![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
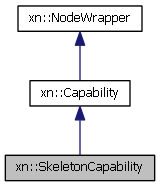
Detailed Description
Purpose: Provides the 'Skeleton' functionality for a xn::UserGenerator node. This capability lets the node generate a skeleton representation for each human user generated by the node.
- Note:
- See Meanings of 'User'.
Usage: Do not instantiate directly. Instead, use UserGenerator::GetSkeletonCap() to obtain an instance.
Data output: Skeleton.
The OpenNI definition of joints refers to a predefined set of points on the human body, as defined by the xn::XnSkeletonJoint enum type. Examples of these joints are XN_SKEL_HEAD, XN_SKEL_TORSO, XN_SKEL_LEFT_ELBOW, XN_SKEL_LEFT_WRIST and XN_SKEL_LEFT_HAND. Note that some of these joints are actual joints, in the conventional sense as termed by the English language, for example, XN_SKEL_LEFT_ELBOW and XN_SKEL_LEFT_WRIST; and other joints are in fact limbs, for example, XN_SKEL_HEAD and XN_SKEL_LEFT_HAND. However, OpenNI defines all these as joints, each joint with a single position coordinate. Each detected joint is defined by its position, which is held as a struct of type xn::XnSkeletonJointPosition, comprising a 3D position coordinate and confidence. The 3D position coordinate defines a point somewhere in the center of the 'joint', for example, the position of the torso will be at the center of the torso, i.e., somewhere in the stomach area.
To graphically draw a complete skeleton, therefore, each joint must be connected by a line to the adjacent point according to the form of the human body.
An application typically uses this skeleton representation to track human motion, or to graphically display all or part of the user as an avatar on screen, and optionally graphically enhance it to make it look more like a full humanoid figure.
Calibration
Tracking of a human user always starts with a process named 'Calibration'. In this process the algorithm performs some implementation-specific logic to enable it to track that user. there is no standard for what calibration is exactly and each implementation may use whatever it needs to produce and calibrate a skeleton. However, a typical way of calibrating the skeleton would be to measure distances between key joints for a particular user and record the data as the calibration data for that particular user.
Some implementations of the user generator node require a human user to pose a specific posture for the calibration process to succeed. The application can check if current implementation requires such a pose by calling NeedPoseForCalibration() method. If the answer is TRUE, the application should call GetCalibrationPose() to find out the name of the pose a human user should take in order to calibrate the algorithm. The application can use the xn::PoseDetectionCapability to find out if a human user is assuming a specific position, and once he does, calibration can start.
The application starts the calibration process by calling RequestCalibration(). There are several events raised during the calibration process, but the most important one is 'Calibration Complete'. Once calibration is done, the application may call StartTracking() to make the algorithm start tracking the skeleton of that human user.
Member Typedef Documentation
typedef void(* xn::SkeletonCapability::CalibrationComplete)(SkeletonCapability &skeleton, XnUserID user, XnCalibrationStatus calibrationError, void *pCookie) |
Signals that a specific user's skeleton has now completed the calibration process, and provides a result status.
Following is an example of a callback header to handle the 'Calibration Complete' event:
OnCalibrationComplete(xn::SkeletonCapability &skeleton, XnUserID user, XnCalibrationStatus calibrationError, void *pCookie)
- Parameters:
-
[in] skeleton SkeletonCapability object that raised the event. [in] user ID of the user holding SkeletonCapability object. [in] calibrationError Status of the calibration. Values are defined by XnCalibrationStatus. [in] pCookie User's cookie, to be delivered to the callback.
typedef void(* xn::SkeletonCapability::CalibrationEnd)(SkeletonCapability &skeleton, XnUserID user, XnBool bSuccess, void *pCookie) |
Signals that a specific user's SkeletonCapability object has now completed the calibration process.
Example of a callback function to handle the 'Calibration Complete' event:
OnCalibrationEnd(xn::SkeletonCapability &skeleton, XnUserID user, XnCalibrationStatus calibrationError, void *pCookie)
Parameters:
hNode [in] Node that raised the event. user [in] ID of the user for which calibration was attempted. bSuccess [in] Indication of whether or not the calibration attempt succeeded. pCookie [in] User-provided cookie that was given when registering to this event.
typedef void(* xn::SkeletonCapability::CalibrationInProgress)(SkeletonCapability &skeleton, XnUserID user, XnCalibrationStatus calibrationError, void *pCookie) |
Signals that a specific user's skeleton is calibrating, and its current status.
