![]() |
OpenNI 1.5.4
|
XnCppWrapper.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_CPP_WRAPPER_H__ 00023 #define __XN_CPP_WRAPPER_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnOpenNI.h> 00029 #include <XnCodecIDs.h> 00030 00031 //--------------------------------------------------------------------------- 00032 // Types 00033 //--------------------------------------------------------------------------- 00034 namespace xn 00035 { 00036 //--------------------------------------------------------------------------- 00037 // Forward Declarations 00038 //--------------------------------------------------------------------------- 00039 class ProductionNode; 00040 class EnumerationErrors; 00041 class NodeInfo; 00042 class NodeInfoList; 00043 class Context; 00044 class Query; 00045 class Generator; 00046 00072 //--------------------------------------------------------------------------- 00073 // Types 00074 //--------------------------------------------------------------------------- 00075 00101 typedef void (XN_CALLBACK_TYPE* StateChangedHandler)(ProductionNode& node, void* pCookie); 00102 00103 //--------------------------------------------------------------------------- 00104 // Internal stuff 00105 //--------------------------------------------------------------------------- 00106 typedef XnStatus (*_XnRegisterStateChangeFuncPtr)(XnNodeHandle hNode, XnStateChangedHandler handler, void* pCookie, XnCallbackHandle* phCallback); 00107 typedef void (*_XnUnregisterStateChangeFuncPtr)(XnNodeHandle hNode, XnCallbackHandle hCallback); 00108 00109 static XnStatus _RegisterToStateChange(_XnRegisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback); 00110 static void _UnregisterFromStateChange(_XnUnregisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, XnCallbackHandle hCallback); 00111 00112 //--------------------------------------------------------------------------- 00113 // Some Utilities 00114 //--------------------------------------------------------------------------- 00119 class Version 00120 { 00121 public: 00122 Version(const XnVersion& version) : m_version(version) {} 00123 Version(XnUInt8 nMajor, XnUInt8 nMinor, XnUInt16 nMaintenance, XnUInt32 nBuild) 00124 { 00125 m_version.nMajor = nMajor; 00126 m_version.nMinor = nMinor; 00127 m_version.nMaintenance = nMaintenance; 00128 m_version.nBuild = nBuild; 00129 } 00130 00131 bool operator==(const Version& other) const 00132 { 00133 return (xnVersionCompare(&m_version, &other.m_version) == 0); 00134 } 00135 bool operator!=(const Version& other) const 00136 { 00137 return (xnVersionCompare(&m_version, &other.m_version) != 0); 00138 } 00139 bool operator<(const Version& other) const 00140 { 00141 return (xnVersionCompare(&m_version, &other.m_version) < 0); 00142 } 00143 bool operator<=(const Version& other) const 00144 { 00145 return (xnVersionCompare(&m_version, &other.m_version) <= 0); 00146 } 00147 bool operator>(const Version& other) const 00148 { 00149 return (xnVersionCompare(&m_version, &other.m_version) > 0); 00150 } 00151 bool operator>=(const Version& other) const 00152 { 00153 return (xnVersionCompare(&m_version, &other.m_version) >= 0); 00154 } 00155 00156 static Version Current() 00157 { 00158 XnVersion version; 00159 xnGetVersion(&version); 00160 return Version(version); 00161 } 00162 00163 XnUInt8 Major() const { return m_version.nMajor; } 00164 XnUInt8 Minor() const { return m_version.nMinor; } 00165 XnUInt16 Maintenance() const { return m_version.nMaintenance; } 00166 XnUInt32 Build() const { return m_version.nBuild; } 00167 00168 XnUInt8& Major() { return m_version.nMajor; } 00169 XnUInt8& Minor() { return m_version.nMinor; } 00170 XnUInt16& Maintenance() { return m_version.nMaintenance; } 00171 XnUInt32& Build() { return m_version.nBuild; } 00172 00173 const XnVersion* GetUnderlying() const { return &m_version; } 00174 XnVersion* GetUnderlying() { return &m_version; } 00175 00176 private: 00177 XnVersion m_version; 00178 }; 00179 00180 //--------------------------------------------------------------------------- 00181 // Meta Data 00182 //--------------------------------------------------------------------------- 00183 00198 class OutputMetaData 00199 { 00200 public: 00206 inline OutputMetaData(const XnUInt8** ppData) : m_ppData(ppData), m_nAllocatedSize(0), m_pAllocatedData(NULL) 00207 { 00208 xnOSMemSet(&m_output, 0, sizeof(XnOutputMetaData)); 00209 } 00210 00214 virtual ~OutputMetaData() { Free(); } 00215 00219 inline XnUInt64 Timestamp() const { return m_output.nTimestamp; } 00220 00224 inline XnUInt64& Timestamp() { return m_output.nTimestamp; } 00225 00230 inline XnUInt32 FrameID() const { return m_output.nFrameID; } 00231 00236 inline XnUInt32& FrameID() { return m_output.nFrameID; } 00237 00246 inline XnUInt32 DataSize() const { return m_output.nDataSize; } 00247 00256 inline XnUInt32& DataSize() { return m_output.nDataSize; } 00257 00262 inline XnBool IsDataNew() const { return m_output.bIsNew; } 00263 00268 inline XnBool& IsDataNew() { return m_output.bIsNew; } 00269 00273 inline const XnOutputMetaData* GetUnderlying() const { return &m_output; } 00277 inline XnOutputMetaData* GetUnderlying() { return &m_output; } 00278 00283 inline const XnUInt8* Data() const { return *m_ppData; } 00288 inline const XnUInt8*& Data() { return *m_ppData; } 00289 00293 inline XnUInt8* WritableData() 00294 { 00295 MakeDataWritable(); 00296 return m_pAllocatedData; 00297 } 00298 00307 XnStatus AllocateData(XnUInt32 nBytes) 00308 { 00309 if (nBytes > m_nAllocatedSize) 00310 { 00311 // reallocate 00312 XnUInt8* pData = (XnUInt8*)xnOSMallocAligned(nBytes, XN_DEFAULT_MEM_ALIGN); 00313 XN_VALIDATE_ALLOC_PTR(pData); 00314 00315 // allocation succeeded, replace 00316 Free(); 00317 m_pAllocatedData = pData; 00318 m_nAllocatedSize = nBytes; 00319 } 00320 00321 DataSize() = nBytes; 00322 *m_ppData = m_pAllocatedData; 00323 00324 return XN_STATUS_OK; 00325 } 00326 00330 void Free() 00331 { 00332 if (m_nAllocatedSize != 0) 00333 { 00334 xnOSFreeAligned(m_pAllocatedData); 00335 m_pAllocatedData = NULL; 00336 m_nAllocatedSize = 0; 00337 } 00338 } 00339 00344 XnStatus MakeDataWritable() 00345 { 00346 XnStatus nRetVal = XN_STATUS_OK; 00347 00348 // check data isn't already writable 00349 if (Data() != m_pAllocatedData || DataSize() > m_nAllocatedSize) 00350 { 00351 const XnUInt8* pOrigData = *m_ppData; 00352 00353 nRetVal = AllocateData(DataSize()); 00354 XN_IS_STATUS_OK(nRetVal); 00355 00356 if (pOrigData != NULL) 00357 { 00358 xnOSMemCopy(m_pAllocatedData, pOrigData, DataSize()); 00359 } 00360 else 00361 { 00362 xnOSMemSet(m_pAllocatedData, 0, DataSize()); 00363 } 00364 } 00365 00366 return (XN_STATUS_OK); 00367 } 00368 00369 protected: 00370 XnUInt8* m_pAllocatedData; 00371 00372 private: 00373 XnOutputMetaData m_output; 00374 00375 const XnUInt8** m_ppData; 00376 XnUInt32 m_nAllocatedSize; 00377 }; 00378 00391 class MapMetaData : public OutputMetaData 00392 { 00393 public: 00400 inline MapMetaData(XnPixelFormat format, const XnUInt8** ppData) : OutputMetaData(ppData) 00401 { 00402 xnOSMemSet(&m_map, 0, sizeof(XnMapMetaData)); 00403 m_map.pOutput = OutputMetaData::GetUnderlying(); 00404 m_map.PixelFormat = format; 00405 } 00406 00412 inline XnUInt32 XRes() const { return m_map.Res.X; } 00418 inline XnUInt32& XRes() { return m_map.Res.X; } 00419 00425 inline XnUInt32 YRes() const { return m_map.Res.Y; } 00431 inline XnUInt32& YRes() { return m_map.Res.Y; } 00432 00441 inline XnUInt32 XOffset() const { return m_map.Offset.X; } 00450 inline XnUInt32& XOffset() { return m_map.Offset.X; } 00451 00460 inline XnUInt32 YOffset() const { return m_map.Offset.Y; } 00469 inline XnUInt32& YOffset() { return m_map.Offset.Y; } 00470 00475 inline XnUInt32 FullXRes() const { return m_map.FullRes.X; } 00476 00477 00482 inline XnUInt32& FullXRes() { return m_map.FullRes.X; } 00483 00487 inline XnUInt32 FullYRes() const { return m_map.FullRes.Y; } 00491 inline XnUInt32& FullYRes() { return m_map.FullRes.Y; } 00492 00496 inline XnUInt32 FPS() const { return m_map.nFPS; } 00500 inline XnUInt32& FPS() { return m_map.nFPS; } 00501 00505 inline XnPixelFormat PixelFormat() const { return m_map.PixelFormat; } 00506 00510 inline const XnMapMetaData* GetUnderlying() const { return &m_map; } 00514 inline XnMapMetaData* GetUnderlying() { return &m_map; } 00515 00519 inline XnUInt32 BytesPerPixel() const 00520 { 00521 switch (PixelFormat()) 00522 { 00523 case XN_PIXEL_FORMAT_RGB24: 00524 return sizeof(XnRGB24Pixel); 00525 case XN_PIXEL_FORMAT_YUV422: 00526 return sizeof(XnYUV422DoublePixel)/2; 00527 case XN_PIXEL_FORMAT_GRAYSCALE_8_BIT: 00528 return sizeof(XnGrayscale8Pixel); 00529 case XN_PIXEL_FORMAT_GRAYSCALE_16_BIT: 00530 return sizeof(XnGrayscale16Pixel); 00531 case XN_PIXEL_FORMAT_MJPEG: 00532 return 2; 00533 default: 00534 XN_ASSERT(FALSE); 00535 return 0; 00536 } 00537 } 00538 00545 XnStatus AllocateData(XnUInt32 nXRes, XnUInt32 nYRes) 00546 { 00547 XnStatus nRetVal = XN_STATUS_OK; 00548 00549 XnUInt32 nSize = nXRes * nYRes * BytesPerPixel(); 00550 nRetVal = OutputMetaData::AllocateData(nSize); 00551 XN_IS_STATUS_OK(nRetVal); 00552 00553 FullXRes() = XRes() = nXRes; 00554 FullYRes() = YRes() = nYRes; 00555 XOffset() = YOffset() = 0; 00556 00557 return (XN_STATUS_OK); 00558 } 00559 00568 XnStatus ReAdjust(XnUInt32 nXRes, XnUInt32 nYRes, const XnUInt8* pExternalBuffer) 00569 { 00570 XnStatus nRetVal = XN_STATUS_OK; 00571 00572 if (pExternalBuffer == NULL) 00573 { 00574 nRetVal = AllocateData(nXRes, nYRes); 00575 XN_IS_STATUS_OK(nRetVal); 00576 } 00577 else 00578 { 00579 FullXRes() = XRes() = nXRes; 00580 FullYRes() = YRes() = nYRes; 00581 XOffset() = YOffset() = 0; 00582 Data() = pExternalBuffer; 00583 DataSize() = nXRes * nYRes * BytesPerPixel(); 00584 } 00585 00586 return (XN_STATUS_OK); 00587 } 00588 00589 protected: 00590 XnPixelFormat& PixelFormatImpl() { return m_map.PixelFormat; } 00591 00592 private: 00593 // block copy ctor and assignment operator 00594 MapMetaData& operator=(const MapMetaData&); 00595 inline MapMetaData(const MapMetaData& other); 00596 00597 // Members 00598 XnMapMetaData m_map; 00599 }; 00600 00608 template<class _pixelType> 00609 class Map 00610 { 00611 public: 00612 inline Map(_pixelType*& pData, XnUInt32& nXRes, XnUInt32 &nYRes) : 00613 m_pData(pData), m_nXRes(nXRes), m_nYRes(nYRes) 00614 {} 00615 00623 inline XnUInt32 XRes() const { return m_nXRes; } 00631 inline XnUInt32 YRes() const { return m_nYRes; } 00632 00644 inline const _pixelType& operator[](XnUInt32 nIndex) const 00645 { 00646 XN_ASSERT(nIndex < (m_nXRes * m_nYRes)); 00647 return m_pData[nIndex]; 00648 } 00660 inline _pixelType& operator[](XnUInt32 nIndex) 00661 { 00662 XN_ASSERT(nIndex < (m_nXRes *m_nYRes)); 00663 return m_pData[nIndex]; 00664 } 00665 00674 inline const _pixelType& operator()(XnUInt32 x, XnUInt32 y) const 00675 { 00676 XN_ASSERT(x < m_nXRes && y < m_nYRes); 00677 return m_pData[y*m_nXRes + x]; 00678 } 00687 inline _pixelType& operator()(XnUInt32 x, XnUInt32 y) 00688 { 00689 XN_ASSERT(x < m_nXRes && y < m_nYRes); 00690 return m_pData[y*m_nXRes + x]; 00691 } 00692 00693 private: 00694 /* block copy ctor and assignment operator */ 00695 Map(const Map& other); 00696 Map& operator=(const Map&); 00697 00698 _pixelType*& m_pData; 00699 XnUInt32& m_nXRes; 00700 XnUInt32& m_nYRes; 00701 }; 00702 00707 typedef Map<XnDepthPixel> DepthMap; 00709 typedef Map<XnUInt8> ImageMap; 00711 typedef Map<XnRGB24Pixel> RGB24Map; 00713 typedef Map<XnGrayscale16Pixel> Grayscale16Map; 00715 typedef Map<XnGrayscale8Pixel> Grayscale8Map; 00717 typedef Map<XnIRPixel> IRMap; 00719 typedef Map<XnLabel> LabelMap; 00754 class DepthMetaData : public MapMetaData 00755 { 00756 public: 00760 inline DepthMetaData() : 00761 MapMetaData(XN_PIXEL_FORMAT_GRAYSCALE_16_BIT, (const XnUInt8**)&m_depth.pData), 00762 m_depthMap(const_cast<XnDepthPixel*&>(m_depth.pData), MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00763 m_writableDepthMap((XnDepthPixel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y) 00764 { 00765 xnOSMemSet(&m_depth, 0, sizeof(XnDepthMetaData)); 00766 m_depth.pMap = MapMetaData::GetUnderlying(); 00767 } 00768 00776 inline void InitFrom(const DepthMetaData& other) 00777 { 00778 xnCopyDepthMetaData(&m_depth, &other.m_depth); 00779 } 00780 00791 inline XnStatus InitFrom(const DepthMetaData& other, XnUInt32 nXRes, XnUInt32 nYRes, const XnDepthPixel* pExternalBuffer) 00792 { 00793 InitFrom(other); 00794 return ReAdjust(nXRes, nYRes, pExternalBuffer); 00795 } 00796 00803 XnStatus CopyFrom(const DepthMetaData& other) 00804 { 00805 // copy props 00806 InitFrom(other); 00807 // and make a copy of the data (this will allocate and copy data) 00808 return MakeDataWritable(); 00809 } 00810 00814 XnStatus ReAdjust(XnUInt32 nXRes, XnUInt32 nYRes, const XnDepthPixel* pExternalBuffer = NULL) 00815 { 00816 return MapMetaData::ReAdjust(nXRes, nYRes, (const XnUInt8*)pExternalBuffer); 00817 } 00818 00824 inline XnDepthPixel ZRes() const { return m_depth.nZRes; } 00830 inline XnDepthPixel& ZRes() { return m_depth.nZRes; } 00831 00844 inline const XnDepthPixel* Data() const { return (const XnDepthPixel*)MapMetaData::Data(); } 00857 inline const XnDepthPixel*& Data() { return (const XnDepthPixel*&)MapMetaData::Data(); } 00858 00859 00863 inline XnDepthPixel* WritableData() { return (XnDepthPixel*)MapMetaData::WritableData(); } 00864 00868 inline const xn::DepthMap& DepthMap() const { return m_depthMap; } 00872 inline xn::DepthMap& WritableDepthMap() 00873 { 00874 MakeDataWritable(); 00875 return m_writableDepthMap; 00876 } 00877 00883 inline const XnDepthPixel& operator[](XnUInt32 nIndex) const 00884 { 00885 XN_ASSERT(nIndex < (XRes()*YRes())); 00886 return Data()[nIndex]; 00887 } 00888 00895 inline const XnDepthPixel& operator()(XnUInt32 x, XnUInt32 y) const 00896 { 00897 XN_ASSERT(x < XRes() && y < YRes()); 00898 return Data()[y*XRes() + x]; 00899 } 00900 00904 inline const XnDepthMetaData* GetUnderlying() const { return &m_depth; } 00908 inline XnDepthMetaData* GetUnderlying() { return &m_depth; } 00909 00910 private: 00911 // block copy ctor and assignment operator (because we can't return errors in those) 00912 DepthMetaData(const DepthMetaData& other); 00913 DepthMetaData& operator=(const DepthMetaData&); 00914 00915 XnDepthMetaData m_depth; 00916 const xn::DepthMap m_depthMap; 00917 xn::DepthMap m_writableDepthMap; 00918 }; 00919 00946 class ImageMetaData : public MapMetaData 00947 { 00948 public: 00949 inline ImageMetaData() : 00950 MapMetaData(XN_PIXEL_FORMAT_RGB24, &m_image.pData), 00951 m_imageMap(const_cast<XnUInt8*&>(m_image.pData), MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00952 m_writableImageMap((XnUInt8*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00953 m_rgb24Map((XnRGB24Pixel*&)m_image.pData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00954 m_writableRgb24Map((XnRGB24Pixel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00955 m_gray16Map((XnGrayscale16Pixel*&)m_image.pData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00956 m_writableGray16Map((XnGrayscale16Pixel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00957 m_gray8Map((XnGrayscale8Pixel*&)m_image.pData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 00958 m_writableGray8Map((XnGrayscale8Pixel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y) 00959 { 00960 xnOSMemSet(&m_image, 0, sizeof(XnImageMetaData)); 00961 m_image.pMap = MapMetaData::GetUnderlying(); 00962 } 00963 00971 inline void InitFrom(const ImageMetaData& other) 00972 { 00973 xnCopyImageMetaData(&m_image, &other.m_image); 00974 } 00975 00987 inline XnStatus InitFrom(const ImageMetaData& other, XnUInt32 nXRes, XnUInt32 nYRes, XnPixelFormat format, const XnUInt8* pExternalBuffer) 00988 { 00989 InitFrom(other); 00990 XnStatus nRetVal = ReAdjust(nXRes, nYRes, format, pExternalBuffer); 00991 XN_IS_STATUS_OK(nRetVal); 00992 PixelFormat() = format; 00993 return XN_STATUS_OK; 00994 } 00995 01003 inline XnStatus AllocateData(XnUInt32 nXRes, XnUInt32 nYRes, XnPixelFormat format) 01004 { 01005 XnPixelFormat origFormat = PixelFormat(); 01006 PixelFormat() = format; 01007 XnStatus nRetVal = MapMetaData::AllocateData(nXRes, nYRes); 01008 if (nRetVal != XN_STATUS_OK) 01009 { 01010 PixelFormat() = origFormat; 01011 return (nRetVal); 01012 } 01013 01014 return XN_STATUS_OK; 01015 } 01016 01022 inline XnStatus CopyFrom(const ImageMetaData& other) 01023 { 01024 // copy props 01025 xnCopyImageMetaData(&m_image, &other.m_image); 01026 // and make a copy of the data (this will allocate and copy data) 01027 return MakeDataWritable(); 01028 } 01029 01039 XnStatus ReAdjust(XnUInt32 nXRes, XnUInt32 nYRes, XnPixelFormat format, const XnUInt8* pExternalBuffer = NULL) 01040 { 01041 XnPixelFormat origFormat = PixelFormat(); 01042 PixelFormat() = format; 01043 XnStatus nRetVal = MapMetaData::ReAdjust(nXRes, nYRes, pExternalBuffer); 01044 if (nRetVal != XN_STATUS_OK) 01045 { 01046 PixelFormat() = origFormat; 01047 return (nRetVal); 01048 } 01049 01050 return XN_STATUS_OK; 01051 } 01052 01064 inline XnPixelFormat PixelFormat() const { return MapMetaData::PixelFormat(); } 01074 inline XnPixelFormat& PixelFormat() { return MapMetaData::PixelFormatImpl(); } 01075 01079 inline XnUInt8* WritableData() { return MapMetaData::WritableData(); } 01080 01085 inline const XnRGB24Pixel* RGB24Data() const { return (const XnRGB24Pixel*)MapMetaData::Data(); } 01090 inline const XnRGB24Pixel*& RGB24Data() { return (const XnRGB24Pixel*&)MapMetaData::Data(); } 01094 inline XnRGB24Pixel* WritableRGB24Data() { return (XnRGB24Pixel*)MapMetaData::WritableData(); } 01095 01101 inline const XnYUV422DoublePixel* YUV422Data() const { return (const XnYUV422DoublePixel*)MapMetaData::Data(); } 01107 inline const XnYUV422DoublePixel*& YUV422Data() { return (const XnYUV422DoublePixel*&)MapMetaData::Data(); } 01111 inline XnYUV422DoublePixel* WritableYUV422Data() { return (XnYUV422DoublePixel*)MapMetaData::WritableData(); } 01112 01117 inline const XnGrayscale8Pixel* Grayscale8Data() const { return (const XnGrayscale8Pixel*)MapMetaData::Data(); } 01122 inline const XnGrayscale8Pixel*& Grayscale8Data() { return (const XnGrayscale8Pixel*&)MapMetaData::Data(); } 01126 inline XnGrayscale8Pixel* WritableGrayscale8Data() { return (XnGrayscale8Pixel*)MapMetaData::WritableData(); } 01127 01132 inline const XnGrayscale16Pixel* Grayscale16Data() const { return (const XnGrayscale16Pixel*)MapMetaData::Data(); } 01137 inline const XnGrayscale16Pixel*& Grayscale16Data() { return (const XnGrayscale16Pixel*&)MapMetaData::Data(); } 01141 inline XnGrayscale16Pixel* WritableGrayscale16Data() { return (XnGrayscale16Pixel*)MapMetaData::WritableData(); } 01142 01146 inline const xn::ImageMap& ImageMap() const { return m_imageMap; } 01150 inline xn::ImageMap& WritableImageMap() { MakeDataWritable(); return m_writableImageMap; } 01151 01157 inline const xn::RGB24Map& RGB24Map() const { return m_rgb24Map; } 01161 inline xn::RGB24Map& WritableRGB24Map() { MakeDataWritable(); return m_writableRgb24Map; } 01162 01166 inline const xn::Grayscale8Map& Grayscale8Map() const { return m_gray8Map; } 01170 inline xn::Grayscale8Map& WritableGrayscale8Map() { MakeDataWritable(); return m_writableGray8Map; } 01171 01176 inline const xn::Grayscale16Map& Grayscale16Map() const { return m_gray16Map; } 01180 inline xn::Grayscale16Map& WritableGrayscale16Map() { MakeDataWritable(); return m_writableGray16Map; } 01181 01185 inline const XnImageMetaData* GetUnderlying() const { return &m_image; } 01189 inline XnImageMetaData* GetUnderlying() { return &m_image; } 01190 01191 private: 01192 // block copy ctor and assignment operator 01193 ImageMetaData(const ImageMetaData& other); 01194 ImageMetaData& operator=(const ImageMetaData&); 01195 01196 XnImageMetaData m_image; 01197 const xn::ImageMap m_imageMap; 01198 xn::ImageMap m_writableImageMap; 01199 const xn::RGB24Map m_rgb24Map; 01200 xn::RGB24Map m_writableRgb24Map; 01201 const xn::Grayscale16Map m_gray16Map; 01202 xn::Grayscale16Map m_writableGray16Map; 01203 const xn::Grayscale8Map m_gray8Map; 01204 xn::Grayscale8Map m_writableGray8Map; 01205 }; 01206 01216 class IRMetaData : public MapMetaData 01217 { 01218 public: 01219 inline IRMetaData() : 01220 MapMetaData(XN_PIXEL_FORMAT_GRAYSCALE_16_BIT, (const XnUInt8**)&m_ir.pData), 01221 m_irMap(const_cast<XnIRPixel*&>(m_ir.pData), MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 01222 m_writableIRMap((XnIRPixel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y) 01223 { 01224 xnOSMemSet(&m_ir, 0, sizeof(XnIRMetaData)); 01225 m_ir.pMap = MapMetaData::GetUnderlying(); 01226 } 01227 01235 inline void InitFrom(const IRMetaData& other) 01236 { 01237 xnCopyIRMetaData(&m_ir, &other.m_ir); 01238 } 01239 01249 inline XnStatus InitFrom(const IRMetaData& other, XnUInt32 nXRes, XnUInt32 nYRes, const XnIRPixel* pExternalBuffer) 01250 { 01251 InitFrom(other); 01252 return ReAdjust(nXRes, nYRes, pExternalBuffer); 01253 } 01254 01260 XnStatus CopyFrom(const IRMetaData& other) 01261 { 01262 // copy props 01263 xnCopyIRMetaData(&m_ir, &other.m_ir); 01264 // and make a copy of the data (this will allocate and copy data) 01265 return MakeDataWritable(); 01266 } 01267 01271 XnStatus ReAdjust(XnUInt32 nXRes, XnUInt32 nYRes, const XnIRPixel* pExternalBuffer = NULL) 01272 { 01273 return MapMetaData::ReAdjust(nXRes, nYRes, (const XnUInt8*)pExternalBuffer); 01274 } 01275 01288 inline const XnIRPixel* Data() const { return (const XnIRPixel*)MapMetaData::Data(); } 01301 inline const XnIRPixel*& Data() { return (const XnIRPixel*&)MapMetaData::Data(); } 01305 inline XnIRPixel* WritableData() { return (XnIRPixel*)MapMetaData::WritableData(); } 01306 01312 inline const XnIRPixel& operator[](XnUInt32 nIndex) const 01313 { 01314 XN_ASSERT(nIndex < (XRes()*YRes())); 01315 return Data()[nIndex]; 01316 } 01317 01324 inline const XnIRPixel& operator()(XnUInt32 x, XnUInt32 y) const 01325 { 01326 XN_ASSERT(x < XRes() && y < YRes()); 01327 return Data()[y*XRes() + x]; 01328 } 01329 01338 inline const xn::IRMap& IRMap() const { return m_irMap; } 01347 inline xn::IRMap& WritableIRMap() { MakeDataWritable(); return m_writableIRMap; } 01348 01352 inline const XnIRMetaData* GetUnderlying() const { return &m_ir; } 01356 inline XnIRMetaData* GetUnderlying() { return &m_ir; } 01357 01358 private: 01359 // block copy ctor and assignment operator 01360 IRMetaData(const IRMetaData& other); 01361 IRMetaData& operator=(const IRMetaData&); 01362 01363 XnIRMetaData m_ir; 01364 const xn::IRMap m_irMap; 01365 xn::IRMap m_writableIRMap; 01366 }; 01367 01374 class AudioMetaData : public OutputMetaData 01375 { 01376 public: 01377 XN_PRAGMA_START_DISABLED_WARNING_SECTION(XN_UNALIGNED_ADDRESS_WARNING_ID); 01378 inline AudioMetaData() : OutputMetaData(&m_audio.pData) 01379 { 01380 xnOSMemSet(&m_audio, 0, sizeof(XnAudioMetaData)); 01381 m_audio.pOutput = OutputMetaData::GetUnderlying(); 01382 } 01383 01384 XN_PRAGMA_STOP_DISABLED_WARNING_SECTION; 01385 01393 inline void InitFrom(const AudioMetaData& other) 01394 { 01395 xnCopyAudioMetaData(&m_audio, &other.m_audio); 01396 } 01397 01401 inline XnUInt8 NumberOfChannels() const { return m_audio.Wave.nChannels; } 01405 inline XnUInt8& NumberOfChannels() { return m_audio.Wave.nChannels; } 01406 01410 inline XnUInt32 SampleRate() const { return m_audio.Wave.nSampleRate; } 01414 inline XnUInt32& SampleRate() { return m_audio.Wave.nSampleRate; } 01415 01419 inline XnUInt16 BitsPerSample() const { return m_audio.Wave.nBitsPerSample; } 01423 inline XnUInt16& BitsPerSample() { return m_audio.Wave.nBitsPerSample; } 01424 01428 inline const XnAudioMetaData* GetUnderlying() const { return &m_audio; } 01432 inline XnAudioMetaData* GetUnderlying() { return &m_audio; } 01433 01434 private: 01435 // block copy ctor and assignment operator 01436 AudioMetaData(const AudioMetaData& other); 01437 AudioMetaData& operator=(const AudioMetaData&); 01438 01439 XnAudioMetaData m_audio; 01440 }; 01441 01450 class SceneMetaData : public MapMetaData 01451 { 01452 public: 01453 inline SceneMetaData() : 01454 MapMetaData(XN_PIXEL_FORMAT_GRAYSCALE_16_BIT, (const XnUInt8**)&m_scene.pData), 01455 m_labelMap(const_cast<XnLabel*&>(m_scene.pData), MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y), 01456 m_writableLabelMap((XnLabel*&)m_pAllocatedData, MapMetaData::GetUnderlying()->Res.X, MapMetaData::GetUnderlying()->Res.Y) 01457 { 01458 xnOSMemSet(&m_scene, 0, sizeof(XnSceneMetaData)); 01459 m_scene.pMap = MapMetaData::GetUnderlying(); 01460 } 01461 01469 inline void InitFrom(const SceneMetaData& other) 01470 { 01471 xnCopySceneMetaData(&m_scene, &other.m_scene); 01472 } 01473 01483 inline XnStatus InitFrom(const SceneMetaData& other, XnUInt32 nXRes, XnUInt32 nYRes, const XnLabel* pExternalBuffer) 01484 { 01485 InitFrom(other); 01486 return ReAdjust(nXRes, nYRes, pExternalBuffer); 01487 } 01488 01494 XnStatus CopyFrom(const SceneMetaData& other) 01495 { 01496 // copy props 01497 xnCopySceneMetaData(&m_scene, &other.m_scene); 01498 // and make a copy of the data (this will allocate and copy data) 01499 return MakeDataWritable(); 01500 } 01501 01505 XnStatus ReAdjust(XnUInt32 nXRes, XnUInt32 nYRes, const XnLabel* pExternalBuffer = NULL) 01506 { 01507 return MapMetaData::ReAdjust(nXRes, nYRes, (const XnUInt8*)pExternalBuffer); 01508 } 01509 01523 inline const XnLabel* Data() const { return (const XnLabel*)MapMetaData::Data(); } 01537 inline const XnLabel*& Data() { return (const XnLabel*&)MapMetaData::Data(); } 01538 01542 inline XnLabel* WritableData() { return (XnLabel*)MapMetaData::WritableData(); } 01543 01547 inline const xn::LabelMap& LabelMap() const { return m_labelMap; } 01551 inline xn::LabelMap& WritableLabelMap() { MakeDataWritable(); return m_writableLabelMap; } 01552 01563 inline const XnLabel& operator[](XnUInt32 nIndex) const 01564 { 01565 XN_ASSERT(nIndex < (XRes()*YRes())); 01566 return Data()[nIndex]; 01567 } 01568 01580 inline const XnLabel& operator()(XnUInt32 x, XnUInt32 y) const 01581 { 01582 XN_ASSERT(x < XRes() && y < YRes()); 01583 return (*this)[y*XRes() + x]; 01584 } 01585 01589 inline const XnSceneMetaData* GetUnderlying() const { return &m_scene; } 01593 inline XnSceneMetaData* GetUnderlying() { return &m_scene; } 01594 01595 private: 01596 // block copy ctor and assignment operator 01597 SceneMetaData(const SceneMetaData& other); 01598 SceneMetaData& operator=(const SceneMetaData&); 01599 01600 XnSceneMetaData m_scene; 01601 const xn::LabelMap m_labelMap; 01602 xn::LabelMap m_writableLabelMap; 01603 }; 01604 01605 //--------------------------------------------------------------------------- 01606 // NodeWrapper 01607 //--------------------------------------------------------------------------- 01608 01628 class NodeWrapper 01629 { 01630 public: 01631 friend class Context; 01632 01638 inline NodeWrapper(XnNodeHandle hNode) : m_hNode(NULL), m_hShuttingDownCallback(NULL) 01639 { 01640 SetHandle(hNode); 01641 } 01642 01643 inline NodeWrapper(const NodeWrapper& other) : m_hNode(NULL), m_hShuttingDownCallback(NULL) 01644 { 01645 SetHandle(other.GetHandle()); 01646 } 01647 01648 inline NodeWrapper& operator=(const NodeWrapper& other) 01649 { 01650 SetHandle(other.GetHandle()); 01651 return *this; 01652 } 01653 01654 inline ~NodeWrapper() 01655 { 01656 SetHandle(NULL); 01657 } 01658 01659 inline operator XnNodeHandle() const { return GetHandle(); } 01660 01664 inline XnNodeHandle GetHandle() const { return m_hNode; } 01665 01671 inline XnBool operator==(const NodeWrapper& other) 01672 { 01673 return (GetHandle() == other.GetHandle()); 01674 } 01675 01681 inline XnBool operator!