![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
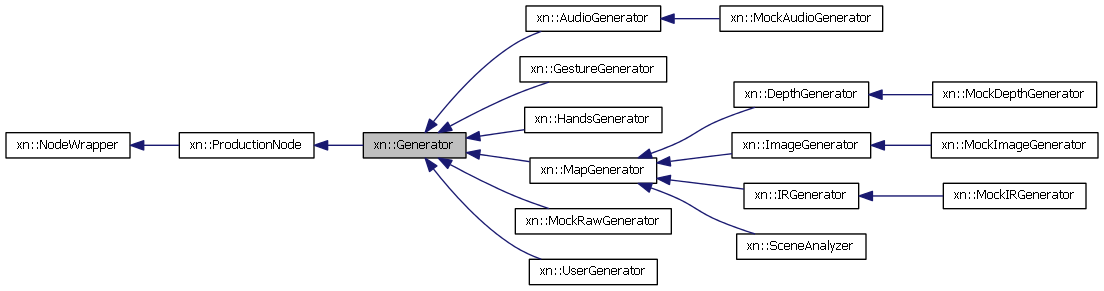
Detailed Description
Purpose: A generator node produces some type of data. This is in addition to the node's basic characteristic, as a derivative of the xn::ProductionNode class, i.e., that it represents a logical entity in the OpenNI Production Graph".
Usage summary: Immediate base class for all generator types. It itself is not usually instantiated.
Class Overview:
Each generator can be in Generating state or Non-Generating state. The application makes the node enter Generating state by calling the xn::Generator::StartGenerating() method.
When a generator node is created, by default it is in Non-Generating state, so it does not generate data.
Generators have a default configuration (for example the FOV resolution) and an application can change the default configuration before actual data generation starts.
Once the application has completed configuring the generator node, the application can cause the node to start generating data.
Typically, the application will configure the generator only in the configuration stage, i.e., in Non-Generating state. The application can usually also configure the node while generating data, however configuration might then take longer and the application could also lose some frames of data. For example, if the application changes the resolution while in the Configuration stage (Non-Generating state), the resolution change occurs immediately; but if the application changes the configuration while the generator is generating data, the resolution change can take up to a few seconds to occur.
Data Storage:
Each call to an 'Update Data' method updates the generator node's application buffer, making the latest data available for applications to access. After a call to an 'Update Data' method, all subsequent calls to GetData() will return exactly the same data until you call 'Update Data' again. The 'Update Data' method can be the generator node's own WaitAndUpdateData() method or one of the Context's 'WaitXUpdateAll' methods.
Getting the Generated Data:
Generator nodes will not output data to make it available for getting, until the application calls the node's WaitAndUpdateData() method (see below) or one of the context's 'WaitXUpdateAll' methods.
Constructor & Destructor Documentation
xn::Generator::Generator | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::Generator::Generator | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
const AlternativeViewPointCapability xn::Generator::GetAlternativeViewPointCap | ( | ) | const [inline] |
Gets an AlternativeViewPointCapability object for accessing Alternative Viewpoint functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_ALTERNATIVE_VIEW_POINT is supported by calling xn::Generator::IsCapabilitySupported().
AlternativeViewPointCapability xn::Generator::GetAlternativeViewPointCap | ( | ) | [inline] |
Gets an AlternativeViewPointCapability object for accessing Alternative Viewpoint functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_ALTERNATIVE_VIEW_POINT is supported by calling xn::Generator::IsCapabilitySupported().
const void* xn::Generator::GetData | ( | ) | [inline] |
Gets the frame data from the generator node. This is the latest data that the generator node has generated.
Remarks:
This method gets the data directly from the Generator node; not from the metadata object.
Compare this method with the Data() method of the xn::OutputMetaData object, where the latter gets the data from the metadata object. GetData() returns the same data as the GetMetaData().Data() method. However, using the GetData() method is slightly more costly.
The application usually should not use this method, but call each generator own data access methods, e.g. DepthGenerator::GetDepthMap().
XnUInt32 xn::Generator::GetDataSize | ( | ) | const [inline] |
Gets the data size of the frame data. This method gets the data size directly from the Generator node.
Remarks:
The size of the data is returned as a number of bytes.
Compare this method with the DataSize() method of the xn::OutputMetaData object, where the latter gets the data from the metadata object. GetDataSize() returns the same data as the GetMetaData().DataSize() method. However, using the GetDataSize() method is slightly more costly.
XnUInt32 xn::Generator::GetFrameID | ( | ) | const [inline] |
Gets the frame ID of the current frame data from the Generator node.
Remarks:
This method gets the frame ID directly from the Generator node.