Example of a callback function to handle the 'Calibration Progress' event:
OnCalibrationProgress(xn::SkeletonCapability &skeleton, XnUserID user, XnCalibrationStatus calibrationError, void *pCookie)
- Parameters:
-
[in] skeleton SkeletonCapability object that raised the event. [in] user ID of the user holding SkeletonCapability object. [in] calibrationError Status of the calibration. Values are defined by XnCalibrationStatus. [in] pCookie User's cookie, to be delivered to the callback.
Remarks
After the 'Calibration Start' event, the 'Calibration in Progress' event is raised every frame until 'Calibration Complete' is raised. It informs the application as to the current state of the calibration process.
typedef void(* xn::SkeletonCapability::CalibrationStart)(SkeletonCapability &skeleton, XnUserID user, void *pCookie) |
Signals that a specific user's SkeletonCapability object is now starting the calibration process.
Example of a callback function to handle the 'Calibration Start' event:
OnCalibrationStart(xn::SkeletonCapability& skeleton, XnUserID user, void* pCookie)
- Parameters:
-
[in] skeleton SkeletonCapability object that raised the event. [in] user The id of the user that's being calibrated. [in] pCookie A user-provided cookie that was given when registering to this event.
Remarks
The application itself initiates calibration by calling the RequestCalibration() method. When a SkeletonCapability object actually starts to perform the calibration, it raises this event.
Constructor & Destructor Documentation
xn::SkeletonCapability::SkeletonCapability | ( | XnNodeHandle | hNode | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::SkeletonCapability::SkeletonCapability | ( | const NodeWrapper & | node | ) | [inline] |
Member Function Documentation
Aborts an existing calibration process currently being executed for a specified user.
- Parameters:
-
[in] user ID of the user.
Remarks
Raises the 'Calibration Complete' event.
XnStatus xn::SkeletonCapability::ClearCalibrationData | ( | XnUInt32 | nSlot | ) | [inline] |
Clears a specified slot of any saved calibration data.
- Parameters:
-
[in] nSlot Slot specifier.
XnStatus xn::SkeletonCapability::EnumerateActiveJoints | ( | XnSkeletonJoint * | pJoints, |
XnUInt16 & | nJoints | ||
) | const [inline] |
Get all active joints.
For full details and usage, see xnEnumerateActiveJointsXnStatus xn::SkeletonCapability::GetCalibrationPose | ( | XnChar * | strPose | ) | const [inline] |
Gets the name of the pose that is required for calibration. The pose and its name reside in the plug-in module that provides the SkeletonCapability. The pose's name and details are defined by the developer of the module.
- Parameters:
-
[out] strPose Name of the pose required for calibration Remarks
This method is to be used only if the NeedPoseForCalibration() method returns TRUE, and only then will this method returns a name.
XnStatus xn::SkeletonCapability::GetSkeletonJoint | ( | XnUserID | user, |
XnSkeletonJoint | eJoint, | ||
XnSkeletonJointTransformation & | Joint | ||
) | const [inline] |
Gets all available information about a specific joint in the skeleton.
- Parameters:
-
[in] user ID of the user. [in] eJoint Joint specifier. [out] Joint Transformation structure for the joint. Includes position and orientation.
XnStatus xn::SkeletonCapability::GetSkeletonJointOrientation | ( | XnUserID | user, |
XnSkeletonJoint | eJoint, | ||
XnSkeletonJointOrientation & | Joint | ||
) | const [inline] |
Gets the orientation of a specific skeleton joint.
- Parameters:
-
[in] user ID of the user. [in] eJoint Joint specifier. [out] Joint Joint's orientation.
XnStatus xn::SkeletonCapability::GetSkeletonJointPosition | ( | XnUserID | user, |
XnSkeletonJoint | eJoint, | ||
XnSkeletonJointPosition & | Joint | ||
) | const [inline] |
Gets the position of one of the skeleton joints in the most recently generated user data.
- Parameters:
-
[in] user ID of the user. [in] eJoint Joint specifier. [out] Joint Latest position of the joint.
Remarks
The joint position is returned as a XnSkeletonJointPosition structure, comprising a real-world coordinate together with a confidence rating.
Confidence can be in the range of 0..1.
0 means 'I'm guessing here'.
1 means 'This is where it is'.
The API is ready for when an implementation decides it is 'fairly certain'. In a typical implementation, 0.5 is used when there is an educated guess, or have run some heuristics to determine the position.
