![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
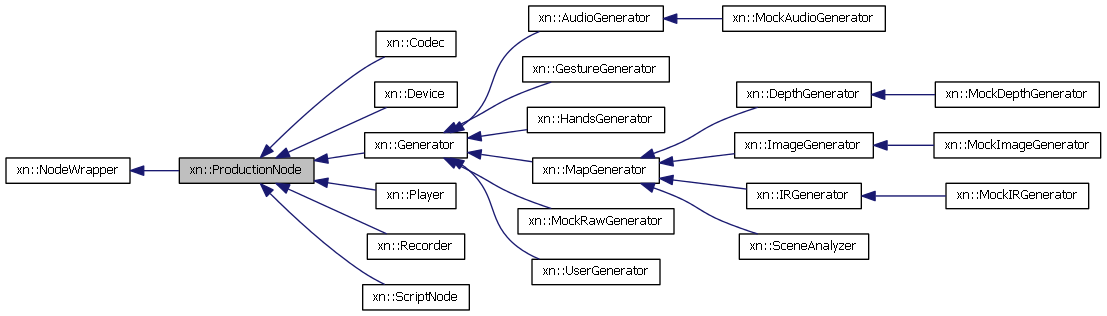
Public Member Functions | |
ProductionNode (XnNodeHandle hNode=NULL) | |
ProductionNode (const NodeWrapper &other) | |
NodeInfo | GetInfo () const |
XnStatus | AddNeededNode (ProductionNode &needed) |
XnStatus | RemoveNeededNode (ProductionNode &needed) |
void | GetContext (Context &context) const |
Context | GetContext () const |
XnBool | IsCapabilitySupported (const XnChar *strCapabilityName) const |
XnStatus | SetIntProperty (const XnChar *strName, XnUInt64 nValue) |
XnStatus | SetRealProperty (const XnChar *strName, XnDouble dValue) |
XnStatus | SetStringProperty (const XnChar *strName, const XnChar *strValue) |
XnStatus | SetGeneralProperty (const XnChar *strName, XnUInt32 nBufferSize, const void *pBuffer) |
XnStatus | GetIntProperty (const XnChar *strName, XnUInt64 &nValue) const |
XnStatus | GetRealProperty (const XnChar *strName, XnDouble &dValue) const |
XnStatus | GetStringProperty (const XnChar *strName, XnChar *csValue, XnUInt32 nBufSize) const |
XnStatus | GetGeneralProperty (const XnChar *strName, XnUInt32 nBufferSize, void *pBuffer) const |
XnStatus | LockForChanges (XnLockHandle *phLock) |
void | UnlockForChanges (XnLockHandle hLock) |
XnStatus | LockedNodeStartChanges (XnLockHandle hLock) |
void | LockedNodeEndChanges (XnLockHandle hLock) |
const ErrorStateCapability | GetErrorStateCap () const |
ErrorStateCapability | GetErrorStateCap () |
GeneralIntCapability | GetGeneralIntCap (const XnChar *strCapability) |
Detailed Description
Purpose: The ProductionNode class is a base class for all production nodes and generator nodes. As such, the ProductionNode class is the fundamental base class of the entire OpenNI interface for building and accessing the production graph.
Usage: Base class for all production nodes; not usually instantiated.
Class Overview:
The OpenNI Production Graph is comprised entirely of production nodes of one type or another. The ProductionNode class itself exposes a very limited functionality set. This is the most basic and common functionality set of all the nodes in the graph.
All types of core generator nodes are derived from this ProductionNode class, for example, the xn::DepthGenerator node and the xn::Device node. Each node type has its own set of methods that it supports.
Two of the most important methods provided by the ProductionNode class are IsCapabilitySupported() and GetContext(), both described further below.
Capabilities Mechanism:
In addition to being the base class for all core generator nodes, the ProductionNode class also provides key support for the OpenNI capabilities mechanism. OpenNI defines core functionality which is always supported by all node implementations of a specific node type. Additional optional functionality is also defined by OpenNI and exposed as "capabilities". The ProductionNode class provides the IsCapabilitySupported() method to check if this specific implementation supports a certain capability.
For a comprehensive overview to the Capabilities mechanism see Capabilities Mechanism.
Constructor & Destructor Documentation
xn::ProductionNode::ProductionNode | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::ProductionNode::ProductionNode | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
XnStatus xn::ProductionNode::AddNeededNode | ( | ProductionNode & | needed | ) | [inline] |
Adds another node to the list of needed nodes for this node.
For full details and usage, see xnAddNeededNodevoid xn::ProductionNode::GetContext | ( | Context & | context | ) | const [inline] |
Gets the node's context.
- Parameters:
-
[out] context The node's context.
Remarks
This method is useful for applications that have to take into account the possibility of multiple contexts. Using this method, the application can access all other nodes and other resources within the same context.
Context xn::ProductionNode::GetContext | ( | ) | const [inline] |
Gets the node's context.
