AsyncGraphics::CameraEntityProxy Class Reference
#include <cameraentityproxy.h>
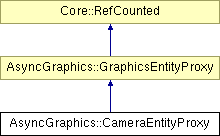
Detailed Description
Client-side proxy of a Graphics::CameraEntity. NOTE: all getter-methods of this class return client-side cached values, not the actual server-side values. Thus they may be off by some amount, since the render thread may run at a different frame rate then the client thread!(C) 2007 Radon Labs GmbH
Public Member Functions | |
CameraEntityProxy () | |
constructor | |
virtual | ~CameraEntityProxy () |
destructor | |
void | SetViewName (const Util::StringAtom &n) |
set the view name which should use this camera | |
const Util::StringAtom & | GetViewName () const |
get the view name which should use this camera | |
void | SetupPerspectiveFov (float fov, float aspect, float zNear, float zFar) |
setup camera for perspective field-of-view projection transform | |
void | SetupOrthogonal (float w, float h, float zNear, float zFar) |
setup camera for orthogonal projection transform | |
const Math::matrix44 & | GetProjTransform () const |
get projection matrix | |
const Math::matrix44 & | GetViewTransform () const |
get view transform (inverse transform) | |
const Math::matrix44 & | GetViewProjTransform () |
get view projection matrix (non-const!) | |
bool | IsPerspective () const |
return true if this is a perspective projection | |
bool | IsOrthogonal () const |
return true if this is an orthogonal transform | |
float | GetZNear () const |
get near plane distance | |
float | GetZFar () const |
get far plane distance | |
float | GetFov () const |
get field-of-view (only if perspective) | |
float | GetAspect () const |
get aspect ration (only if perspective) | |
float | GetNearWidth () const |
get width of near plane | |
float | GetNearHeight () const |
get height of near plane | |
float | GetFarWidth () const |
get width of far plane | |
float | GetFarHeight () const |
get height of far plane | |
bool | IsValid () const |
return true if entity is valid (is attached to a stage) | |
Graphics::GraphicsEntity::Type | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< StageProxy > & | GetStageProxy () const |
get the stage proxy this entity is attached to | |
bool | IsAttachedToStageProxy () const |
return true if entity is attached to stage | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | Setup (const Ptr< StageProxy > &stage) |
called by stage when entity should setup itself | |
virtual void | OnTransformChanged () |
called when transform matrix changed | |
void | UpdateViewProjMatrix () |
update the view projection matrix | |
void | SetType (Graphics::GraphicsEntity::Type t) |
set graphics entity type, called from constructor of subclass | |
virtual void | Discard () |
called by stage when entity should discard itself | |
void | ValidateEntityHandle (bool wait=false) |
validate entity handle | |
void | SendCreateMsg (const Ptr< CreateGraphicsEntity > &msg) |
send off a specific create message from the subclass | |
void | SendMsg (const Ptr< GraphicsEntityMsg > &msg) |
send a message to the server-side graphics entity | |
bool | IsEntityHandleValid () const |
test if the entity handle is valid |
Member Function Documentation
void AsyncGraphics::CameraEntityProxy::SetupPerspectiveFov | ( | float | fov_, | |
float | aspect_, | |||
float | zNear_, | |||
float | zFar_ | |||
) |
setup camera for perspective field-of-view projection transform
Setup camera as perspective projection. NOTE: this method is a copy of CameraEntity::SetupPerspectiveFov()!
void AsyncGraphics::CameraEntityProxy::SetupOrthogonal | ( | float | w, | |
float | h, | |||
float | zNear_, | |||
float | zFar_ | |||
) |
setup camera for orthogonal projection transform
Setup camera as orthogonal projection. NOTE: this method is a copy of CameraEntity::SetupOrthogonal()!
const Math::matrix44 & AsyncGraphics::CameraEntityProxy::GetViewTransform | ( | ) | const [inline] |
get view transform (inverse transform)
NOTE: The matrix returned here may be off a little bit from view matrix used in the render thread because the game thread may run at a slower frame rate then rendering! It is not possible to get an exact view matrix outside of the render thread.
const Math::matrix44 & AsyncGraphics::CameraEntityProxy::GetViewProjTransform | ( | ) | [inline] |
get view projection matrix (non-const!)
NOTE: The matrix returned here may be off a little bit from viewProj matrix used in the render thread because the game thread may run at a slower frame rate then rendering! It is not possible to get an exact viewProj matrix outside of the render thread.
void AsyncGraphics::CameraEntityProxy::Setup | ( | const Ptr< StageProxy > & | stageProxy_ | ) | [protected, virtual] |
called by stage when entity should setup itself
Setup the server-side camera entity.
Reimplemented from AsyncGraphics::GraphicsEntityProxy.
void AsyncGraphics::CameraEntityProxy::OnTransformChanged | ( | ) | [protected, virtual] |
called when transform matrix changed
We need to keep track of modifications of the transformation matrix.
Reimplemented from AsyncGraphics::GraphicsEntityProxy.
void AsyncGraphics::CameraEntityProxy::UpdateViewProjMatrix | ( | ) | [protected] |
update the view projection matrix
Updates the view-projection matrix.
void AsyncGraphics::GraphicsEntityProxy::Discard | ( | ) | [protected, virtual, inherited] |
called by stage when entity should discard itself
Discard the server-side graphics entity. This method is called from StageProxy::RemoveEntityProxy(). If the server-side entity hasn't been created yet, this method needs to wait for completion, since the entity handle won't be available yet!
void AsyncGraphics::GraphicsEntityProxy::ValidateEntityHandle | ( | bool | wait = false |
) | [protected, inherited] |
validate entity handle
Tries to validate the entity handle by checking whether the pending creation message has already been handled by the server side. If the wait flag is true, the method will wait until the entity has been created on the server side. The default is to NOT wait. If the graphics entity handle becomes valid, all pending messages will be sent off to the
void AsyncGraphics::GraphicsEntityProxy::SendCreateMsg | ( | const Ptr< CreateGraphicsEntity > & | msg | ) | [protected, inherited] |
send off a specific create message from the subclass
This method must be called from the Setup() method of a subclass to send off a specific creation message. The message will be stored in the proxy to get the entity handle back later when the server-side graphics entity has been created.
void AsyncGraphics::GraphicsEntityProxy::SendMsg | ( | const Ptr< GraphicsEntityMsg > & | msg | ) | [protected, inherited] |
send a message to the server-side graphics entity
Send a generic GraphicsEntityMsg to the server-side entity. Do not initialize the entity handle of the message before calling this method, since the server-side entity doesn't have to exist yet. In this case, the message will be queued up and sent off as soon as the server-side entity becomes valid.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.