=(const NodeWrapper& other) 01682 { 01683 return (GetHandle() != other.GetHandle()); 01684 } 01685 01699 inline XnBool IsValid() const { return (GetHandle() != NULL); } 01700 01701 /* 01702 * @brief Gets the instance name of a node. Unless the application made a specific 01703 * request for a specific name, the name will be of the form: "Depth1", "Image2", etc. 01704 */ 01705 const XnChar* GetName() const {return xnGetNodeName(GetHandle()); } 01706 01711 inline XnStatus AddRef() { return xnProductionNodeAddRef(GetHandle()); } 01712 01717 inline void Release() 01718 { 01719 SetHandle(NULL); 01720 } 01721 01722 01726 inline void SetHandle(XnNodeHandle hNode) 01727 { 01728 if (m_hNode == hNode) 01729 { 01730 // Optimization: do nothing 01731 return; 01732 } 01733 01734 // check currently held node. If we're holding a node, release it 01735 if (m_hNode != NULL) 01736 { 01737 XnContext* pContext = xnGetRefContextFromNodeHandle(m_hNode); 01738 xnContextUnregisterFromShutdown(pContext, m_hShuttingDownCallback); 01739 xnContextRelease(pContext); 01740 xnProductionNodeRelease(m_hNode); 01741 } 01742 01743 // check new node handle, if it points to a node, add ref to it 01744 if (hNode != NULL) 01745 { 01746 XnStatus nRetVal = xnProductionNodeAddRef(hNode); 01747 XN_ASSERT(nRetVal == XN_STATUS_OK); 01748 XN_REFERENCE_VARIABLE(nRetVal); 01749 01750 XnContext* pContext = xnGetRefContextFromNodeHandle(hNode); 01751 01752 nRetVal = xnContextRegisterForShutdown(pContext, ContextShuttingDownCallback, this, &m_hShuttingDownCallback); 01753 XN_ASSERT(nRetVal == XN_STATUS_OK); 01754 01755 xnContextRelease(pContext); 01756 } 01757 01758 m_hNode = hNode; 01759 } 01760 01761 inline void TakeOwnership(XnNodeHandle hNode) 01762 { 01763 SetHandle(hNode); 01764 01765 if (hNode != NULL) 01766 { 01767 xnProductionNodeRelease(hNode); 01768 } 01769 } 01770 01772 inline XnStatus XN_API_DEPRECATED("Please use AddRef() instead.") Ref() { return AddRef(); } 01773 inline void XN_API_DEPRECATED("Please use Release() instead.") Unref() { Release(); } 01776 private: 01777 XnNodeHandle m_hNode; 01778 XnCallbackHandle m_hShuttingDownCallback; 01779 01780 static void XN_CALLBACK_TYPE ContextShuttingDownCallback(XnContext* /*pContext*/, void* pCookie) 01781 { 01782 NodeWrapper* pThis = (NodeWrapper*)pCookie; 01783 pThis->m_hNode = NULL; 01784 } 01785 }; 01786 01787 //--------------------------------------------------------------------------- 01788 // Node Info 01789 //--------------------------------------------------------------------------- 01790 01802 class NodeInfo 01803 { 01804 public: 01810 NodeInfo(XnNodeInfo* pInfo) : m_pNeededNodes(NULL), m_bOwnerOfNode(FALSE) 01811 { 01812 SetUnderlyingObject(pInfo); 01813 } 01814 01820 NodeInfo(const NodeInfo& other) : m_pNeededNodes(NULL), m_bOwnerOfNode(FALSE) 01821 { 01822 SetUnderlyingObject(other.m_pInfo); 01823 } 01824 01828 ~NodeInfo() 01829 { 01830 SetUnderlyingObject(NULL); 01831 } 01832 01838 inline NodeInfo& operator=(const NodeInfo& other) 01839 { 01840 SetUnderlyingObject(other.m_pInfo); 01841 return *this; 01842 } 01843 01847 inline operator XnNodeInfo*() 01848 { 01849 return m_pInfo; 01850 } 01851 01857 inline XnStatus SetInstanceName(const XnChar* strName) 01858 { 01859 return xnNodeInfoSetInstanceName(m_pInfo, strName); 01860 } 01861 01875 inline const XnProductionNodeDescription& GetDescription() const 01876 { 01877 return *xnNodeInfoGetDescription(m_pInfo); 01878 } 01879 01891 inline const XnChar* GetInstanceName() const 01892 { 01893 return xnNodeInfoGetInstanceName(m_pInfo); 01894 } 01895 01907 inline const XnChar* GetCreationInfo() const 01908 { 01909 return xnNodeInfoGetCreationInfo(m_pInfo); 01910 } 01911 01912 /* 01913 * @brief Gets the list of dependant nodes for this node alternative. 01914 * These are any other node alternatives that the node is dependant on. 01915 */ 01916 inline NodeInfoList& GetNeededNodes() const; 01917 01925 inline XnStatus GetInstance(ProductionNode& node) const; 01926 01931 inline const void* GetAdditionalData() const 01932 { 01933 return xnNodeInfoGetAdditionalData(m_pInfo); 01934 } 01935 01940 inline XnStatus GetTreeStringRepresentation(XnChar* csResultBuffer, XnUInt32 nBufferSize) const 01941 { 01942 return xnNodeInfoGetTreeStringRepresentation(m_pInfo, csResultBuffer, nBufferSize); 01943 } 01944 01945 private: 01946 inline void SetUnderlyingObject(XnNodeInfo* pInfo); 01947 01948 XnNodeInfo* m_pInfo; 01949 mutable NodeInfoList* m_pNeededNodes; 01950 XnBool m_bOwnerOfNode; // backwards compatibility 01951 friend class Context; 01952 }; 01953 01954 //--------------------------------------------------------------------------- 01955 // Query 01956 //--------------------------------------------------------------------------- 01957 01976 class Query 01977 { 01978 public: 01979 inline Query() : m_bAllocated(TRUE) 01980 { 01981 xnNodeQueryAllocate(&m_pQuery); 01982 } 01983 01984 inline Query(XnNodeQuery* pNodeQuery) : m_bAllocated(FALSE), m_pQuery(pNodeQuery) 01985 { 01986 } 01987 01988 ~Query() 01989 { 01990 if (m_bAllocated) 01991 { 01992 xnNodeQueryFree(m_pQuery); 01993 } 01994 } 01995 01999 inline const XnNodeQuery* GetUnderlyingObject() const { return m_pQuery; } 02000 inline XnNodeQuery* GetUnderlyingObject() { return m_pQuery; } 02001 02006 inline XnStatus SetVendor(const XnChar* strVendor) 02007 { 02008 return xnNodeQuerySetVendor(m_pQuery, strVendor); 02009 } 02010 02019 inline XnStatus SetName(const XnChar* strName) 02020 { 02021 return xnNodeQuerySetName(m_pQuery, strName); 02022 } 02023 02027 inline XnStatus SetMinVersion(const XnVersion& minVersion) 02028 { 02029 return xnNodeQuerySetMinVersion(m_pQuery, &minVersion); 02030 } 02031 02035 inline XnStatus SetMinVersion(const Version& minVersion) 02036 { 02037 return xnNodeQuerySetMinVersion(m_pQuery, minVersion.GetUnderlying()); 02038 } 02039 02043 inline XnStatus SetMaxVersion(const XnVersion& maxVersion) 02044 { 02045 return xnNodeQuerySetMaxVersion(m_pQuery, &maxVersion); 02046 } 02047 02051 inline XnStatus SetMaxVersion(const Version& maxVersion) 02052 { 02053 return xnNodeQuerySetMaxVersion(m_pQuery, maxVersion.GetUnderlying()); 02054 } 02055 02068 inline XnStatus AddSupportedCapability(const XnChar* strNeededCapability) 02069 { 02070 return xnNodeQueryAddSupportedCapability(m_pQuery, strNeededCapability); 02071 } 02072 02083 inline XnStatus AddSupportedMapOutputMode(const XnMapOutputMode& MapOutputMode) 02084 { 02085 return xnNodeQueryAddSupportedMapOutputMode(m_pQuery, &MapOutputMode); 02086 } 02087 02092 inline XnStatus SetSupportedMinUserPositions(const XnUInt32 nCount) 02093 { 02094 return xnNodeQuerySetSupportedMinUserPositions(m_pQuery, nCount); 02095 } 02096 02108 inline XnStatus SetExistingNodeOnly(XnBool bExistingNode) 02109 { 02110 return xnNodeQuerySetExistingNodeOnly(m_pQuery, bExistingNode); 02111 } 02112 02117 inline XnStatus AddNeededNode(const XnChar* strInstanceName) 02118 { 02119 return xnNodeQueryAddNeededNode(m_pQuery, strInstanceName); 02120 } 02121 02126 inline XnStatus SetCreationInfo(const XnChar* strCreationInfo) 02127 { 02128 return xnNodeQuerySetCreationInfo(m_pQuery, strCreationInfo); 02129 } 02130 02131 private: 02132 XnNodeQuery* m_pQuery; 02133 XnBool m_bAllocated; 02134 }; 02135 02136 //--------------------------------------------------------------------------- 02137 // Node Info List 02138 //--------------------------------------------------------------------------- 02139 02144 class NodeInfoList 02145 { 02146 public: 02150 class Iterator 02151 { 02152 public: 02153 friend class NodeInfoList; 02154 02167 XnBool operator==(const Iterator& other) const 02168 { 02169 return m_it.pCurrent == other.m_it.pCurrent; 02170 } 02171 02178 XnBool operator!=(const Iterator& other) const 02179 { 02180 return m_it.pCurrent != other.m_it.pCurrent; 02181 } 02182 02187 inline Iterator& operator++() 02188 { 02189 UpdateInternalObject(xnNodeInfoListGetNext(m_it)); 02190 return *this; 02191 } 02192 02197 inline Iterator operator++(int) 02198 { 02199 XnNodeInfoListIterator curr = m_it; 02200 UpdateInternalObject(xnNodeInfoListGetNext(m_it)); 02201 return Iterator(curr); 02202 } 02203 02207 inline Iterator& operator--() 02208 { 02209 UpdateInternalObject(xnNodeInfoListGetPrevious(m_it)); 02210 return *this; 02211 } 02212 02216 inline Iterator operator--(int) 02217 { 02218 XnNodeInfoListIterator curr = m_it; 02219 UpdateInternalObject(xnNodeInfoListGetPrevious(m_it)); 02220 return Iterator(curr); 02221 } 02222 02226 inline NodeInfo operator*() 02227 { 02228 return m_Info; 02229 } 02230 02231 private: 02232 inline Iterator(XnNodeInfoListIterator it) : m_Info(NULL) 02233 { 02234 UpdateInternalObject(it); 02235 } 02236 02237 inline void UpdateInternalObject(XnNodeInfoListIterator it) 02238 { 02239 m_it = it; 02240 if (xnNodeInfoListIteratorIsValid(it)) 02241 { 02242 XnNodeInfo* pInfo = xnNodeInfoListGetCurrent(it); 02243 m_Info = NodeInfo(pInfo); 02244 } 02245 else 02246 { 02247 m_Info = NodeInfo(NULL); 02248 } 02249 } 02250 02251 NodeInfo m_Info; 02252 XnNodeInfoListIterator m_it; 02253 }; 02254 02258 inline NodeInfoList() 02259 { 02260 xnNodeInfoListAllocate(&m_pList); 02261 m_bAllocated = TRUE; 02262 } 02263 02270 inline NodeInfoList(XnNodeInfoList* pList) : m_pList(pList), m_bAllocated(FALSE) {} 02271 02272 inline ~NodeInfoList() 02273 { 02274 FreeImpl(); 02275 } 02276 02280 inline XnNodeInfoList* GetUnderlyingObject() const { return m_pList; } 02281 02288 inline void ReplaceUnderlyingObject(XnNodeInfoList* pList) 02289 { 02290 FreeImpl(); 02291 m_pList = pList; 02292 m_bAllocated = TRUE; 02293 } 02294 02299 inline XnStatus Add(XnProductionNodeDescription& description, const XnChar* strCreationInfo, NodeInfoList* pNeededNodes) 02300 { 02301 XnNodeInfoList* pList = (pNeededNodes == NULL) ? NULL : pNeededNodes->GetUnderlyingObject(); 02302 return xnNodeInfoListAdd(m_pList, &description, strCreationInfo, pList); 02303 } 02304 02309 inline XnStatus AddEx(XnProductionNodeDescription& description, const XnChar* strCreationInfo, NodeInfoList* pNeededNodes, const void* pAdditionalData, XnFreeHandler pFreeHandler) 02310 { 02311 XnNodeInfoList* pList = (pNeededNodes == NULL) ? NULL : pNeededNodes->GetUnderlyingObject(); 02312 return xnNodeInfoListAddEx(m_pList, &description, strCreationInfo, pList, pAdditionalData, pFreeHandler); 02313 } 02314 02319 inline XnStatus AddNode(NodeInfo& info) 02320 { 02321 return xnNodeInfoListAddNode(m_pList, info); 02322 } 02323 02328 inline XnStatus AddNodeFromAnotherList(Iterator& it) 02329 { 02330 return xnNodeInfoListAddNodeFromList(m_pList, it.m_it); 02331 } 02332 02337 inline Iterator Begin() const 02338 { 02339 return Iterator(xnNodeInfoListGetFirst(m_pList)); 02340 } 02341 02346 inline Iterator End() const 02347 { 02348 XnNodeInfoListIterator it = { NULL }; 02349 return Iterator(it); 02350 } 02351 02356 inline Iterator RBegin() const 02357 { 02358 return Iterator(xnNodeInfoListGetLast(m_pList)); 02359 } 02360 02365 inline Iterator REnd() const 02366 { 02367 XnNodeInfoListIterator it = { NULL }; 02368 return Iterator(it); 02369 } 02370 02375 inline XnStatus Remove(Iterator& it) 02376 { 02377 return xnNodeInfoListRemove(m_pList, it.m_it); 02378 } 02379 02384 inline XnStatus Clear() 02385 { 02386 return xnNodeInfoListClear(m_pList); 02387 } 02388 02393 inline XnStatus Append(NodeInfoList& other) 02394 { 02395 return xnNodeInfoListAppend(m_pList, other.GetUnderlyingObject()); 02396 } 02397 02401 inline XnBool IsEmpty() 02402 { 02403 return xnNodeInfoListIsEmpty(m_pList); 02404 } 02405 02410 inline XnStatus FilterList(Context& context, Query& query); 02411 02412 private: 02413 inline void FreeImpl() 02414 { 02415 if (m_bAllocated) 02416 { 02417 xnNodeInfoListFree(m_pList); 02418 m_bAllocated = FALSE; 02419 m_pList = NULL; 02420 } 02421 } 02422 02423 XnNodeInfoList* m_pList; 02424 XnBool m_bAllocated; 02425 }; 02426 02427 //--------------------------------------------------------------------------- 02428 // Production Nodes Functionality 02429 //--------------------------------------------------------------------------- 02430 02435 class Capability : public NodeWrapper 02436 { 02437 public: 02443 Capability(XnNodeHandle hNode) : NodeWrapper(hNode) {} 02444 Capability(const NodeWrapper& node) : NodeWrapper(node) {} 02445 }; 02446 02480 class ErrorStateCapability : public Capability 02481 { 02482 public: 02488 ErrorStateCapability(XnNodeHandle hNode) : Capability(hNode) {} 02489 ErrorStateCapability(const NodeWrapper& node) : Capability(node) {} 02490 02494 inline XnStatus GetErrorState() const 02495 { 02496 return xnGetNodeErrorState(GetHandle()); 02497 } 02498 02508 inline XnStatus RegisterToErrorStateChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 02509 { 02510 return _RegisterToStateChange(xnRegisterToNodeErrorStateChange, GetHandle(), handler, pCookie, hCallback); 02511 } 02512 02520 inline void UnregisterFromErrorStateChange(XnCallbackHandle hCallback) 02521 { 02522 _UnregisterFromStateChange(xnUnregisterFromNodeErrorStateChange, GetHandle(), hCallback); 02523 } 02524 }; 02525 02531 class GeneralIntCapability : public Capability 02532 { 02533 public: 02540 GeneralIntCapability(XnNodeHandle hNode, const XnChar* strCap) : Capability(hNode), m_strCap(strCap) {} 02541 GeneralIntCapability(const NodeWrapper& node, const XnChar* strCap) : Capability(node), m_strCap(strCap) {} 02542 02547 inline void GetRange(XnInt32& nMin, XnInt32& nMax, XnInt32& nStep, XnInt32& nDefault, XnBool& bIsAutoSupported) const 02548 { 02549 xnGetGeneralIntRange(GetHandle(), m_strCap, &nMin, &nMax, &nStep, &nDefault, &bIsAutoSupported); 02550 } 02551 02556 inline XnInt32 Get() 02557 { 02558 XnInt32 nValue; 02559 xnGetGeneralIntValue(GetHandle(), m_strCap, &nValue); 02560 return nValue; 02561 } 02562 02567 inline XnStatus Set(XnInt32 nValue) 02568 { 02569 return xnSetGeneralIntValue(GetHandle(), m_strCap, nValue); 02570 } 02571 02581 XnStatus RegisterToValueChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback); 02582 02590 void UnregisterFromValueChange(XnCallbackHandle hCallback); 02591 02592 private: 02593 const XnChar* m_strCap; 02594 }; 02595 02631 class ProductionNode : public NodeWrapper 02632 { 02633 public: 02639 inline ProductionNode(XnNodeHandle hNode = NULL) : NodeWrapper(hNode) {} 02640 inline ProductionNode(const NodeWrapper& other) : NodeWrapper(other) {} 02641 02650 inline NodeInfo GetInfo() const { return NodeInfo(xnGetNodeInfo(GetHandle())); } 