Remarks
This method is useful for applications that have to take into account the possibility of multiple contexts. Using this method, the application can access all other nodes and other resources within the same context.
const ErrorStateCapability xn::ProductionNode::GetErrorStateCap | ( | ) | const [inline] |
Gets an xn::ErrorStateCapability object for accessing the functionality of the Error State capability.
Remarks: The application is responsible for first checking if XN_CAPABILITY_ERROR_STATE is supported by calling xn::ProductionNode::IsCapabilitySupported().
ErrorStateCapability xn::ProductionNode::GetErrorStateCap | ( | ) | [inline] |
Gets an xn::ErrorStateCapability object for accessing the functionality of the Error State capability.
Remarks: The application is responsible for first checking if XN_CAPABILITY_ERROR_STATE is supported by calling xn::ProductionNode::IsCapabilitySupported()
GeneralIntCapability xn::ProductionNode::GetGeneralIntCap | ( | const XnChar * | strCapability | ) | [inline] |
Gets an GeneralIntCapability object for accessing the capability functionality.
Remarks:
It is the application responsibility to check first if the capability is supported by calling IsCapabilitySupported().
- Parameters:
-
[in] strCapability Name of the capability to get
XnStatus xn::ProductionNode::GetGeneralProperty | ( | const XnChar * | strName, |
XnUInt32 | nBufferSize, | ||
void * | pBuffer | ||
) | const [inline] |
Gets a buffer property.
For full details and usage, see xnGetGeneralPropertyNodeInfo xn::ProductionNode::GetInfo | ( | ) | const [inline] |
Gets information about the node.
Remarks
This method returns a xn::NodeInfo object containing information such as the node description and the identities of dependent nodes.
XnStatus xn::ProductionNode::GetIntProperty | ( | const XnChar * | strName, |
XnUInt64 & | nValue | ||
) | const [inline] |
Gets an integer property.
For full details and usage, see xnGetIntPropertyXnStatus xn::ProductionNode::GetRealProperty | ( | const XnChar * | strName, |
XnDouble & | dValue | ||
) | const [inline] |
Gets a real property.
For full details and usage, see xnGetRealPropertyXnStatus xn::ProductionNode::GetStringProperty | ( | const XnChar * | strName, |
XnChar * | csValue, | ||
XnUInt32 | nBufSize | ||
) | const [inline] |
Gets a string property.
For full details and usage, see xnGetStringPropertyXnBool xn::ProductionNode::IsCapabilitySupported | ( | const XnChar * | strCapabilityName | ) | const [inline] |
Returns whether a production node supports a specific capability.
- Parameters:
-
[in] strCapabilityName The capability name to check.
Remarks
The application specifies the capability by supplying the capability's name in the strCapabilityName parameter. Before attempting to access any capability this method should be used to check that this node actually supports this capability.
void xn::ProductionNode::LockedNodeEndChanges | ( | XnLockHandle | hLock | ) | [inline] |
Ends changes request on a locked node.
For full details and usage, see xnLockedNodeEndChangesXnStatus xn::ProductionNode::LockedNodeStartChanges | ( | XnLockHandle | hLock | ) | [inline] |
Start changes request on a locked node, without releasing that lock.
For full details and usage, see xnLockedNodeStartChangesXnStatus xn::ProductionNode::LockForChanges | ( | XnLockHandle * | phLock | ) | [inline] |
Locks a node, not allowing any changes (any "set" function).
For full details and usage, see xnLockNodeForChangesXnStatus xn::ProductionNode::RemoveNeededNode | ( | ProductionNode & | needed | ) | [inline] |
Removes a needed node from the list of needed nodes.
For full details and usage, see xnRemoveNeededNodeXnStatus xn::ProductionNode::SetGeneralProperty | ( | const XnChar * | strName, |
XnUInt32 | nBufferSize, | ||
const void * | pBuffer | ||
) | [inline] |
Sets a buffer property.
For full details and usage, see xnSetGeneralPropertyXnStatus xn::ProductionNode::SetIntProperty | ( | const XnChar * | strName, |
XnUInt64 | nValue | ||
) | [inline] |
Sets an integer property.
For full details and usage, see xnSetIntPropertyXnStatus xn::ProductionNode::SetRealProperty | ( | const XnChar * | strName, |
XnDouble | dValue | ||
) | [inline] |
Sets a real property.
For full details and usage, see xnSetRealPropertyXnStatus xn::ProductionNode::SetStringProperty | ( | const XnChar * | strName, |
const XnChar * | strValue | ||
) | [inline] |
Sets a string property.
For full details and usage, see xnSetStringPropertyvoid xn::ProductionNode::UnlockForChanges | ( | XnLockHandle | hLock | ) | [inline] |
Unlocks a previously locked node.
For full details and usage, see xnUnlockNodeForChangesThe documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
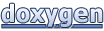