![]() |
OpenNI 1.5.4
|
Detailed Description
This page details functions for handling production nodes lists. The lists are implemented as doubly-linked lists, but it's not recommended to access the list members directly. instead, use the provided functions for handling the list.
Adding and Removing Node Info Objects
Nodes can be added to the list using xnNodeInfoListAdd(). Note that once an info object is part of the list, it will be freed if that list is freed. A node can be removed by calling xnNodeInfoListRemove(), which also frees that element.
A short example:
// Start with an empty list XnNodeInfoList* pList; nRetVal = xnNodeInfoListAllocate(&pList); // TODO: check error code // Add first pList = xnNodeInfoListAdd(pList, pDescription1, NULL, NULL); // Add second pList = xnNodeInfoListAdd(pList, pDescription2, NULL, NULL);
Enumeration
Forward Iteration:
for (XnNodeInfoListIterator it = xnNodeInfoListGetFirst(pList); xnNodeInfoListIteratorIsValid(it); it = xnNodeInfoListGetNext(it)) { XnNodeInfo* pCurrent = xnNodeInfoListGetCurrent(it); ... }
Backwards Iteration:
for (XnNodeInfoListIterator it = xnNodeInfoListGetLast(pList); xnNodeInfoListIteratorIsValid(it); it = xnNodeInfoListGetPrevious(it)) { XnNodeInfo* pCurrent = xnNodeInfoListGetCurrent(it); ... }
Function Documentation
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAdd | ( | XnNodeInfoList * | pList, |
const XnProductionNodeDescription * | pDescription, | ||
const XnChar * | strCreationInfo, | ||
XnNodeInfoList * | pNeededNodes | ||
) |
Creates and adds a single XnNodeInfo object to the list. See also xnNodeInfoListAddEx().
- Parameters:
-
pList [in] The list. pDescription [in] The description of this production node. strCreationInfo [in] Optional. Additional needed information for instantiation. pNeededNodes [in] Optional. A list of needed nodes.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAddEx | ( | XnNodeInfoList * | pList, |
const XnProductionNodeDescription * | pDescription, | ||
const XnChar * | strCreationInfo, | ||
XnNodeInfoList * | pNeededNodes, | ||
const void * | pAdditionalData, | ||
XnFreeHandler | pFreeHandler | ||
) |
Creates and adds a single XnNodeInfo object to the list, with additional data. This data can be later extracted using the xnNodeInfoGetAdditionalData(). Before the node info object is freed, the pFreeHandler callback will be called, so it could free the additional data object.
- Parameters:
-
pList [in] The list. pDescription [in] The description of this production node. strCreationInfo [in] Optional. Additional needed information for instantiation. pNeededNodes [in] Optional. A list of needed nodes. pAdditionalData [in] Additional data, which is specific to this node. pFreeHandler [in] Optional. A callback function for freeing the additional data.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAddNode | ( | XnNodeInfoList * | pList, |
XnNodeInfo * | pNode | ||
) |
Adds a single XnNodeInfo object to the list.
- Parameters:
-
pList [in] The list. pNode [in] The node to add.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAddNodeFromList | ( | XnNodeInfoList * | pList, |
XnNodeInfoListIterator | otherListIt | ||
) |
Adds a node from another list to this list (the node is not removed from the other list).
- Parameters:
-
pList [in] The list. otherListIt [in] An iterator obtained from another list.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAllocate | ( | XnNodeInfoList ** | ppList | ) |
Allocates a XnNodeInfoList object. This object should be freed using xnNodeInfoListFree().
- Parameters:
-
ppList [out] The list.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListAppend | ( | XnNodeInfoList * | pList, |
XnNodeInfoList * | pOther | ||
) |
Appends another list at the end of this list. Note that the other list becomes empty, but still needs to be freed.
- Parameters:
-
pList [in] A list. pOther [in] The list to be appended.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListClear | ( | XnNodeInfoList * | pList | ) |
Clears a node info list, freeing all the elements in it.
- Parameters:
-
pList [in] The list to be freed.
XN_C_API void XN_C_DECL xnNodeInfoListFree | ( | XnNodeInfoList * | pList | ) |
Frees a XnNodeInfoList object previously allocated with xnNodeInfoListAllocate().
- Parameters:
-
pList [out] The list.
XN_C_API XnNodeInfo* XN_C_DECL xnNodeInfoListGetCurrent | ( | XnNodeInfoListIterator | it | ) |
Gets current element from an iterator.
- Parameters:
-
it [in] An iterator.
- Returns:
- an XnNodeInfo pointer.
XN_C_API XnNodeInfoListIterator XN_C_DECL xnNodeInfoListGetFirst | ( | XnNodeInfoList * | pList | ) |
Gets the first element of the list.
- Parameters:
-
pList [in] [Optional] A list.
- Returns:
- an iterator to the first element of the list, or NULL if the list is empty.
XN_C_API XnNodeInfoListIterator XN_C_DECL xnNodeInfoListGetLast | ( | XnNodeInfoList * | pList | ) |
Gets the last element of the list.
- Parameters:
-
pList [in] [Optional] A list.
- Returns:
- an iterator to the last element of the list, or NULL if the list is empty.
XN_C_API XnNodeInfoListIterator XN_C_DECL xnNodeInfoListGetNext | ( | XnNodeInfoListIterator | it | ) |
Gets an iterator to the next element from a current iterator.
- Parameters:
-
it [in] An iterator.
- Returns:
- an iterator to the next element.
XN_C_API XnNodeInfoListIterator XN_C_DECL xnNodeInfoListGetPrevious | ( | XnNodeInfoListIterator | it | ) |
Gets an iterator to the previous element from a current iterator.
- Parameters:
-
it [in] An iterator.
- Returns:
- an iterator to the previous element.
XN_C_API XnBool XN_C_DECL xnNodeInfoListIsEmpty | ( | XnNodeInfoList * | pList | ) |
Checks if the given list is empty
- Parameters:
-
pList [in] A list.
XN_C_API XnBool XN_C_DECL xnNodeInfoListIteratorIsValid | ( | XnNodeInfoListIterator | it | ) |
Checks if the current iterator points to a valid location.
- Parameters:
-
it [in] An iterator.
XN_C_API XnStatus XN_C_DECL xnNodeInfoListRemove | ( | XnNodeInfoList * | pList, |
XnNodeInfoListIterator | it | ||
) |
Removes an element from the list, and frees it.
- Parameters:
-
pList [in] The list. it [in] Iterator to the element that should be removed.
Generated on Wed May 16 2012 10:16:06 for OpenNI 1.5.4 by
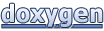