02651 02656 inline XnStatus AddNeededNode(ProductionNode& needed) 02657 { 02658 return xnAddNeededNode(GetHandle(), needed.GetHandle()); 02659 } 02660 02665 inline XnStatus RemoveNeededNode(ProductionNode& needed) 02666 { 02667 return xnRemoveNeededNode(GetHandle(), needed.GetHandle()); 02668 } 02669 02681 inline void GetContext(Context& context) const; 02682 02692 inline Context GetContext() const; 02693 02705 inline XnBool IsCapabilitySupported(const XnChar* strCapabilityName) const 02706 { 02707 return xnIsCapabilitySupported(GetHandle(), strCapabilityName); 02708 } 02709 02714 inline XnStatus SetIntProperty(const XnChar* strName, XnUInt64 nValue) 02715 { 02716 return xnSetIntProperty(GetHandle(), strName, nValue); 02717 } 02718 02723 inline XnStatus SetRealProperty(const XnChar* strName, XnDouble dValue) 02724 { 02725 return xnSetRealProperty(GetHandle(), strName, dValue); 02726 } 02727 02732 inline XnStatus SetStringProperty(const XnChar* strName, const XnChar* strValue) 02733 { 02734 return xnSetStringProperty(GetHandle(), strName, strValue); 02735 } 02736 02741 inline XnStatus SetGeneralProperty(const XnChar* strName, XnUInt32 nBufferSize, const void* pBuffer) 02742 { 02743 return xnSetGeneralProperty(GetHandle(), strName, nBufferSize, pBuffer); 02744 } 02745 02750 inline XnStatus GetIntProperty(const XnChar* strName, XnUInt64& nValue) const 02751 { 02752 return xnGetIntProperty(GetHandle(), strName, &nValue); 02753 } 02754 02759 inline XnStatus GetRealProperty(const XnChar* strName, XnDouble &dValue) const 02760 { 02761 return xnGetRealProperty(GetHandle(), strName, &dValue); 02762 } 02763 02768 inline XnStatus GetStringProperty(const XnChar* strName, XnChar* csValue, XnUInt32 nBufSize) const 02769 { 02770 return xnGetStringProperty(GetHandle(), strName, csValue, nBufSize); 02771 } 02772 02777 inline XnStatus GetGeneralProperty(const XnChar* strName, XnUInt32 nBufferSize, void* pBuffer) const 02778 { 02779 return xnGetGeneralProperty(GetHandle(), strName, nBufferSize, pBuffer); 02780 } 02781 02786 inline XnStatus LockForChanges(XnLockHandle* phLock) 02787 { 02788 return xnLockNodeForChanges(GetHandle(), phLock); 02789 } 02790 02795 inline void UnlockForChanges(XnLockHandle hLock) 02796 { 02797 xnUnlockNodeForChanges(GetHandle(), hLock); 02798 } 02799 02804 inline XnStatus LockedNodeStartChanges(XnLockHandle hLock) 02805 { 02806 return xnLockedNodeStartChanges(GetHandle(), hLock); 02807 } 02808 02813 inline void LockedNodeEndChanges(XnLockHandle hLock) 02814 { 02815 xnLockedNodeEndChanges(GetHandle(), hLock); 02816 } 02817 02826 inline const ErrorStateCapability GetErrorStateCap() const 02827 { 02828 return ErrorStateCapability(GetHandle()); 02829 } 02830 02839 inline ErrorStateCapability GetErrorStateCap() 02840 { 02841 return ErrorStateCapability(GetHandle()); 02842 } 02843 02855 inline GeneralIntCapability GetGeneralIntCap(const XnChar* strCapability) 02856 { 02857 return GeneralIntCapability(GetHandle(), strCapability); 02858 } 02859 }; 02860 02870 class DeviceIdentificationCapability : public Capability 02871 { 02872 public: 02878 DeviceIdentificationCapability(XnNodeHandle hNode) : Capability(hNode) {} 02879 DeviceIdentificationCapability(const NodeWrapper& node) : Capability(node) {} 02880 02890 inline XnStatus GetDeviceName(XnChar* strBuffer, XnUInt32 nBufferSize) 02891 { 02892 return xnGetDeviceName(GetHandle(), strBuffer, &nBufferSize); 02893 } 02894 02904 inline XnStatus GetVendorSpecificData(XnChar* strBuffer, XnUInt32 nBufferSize) 02905 { 02906 return xnGetVendorSpecificData(GetHandle(), strBuffer, &nBufferSize); 02907 } 02908 02918 inline XnStatus GetSerialNumber(XnChar* strBuffer, XnUInt32 nBufferSize) 02919 { 02920 return xnGetSerialNumber(GetHandle(), strBuffer, &nBufferSize); 02921 } 02922 }; 02923 02937 class Device : public ProductionNode 02938 { 02939 public: 02940 inline Device(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 02941 inline Device(const NodeWrapper& other) : ProductionNode(other) {} 02942 02951 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 02952 02966 inline DeviceIdentificationCapability GetIdentificationCap() 02967 { 02968 return DeviceIdentificationCapability(GetHandle()); 02969 } 02970 }; 02971 03019 class MirrorCapability : public Capability 03020 { 03021 public: 03022 inline MirrorCapability(XnNodeHandle hNode) : Capability(hNode) {} 03023 MirrorCapability(const NodeWrapper& node) : Capability(node) {} 03024 03031 inline XnStatus SetMirror(XnBool bMirror) 03032 { 03033 return xnSetMirror(GetHandle(), bMirror); 03034 } 03035 03039 inline XnBool IsMirrored() const 03040 { 03041 return xnIsMirrored(GetHandle()); 03042 } 03043 03053 inline XnStatus RegisterToMirrorChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 03054 { 03055 return _RegisterToStateChange(xnRegisterToMirrorChange, GetHandle(), handler, pCookie, hCallback); 03056 } 03057 03065 inline void UnregisterFromMirrorChange(XnCallbackHandle hCallback) 03066 { 03067 _UnregisterFromStateChange(xnUnregisterFromMirrorChange, GetHandle(), hCallback); 03068 } 03069 }; 03070 03103 class AlternativeViewPointCapability : public Capability 03104 { 03105 public: 03106 inline AlternativeViewPointCapability(XnNodeHandle hNode) : Capability(hNode) {} 03107 AlternativeViewPointCapability(const NodeWrapper& node) : Capability(node) {} 03108 03123 inline XnBool IsViewPointSupported(ProductionNode& otherNode) const 03124 { 03125 return xnIsViewPointSupported(GetHandle(), otherNode.GetHandle()); 03126 } 03127 03135 inline XnStatus SetViewPoint(ProductionNode& otherNode) 03136 { 03137 return xnSetViewPoint(GetHandle(), otherNode.GetHandle()); 03138 } 03139 03143 inline XnStatus ResetViewPoint() 03144 { 03145 return xnResetViewPoint(GetHandle()); 03146 } 03147 03155 inline XnBool IsViewPointAs(ProductionNode& otherNode) const 03156 { 03157 return xnIsViewPointAs(GetHandle(), otherNode.GetHandle()); 03158 } 03159 03169 inline XnStatus RegisterToViewPointChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 03170 { 03171 return _RegisterToStateChange(xnRegisterToViewPointChange, GetHandle(), handler, pCookie, hCallback); 03172 } 03173 03181 inline void UnregisterFromViewPointChange(XnCallbackHandle hCallback) 03182 { 03183 _UnregisterFromStateChange(xnUnregisterFromViewPointChange, GetHandle(), hCallback); 03184 } 03185 }; 03186 03208 class FrameSyncCapability : public Capability 03209 { 03210 public: 03211 inline FrameSyncCapability(XnNodeHandle hNode) : Capability(hNode) {} 03212 FrameSyncCapability(const NodeWrapper& node) : Capability(node) {} 03213 03222 inline XnBool CanFrameSyncWith(Generator& other) const; 03223 03230 inline XnStatus FrameSyncWith(Generator& other); 03231 03236 inline XnStatus StopFrameSyncWith(Generator& other); 03237 03244 inline XnBool IsFrameSyncedWith(Generator& other) const; 03245 03255 inline XnStatus RegisterToFrameSyncChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 03256 { 03257 return _RegisterToStateChange(xnRegisterToFrameSyncChange, GetHandle(), handler, pCookie, hCallback); 03258 } 03259 03267 inline void UnregisterFromFrameSyncChange(XnCallbackHandle hCallback) 03268 { 03269 _UnregisterFromStateChange(xnUnregisterFromFrameSyncChange, GetHandle(), hCallback); 03270 } 03271 }; 03272 03325 class Generator : public ProductionNode 03326 { 03327 public: 03333 inline Generator(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 03334 inline Generator(const NodeWrapper& other) : ProductionNode(other) {} 03335 03355 inline XnStatus StartGenerating() 03356 { 03357 return xnStartGenerating(GetHandle()); 03358 } 03359 03363 inline XnBool IsGenerating() const 03364 { 03365 return xnIsGenerating(GetHandle()); 03366 } 03367 03376 inline XnStatus StopGenerating() 03377 { 03378 return xnStopGenerating(GetHandle()); 03379 } 03380 03394 inline XnStatus RegisterToGenerationRunningChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle &hCallback) 03395 { 03396 return _RegisterToStateChange(xnRegisterToGenerationRunningChange, GetHandle(), handler, pCookie, hCallback); 03397 } 03398 03406 inline void UnregisterFromGenerationRunningChange(XnCallbackHandle hCallback) 03407 { 03408 _UnregisterFromStateChange(xnUnregisterFromGenerationRunningChange, GetHandle(), hCallback); 03409 } 03410 03424 inline XnStatus RegisterToNewDataAvailable(StateChangedHandler handler, void* pCookie, XnCallbackHandle &hCallback) 03425 { 03426 return _RegisterToStateChange(xnRegisterToNewDataAvailable, GetHandle(), handler, pCookie, hCallback); 03427 } 03428 03436 inline void UnregisterFromNewDataAvailable(XnCallbackHandle hCallback) 03437 { 03438 _UnregisterFromStateChange(xnUnregisterFromNewDataAvailable, GetHandle(), hCallback); 03439 } 03440 03449 inline XnBool IsNewDataAvailable(XnUInt64* pnTimestamp = NULL) const 03450 { 03451 return xnIsNewDataAvailable(GetHandle(), pnTimestamp); 03452 } 03453 03472 inline XnStatus WaitAndUpdateData() 03473 { 03474 return xnWaitAndUpdateData(GetHandle()); 03475 } 03476 03481 inline XnBool IsDataNew() const 03482 { 03483 return xnIsDataNew(GetHandle()); 03484 } 03485 03503 inline const void* GetData() 03504 { 03505 return xnGetData(GetHandle()); 03506 } 03507 03521 inline XnUInt32 GetDataSize() const 03522 { 03523 return xnGetDataSize(GetHandle()); 03524 } 03525 03539 inline XnUInt64 GetTimestamp() const 03540 { 03541 return xnGetTimestamp(GetHandle()); 03542 } 03543 03556 inline XnUInt32 GetFrameID() const 03557 { 03558 return xnGetFrameID(GetHandle()); 03559 } 03560 03569 inline const MirrorCapability GetMirrorCap() const 03570 { 03571 return MirrorCapability(GetHandle()); 03572 } 03573 03582 inline MirrorCapability GetMirrorCap() 03583 { 03584 return MirrorCapability(GetHandle()); 03585 } 03586 03597 inline const AlternativeViewPointCapability GetAlternativeViewPointCap() const 03598 { 03599 return AlternativeViewPointCapability(GetHandle()); 03600 } 03601 03612 inline AlternativeViewPointCapability GetAlternativeViewPointCap() 03613 { 03614 return AlternativeViewPointCapability(GetHandle()); 03615 } 03616 03626 inline const FrameSyncCapability GetFrameSyncCap() const 03627 { 03628 return FrameSyncCapability(GetHandle()); 03629 } 03630 03640 inline FrameSyncCapability GetFrameSyncCap() 03641 { 03642 return FrameSyncCapability(GetHandle()); 03643 } 03644 }; 03645 03690 class Recorder : public ProductionNode 03691 { 03692 public: 03698 inline Recorder(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 03699 inline Recorder(const NodeWrapper& other) : ProductionNode(other) {} 03700 03708 inline XnStatus Create(Context& context, const XnChar* strFormatName = NULL); 03709 03718 inline XnStatus SetDestination(XnRecordMedium destType, const XnChar* strDest) 03719 { 03720 return xnSetRecorderDestination(GetHandle(), destType, strDest); 03721 } 03722 03732 inline XnStatus GetDestination(XnRecordMedium& destType, XnChar* strDest, XnUInt32 nBufSize) 03733 { 03734 return xnGetRecorderDestination(GetHandle(), &destType, strDest, nBufSize); 03735 } 03736 03746 inline XnStatus AddNodeToRecording(ProductionNode& Node, XnCodecID compression = XN_CODEC_NULL) 03747 { 03748 return xnAddNodeToRecording(GetHandle(), Node.GetHandle(), compression); 03749 } 03750 03757 inline XnStatus RemoveNodeFromRecording(ProductionNode& Node) 03758 { 03759 return xnRemoveNodeFromRecording(GetHandle(), Node.GetHandle()); 03760 } 03761 03774 inline XnStatus Record() 03775 { 03776 return xnRecord(GetHandle()); 03777 } 03778 }; 03779 03807 class Player : public ProductionNode 03808 { 03809 public: 03815 inline Player(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 03816 inline Player(const NodeWrapper& other) : ProductionNode(other) {} 03817 03826 inline XnStatus Create(Context& context, const XnChar* strFormatName); 03827 03828 03843 inline XnStatus SetRepeat(XnBool bRepeat) 03844 { 03845 return xnSetPlayerRepeat(GetHandle(), bRepeat); 03846 } 03847 03852 inline XnStatus SetSource(XnRecordMedium sourceType, const XnChar* strSource) 03853 { 03854 return xnSetPlayerSource(GetHandle(), sourceType, strSource); 03855 } 03856 03865 inline XnStatus GetSource(XnRecordMedium &sourceType, XnChar* strSource, XnUInt32 nBufSize) const 03866 { 03867 return xnGetPlayerSource(GetHandle(), &sourceType, strSource, nBufSize); 03868 } 03869 03874 inline XnStatus ReadNext() 03875 { 03876 return xnPlayerReadNext(GetHandle()); 03877 } 03878 03903 inline XnStatus SeekToTimeStamp(XnInt64 nTimeOffset, XnPlayerSeekOrigin origin) 03904 { 03905 return xnSeekPlayerToTimeStamp(GetHandle(), nTimeOffset, origin); 03906 } 03907 03932 inline XnStatus SeekToFrame(const XnChar* strNodeName, XnInt32 nFrameOffset, XnPlayerSeekOrigin origin) 03933 { 03934 return xnSeekPlayerToFrame(GetHandle(), strNodeName, nFrameOffset, origin); 03935 } 03936 03942 inline XnStatus TellTimestamp(XnUInt64& nTimestamp) const 03943 { 03944 return xnTellPlayerTimestamp(GetHandle(), &nTimestamp); 03945 } 03946 03959 inline XnStatus TellFrame(const XnChar* strNodeName, XnUInt32& nFrame) const 03960 { 03961 return xnTellPlayerFrame(GetHandle(), strNodeName, &nFrame); 03962 } 03963 03971 inline XnStatus GetNumFrames(const XnChar* strNodeName, XnUInt32& nFrames) const 03972 { 03973 return xnGetPlayerNumFrames(GetHandle(), strNodeName, &nFrames); 03974 } 03975 03980 inline const XnChar* GetSupportedFormat() const 03981 { 03982 return xnGetPlayerSupportedFormat(GetHandle()); 03983 } 03984 03989 inline XnStatus EnumerateNodes(NodeInfoList& list) const 03990 { 03991 XnNodeInfoList* pList; 03992 XnStatus nRetVal = xnEnumeratePlayerNodes(GetHandle(), &pList); 03993 XN_IS_STATUS_OK(nRetVal); 03994 03995 list.ReplaceUnderlyingObject(pList); 03996 03997 return (XN_STATUS_OK); 03998 } 03999 04008 inline XnBool IsEOF() const 04009 { 04010 return xnIsPlayerAtEOF(GetHandle()); 04011 } 04012 04022 inline XnStatus RegisterToEndOfFileReached(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04023 { 04024 return _RegisterToStateChange(xnRegisterToEndOfFileReached, GetHandle(), handler, pCookie, hCallback); 04025 } 04026 04034 inline void