XnBool xn::SkeletonCapability::IsCalibrated | ( | XnUserID | user | ) | const [inline] |
Returns whether a user has been calibrated. see Calibration.
- Parameters:
-
[in] user ID of the user.
Remarks
This is a utility method. It is an alternative approach to saving the states from the events that notify stages in calibration.
XnBool xn::SkeletonCapability::IsCalibrating | ( | XnUserID | user | ) | const [inline] |
Returns whether a user is being calibrated right now. see Calibration.
- Parameters:
-
[in] user ID of the user.
Remarks
This is a utility method. It is an alternative approach to saving the states from the events that notify stages in calibration.
XnBool xn::SkeletonCapability::IsCalibrationData | ( | XnUInt32 | nSlot | ) | const [inline] |
Returns whether a specific slot already holds calibration data.
- Parameters:
-
[in] nSlot Slot specifier.
XnBool xn::SkeletonCapability::IsJointActive | ( | XnSkeletonJoint | eJoint | ) | const [inline] |
Checks if a specific joint is tracked or not.
- Parameters:
-
[in] eJoint Specifies the joint.
XnBool xn::SkeletonCapability::IsJointAvailable | ( | XnSkeletonJoint | eJoint | ) | const [inline] |
Returns whether a specific skeleton joint is supported by the SkeletonCapability object.
- Parameters:
-
[in] eJoint Joint to check.
XnBool xn::SkeletonCapability::IsProfileAvailable | ( | XnSkeletonProfile | eProfile | ) | const [inline] |
Returns whether a specific skeleton profile is supported by the SkeletonCapability object.
- Parameters:
-
[in] eProfile Profile to check.
XnBool xn::SkeletonCapability::IsTracking | ( | XnUserID | user | ) | const [inline] |
Returns whether a user is currently being tracked.
- Parameters:
-
[in] user ID of the user.
Remarks
This is a utility method. It is an alternative approach to saving the states from the events that notify stages in calibration and tracking.
Loads calibration data of a specified user. The calibration data includes the lengths of the human user's limbs.
- Parameters:
-
[in] user ID of the user. [in] nSlot Slot to use for saving the calibration data
XnStatus xn::SkeletonCapability::LoadCalibrationDataFromFile | ( | XnUserID | user, |
const XnChar * | strFileName | ||
) | [inline] |
Loads skeleton calibration data from a file to a skeleton.
- Parameters:
-
[in] user ID of the user. [in] strFileName File to load the calibration data from.
XnBool xn::SkeletonCapability::NeedPoseForCalibration | ( | ) | const [inline] |
Returns whether a specific pose is required for calibration. This setting is applicable to all users.
Remarks
This is a setting made by the developer of the SkeletonCapability plug-in.
XnStatus xn::SkeletonCapability::RegisterToCalibrationComplete | ( | CalibrationComplete | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Calibration Complete' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::SkeletonCapability::RegisterToCalibrationInProgress | ( | CalibrationInProgress | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Calibration In Progress' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::SkeletonCapability::RegisterToCalibrationStart | ( | CalibrationStart | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Calibration Start' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
See Calibration for information about calibration.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::SkeletonCapability::RegisterToJointConfigurationChange | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Joint Configuration Change' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
Starts the calibration process to calibrate a user.
- Parameters:
-
[in] user ID of the user. [in] bForce Disregard previous calibration to force a further calibration.
Values:
True: Disregards any previous calibration and forces a new calibration.
False: Starts the calibration process only if no calibration data is available for the skeleton.
This means that if the user was previously calibrated (which actually means measuring the length of its limbs, and estimating its torso, to be used in its skeletal representation), and another calibration was requested, to either make do with what we have (false), or forget the previous measurements and perform calibration again.
Remarks
This method is typically called from inside an event handler for the 'Pose Detected' event, signaling that a pose was detected in the FOV. Once a valid pose has been detected the application will want to start calibrating the user.
It can also be called outside of that, in implementations that don't require a pose.
Discards a skeleton's calibration.
- Parameters:
-
[in] user ID of the user.
Remarks
Utility method for resetting a user to its initial state. For use in development.
Saves the calibration data of a specified user. The calibration data includes the lengths of the human user's limbs.
- Parameters:
-
[in] user ID of the user. [in] nSlot Slot to use for saving the calibration data
Remarks
The slots mechanism allows saving the calibration to memory instead of to files. When used, a calibration is saved to a slot, and may be reloaded from it.
