Win32::Win32FSWrapper Class Reference
#include <win32fswrapper.h>
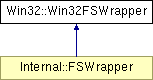
Detailed Description
Internal filesystem wrapper for Win32. All paths must be native Win32 paths.(C) 2006 Radon Labs GmbH
Static Public Member Functions | |
static Handle | OpenFile (const Util::String &path, IO::Stream::AccessMode accessMode, IO::Stream::AccessPattern accessPattern) |
open a file | |
static void | CloseFile (Handle h) |
close a file | |
static void | Write (Handle h, const void *buf, IO::Stream::Size numBytes) |
write to a file | |
static IO::Stream::Size | Read (Handle h, void *buf, IO::Stream::Size numBytes) |
read from a file | |
static void | Seek (Handle h, IO::Stream::Offset offset, IO::Stream::SeekOrigin orig) |
seek in a file | |
static IO::Stream::Position | Tell (Handle h) |
get position in file | |
static void | Flush (Handle h) |
flush a file | |
static bool | Eof (Handle h) |
return true if at end-of-file | |
static IO::Stream::Size | GetFileSize (Handle h) |
get size of a file in bytes | |
static void | SetReadOnly (const Util::String &path, bool readOnly) |
set read-only status of a file | |
static bool | IsReadOnly (const Util::String &path) |
get read-only status of a file | |
static bool | DeleteFile (const Util::String &path) |
delete a file | |
static bool | DeleteDirectory (const Util::String &path) |
delete an empty directory | |
static bool | FileExists (const Util::String &path) |
return true if a file exists | |
static bool | DirectoryExists (const Util::String &path) |
return true if a directory exists | |
static IO::FileTime | GetFileWriteTime (const Util::String &path) |
get the last write-access time stamp of a file | |
static bool | CreateDirectory (const Util::String &path) |
create a directory | |
static Util::Array < Util::String > | ListFiles (const Util::String &dirPath, const Util::String &pattern) |
list all files in a directory | |
static Util::Array < Util::String > | ListDirectories (const Util::String &dirPath, const Util::String &pattern) |
list all subdirectories in a directory | |
static Util::String | GetUserDirectory () |
get path to the current user's home directory (for user: standard assign) | |
static Util::String | GetTempDirectory () |
get path to the current user's temp directory (for temp: standard assign) | |
static Util::String | GetHomeDirectory () |
get path to the current application directory (for home: standard assign) | |
static Util::String | GetBinDirectory () |
get path to the current bin directory (for bin: standard assign) | |
static bool | IsDeviceName (const Util::String &str) |
return true when the string is a device name (e.g. "C:") |
Member Function Documentation
Win32FSWrapper::Handle Win32::Win32FSWrapper::OpenFile | ( | const Util::String & | path, | |
IO::Stream::AccessMode | accessMode, | |||
IO::Stream::AccessPattern | accessPattern | |||
) | [static] |
open a file
Open a file using the Win32 function CreateFile(). Returns a handle to the file which must be passed to the other Win32FSWrapper file methods. If opening the file fails, the function will return 0. The filename must be a native Win32 path (no assigns, etc...).
void Win32::Win32FSWrapper::CloseFile | ( | Handle | handle | ) | [static] |
close a file
Closes a file opened by Win32FSWrapper::OpenFile().
Stream::Position Win32::Win32FSWrapper::Tell | ( | Handle | handle | ) | [static] |
get position in file
Get current position in file.
void Win32::Win32FSWrapper::Flush | ( | Handle | handle | ) | [static] |
flush a file
Flush unwritten data to file.
bool Win32::Win32FSWrapper::Eof | ( | Handle | handle | ) | [static] |
return true if at end-of-file
Returns true if current position is at end of file.
Stream::Size Win32::Win32FSWrapper::GetFileSize | ( | Handle | handle | ) | [static] |
get size of a file in bytes
Returns the size of a file in bytes.
void Win32::Win32FSWrapper::SetReadOnly | ( | const Util::String & | path, | |
bool | readOnly | |||
) | [static] |
set read-only status of a file
Set the read-only status of a file.
bool Win32::Win32FSWrapper::IsReadOnly | ( | const Util::String & | path | ) | [static] |
get read-only status of a file
Get the read-only status of a file.
bool Win32::Win32FSWrapper::DeleteFile | ( | const Util::String & | path | ) | [static] |
delete a file
Deletes a file. Returns true if the operation was successful. The delete will fail if the fail doesn't exist or the file is read-only.
bool Win32::Win32FSWrapper::DeleteDirectory | ( | const Util::String & | path | ) | [static] |
delete an empty directory
Delete an empty directory. Returns true if the operation was successful.
bool Win32::Win32FSWrapper::FileExists | ( | const Util::String & | path | ) | [static] |
return true if a file exists
Return true if a file exists.
bool Win32::Win32FSWrapper::DirectoryExists | ( | const Util::String & | path | ) | [static] |
return true if a directory exists
Return true if a directory exists.
FileTime Win32::Win32FSWrapper::GetFileWriteTime | ( | const Util::String & | path | ) | [static] |
get the last write-access time stamp of a file
Return the last write-access time to a file.
bool Win32::Win32FSWrapper::CreateDirectory | ( | const Util::String & | path | ) | [static] |
create a directory
Creates a new directory.
Array< String > Win32::Win32FSWrapper::ListFiles | ( | const Util::String & | dirPath, | |
const Util::String & | pattern | |||
) | [static] |
list all files in a directory
Lists all files in a directory, filtered by a pattern.
Array< String > Win32::Win32FSWrapper::ListDirectories | ( | const Util::String & | dirPath, | |
const Util::String & | pattern | |||
) | [static] |
list all subdirectories in a directory
Lists all subdirectories in a directory, filtered by a pattern. This will not return the special directories ".." and ".".
String Win32::Win32FSWrapper::GetUserDirectory | ( | ) | [static] |
get path to the current user's home directory (for user: standard assign)
This method should return the path to the current user's home directory. This is the directory where application can write their data to. Under windows, this is the "My Files" directory.
String Win32::Win32FSWrapper::GetTempDirectory | ( | ) | [static] |
get path to the current user's temp directory (for temp: standard assign)
This method should return a directory for temporary files with read/write access for the current user.
String Win32::Win32FSWrapper::GetHomeDirectory | ( | ) | [static] |
get path to the current application directory (for home: standard assign)
This method should return the installation directory of the application. Under Nebula3, this is either the directory where the executable is located, or 2 levels above the executable (if it is in home:bin/win32).
String Win32::Win32FSWrapper::GetBinDirectory | ( | ) | [static] |
get path to the current bin directory (for bin: standard assign)
This method sould return the directory where the application executable is located.