UnregisterFromEndOfFileReached(XnCallbackHandle hCallback) 04035 { 04036 _UnregisterFromStateChange(xnUnregisterFromEndOfFileReached, GetHandle(), hCallback); 04037 } 04038 04057 inline XnStatus SetPlaybackSpeed(XnDouble dSpeed) 04058 { 04059 return xnSetPlaybackSpeed(GetHandle(), dSpeed); 04060 } 04061 04066 inline XnDouble GetPlaybackSpeed() const 04067 { 04068 return xnGetPlaybackSpeed(GetHandle()); 04069 } 04070 }; 04071 04099 class CroppingCapability : public Capability 04100 { 04101 public: 04107 inline CroppingCapability(XnNodeHandle hNode) : Capability(hNode) {} 04108 CroppingCapability(const NodeWrapper& node) : Capability(node) {} 04109 04119 inline XnStatus SetCropping(const XnCropping& Cropping) 04120 { 04121 return xnSetCropping(GetHandle(), &Cropping); 04122 } 04123 04134 inline XnStatus GetCropping(XnCropping& Cropping) const 04135 { 04136 return xnGetCropping(GetHandle(), &Cropping); 04137 } 04138 04148 inline XnStatus RegisterToCroppingChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04149 { 04150 return _RegisterToStateChange(xnRegisterToCroppingChange, GetHandle(), handler, pCookie, hCallback); 04151 } 04152 04160 inline void UnregisterFromCroppingChange(XnCallbackHandle hCallback) 04161 { 04162 _UnregisterFromStateChange(xnUnregisterFromCroppingChange, GetHandle(), hCallback); 04163 } 04164 }; 04165 04174 class AntiFlickerCapability : public Capability 04175 { 04176 public: 04182 inline AntiFlickerCapability(XnNodeHandle hNode) : Capability(hNode) {} 04183 AntiFlickerCapability(const NodeWrapper& node) : Capability(node) {} 04184 04189 inline XnStatus SetPowerLineFrequency(XnPowerLineFrequency nFrequency) 04190 { 04191 return xnSetPowerLineFrequency(GetHandle(), nFrequency); 04192 } 04193 04198 inline XnPowerLineFrequency GetPowerLineFrequency() 04199 { 04200 return xnGetPowerLineFrequency(GetHandle()); 04201 } 04202 04212 inline XnStatus RegisterToPowerLineFrequencyChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04213 { 04214 return _RegisterToStateChange(xnRegisterToPowerLineFrequencyChange, GetHandle(), handler, pCookie, hCallback); 04215 } 04216 04224 inline void UnregisterFromPowerLineFrequencyChange(XnCallbackHandle hCallback) 04225 { 04226 _UnregisterFromStateChange(xnUnregisterFromPowerLineFrequencyChange, GetHandle(), hCallback); 04227 } 04228 }; 04229 04253 class MapGenerator : public Generator 04254 { 04255 public: 04261 inline MapGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 04262 inline MapGenerator(const NodeWrapper& other) : Generator(other) {} 04263 04271 inline XnUInt32 GetSupportedMapOutputModesCount() const 04272 { 04273 return xnGetSupportedMapOutputModesCount(GetHandle()); 04274 } 04275 04286 inline XnStatus GetSupportedMapOutputModes(XnMapOutputMode* aModes, XnUInt32& nCount) const 04287 { 04288 return xnGetSupportedMapOutputModes(GetHandle(), aModes, &nCount); 04289 } 04290 04304 inline XnStatus SetMapOutputMode(const XnMapOutputMode& OutputMode) 04305 { 04306 return xnSetMapOutputMode(GetHandle(), &OutputMode); 04307 } 04308 04326 inline XnStatus GetMapOutputMode(XnMapOutputMode &OutputMode) const 04327 { 04328 return xnGetMapOutputMode(GetHandle(), &OutputMode); 04329 } 04330 04339 inline XnUInt32 GetBytesPerPixel() const 04340 { 04341 return xnGetBytesPerPixel(GetHandle()); 04342 } 04343 04353 inline XnStatus RegisterToMapOutputModeChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04354 { 04355 return _RegisterToStateChange(xnRegisterToMapOutputModeChange, GetHandle(), handler, pCookie, hCallback); 04356 } 04357 04365 inline void UnregisterFromMapOutputModeChange(XnCallbackHandle hCallback) 04366 { 04367 _UnregisterFromStateChange(xnUnregisterFromMapOutputModeChange, GetHandle(), hCallback); 04368 } 04369 04378 inline const CroppingCapability GetCroppingCap() const 04379 { 04380 return CroppingCapability(GetHandle()); 04381 } 04382 04391 inline CroppingCapability GetCroppingCap() 04392 { 04393 return CroppingCapability(GetHandle()); 04394 } 04395 04401 inline GeneralIntCapability GetBrightnessCap() 04402 { 04403 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_BRIGHTNESS); 04404 } 04405 04411 inline GeneralIntCapability GetContrastCap() 04412 { 04413 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_CONTRAST); 04414 } 04415 04421 inline GeneralIntCapability GetHueCap() 04422 { 04423 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_HUE); 04424 } 04425 04431 inline GeneralIntCapability GetSaturationCap() 04432 { 04433 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_SATURATION); 04434 } 04435 04441 inline GeneralIntCapability GetSharpnessCap() 04442 { 04443 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_SHARPNESS); 04444 } 04445 04451 inline GeneralIntCapability GetGammaCap() 04452 { 04453 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_GAMMA); 04454 } 04455 04461 inline GeneralIntCapability GetWhiteBalanceCap() 04462 { 04463 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_COLOR_TEMPERATURE); 04464 } 04465 04471 inline GeneralIntCapability GetBacklightCompensationCap() 04472 { 04473 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_BACKLIGHT_COMPENSATION); 04474 } 04475 04481 inline GeneralIntCapability GetGainCap() 04482 { 04483 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_GAIN); 04484 } 04485 04491 inline GeneralIntCapability GetPanCap() 04492 { 04493 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_PAN); 04494 } 04495 04501 inline GeneralIntCapability GetTiltCap() 04502 { 04503 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_TILT); 04504 } 04505 04511 inline GeneralIntCapability GetRollCap() 04512 { 04513 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_ROLL); 04514 } 04515 04521 inline GeneralIntCapability GetZoomCap() 04522 { 04523 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_ZOOM); 04524 } 04525 04531 inline GeneralIntCapability GetExposureCap() 04532 { 04533 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_EXPOSURE); 04534 } 04535 04541 inline GeneralIntCapability GetIrisCap() 04542 { 04543 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_IRIS); 04544 } 04545 04551 inline GeneralIntCapability GetFocusCap() 04552 { 04553 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_FOCUS); 04554 } 04555 04561 inline GeneralIntCapability GetLowLightCompensationCap() 04562 { 04563 return GeneralIntCapability(GetHandle(), XN_CAPABILITY_LOW_LIGHT_COMPENSATION); 04564 } 04565 04571 inline AntiFlickerCapability GetAntiFlickerCap() 04572 { 04573 return AntiFlickerCapability(GetHandle()); 04574 } 04575 }; 04576 04585 class UserPositionCapability : public Capability 04586 { 04587 public: 04593 inline UserPositionCapability(XnNodeHandle hNode = NULL) : Capability(hNode) {} 04594 UserPositionCapability(const NodeWrapper& node) : Capability(node) {} 04595 04600 inline XnUInt32 GetSupportedUserPositionsCount() const 04601 { 04602 return xnGetSupportedUserPositionsCount(GetHandle()); 04603 } 04604 04609 inline XnStatus SetUserPosition(XnUInt32 nIndex, const XnBoundingBox3D& Position) 04610 { 04611 return xnSetUserPosition(GetHandle(), nIndex, &Position); 04612 } 04613 04618 inline XnStatus GetUserPosition(XnUInt32 nIndex, XnBoundingBox3D& Position) const 04619 { 04620 return xnGetUserPosition(GetHandle(), nIndex, &Position); 04621 } 04622 04632 inline XnStatus RegisterToUserPositionChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04633 { 04634 return _RegisterToStateChange(xnRegisterToUserPositionChange, GetHandle(), handler, pCookie, hCallback); 04635 } 04636 04644 inline void UnregisterFromUserPositionChange(XnCallbackHandle hCallback) 04645 { 04646 _UnregisterFromStateChange(xnUnregisterFromUserPositionChange, GetHandle(), hCallback); 04647 } 04648 }; 04649 04694 class DepthGenerator : public MapGenerator 04695 { 04696 public: 04702 inline DepthGenerator(XnNodeHandle hNode = NULL) : MapGenerator(hNode) {} 04703 inline DepthGenerator(const NodeWrapper& other) : MapGenerator(other) {} 04704 04712 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 04713 04730 inline void GetMetaData(DepthMetaData& metaData) const 04731 { 04732 xnGetDepthMetaData(GetHandle(), metaData.GetUnderlying()); 04733 } 04734 04739 inline const XnDepthPixel* GetDepthMap() const 04740 { 04741 return xnGetDepthMap(GetHandle()); 04742 } 04743 04753 inline XnDepthPixel GetDeviceMaxDepth() const 04754 { 04755 return xnGetDeviceMaxDepth(GetHandle()); 04756 } 04757 04776 inline XnStatus GetFieldOfView(XnFieldOfView& FOV) const 04777 { 04778 return xnGetDepthFieldOfView(GetHandle(), &FOV); 04779 } 04780 04790 inline XnStatus RegisterToFieldOfViewChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 04791 { 04792 return _RegisterToStateChange(xnRegisterToDepthFieldOfViewChange, GetHandle(), handler, pCookie, hCallback); 04793 } 04794 04802 inline void UnregisterFromFieldOfViewChange(XnCallbackHandle hCallback) 04803 { 04804 _UnregisterFromStateChange(xnUnregisterFromDepthFieldOfViewChange, GetHandle(), hCallback); 04805 } 04806 04816 inline XnStatus ConvertProjectiveToRealWorld(XnUInt32 nCount, const XnPoint3D aProjective[], XnPoint3D aRealWorld[]) const 04817 { 04818 return xnConvertProjectiveToRealWorld(GetHandle(), nCount, aProjective, aRealWorld); 04819 } 04820 04830 inline XnStatus ConvertRealWorldToProjective(XnUInt32 nCount, const XnPoint3D aRealWorld[], XnPoint3D aProjective[]) const 04831 { 04832 return xnConvertRealWorldToProjective(GetHandle(), nCount, aRealWorld, aProjective); 04833 } 04834 04840 inline const UserPositionCapability GetUserPositionCap() const 04841 { 04842 return UserPositionCapability(GetHandle()); 04843 } 04844 04850 inline UserPositionCapability GetUserPositionCap() 04851 { 04852 return UserPositionCapability(GetHandle()); 04853 } 04854 }; 04855 04860 class MockDepthGenerator : public DepthGenerator 04861 { 04862 public: 04868 inline MockDepthGenerator(XnNodeHandle hNode = NULL) : DepthGenerator(hNode) {} 04869 inline MockDepthGenerator(const NodeWrapper& other) : DepthGenerator(other) {} 04870 04877 XnStatus Create(Context& context, const XnChar* strName = NULL); 04878 04886 XnStatus CreateBasedOn(DepthGenerator& other, const XnChar* strName = NULL); 04887 04892 inline XnStatus SetData(XnUInt32 nFrameID, XnUInt64 nTimestamp, XnUInt32 nDataSize, const XnDepthPixel* pDepthMap) 04893 { 04894 return xnMockDepthSetData(GetHandle(), nFrameID, nTimestamp, nDataSize, pDepthMap); 04895 } 04896 04905 inline XnStatus SetData(const DepthMetaData& depthMD, XnUInt32 nFrameID, XnUInt64 nTimestamp) 04906 { 04907 return SetData(nFrameID, nTimestamp, depthMD.DataSize(), depthMD.Data()); 04908 } 04909 04915 inline XnStatus SetData(const DepthMetaData& depthMD) 04916 { 04917 return SetData(depthMD, depthMD.FrameID(), depthMD.Timestamp()); 04918 } 04919 }; 04920 04939 class ImageGenerator : public MapGenerator 04940 { 04941 public: 04947 inline ImageGenerator(XnNodeHandle hNode = NULL) : MapGenerator(hNode) {} 04948 inline ImageGenerator(const NodeWrapper& other) : MapGenerator(other) {} 04949 04955 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 04956 04973 inline void GetMetaData(ImageMetaData& metaData) const 04974 { 04975 xnGetImageMetaData(GetHandle(), metaData.GetUnderlying()); 04976 } 04977 04982 inline const XnRGB24Pixel* GetRGB24ImageMap() const 04983 { 04984 return xnGetRGB24ImageMap(GetHandle()); 04985 } 04986 04991 inline const XnYUV422DoublePixel* GetYUV422ImageMap() const 04992 { 04993 return xnGetYUV422ImageMap(GetHandle()); 04994 } 04995 05000 inline const XnGrayscale8Pixel* GetGrayscale8ImageMap() const 05001 { 05002 return xnGetGrayscale8ImageMap(GetHandle()); 05003 } 05004 05009 inline const XnGrayscale16Pixel* GetGrayscale16ImageMap() const 05010 { 05011 return xnGetGrayscale16ImageMap(GetHandle()); 05012 } 05013 05018 inline const XnUInt8* GetImageMap() const 05019 { 05020 return xnGetImageMap(GetHandle()); 05021 } 05022 05033 inline XnBool IsPixelFormatSupported(XnPixelFormat Format) const 05034 { 05035 return xnIsPixelFormatSupported(GetHandle(), Format); 05036 } 05037 05055 inline XnStatus SetPixelFormat(XnPixelFormat Format) 05056 { 05057 return xnSetPixelFormat(GetHandle(), Format); 05058 } 05059 05070 inline XnPixelFormat GetPixelFormat() const 05071 { 05072 return xnGetPixelFormat(GetHandle()); 05073 } 05074 05084 inline XnStatus RegisterToPixelFormatChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 05085 { 05086 return _RegisterToStateChange(xnRegisterToPixelFormatChange, GetHandle(), handler, pCookie, hCallback); 05087 } 05088 05096 inline void UnregisterFromPixelFormatChange(XnCallbackHandle hCallback) 05097 { 05098 _UnregisterFromStateChange(xnUnregisterFromPixelFormatChange, GetHandle(), hCallback); 05099 } 05100 }; 05101 05106 class MockImageGenerator : public ImageGenerator 05107 { 05108 public: 05114 inline MockImageGenerator(XnNodeHandle hNode = NULL) : ImageGenerator(hNode) {} 05115 inline MockImageGenerator(const NodeWrapper& other) : ImageGenerator(other) {} 05116 05123 XnStatus Create(Context& context, const XnChar* strName = NULL); 05124 05132 XnStatus CreateBasedOn(ImageGenerator& other, const XnChar* strName = NULL); 05133 05138 inline XnStatus SetData(XnUInt32 nFrameID, XnUInt64 nTimestamp, XnUInt32 nDataSize, const XnUInt8* pImageMap) 05139 { 05140 return xnMockImageSetData(GetHandle(), nFrameID, nTimestamp, nDataSize, pImageMap); 05141 } 05142 05151 inline XnStatus SetData(const ImageMetaData& imageMD, XnUInt32 nFrameID, XnUInt64 nTimestamp) 05152 { 05153 return SetData(nFrameID, nTimestamp, imageMD.DataSize(), imageMD.Data()); 05154 } 05155 05161 inline XnStatus SetData(const ImageMetaData& imageMD) 05162 { 05163 return SetData(imageMD, imageMD.FrameID(), imageMD.Timestamp()); 05164 } 05165 }; 05166 05175 class IRGenerator : public MapGenerator 05176 { 05177 public: 05183 inline IRGenerator(XnNodeHandle hNode = NULL) : MapGenerator(hNode) {} 05184 inline IRGenerator(const NodeWrapper& other) : MapGenerator(other) {} 05185 05192 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 05193 05210 inline