BaseGameFeature::EnvEntityManager Class Reference
#include <enventitymanager.h>
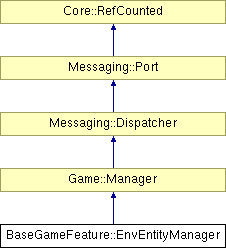
Detailed Description
Manages creation and updating of environment entities. All simple environment entities are kept in a single game entity to prevent pollution of the game entity pool with entities that don't actually do anything. Non-simple environment entities (entities with animations or physics) will still be created as normal game entities.The EnvEntityManager basically hides all differences between those types of environment entities.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
EnvEntityManager () | |
constructor | |
virtual | ~EnvEntityManager () |
destructor | |
virtual void | OnDeactivate () |
called when removed from game server | |
void | CreateEnvEntity (const Util::Array< Attr::Attribute > &attrs) |
create environment entity from scratch from provided attributes | |
void | CreateEnvEntity (const Ptr< Db::ValueTable > &instTable, IndexT instTableRowIndex) |
create an environment entity and attach to world | |
bool | EnvEntityExists (const Util::String &id) const |
check if an environment entity exists by _ID | |
void | SetEnvEntityTransform (const Util::String &id, const Math::matrix44 &m) |
set the world transform of an environment entity | |
void | DeleteEnvEntity (const Util::String &id) |
delete environment with given id | |
Util::Array< Ptr < Graphics::ModelEntity > > | GetGraphicsEntities (const Util::String &id) const |
get graphics entities by id (may return empty array) | |
void | ClearEnvEntity () |
clear enviornment entity | |
virtual void | OnActivate () |
called when attached to game server | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array < const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void BaseGameFeature::EnvEntityManager::CreateEnvEntity | ( | const Util::Array< Attr::Attribute > & | attrs | ) |
create environment entity from scratch from provided attributes
Create an simple environment entity from scratch. The following attributes should be provided:
- Attr::Graphics
- Attr::Physics
- Attr::_ID
- Attr::Transform
- Attr::AnimPath
void BaseGameFeature::EnvEntityManager::CreateEnvEntity | ( | const Ptr< Db::ValueTable > & | instTable, | |
IndexT | instTableRowIndex | |||
) |
create an environment entity and attach to world
Create an environment entity from its database attributes. This could end up in a bunch of graphics entities and collide shapes pooled in the central env entity, or as a traditional game object (if the environment object is animated or has physics).
bool BaseGameFeature::EnvEntityManager::EnvEntityExists | ( | const Util::String & | id | ) | const |
check if an environment entity exists by _ID
Return true if an environment entity with the given id exists.
void BaseGameFeature::EnvEntityManager::SetEnvEntityTransform | ( | const Util::String & | id, | |
const Math::matrix44 & | m | |||
) |
set the world transform of an environment entity
Set transformation of an environment entity.
void BaseGameFeature::EnvEntityManager::DeleteEnvEntity | ( | const Util::String & | id | ) |
delete environment with given id
Delete an environment entity.
Util::Array< Ptr< Graphics::ModelEntity > > BaseGameFeature::EnvEntityManager::GetGraphicsEntities | ( | const Util::String & | id | ) | const |
get graphics entities by id (may return empty array)
Returns the graphics entities of the given environment entity.
void Game::Manager::OnActivate | ( | ) | [virtual, inherited] |
called when attached to game server
This method is called when the manager is attached to the game server. The manager base class will register its message port with the message server.
Reimplemented in BaseGameFeature::CategoryManager, BaseGameFeature::EnvQueryManager, and BaseGameFeature::GlobalAttrsManager.
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.