Compare this method with the GetFrameID() method of the xn::OutputMetaData object, where the latter gets the data from the metadata object. GetFrameID() returns the same data as the GetMetaData().GetFrameID() method. However, using the GetFrameID() method is slightly more costly.
const FrameSyncCapability xn::Generator::GetFrameSyncCap | ( | ) | const [inline] |
Gets an FrameSyncCapability object for accessing Frame Sync functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_FRAME_SYNC is supported by calling xn::Generator::IsCapabilitySupported().
FrameSyncCapability xn::Generator::GetFrameSyncCap | ( | ) | [inline] |
Gets an FrameSyncCapability object for accessing Frame Sync functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_FRAME_SYNC is supported by calling xn::Generator::IsCapabilitySupported().
const MirrorCapability xn::Generator::GetMirrorCap | ( | ) | const [inline] |
Gets a MirrorCapability object for accessing Mirror functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_MIRROR is supported by calling xn::ProductionNode::IsCapabilitySupported().
MirrorCapability xn::Generator::GetMirrorCap | ( | ) | [inline] |
Gets a MirrorCapability object for accessing Mirror functionality.
Remarks:
It is the application's responsibility to check first if XN_CAPABILITY_MIRROR is supported by calling xn::ProductionNode::IsCapabilitySupported().
XnUInt64 xn::Generator::GetTimestamp | ( | ) | const [inline] |
Gets the frame timestamp from the Generator node This method gets the timestamp directly from the Generator node.
Remarks:
The time is returned in microseconds.
Compare this method with the GetTimestamp() method of the xn::OutputMetaData object, where the latter gets the data from the metadata object. GetTimestamp() returns the same data as the GetMetaData().GetTimestamp() method. However, using the GetTimestamp() method is slightly more costly.
XnBool xn::Generator::IsDataNew | ( | ) | const [inline] |
Returns whether this node's frame data was updated by the most recent call to any 'WaitXUpdateAll()' function, e.g., xn::Context::WaitAnyUpdateAll().
XnBool xn::Generator::IsGenerating | ( | ) | const [inline] |
Returns whether the node is currently in Generating state.
XnBool xn::Generator::IsNewDataAvailable | ( | XnUInt64 * | pnTimestamp = NULL | ) | const [inline] |
Returns whether the node has new data available. The new data is available for updating, but can not yet be accessed by the application. The application needs to call 'Update Data' to gain access to that new data.
- Parameters:
-
[out] pnTimestamp [Optional] If new data is available (TRUE is returned from the method), this param will hold the timestamp of that new data.
XnStatus xn::Generator::RegisterToGenerationRunningChange | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Generation State Change' event.
Registers a callback function to be called when generation starts or stops, i.e., when the generation state toggles between generating and not generating.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::Generator::RegisterToNewDataAvailable | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'New Data Available' event.
The 'New Data Available' event is raised whenever a generator node has new data available. The new data is available for updating, but can not yet be accessed by the application. The application needs to call 'Update Data' to gain access to that new data.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::Generator::StartGenerating | ( | ) | [inline] |
Enters the node into Generating state.
Enters the node into Generating state. In this state the node generates new frames.
After the application has called this method it can call an 'Update Data' method, e.g., xn::Generator::WaitAndUpdateData(), to make a new frame available for getting. The application can then get the data (for example, using a metadata GetData() method, or some other mechanism depending on the type of node).
Remarks:
On entering the node into Generating state, OpenNI enters also all dependent nodes into Generating state. For example, if a UserGenerator node depends on data input from a DepthGenerator node, and the UserGenerator node is entered into Generating state, then OpenNI will enter also the DepthGenerator node into Generating state.
XnStatus xn::Generator::StopGenerating | ( | ) | [inline] |
Makes the node leave Generating state (enters Non-Generating state).
Remarks:
If the node has metadata, after calling this method the data is still available as a saved frame object in the metadata object.
void xn::Generator::UnregisterFromGenerationRunningChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the 'Generation State Change' event handler.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::Generator::UnregisterFromNewDataAvailable | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the event handler for the 'New Data Available' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
XnStatus xn::Generator::WaitAndUpdateData | ( | ) | [inline] |
Updates the generator node to the latest available data, first waiting for the node to have new data available.
Remarks:
This method requests from OpenNI to cause the node to update its application buffers with new data, if it has new data available.
At this stage the generator node has "generated" new data. This method returns a success status. The application can now read the newly generated data.
An error situation is defined as: after a preset timeout, the node has not yet notified OpenNI it has new data available. On error, the method stops waiting and returns an error status.
- Return values:
-
XN_STATUS_INVALID_OPERATION This production node is not a generator. XN_STATUS_WAIT_DATA_TIMEOUT No new data available within 2 seconds.
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
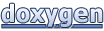