







The classes in the Microsoft.Speech.Recognition.SrgsGrammar namespace (and described in Use the SrgsGrammar Building Blocks (Microsoft.Speech)) can be used to create a grammar. The first code example in this topic creates a grammar with enough complexity to recognize three parts of speech (subject, verb, and direct object) in a simple declarative sentence such as "Mary bought an apple." The grammar recognizes six different subjects, five verbs, and five direct objects, a total of 150 distinct sentences that it can recognize. Counting the articles "a," "an," and "the" brings the total number of recognized sentences to 600.
The second example in this topic is the definition for an event handler for the SpeechRecognized event. This event handler uses the semantic keys ("theSubject", "theVerb", and "theObject") to determine the semantic values recognized by the grammar.
The third example in this topic shows an XML-format grammar that conforms to the Speech Recognition Grammar Specification (SRGS) Version 1.0 and is functionally equivalent to the grammar created in the first example.
Example 1: Create a Dynamic Grammar using SrgsGrammar
The following example creates a grammar using the classes available in the Microsoft.Speech.Recognition.SrgsGrammar namespace.
![]() |
---|
The SpeechRecognitionEngine instance, sre, is declared at the class level outside of the method shown in this example. |
C# |
![]() |
---|---|
private void Form1_Load(object sender, EventArgs e) { sre = new SpeechRecognitionEngine(new System.Globalization.CultureInfo("en-US")) // Create the "Subject" rule. SrgsRule subjRule = new SrgsRule("id_Subject"); SrgsOneOf subjList = new SrgsOneOf(new string[] { "I", "you", "he", "she", "Tom", "Mary" }); subjRule.Add(subjList); // Create the "Verb" rule. SrgsRule verbRule = new SrgsRule("id_Verb"); SrgsOneOf verbList = new SrgsOneOf(new string[] { "ate", "bought", "saw", "sold", "wanted" }); verbRule.Add(verbList); // Create the "Object" rule. SrgsRule objRule = new SrgsRule("id_Object"); SrgsOneOf objList = new SrgsOneOf(new string[] { "apple", "banana", "pear", "peach", "melon" }); objRule.Add(objList); // Create the root rule. // In this grammar, the root rule contains references to the other three rules. SrgsRule rootRule = new SrgsRule("Subj_Verb_Obj"); rootRule.Scope = SrgsRuleScope.Public; // Create the "Subject" and "Verb" rule references and add them to the SrgsDocument. SrgsRuleRef subjRef = new SrgsRuleRef(subjRule, "theSubject"); rootRule.Add(subjRef); SrgsRuleRef verbRef = new SrgsRuleRef(verbRule, "theVerb"); rootRule.Add(verbRef); // Add logic to handle articles: "the", "a", "and", occurring zero or one time. SrgsOneOf articles = new SrgsOneOf(new SrgsItem[] { (new SrgsItem(0, 1, "the")), new SrgsItem(0, 1, "a"), new SrgsItem(0, 1, "an") }); rootRule.Add(articles); // Create the "Object" rule reference and add it to the SrgsDocument. SrgsRuleRef objRef = new SrgsRuleRef(objRule, "theObject"); rootRule.Add(objRef); // Create an SrgsDocument object that contains all four rules. SrgsDocument document = new SrgsDocument(); document.Rules.Add(new SrgsRule[] { rootRule, subjRule, verbRule, objRule }); // Set "rootRule" as the root rule of the grammar. document.Root = rootRule; // Create a Grammar object, initializing it with the root rule. Grammar g = new Grammar(document, "Subj_Verb_Obj"); // Load the Grammar object into the recognizer. sr.LoadGrammar(g); // Produce an XML file that contains the grammar. System.Xml.XmlWriter writer = System.Xml.XmlWriter.Create("c:\\test\\srgsDocument.xml"); document.WriteSrgs(writer); writer.Close(); // Attach a handler for the SpeechRecognized event. sre.SpeechRecognized += new EventHandler<SpeechRecognizedEventArgs>(sre_SpeechRecognized); // Configure the input to the SpeechRecognitionEngine object. sre.SetInputToDefaultAudioDevice(); // Start asynchronous recognition. sre.RecognizeAsync(); } |
Example 2: Parse Semantic Content in Event Handler
The following is a handler for the SpeechRecognized event on the SpeechRecognitionEngine instance, sre, that is used in the preceding sample code. This handler is registered in the last line of the preceding sample. When the SpeechRecognized event is raised, the following handler is called. The e argument in this handler provides access to semantic values when speech is recognized.