XnStatus xn::SkeletonCapability::SaveCalibrationDataToFile | ( | XnUserID | user, |
const XnChar * | strFileName | ||
) | [inline] |
Saves the skeleton's current calibration data to a file.
- Parameters:
-
[in] user ID of the user. [in] strFileName Name of the file for saving the calibration data.
Remarks
The application is the one that should match the user, however it sees fit. For example, if a calibration was saved as C:\john_smith.bin, and then later another application recognizes John Smith (let's say, through face recognition), the calibration data can be loaded, instead of performing the entire calibration process.
This is a very useful tool for developers. They can save their own calibration, and test their application without calibrating each time (going into pose, spend time on calibration).
XnStatus xn::SkeletonCapability::SetJointActive | ( | XnSkeletonJoint | eJoint, |
XnBool | bState | ||
) | [inline] |
Changes the state of a specific skeleton joint to be active or inactive. The xn::UserGenerator node generates output data for the active joints only. This state setting applies to all skeletons that the xn::UserGenerator node generates.
- Parameters:
-
[in] eJoint Specifies the joint. [in] bState New activation state (true/false).
Remarks
This method lets the application set the skeleton profile down to a finer resolution than is possible with the SetSkeletonProfile() method.
The joint selector parameter (xn::XnSkeletonJoint) lets the application choose specific parts of a skeleton, e.g., a specific hand or foot.
Since this method provides the flexibility to request only the data that the application requires, this can help to significantly improve response time. Setting a smaller skeleton profile can reduce the processing load on the computer, which can cause a significant increase in avatar responsiveness (implementation-dependent). Some implementations may produce all the data they can, and only filter it at the end according to the configuration.
XnStatus xn::SkeletonCapability::SetSkeletonProfile | ( | XnSkeletonProfile | eProfile | ) | [inline] |
Sets the skeleton profile. The skeleton profile specifies which joints are to be active, and which to be inactive. The UserGenerator node generates output data for the active joints only. This profile applies to all skeletons that the UserGenerator node generates.
- Parameters:
-
[in] eProfile Specifies the profile to set.
Remarks
The profile (xn::XnSkeletonProfile) enables the application to choose between generating all joints, just the upper or lower torso, or just the head and hands only.
Since this method provides the flexibility to request only the data that the application requires, this can help to significantly improve response time: setting a smaller skeleton profile reduces the processing load on the computer, which can cause a significant increase in avatar responsiveness.
Use the SetJointActive() method for selecting the profile down to a finer resolution than possible with this method, e.g., to be able to select a specific hand or foot.
OpenNI does not standardize the initial profile set. If no profile is set, each implementation may have its own default profile. For example, PrimeSense implementation's default profile is NONE. This means if no profile is set, no joint will be available.
Setting a profile changes all the joint configuration to that profile. This means you can set a profile, then refine specific joints (adding or removing). You can't do it the other way around.
XnStatus xn::SkeletonCapability::SetSmoothing | ( | XnFloat | fSmoothingFactor | ) | [inline] |
Sets the smoothing factor for all users generated by this xn::UserGenerator node.
- Parameters:
-
[in] fSmoothingFactor Smoothing factor
Range: 0 to 1
Remarks
The human body is identified from its physical depth. Since the human body is not absolutely steady, the human body being calibrated may be too jittery to easily obtain its position. This method provides an algorithm to smooth the jitter. You provide the desired smoothing factor as a parameter.
Starts tracking a skeleton.
- Parameters:
-
[in] user ID of the user.
Remarks
This method is used for tracking the motion of the skeleton, representing a human user body, within a real-life (3D) scene for analysis, interpretation, and use by the application.
While the skeleton is being tracked, the application can call the GetSkeletonJointPosition() method to get data about one of the joint positions.
Stops tracking a skeleton.
- Parameters:
-
[in] user ID of the user.
void xn::SkeletonCapability::UnregisterFromCalibrationComplete | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Calibration Complete' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::SkeletonCapability::UnregisterFromCalibrationInProgress | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Calibration In Progress' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
XnStatus xn::SkeletonCapability::UnregisterFromCalibrationStart | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters a handler from the 'Calibration Start' event.
- Parameters:
-
[in] hCallback Handle received from registration.
Remarks
Due to historical reasons, this function has an XnStatus return value. In practice, it will always succeed. The user can safely ignore the return value.
void xn::SkeletonCapability::UnregisterFromJointConfigurationChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Joint Configuration Change' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
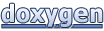