void GetMetaData(IRMetaData& metaData) const 05211 { 05212 xnGetIRMetaData(GetHandle(), metaData.GetUnderlying()); 05213 } 05214 05218 inline const XnIRPixel* GetIRMap() const 05219 { 05220 return xnGetIRMap(GetHandle()); 05221 } 05222 }; 05223 05228 class MockIRGenerator : public IRGenerator 05229 { 05230 public: 05236 inline MockIRGenerator(XnNodeHandle hNode = NULL) : IRGenerator(hNode) {} 05237 inline MockIRGenerator(const NodeWrapper& other) : IRGenerator(other) {} 05238 05245 XnStatus Create(Context& context, const XnChar* strName = NULL); 05252 XnStatus CreateBasedOn(IRGenerator& other, const XnChar* strName = NULL); 05253 05258 inline XnStatus SetData(XnUInt32 nFrameID, XnUInt64 nTimestamp, XnUInt32 nDataSize, const XnIRPixel* pIRMap) 05259 { 05260 return xnMockIRSetData(GetHandle(), nFrameID, nTimestamp, nDataSize, pIRMap); 05261 } 05262 05271 inline XnStatus SetData(const IRMetaData& irMD, XnUInt32 nFrameID, XnUInt64 nTimestamp) 05272 { 05273 return SetData(nFrameID, nTimestamp, irMD.DataSize(), irMD.Data()); 05274 } 05275 05281 inline XnStatus SetData(const IRMetaData& irMD) 05282 { 05283 return SetData(irMD, irMD.FrameID(), irMD.Timestamp()); 05284 } 05285 }; 05286 05354 class GestureGenerator : public Generator 05355 { 05356 public: 05362 inline GestureGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 05363 inline GestureGenerator(const NodeWrapper& other) : Generator(other) {} 05364 05378 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 05379 05393 inline XnStatus AddGesture(const XnChar* strGesture, XnBoundingBox3D* pArea) 05394 { 05395 return xnAddGesture(GetHandle(), strGesture, pArea); 05396 } 05397 05405 inline XnStatus RemoveGesture(const XnChar* strGesture) 05406 { 05407 return xnRemoveGesture(GetHandle(), strGesture); 05408 } 05409 05418 inline XnStatus GetAllActiveGestures(XnChar** astrGestures, XnUInt32 nNameLength, XnUInt16& nGestures) const 05419 { 05420 return xnGetAllActiveGestures(GetHandle(), astrGestures, nNameLength, &nGestures); 05421 } 05422 05427 inline XnUInt16 GetNumberOfAvailableGestures() const 05428 { 05429 return xnGetNumberOfAvailableGestures(GetHandle()); 05430 } 05431 05440 inline XnStatus EnumerateAllGestures(XnChar** astrGestures, XnUInt32 nNameLength, XnUInt16& nGestures) const 05441 { 05442 return xnEnumerateAllGestures(GetHandle(), astrGestures, nNameLength, &nGestures); 05443 } 05444 05450 inline XnBool IsGestureAvailable(const XnChar* strGesture) const 05451 { 05452 return xnIsGestureAvailable(GetHandle(), strGesture); 05453 } 05454 05459 inline XnBool IsGestureProgressSupported(const XnChar* strGesture) const 05460 { 05461 return xnIsGestureProgressSupported(GetHandle(), strGesture); 05462 } 05463 05485 typedef void (XN_CALLBACK_TYPE* GestureRecognized)(GestureGenerator& generator, const XnChar* strGesture, const XnPoint3D* pIDPosition, const XnPoint3D* pEndPosition, void* pCookie); 05486 05513 typedef void (XN_CALLBACK_TYPE* GestureProgress)(GestureGenerator& generator, const XnChar* strGesture, const XnPoint3D* pPosition, XnFloat fProgress, void* pCookie); 05514 05526 XnStatus RegisterGestureCallbacks(GestureRecognized RecognizedCB, GestureProgress ProgressCB, void* pCookie, XnCallbackHandle& hCallback) 05527 { 05528 XnStatus nRetVal = XN_STATUS_OK; 05529 05530 GestureCookie* pGestureCookie; 05531 XN_VALIDATE_ALLOC(pGestureCookie, GestureCookie); 05532 pGestureCookie->recognizedHandler = RecognizedCB; 05533 pGestureCookie->progressHandler = ProgressCB; 05534 pGestureCookie->pUserCookie = pCookie; 05535 05536 nRetVal = xnRegisterGestureCallbacks(GetHandle(), GestureRecognizedCallback, GestureProgressCallback, pGestureCookie, &pGestureCookie->hCallback); 05537 if (nRetVal != XN_STATUS_OK) 05538 { 05539 xnOSFree(pGestureCookie); 05540 return (nRetVal); 05541 } 05542 05543 hCallback = pGestureCookie; 05544 05545 return (XN_STATUS_OK); 05546 } 05547 05555 inline void UnregisterGestureCallbacks(XnCallbackHandle hCallback) 05556 { 05557 GestureCookie* pGestureCookie = (GestureCookie*)hCallback; 05558 xnUnregisterGestureCallbacks(GetHandle(), pGestureCookie->hCallback); 05559 xnOSFree(pGestureCookie); 05560 } 05561 05571 inline XnStatus RegisterToGestureChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 05572 { 05573 return _RegisterToStateChange(xnRegisterToGestureChange, GetHandle(), handler, pCookie, hCallback); 05574 } 05575 05583 inline void UnregisterFromGestureChange(XnCallbackHandle hCallback) 05584 { 05585 _UnregisterFromStateChange(xnUnregisterFromGestureChange, GetHandle(), hCallback); 05586 } 05587 05615 typedef void (XN_CALLBACK_TYPE* GestureIntermediateStageCompleted)(GestureGenerator& generator, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 05616 05626 XnStatus RegisterToGestureIntermediateStageCompleted(GestureIntermediateStageCompleted handler, void* pCookie, XnCallbackHandle& hCallback) 05627 { 05628 XnStatus nRetVal = XN_STATUS_OK; 05629 05630 GestureIntermediateStageCompletedCookie* pGestureCookie; 05631 XN_VALIDATE_ALLOC(pGestureCookie, GestureIntermediateStageCompletedCookie); 05632 pGestureCookie->handler = handler; 05633 pGestureCookie->pUserCookie = pCookie; 05634 05635 nRetVal = xnRegisterToGestureIntermediateStageCompleted(GetHandle(), GestureIntermediateStageCompletedCallback, pGestureCookie, &pGestureCookie->hCallback); 05636 if (nRetVal != XN_STATUS_OK) 05637 { 05638 xnOSFree(pGestureCookie); 05639 return (nRetVal); 05640 } 05641 05642 hCallback = pGestureCookie; 05643 05644 return (XN_STATUS_OK); 05645 } 05646 05654 inline void UnregisterFromGestureIntermediateStageCompleted(XnCallbackHandle hCallback) 05655 { 05656 GestureIntermediateStageCompletedCookie* pGestureCookie = (GestureIntermediateStageCompletedCookie*)hCallback; 05657 xnUnregisterFromGestureIntermediateStageCompleted(GetHandle(), pGestureCookie->hCallback); 05658 xnOSFree(pGestureCookie); 05659 } 05660 05679 typedef void (XN_CALLBACK_TYPE* GestureReadyForNextIntermediateStage)(GestureGenerator& generator, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 05680 05690 XnStatus RegisterToGestureReadyForNextIntermediateStage(GestureReadyForNextIntermediateStage handler, void* pCookie, XnCallbackHandle& hCallback) 05691 { 05692 XnStatus nRetVal = XN_STATUS_OK; 05693 05694 GestureReadyForNextIntermediateStageCookie* pGestureCookie; 05695 XN_VALIDATE_ALLOC(pGestureCookie, GestureReadyForNextIntermediateStageCookie); 05696 pGestureCookie->handler = handler; 05697 pGestureCookie->pUserCookie = pCookie; 05698 05699 nRetVal = xnRegisterToGestureReadyForNextIntermediateStage(GetHandle(), GestureReadyForNextIntermediateStageCallback, pGestureCookie, &pGestureCookie->hCallback); 05700 if (nRetVal != XN_STATUS_OK) 05701 { 05702 xnOSFree(pGestureCookie); 05703 return (nRetVal); 05704 } 05705 05706 hCallback = pGestureCookie; 05707 05708 return (XN_STATUS_OK); 05709 } 05710 05718 inline void UnregisterFromGestureReadyForNextIntermediateStageCallbacks(XnCallbackHandle hCallback) 05719 { 05720 GestureReadyForNextIntermediateStageCookie* pGestureCookie = (GestureReadyForNextIntermediateStageCookie*)hCallback; 05721 xnUnregisterFromGestureReadyForNextIntermediateStage(GetHandle(), pGestureCookie->hCallback); 05722 xnOSFree(pGestureCookie); 05723 } 05724 05726 inline XnStatus XN_API_DEPRECATED("Use GetAllActiveGestures() instead") GetActiveGestures(XnChar*& astrGestures, XnUInt16& nGestures) const 05727 { 05728 return xnGetActiveGestures(GetHandle(), &astrGestures, &nGestures); 05729 } 05730 inline XnStatus XN_API_DEPRECATED("Use EnumerateAllGestures() instead") EnumerateGestures(XnChar*& astrGestures, XnUInt16& nGestures) const 05731 { 05732 return xnEnumerateGestures(GetHandle(), &astrGestures, &nGestures); 05733 } 05736 private: 05737 typedef struct GestureCookie 05738 { 05739 GestureRecognized recognizedHandler; 05740 GestureProgress progressHandler; 05741 void* pUserCookie; 05742 XnCallbackHandle hCallback; 05743 } GestureCookie; 05744 05745 static void XN_CALLBACK_TYPE GestureRecognizedCallback(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pIDPosition, const XnPoint3D* pEndPosition, void* pCookie) 05746 { 05747 GestureCookie* pGestureCookie = (GestureCookie*)pCookie; 05748 GestureGenerator gen(hNode); 05749 if (pGestureCookie->recognizedHandler != NULL) 05750 { 05751 pGestureCookie->recognizedHandler(gen, strGesture, pIDPosition, pEndPosition, pGestureCookie->pUserCookie); 05752 } 05753 } 05754 05755 static void XN_CALLBACK_TYPE GestureProgressCallback(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, XnFloat fProgress, void* pCookie) 05756 { 05757 GestureCookie* pGestureCookie = (GestureCookie*)pCookie; 05758 GestureGenerator gen(hNode); 05759 if (pGestureCookie->progressHandler != NULL) 05760 { 05761 pGestureCookie->progressHandler(gen, strGesture, pPosition, fProgress, pGestureCookie->pUserCookie); 05762 } 05763 } 05764 05765 typedef struct GestureIntermediateStageCompletedCookie 05766 { 05767 GestureIntermediateStageCompleted handler; 05768 void* pUserCookie; 05769 XnCallbackHandle hCallback; 05770 } GestureIntermediateStageCompletedCookie; 05771 05772 static void XN_CALLBACK_TYPE GestureIntermediateStageCompletedCallback(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie) 05773 { 05774 GestureIntermediateStageCompletedCookie* pGestureCookie = (GestureIntermediateStageCompletedCookie*)pCookie; 05775 GestureGenerator gen(hNode); 05776 if (pGestureCookie->handler != NULL) 05777 { 05778 pGestureCookie->handler(gen, strGesture, pPosition, pGestureCookie->pUserCookie); 05779 } 05780 } 05781 05782 typedef struct GestureReadyForNextIntermediateStageCookie 05783 { 05784 GestureReadyForNextIntermediateStage handler; 05785 void* pUserCookie; 05786 XnCallbackHandle hCallback; 05787 } GestureReadyForNextIntermediateStageCookie; 05788 05789 static void XN_CALLBACK_TYPE GestureReadyForNextIntermediateStageCallback(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie) 05790 { 05791 GestureReadyForNextIntermediateStageCookie* pGestureCookie = (GestureReadyForNextIntermediateStageCookie*)pCookie; 05792 GestureGenerator gen(hNode); 05793 if (pGestureCookie->handler != NULL) 05794 { 05795 pGestureCookie->handler(gen, strGesture, pPosition, pGestureCookie->pUserCookie); 05796 } 05797 } 05798 }; 05799 05818 class SceneAnalyzer : public MapGenerator 05819 { 05820 public: 05826 inline SceneAnalyzer(XnNodeHandle hNode = NULL) : MapGenerator(hNode) {} 05827 inline SceneAnalyzer(const NodeWrapper& other) : MapGenerator(other) {} 05828 05836 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 05837 05838 05858 inline void GetMetaData(SceneMetaData& metaData) const 05859 { 05860 xnGetSceneMetaData(GetHandle(), metaData.GetUnderlying()); 05861 } 05862 05867 inline const XnLabel* GetLabelMap() const 05868 { 05869 return xnGetLabelMap(GetHandle()); 05870 } 05871 05877 inline XnStatus GetFloor(XnPlane3D& Plane) const 05878 { 05879 return xnGetFloor(GetHandle(), &Plane); 05880 } 05881 }; 05882 05890 class HandTouchingFOVEdgeCapability : public Capability 05891 { 05892 public: 05898 inline HandTouchingFOVEdgeCapability(XnNodeHandle hNode) : Capability(hNode) {} 05899 HandTouchingFOVEdgeCapability(const NodeWrapper& node) : Capability(node) {} 05900 05911 typedef void (XN_CALLBACK_TYPE* HandTouchingFOVEdge)(HandTouchingFOVEdgeCapability& touchingfov, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, XnDirection eDir, void* pCookie); 05912 05922 inline XnStatus RegisterToHandTouchingFOVEdge(HandTouchingFOVEdge handler, void* pCookie, XnCallbackHandle& hCallback) 05923 { 05924 XnStatus nRetVal = XN_STATUS_OK; 05925 05926 HandTouchingFOVEdgeCookie* pHandCookie; 05927 XN_VALIDATE_ALLOC(pHandCookie, HandTouchingFOVEdgeCookie); 05928 pHandCookie->handler = handler; 05929 pHandCookie->pUserCookie = pCookie; 05930 05931 nRetVal = xnRegisterToHandTouchingFOVEdge(GetHandle(), HandTouchingFOVEdgeCB, pHandCookie, &pHandCookie->hCallback); 05932 if (nRetVal != XN_STATUS_OK) 05933 { 05934 xnOSFree(pHandCookie); 05935 return (nRetVal); 05936 } 05937 05938 hCallback = pHandCookie; 05939 05940 return (XN_STATUS_OK); 05941 } 05942 05950 inline void UnregisterFromHandTouchingFOVEdge(XnCallbackHandle hCallback) 05951 { 05952 HandTouchingFOVEdgeCookie* pHandCookie = (HandTouchingFOVEdgeCookie*)hCallback; 05953 xnUnregisterFromHandTouchingFOVEdge(GetHandle(), pHandCookie->hCallback); 05954 xnOSFree(pHandCookie); 05955 } 05956 private: 05957 typedef struct HandTouchingFOVEdgeCookie 05958 { 05959 HandTouchingFOVEdge handler; 05960 void* pUserCookie; 05961 XnCallbackHandle hCallback; 05962 } HandTouchingFOVEdgeCookie; 05963 05964 static void XN_CALLBACK_TYPE HandTouchingFOVEdgeCB(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, XnDirection eDir, void* pCookie) 05965 { 05966 HandTouchingFOVEdgeCookie* pHandCookie = (HandTouchingFOVEdgeCookie*)pCookie; 05967 HandTouchingFOVEdgeCapability cap(hNode); 05968 if (pHandCookie->handler != NULL) 05969 { 05970 pHandCookie->handler(cap, user, pPosition, fTime, eDir, pHandCookie->pUserCookie); 05971 } 05972 } 05973 05974 }; 05975 06063 class HandsGenerator : public Generator 06064 { 06065 public: 06071 inline HandsGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 06072 inline HandsGenerator(const NodeWrapper& other) : Generator(other) {} 06073 06081 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 06082 06098 typedef void (XN_CALLBACK_TYPE* HandCreate)(HandsGenerator& generator, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 06099 06116 typedef void (XN_CALLBACK_TYPE* HandUpdate)(HandsGenerator& generator, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 06117 06131 typedef void (XN_CALLBACK_TYPE* HandDestroy)(HandsGenerator& generator, XnUserID user, XnFloat fTime, void* pCookie); 06132 06145 inline XnStatus RegisterHandCallbacks(HandCreate CreateCB, HandUpdate UpdateCB, HandDestroy DestroyCB, void* pCookie, XnCallbackHandle& hCallback) 06146 { 06147 XnStatus nRetVal = XN_STATUS_OK; 06148 06149 HandCookie* pHandCookie; 06150 XN_VALIDATE_ALLOC(pHandCookie, HandCookie); 06151 pHandCookie->createHandler = CreateCB; 06152 pHandCookie->updateHandler = UpdateCB; 06153 pHandCookie->destroyHandler = DestroyCB; 06154 pHandCookie->pUserCookie = pCookie; 06155 06156 nRetVal = xnRegisterHandCallbacks(GetHandle(), HandCreateCB, HandUpdateCB, HandDestroyCB, pHandCookie, &pHandCookie->hCallback); 06157 if (nRetVal != XN_STATUS_OK) 06158 { 06159 xnOSFree(pHandCookie); 06160 return (nRetVal); 06161 } 06162 06163 hCallback = pHandCookie; 06164 06165 return (XN_STATUS_OK); 06166 } 06167 06175 inline void UnregisterHandCallbacks(XnCallbackHandle hCallback) 06176 { 06177 HandCookie* pHandCookie = (HandCookie*)hCallback; 06178 xnUnregisterHandCallbacks(GetHandle(), pHandCookie->hCallback); 06179 xnOSFree(pHandCookie); 06180 } 06181 06195 inline XnStatus StopTracking(XnUserID user) 06196 { 06197 return xnStopTracking(GetHandle(), user); 06198 } 06199 06208 inline XnStatus StopTrackingAll() 06209 { 06210 return xnStopTrackingAll(GetHandle()); 06211 } 06212 06229 inline XnStatus StartTracking(const XnPoint3D& ptPosition) 06230 { 06231 return xnStartTracking(GetHandle(), &ptPosition); 06232 } 06233 06250 inline XnStatus SetSmoothing(XnFloat fSmoothingFactor) 06251 { 06252 return xnSetTrackingSmoothing(GetHandle(), fSmoothingFactor); 06253 } 06254 06262 inline const HandTouchingFOVEdgeCapability GetHandTouchingFOVEdgeCap() const 06263 { 06264 return HandTouchingFOVEdgeCapability(GetHandle()); 06265 } 06266 06274 inline HandTouchingFOVEdgeCapability GetHandTouchingFOVEdgeCap() 06275 { 06276 return HandTouchingFOVEdgeCapability(GetHandle()); 06277 } 06278 06279 private: 06280 typedef struct HandCookie 06281 { 06282 HandCreate createHandler; 06283 HandUpdate updateHandler; 06284 HandDestroy destroyHandler; 06285 void* pUserCookie; 06286 XnCallbackHandle hCallback; 06287 } HandCookie; 06288 06289 static void XN_CALLBACK_TYPE HandCreateCB(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie) 06290 { 06291 HandCookie* pHandCookie = (HandCookie*)pCookie; 06292 HandsGenerator gen(hNode); 06293 if (pHandCookie->createHandler != NULL) 06294 { 06295 pHandCookie->createHandler(gen, user, pPosition, fTime, pHandCookie->pUserCookie); 06296 } 06297 } 06298 static void XN_CALLBACK_TYPE HandUpdateCB(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie) 06299 { 06300 HandCookie* pHandCookie = (HandCookie*)pCookie; 06301 HandsGenerator gen(hNode); 06302 if (pHandCookie->updateHandler != NULL) 06303 { 06304 pHandCookie->updateHandler(gen, user, pPosition, fTime, pHandCookie->pUserCookie); 06305 } 06306 } 06307 static void XN_CALLBACK_TYPE HandDestroyCB(XnNodeHandle hNode, XnUserID user, XnFloat fTime, void* pCookie) 06308 { 06309 HandCookie* pHandCookie = (HandCookie*)pCookie; 06310 HandsGenerator gen(hNode); 06311 if (pHandCookie->destroyHandler != NULL) 06312 { 06313 pHandCookie->destroyHandler(gen, user, fTime, pHandCookie->pUserCookie); 06314 } 06315 } 06316 }; 06317 06374 class SkeletonCapability : public Capability 06375 { 06376 public: 06382 inline SkeletonCapability(XnNodeHandle hNode) : Capability(hNode) {} 06383 SkeletonCapability(const NodeWrapper& node) : Capability(node) {} 06384 06390 inline XnBool IsJointAvailable(XnSkeletonJoint eJoint) const 06391 { 06392 return xnIsJointAvailable(GetHandle(), eJoint); 06393 } 06394 06400 inline XnBool IsProfileAvailable(XnSkeletonProfile eProfile) const 06401 { 06402 return xnIsProfileAvailable(GetHandle(), eProfile); 06403 } 06404 06435 inline XnStatus SetSkeletonProfile(XnSkeletonProfile eProfile) 06436 { 06437 return xnSetSkeletonProfile(GetHandle(), eProfile); 06438 } 06439 06463 inline XnStatus SetJointActive(XnSkeletonJoint eJoint, XnBool bState) 06464 { 06465 return xnSetJointActive(GetHandle(), eJoint, bState); 06466 } 06467 06473 inline XnBool IsJointActive(XnSkeletonJoint eJoint) const 06474 { 06475 return xnIsJointActive(GetHandle(), eJoint); 06476 } 06477 06487 inline XnStatus RegisterToJointConfigurationChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 06488 { 06489 return _RegisterToStateChange(xnRegisterToJointConfigurationChange, GetHandle(), handler, pCookie, hCallback); 06490 } 06491 06499 inline void UnregisterFromJointConfigurationChange(XnCallbackHandle hCallback) 06500 { 06501 _UnregisterFromStateChange(xnUnregisterFromJointConfigurationChange, GetHandle(), hCallback); 06502 } 06503 06508 inline XnStatus EnumerateActiveJoints(XnSkeletonJoint* pJoints, XnUInt16& nJoints) const 06509 { 06510 return xnEnumerateActiveJoints(GetHandle(), pJoints, &nJoints); 06511 } 06512 06521 inline XnStatus GetSkeletonJoint(XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointTransformation& Joint) const 06522 { 06523 return xnGetSkeletonJoint(GetHandle(), user, eJoint, &Joint); 06524 } 06525 06547 inline XnStatus GetSkeletonJointPosition(XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointPosition& Joint) const 06548 { 06549 return xnGetSkeletonJointPosition(GetHandle(), user, eJoint, &Joint); 06550 } 06551 06559 inline XnStatus GetSkeletonJointOrientation(XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointOrientation& Joint) const 06560 { 06561 return xnGetSkeletonJointOrientation(GetHandle(), user, eJoint, &Joint); 06562 } 06563 06574 inline XnBool IsTracking(XnUserID user) const 06575 { 06576 return xnIsSkeletonTracking(GetHandle(), user); 06577 } 06578 06589 inline XnBool IsCalibrated(XnUserID user) const 06590 { 06591 return xnIsSkeletonCalibrated(GetHandle(), user); 06592 } 06593 06604 inline XnBool IsCalibrating(XnUserID user) const 06605 { 06606 return xnIsSkeletonCalibrating(GetHandle(), user); 06607 } 06608 06633 inline XnStatus RequestCalibration(XnUserID user, XnBool bForce) 06634 { 06635 return xnRequestSkeletonCalibration(GetHandle(), user, bForce); 06636 } 06637 06647 inline XnStatus AbortCalibration(XnUserID user) 06648 { 06649 return xnAbortSkeletonCalibration(GetHandle(), user); 06650 } 06651 06670 inline XnStatus SaveCalibrationDataToFile(XnUserID user, const XnChar* strFileName) 06671 { 06672 return xnSaveSkeletonCalibrationDataToFile(GetHandle(), user, strFileName); 06673 } 06674 06681 inline XnStatus LoadCalibrationDataFromFile(XnUserID user, const XnChar* strFileName) 06682 { 06683 return xnLoadSkeletonCalibrationDataFromFile(GetHandle(), user, strFileName); 06684 } 06685 06697 inline XnStatus SaveCalibrationData(XnUserID user, XnUInt32 nSlot) 06698 { 06699 return xnSaveSkeletonCalibrationData(GetHandle(), user, nSlot); 06700 } 06701 06708 inline XnStatus LoadCalibrationData(XnUserID user, XnUInt32 nSlot) 06709 { 06710 return xnLoadSkeletonCalibrationData(GetHandle(), user, nSlot); 06711 } 06712 06718 inline XnStatus ClearCalibrationData(XnUInt32 nSlot) 06719 { 06720 return xnClearSkeletonCalibrationData(GetHandle(), nSlot); 06721 } 06722 06728 inline XnBool IsCalibrationData(XnUInt32 nSlot) const 06729 { 06730 return xnIsSkeletonCalibrationData(GetHandle(), nSlot); 06731 } 06732 06747 inline XnStatus StartTracking(XnUserID user) 06748 { 06749 return xnStartSkeletonTracking(GetHandle(), user); 06750 } 06751 06756 inline XnStatus StopTracking(XnUserID user) 06757 { 06758 return xnStopSkeletonTracking(GetHandle(), user); 06759 } 06760 06769 inline XnStatus Reset(XnUserID user) 06770 { 06771 return xnResetSkeleton(GetHandle(), user); 06772 } 06773 06782 inline XnBool NeedPoseForCalibration() const 06783 { 06784 return xnNeedPoseForSkeletonCalibration(GetHandle()); 06785 } 06786 06799 inline XnStatus GetCalibrationPose(XnChar* strPose) const 06800 { 06801 return xnGetSkeletonCalibrationPose(GetHandle(), strPose); 06802 } 06803 06816 inline XnStatus SetSmoothing(XnFloat fSmoothingFactor) 06817 { 06818 return xnSetSkeletonSmoothing(GetHandle(), fSmoothingFactor); 06819 } 06820 06842 typedef void (XN_CALLBACK_TYPE* CalibrationStart)(SkeletonCapability& skeleton, XnUserID user, void* pCookie); 06843 06861 typedef void (XN_CALLBACK_TYPE* CalibrationEnd)(SkeletonCapability& skeleton, XnUserID user, XnBool bSuccess, void* pCookie); 06862 06874 inline XnStatus RegisterToCalibrationStart(CalibrationStart handler, void* pCookie, XnCallbackHandle& hCallback) 06875 { 06876 XnStatus nRetVal = XN_STATUS_OK; 06877 CalibrationStartCookie* pCalibrationCookie; 06878 XN_VALIDATE_ALLOC(pCalibrationCookie, CalibrationStartCookie); 06879 pCalibrationCookie->handler = handler; 06880 pCalibrationCookie->pUserCookie = pCookie; 06881 nRetVal = xnRegisterToCalibrationStart(GetHandle(), CalibrationStartCallback, pCalibrationCookie, &pCalibrationCookie->hCallback); 06882 if (nRetVal != XN_STATUS_OK) 06883 { 06884 xnOSFree(pCalibrationCookie); 06885 return nRetVal; 06886 } 06887 hCallback = pCalibrationCookie; 06888 return XN_STATUS_OK; 06889 } 06890 06901 inline XnStatus UnregisterFromCalibrationStart(XnCallbackHandle hCallback) 06902 { 06903 CalibrationStartCookie* pCalibrationCookie = (CalibrationStartCookie*)hCallback; 06904 xnUnregisterFromCalibrationStart(GetHandle(), pCalibrationCookie->hCallback); 06905 xnOSFree(pCalibrationCookie); 06906 return XN_STATUS_OK; 06907 } 06908 06930 typedef void (XN_CALLBACK_TYPE* CalibrationInProgress)(SkeletonCapability& skeleton, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 06931 06941 inline XnStatus RegisterToCalibrationInProgress(CalibrationInProgress handler, void* pCookie, XnCallbackHandle& hCallback) 06942 { 06943 XnStatus nRetVal = XN_STATUS_OK; 06944 06945 CalibrationInProgressCookie* pSkeletonCookie; 06946 XN_VALIDATE_ALLOC(pSkeletonCookie, CalibrationInProgressCookie); 06947 pSkeletonCookie->handler = handler; 06948 pSkeletonCookie->pUserCookie = pCookie; 06949 06950 nRetVal = xnRegisterToCalibrationInProgress(GetHandle(), CalibrationInProgressCallback, pSkeletonCookie, &pSkeletonCookie->hCallback); 06951 if (nRetVal != XN_STATUS_OK) 06952 { 06953 xnOSFree(pSkeletonCookie); 06954 return (nRetVal); 06955 } 06956 06957 hCallback = pSkeletonCookie; 06958 06959 return (XN_STATUS_OK); 06960 } 06961 06969 inline void UnregisterFromCalibrationInProgress(XnCallbackHandle hCallback) 06970 { 06971 CalibrationInProgressCookie* pSkeletonCookie = (CalibrationInProgressCookie*)hCallback; 06972 xnUnregisterFromCalibrationInProgress(GetHandle(), pSkeletonCookie->hCallback); 06973 xnOSFree(pSkeletonCookie); 06974 } 06975 06993 typedef void (XN_CALLBACK_TYPE* CalibrationComplete)(SkeletonCapability& skeleton, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 06994 07004 inline XnStatus RegisterToCalibrationComplete(CalibrationComplete handler, void* pCookie, XnCallbackHandle& hCallback) 07005 { 07006 XnStatus nRetVal = XN_STATUS_OK; 07007 07008 CalibrationCompleteCookie* pSkeletonCookie; 07009 XN_VALIDATE_ALLOC(pSkeletonCookie, CalibrationCompleteCookie); 07010 pSkeletonCookie->handler = handler; 07011 pSkeletonCookie->pUserCookie = pCookie; 07012 07013 nRetVal = xnRegisterToCalibrationComplete(GetHandle(), CalibrationCompleteCallback, pSkeletonCookie, &pSkeletonCookie->hCallback); 07014 if (nRetVal != XN_STATUS_OK) 07015 { 07016 xnOSFree(pSkeletonCookie); 07017 return (nRetVal); 07018 } 07019 07020 hCallback = pSkeletonCookie; 07021 07022 return (XN_STATUS_OK); 07023 } 07024 07032 inline void UnregisterFromCalibrationComplete(XnCallbackHandle hCallback) 07033 { 07034 CalibrationCompleteCookie* pSkeletonCookie = (CalibrationCompleteCookie*)hCallback; 07035 xnUnregisterFromCalibrationComplete(GetHandle(), pSkeletonCookie->hCallback); 07036 xnOSFree(pSkeletonCookie); 07037 } 07038 07040 XN_API_DEPRECATED("Use the overload with one argument - the bState parameter is useless") 07041 inline XnBool IsJointActive(XnSkeletonJoint eJoint, XnBool /*bState*/) const 07042 { 07043 return xnIsJointActive(GetHandle(), eJoint); 07044 } 07045 07046 inline XnStatus XN_API_DEPRECATED("Please use RegisterToCalibrationStart/Complete") RegisterCalibrationCallbacks(CalibrationStart CalibrationStartCB, CalibrationEnd CalibrationEndCB, void* pCookie, XnCallbackHandle& hCallback) 07047 { 07048 XnStatus nRetVal = XN_STATUS_OK; 07049 07050 SkeletonCookie* pSkeletonCookie; 07051 XN_VALIDATE_ALLOC(pSkeletonCookie, SkeletonCookie); 07052 pSkeletonCookie->startHandler = CalibrationStartCB; 07053 pSkeletonCookie->endHandler = CalibrationEndCB; 07054 pSkeletonCookie->pUserCookie = pCookie; 07055 07056 #pragma warning (push) 07057 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 07058 nRetVal = xnRegisterCalibrationCallbacks(GetHandle(), CalibrationStartBundleCallback, CalibrationEndBundleCallback, pSkeletonCookie, &pSkeletonCookie->hCallback); 07059 #pragma warning (pop) 07060 if (nRetVal != XN_STATUS_OK) 07061 { 07062 xnOSFree(pSkeletonCookie); 07063 return (nRetVal); 07064 } 07065 07066 hCallback = pSkeletonCookie; 07067 07068 return (XN_STATUS_OK); 07069 } 07070 07071 inline void XN_API_DEPRECATED("Please use UnregisterFromCalibrationStart/Complete") UnregisterCalibrationCallbacks(XnCallbackHandle hCallback) 07072 { 07073 SkeletonCookie* pSkeletonCookie = (SkeletonCookie*)hCallback; 07074 #pragma warning (push) 07075 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 07076 xnUnregisterCalibrationCallbacks(GetHandle(), pSkeletonCookie->hCallback); 07077 #pragma warning (pop) 07078 xnOSFree(pSkeletonCookie); 07079 } 07082 private: 07083 typedef struct SkeletonCookie 07084 { 07085 CalibrationStart startHandler; 07086 CalibrationEnd endHandler; 07087 void* pUserCookie; 07088 XnCallbackHandle hCallback; 07089 } SkeletonCookie; 07090 07091 static void XN_CALLBACK_TYPE CalibrationStartBundleCallback(XnNodeHandle hNode, XnUserID user, void* pCookie) 07092 { 07093 SkeletonCookie* pSkeletonCookie = (SkeletonCookie*)pCookie; 07094 SkeletonCapability cap(hNode); 07095 if (pSkeletonCookie->startHandler != NULL) 07096 { 07097 pSkeletonCookie->startHandler(cap, user, pSkeletonCookie->pUserCookie); 07098 } 07099 } 07100 07101 static void XN_CALLBACK_TYPE CalibrationEndBundleCallback(XnNodeHandle hNode, XnUserID user, XnBool bSuccess, void* pCookie) 07102 { 07103 SkeletonCookie* pSkeletonCookie = (SkeletonCookie*)pCookie; 07104 SkeletonCapability cap(hNode); 07105 if (pSkeletonCookie->endHandler != NULL) 07106 { 07107 