C# |
![]() |
---|---|
void sre_SpeechRecognized(object sender, SpeechRecognizedEventArgs e) { string str = e.Result.Text; string subj = "unassigned", verb = "unassigned", obj = "unassigned"; bool errorCondition = false; if (e.Result != null) { if (e.Result.Semantics != null && e.Result.Semantics.Count != 0) { SemanticValue semantics = e.Result.Semantics; if (e.Result.Semantics.ContainsKey("theSubject")) { subj = e.Result.Semantics["theSubject"].Value.ToString(); } else errorCondition = true; if (e.Result.Semantics.ContainsKey("theVerb")) { verb = e.Result.Semantics["theVerb"].Value.ToString(); } else errorCondition = true; if (e.Result.Semantics.ContainsKey("theObject")) { obj = e.Result.Semantics["theObject"].Value.ToString(); } else errorCondition = true; if (!errorCondition) { MessageBox.Show(String.Format( "Reco string: {0}\nSubject: {1}\nVerb: {2}\nObject: {3}", str, subj, verb, obj)); } else { MessageBox.Show( "e.Result.Semantics is null or e.Result.Semantics.Count == 0"); } } else { MessageBox.Show("e.Result is null"); } } |
Example 3: SRGS XML Grammar
The following XML grammar can be used to recognize simple declarative sentences of the form <Subject><Verb><article (optional)><Object> with varying subjects, verbs, and direct objects. A typical sentence that is recognized is "Mary bought the apple."
In this grammar, the subject can be "I", "you", "he", "she", "Tom", or "Mary". The verb can be "ate", "bought", "saw", "sold", or "wanted". The object can be "apple", "banana", "pear", "peach", or "melon".
In the following illustration, a spoken sentence is recognized as long as it contains one of the words in the first one-of element, a word in the second one-of element, and a word in the fourth one-of element. The "0-1" notations on the items in the third one-of element represent the minimum and maximum repetition counts for these words, and mean that an input sentence can contain zero or one of the words in the third one-of element.
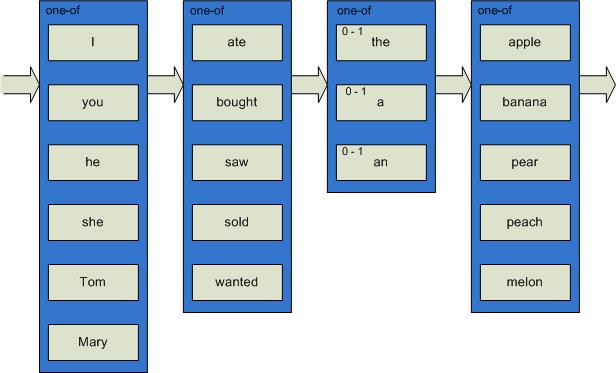
This grammar consists of four rules. The first rule, "Subj_Verb_Obj", is the root rule and consists of the following elements:
-
A ruleref element that references a rule that recognizes the valid subjects for an input sentence.
-
A ruleref that references a rule that recognizes the valid verbs for the sentence.
-
A one-of element that recognizes the articles "the", "a", and "an", which are made optional by the repeat attribute of their enclosing item elements.
-
A final ruleref element that references a rule that recognizes the valid direct objects for the sentence.
For more information about these SRGS elements, see ruleref Element (Microsoft.Speech), one-of Element (Microsoft.Speech), and item Element (Microsoft.Speech).
The following XML example is functionally equivalent to the grammar created in Example 1, above. The XML example uses syntax according the format "semantics-ms/1.0", which is declared in the opening tag of the grammar element. The symbol "$" refers to the Rule Variable of the containing rule element. The expression "$.Subject" refers to the property named "Subject" of the Rule Variable on the rule "Subj_Verb_Obj". The symbol "$$" refers to the latest result from the rule that is the target of the rule reference, and does not specify a property on the target rule. See Semantic Results Content (Microsoft.Speech) and Grammar Rule Reference Referencing (Microsoft.Speech) for more information.
XML |
![]() |
---|---|
<?xml version="1.0" encoding="UTF-8" ?> <grammar version="1.0" xml:lang="en-US" mode="voice" root="Subj_Verb_Obj" tag-format="semantics-ms/1.0" xmlns="http://www.w3.org/2001/06/grammar" > <rule id="Subj_Verb_Obj" scope="public"> <ruleref uri="#id_Subject" /> <tag> $.Subject = $$; </tag> <ruleref uri="#id_Verb" /> <tag> $.Verb = $$; </tag> <one-of> <item repeat="0-1">the</item> <item repeat="0-1">a</item> <item repeat="0-1">an</item> </one-of> <ruleref uri="#id_Object" /> <tag> $.Object = $$; </tag> </rule> <rule id="id_Subject" scope="private"> <one-of> <item> I </item> <item> you </item> <item> he </item> <item> she </item> <item> Tom </item> <item> Mary </item> </one-of> </rule> <rule id="id_Verb" scope="private"> <one-of> <item> ate </item> <item> bought </item> <item> saw </item> <item> sold </item> <item> wanted </item> </one-of> </rule> <rule id="id_Object" scope="private"> <one-of> <item> apple </item> <item> banana </item> <item> pear </item> <item> peach </item> <item> melon </item> </one-of> </rule> </grammar> |