode::UpdateManagedTextureVariables | ( | ) | [protected, inherited] |
update managed texture variables
This method transfers texture from our managed texture objects into their associated shader variable. This is necessary since the actual texture of a managed texture may change from frame to frame because of resource management.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.