pSkeletonCookie->endHandler(cap, user, bSuccess, pSkeletonCookie->pUserCookie); 07108 } 07109 } 07110 07111 typedef struct CalibrationStartCookie 07112 { 07113 CalibrationStart handler; 07114 void* pUserCookie; 07115 XnCallbackHandle hCallback; 07116 } CalibrationStartCookie; 07117 07118 static void XN_CALLBACK_TYPE CalibrationStartCallback(XnNodeHandle hNode, XnUserID user, void* pCookie) 07119 { 07120 CalibrationStartCookie* pCalibrationCookie = (CalibrationStartCookie*)pCookie; 07121 SkeletonCapability cap(hNode); 07122 if (pCalibrationCookie->handler != NULL) 07123 { 07124 pCalibrationCookie->handler(cap, user, pCalibrationCookie->pUserCookie); 07125 } 07126 } 07127 07128 typedef struct CalibrationInProgressCookie 07129 { 07130 CalibrationInProgress handler; 07131 void* pUserCookie; 07132 XnCallbackHandle hCallback; 07133 } CalibrationInProgressCookie; 07134 07135 static void XN_CALLBACK_TYPE CalibrationInProgressCallback(XnNodeHandle hNode, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie) 07136 { 07137 CalibrationInProgressCookie* pSkeletonCookie = (CalibrationInProgressCookie*)pCookie; 07138 SkeletonCapability cap(hNode); 07139 if (pSkeletonCookie->handler != NULL) 07140 { 07141 pSkeletonCookie->handler(cap, user, calibrationError, pSkeletonCookie->pUserCookie); 07142 } 07143 } 07144 07145 typedef struct CalibrationCompleteCookie 07146 { 07147 CalibrationComplete handler; 07148 void* pUserCookie; 07149 XnCallbackHandle hCallback; 07150 } CalibrationCompleteCookie; 07151 07152 static void XN_CALLBACK_TYPE CalibrationCompleteCallback(XnNodeHandle hNode, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie) 07153 { 07154 CalibrationCompleteCookie* pSkeletonCookie = (CalibrationCompleteCookie*)pCookie; 07155 SkeletonCapability cap(hNode); 07156 if (pSkeletonCookie->handler != NULL) 07157 { 07158 pSkeletonCookie->handler(cap, user, calibrationError, pSkeletonCookie->pUserCookie); 07159 } 07160 } 07161 }; 07162 07184 class PoseDetectionCapability : public Capability 07185 { 07186 public: 07192 inline PoseDetectionCapability(XnNodeHandle hNode) : Capability(hNode) {} 07193 PoseDetectionCapability(const NodeWrapper& node) : Capability(node) {} 07194 07208 typedef void (XN_CALLBACK_TYPE* PoseDetection)(PoseDetectionCapability& pose, const XnChar* strPose, XnUserID user, void* pCookie); 07209 07219 inline XnUInt32 GetNumberOfPoses() const 07220 { 07221 return xnGetNumberOfPoses(GetHandle()); 07222 } 07223 07236 inline XnStatus GetAllAvailablePoses(XnChar** pstrPoses, XnUInt32 nNameLength, XnUInt32& nPoses) const 07237 { 07238 return xnGetAllAvailablePoses(GetHandle(), pstrPoses, nNameLength, &nPoses); 07239 } 07240 07241 inline XnBool IsPoseSupported(const XnChar* strPose) 07242 { 07243 return xnIsPoseSupported(GetHandle(), strPose); 07244 } 07245 07246 inline XnStatus GetPoseStatus(XnUserID userID, const XnChar* poseName, XnUInt64& poseTime, XnPoseDetectionStatus& eStatus, XnPoseDetectionState& eState) 07247 { 07248 return xnGetPoseStatus(GetHandle(), userID, poseName, &poseTime, &eStatus, &eState); 07249 } 07250 07266 inline XnStatus StartPoseDetection(const XnChar* strPose, XnUserID user) 07267 { 07268 return xnStartPoseDetection(GetHandle(), strPose, user); 07269 } 07270 07282 inline XnStatus StopPoseDetection(XnUserID user) 07283 { 07284 return xnStopPoseDetection(GetHandle(), user); 07285 } 07286 07290 inline XnStatus StopSinglePoseDetection(XnUserID user, const XnChar* strPose) 07291 { 07292 return xnStopSinglePoseDetection(GetHandle(), user, strPose); 07293 } 07294 07304 inline XnStatus RegisterToPoseDetected(PoseDetection handler, void* pCookie, XnCallbackHandle& hCallback) 07305 { 07306 XnStatus nRetVal = XN_STATUS_OK; 07307 PoseDetectionCookie* pPoseCookie; 07308 XN_VALIDATE_ALLOC(pPoseCookie, PoseDetectionCookie); 07309 pPoseCookie->handler = handler; 07310 pPoseCookie->pPoseCookie = pCookie; 07311 07312 nRetVal = xnRegisterToPoseDetected(GetHandle(), PoseDetectionCallback, pPoseCookie, &pPoseCookie->hCallback); 07313 if (nRetVal != XN_STATUS_OK) 07314 { 07315 xnOSFree(pPoseCookie); 07316 return nRetVal; 07317 } 07318 hCallback = pPoseCookie; 07319 return XN_STATUS_OK; 07320 } 07321 07331 inline XnStatus RegisterToOutOfPose(PoseDetection handler, void* pCookie, XnCallbackHandle& hCallback) 07332 { 07333 XnStatus nRetVal = XN_STATUS_OK; 07334 PoseDetectionCookie* pPoseCookie; 07335 XN_VALIDATE_ALLOC(pPoseCookie, PoseDetectionCookie); 07336 pPoseCookie->handler = handler; 07337 pPoseCookie->pPoseCookie = pCookie; 07338 07339 nRetVal = xnRegisterToOutOfPose(GetHandle(), PoseDetectionCallback, pPoseCookie, &pPoseCookie->hCallback); 07340 if (nRetVal != XN_STATUS_OK) 07341 { 07342 xnOSFree(pPoseCookie); 07343 return nRetVal; 07344 } 07345 hCallback = pPoseCookie; 07346 return XN_STATUS_OK; 07347 } 07355 inline void UnregisterFromPoseDetected(XnCallbackHandle hCallback) 07356 { 07357 PoseDetectionCookie* pPoseCookie = (PoseDetectionCookie*)hCallback; 07358 xnUnregisterFromPoseDetected(GetHandle(), pPoseCookie->hCallback); 07359 xnOSFree(pPoseCookie); 07360 } 07368 inline void UnregisterFromOutOfPose(XnCallbackHandle hCallback) 07369 { 07370 PoseDetectionCookie* pPoseCookie = (PoseDetectionCookie*)hCallback; 07371 xnUnregisterFromOutOfPose(GetHandle(), pPoseCookie->hCallback); 07372 xnOSFree(pPoseCookie); 07373 } 07374 07397 typedef void (XN_CALLBACK_TYPE* PoseInProgress)(PoseDetectionCapability& pose, const XnChar* strPose, XnUserID user, XnPoseDetectionStatus poseError, void* pCookie); 07398 07415 inline XnStatus RegisterToPoseInProgress(PoseInProgress handler, void* pCookie, XnCallbackHandle& hCallback) 07416 { 07417 XnStatus nRetVal = XN_STATUS_OK; 07418 07419 PoseInProgressCookie* pPoseCookie; 07420 XN_VALIDATE_ALLOC(pPoseCookie, PoseInProgressCookie); 07421 pPoseCookie->handler = handler; 07422 pPoseCookie->pPoseCookie = pCookie; 07423 07424 nRetVal = xnRegisterToPoseDetectionInProgress(GetHandle(), PoseDetectionInProgressCallback, pPoseCookie, &pPoseCookie->hCallback); 07425 if (nRetVal != XN_STATUS_OK) 07426 { 07427 xnOSFree(pPoseCookie); 07428 return (nRetVal); 07429 } 07430 07431 hCallback = pPoseCookie; 07432 07433 return (XN_STATUS_OK); 07434 } 07435 07443 inline void UnregisterFromPoseInProgress(XnCallbackHandle hCallback) 07444 { 07445 PoseInProgressCookie* pPoseCookie = (PoseInProgressCookie*)hCallback; 07446 xnUnregisterFromPoseDetectionInProgress(GetHandle(), pPoseCookie->hCallback); 07447 xnOSFree(pPoseCookie); 07448 } 07449 07451 inline XnStatus XN_API_DEPRECATED("Use GetAllAvailablePoses() instead") GetAvailablePoses(XnChar** pstrPoses, XnUInt32& nPoses) const 07452 { 07453 return xnGetAvailablePoses(GetHandle(), pstrPoses, &nPoses); 07454 } 07455 07456 inline XnStatus XN_API_DEPRECATED("Please use RegisterToPoseDetected/RegisterToOutOfPose instead") RegisterToPoseCallbacks(PoseDetection PoseStartCB, PoseDetection PoseEndCB, void* pCookie, XnCallbackHandle& hCallback) 07457 { 07458 XnStatus nRetVal = XN_STATUS_OK; 07459 07460 PoseCookie* pPoseCookie; 07461 XN_VALIDATE_ALLOC(pPoseCookie, PoseCookie); 07462 pPoseCookie->startHandler = PoseStartCB; 07463 pPoseCookie->endHandler = PoseEndCB; 07464 pPoseCookie->pPoseCookie = pCookie; 07465 07466 #pragma warning (push) 07467 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 07468 nRetVal = xnRegisterToPoseCallbacks(GetHandle(), PoseDetectionStartBundleCallback, PoseDetectionStartEndBundleCallback, pPoseCookie, &pPoseCookie->hCallback); 07469 #pragma warning (pop) 07470 if (nRetVal != XN_STATUS_OK) 07471 { 07472 xnOSFree(pPoseCookie); 07473 return (nRetVal); 07474 } 07475 07476 hCallback = pPoseCookie; 07477 07478 return (XN_STATUS_OK); 07479 } 07480 07481 inline void XN_API_DEPRECATED("Please use UnregisterFromPoseDetected/UnregisterFromOutOfPose instead") UnregisterFromPoseCallbacks(XnCallbackHandle hCallback) 07482 { 07483 PoseCookie* pPoseCookie = (PoseCookie*)hCallback; 07484 #pragma warning (push) 07485 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 07486 xnUnregisterFromPoseCallbacks(GetHandle(), pPoseCookie->hCallback); 07487 #pragma warning (pop) 07488 xnOSFree(pPoseCookie); 07489 } 07492 private: 07493 typedef struct PoseCookie 07494 { 07495 PoseDetection startHandler; 07496 PoseDetection endHandler; 07497 void* pPoseCookie; 07498 XnCallbackHandle hCallback; 07499 } PoseCookie; 07500 07501 static void XN_CALLBACK_TYPE PoseDetectionStartBundleCallback(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, void* pCookie) 07502 { 07503 PoseCookie* pPoseCookie = (PoseCookie*)pCookie; 07504 PoseDetectionCapability cap(hNode); 07505 if (pPoseCookie->startHandler != NULL) 07506 { 07507 pPoseCookie->startHandler(cap, strPose, user, pPoseCookie->pPoseCookie); 07508 } 07509 } 07510 07511 static void XN_CALLBACK_TYPE PoseDetectionStartEndBundleCallback(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, void* pCookie) 07512 { 07513 PoseCookie* pPoseCookie = (PoseCookie*)pCookie; 07514 PoseDetectionCapability cap(hNode); 07515 if (pPoseCookie->endHandler != NULL) 07516 { 07517 pPoseCookie->endHandler(cap, strPose, user, pPoseCookie->pPoseCookie); 07518 } 07519 } 07520 typedef struct PoseDetectionCookie 07521 { 07522 PoseDetection handler; 07523 void* pPoseCookie; 07524 XnCallbackHandle hCallback; 07525 } PoseDetectionCookie; 07526 static void XN_CALLBACK_TYPE PoseDetectionCallback(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, void* pCookie) 07527 { 07528 PoseDetectionCookie* pPoseDetectionCookie = (PoseDetectionCookie*)pCookie; 07529 PoseDetectionCapability cap(hNode); 07530 if (pPoseDetectionCookie->handler != NULL) 07531 { 07532 pPoseDetectionCookie->handler(cap, strPose, user, pPoseDetectionCookie->pPoseCookie); 07533 } 07534 } 07535 07536 typedef struct PoseInProgressCookie 07537 { 07538 PoseInProgress handler; 07539 void* pPoseCookie; 07540 XnCallbackHandle hCallback; 07541 } PoseInProgressCookie; 07542 07543 static void XN_CALLBACK_TYPE PoseDetectionInProgressCallback(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, XnPoseDetectionStatus poseErrors, void* pCookie) 07544 { 07545 PoseInProgressCookie* pPoseCookie = (PoseInProgressCookie*)pCookie; 07546 PoseDetectionCapability cap(hNode); 07547 if (pPoseCookie->handler != NULL) 07548 { 07549 pPoseCookie->handler(cap, strPose, user, poseErrors, pPoseCookie->pPoseCookie); 07550 } 07551 } 07552 }; 07553 07665 class UserGenerator : public Generator 07666 { 07667 public: 07673 inline UserGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 07674 inline UserGenerator(const NodeWrapper& other) : Generator(other) {} 07675 07683 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 07684 07697 typedef void (XN_CALLBACK_TYPE* UserHandler)(UserGenerator& generator, XnUserID user, void* pCookie); 07698 07706 inline XnUInt16 GetNumberOfUsers() const 07707 { 07708 return xnGetNumberOfUsers(GetHandle()); 07709 } 07710 07733 inline XnStatus GetUsers(XnUserID aUsers[], XnUInt16& nUsers) const 07734 { 07735 return xnGetUsers(GetHandle(), aUsers, &nUsers); 07736 } 07737 07757 inline XnStatus GetCoM(XnUserID user, XnPoint3D& com) const 07758 { 07759 return xnGetUserCoM(GetHandle(), user, &com); 07760 } 07761 07777 inline XnStatus GetUserPixels(XnUserID user, SceneMetaData& smd) const 07778 { 07779 return xnGetUserPixels(GetHandle(), user, smd.GetUnderlying()); 07780 } 07781 07792 inline XnStatus RegisterUserCallbacks(UserHandler NewUserCB, UserHandler LostUserCB, void* pCookie, XnCallbackHandle& hCallback) 07793 { 07794 XnStatus nRetVal = XN_STATUS_OK; 07795 07796 UserCookie* pUserCookie; 07797 XN_VALIDATE_ALLOC(pUserCookie, UserCookie); 07798 pUserCookie->newHandler = NewUserCB; 07799 pUserCookie->lostHandler = LostUserCB; 07800 pUserCookie->pUserCookie = pCookie; 07801 07802 nRetVal = xnRegisterUserCallbacks(GetHandle(), NewUserCallback, LostUserCallback, pUserCookie, &pUserCookie->hCallback); 07803 if (nRetVal != XN_STATUS_OK) 07804 { 07805 xnOSFree(pUserCookie); 07806 return (nRetVal); 07807 } 07808 07809 hCallback = pUserCookie; 07810 07811 return (XN_STATUS_OK); 07812 } 07813 07821 inline void UnregisterUserCallbacks(XnCallbackHandle hCallback) 07822 { 07823 UserCookie* pUserCookie = (UserCookie*)hCallback; 07824 xnUnregisterUserCallbacks(GetHandle(), pUserCookie->hCallback); 07825 xnOSFree(pUserCookie); 07826 } 07827 07839 inline const SkeletonCapability GetSkeletonCap() const 07840 { 07841 return SkeletonCapability(GetHandle()); 07842 } 07843 07855 inline SkeletonCapability GetSkeletonCap() 07856 { 07857 return SkeletonCapability(GetHandle()); 07858 } 07859 07870 inline const PoseDetectionCapability GetPoseDetectionCap() const 07871 { 07872 return PoseDetectionCapability(GetHandle()); 07873 } 07874 07885 inline PoseDetectionCapability GetPoseDetectionCap() 07886 { 07887 return PoseDetectionCapability(GetHandle()); 07888 } 07889 07899 inline XnStatus RegisterToUserExit(UserHandler handler, void* pCookie, XnCallbackHandle& hCallback) 07900 { 07901 XnStatus nRetVal = XN_STATUS_OK; 07902 07903 UserSingleCookie* pUserCookie; 07904 XN_VALIDATE_ALLOC(pUserCookie, UserSingleCookie); 07905 pUserCookie->handler = handler; 07906 pUserCookie->pUserCookie = pCookie; 07907 07908 nRetVal = xnRegisterToUserExit(GetHandle(), UserSingleCallback, pUserCookie, &pUserCookie->hCallback); 07909 if (nRetVal != XN_STATUS_OK) 07910 { 07911 xnOSFree(pUserCookie); 07912 return (nRetVal); 07913 } 07914 07915 hCallback = pUserCookie; 07916 07917 return (XN_STATUS_OK); 07918 } 07919 07927 inline void UnregisterFromUserExit(XnCallbackHandle hCallback) 07928 { 07929 UserSingleCookie* pUserCookie = (UserSingleCookie*)hCallback; 07930 xnUnregisterFromUserExit(GetHandle(), pUserCookie->hCallback); 07931 xnOSFree(pUserCookie); 07932 } 07933 07943 inline XnStatus RegisterToUserReEnter(UserHandler handler, void* pCookie, XnCallbackHandle& hCallback) 07944 { 07945 XnStatus nRetVal = XN_STATUS_OK; 07946 07947 UserSingleCookie* pUserCookie; 07948 XN_VALIDATE_ALLOC(pUserCookie, UserSingleCookie); 07949 pUserCookie->handler = handler; 07950 pUserCookie->pUserCookie = pCookie; 07951 07952 nRetVal = xnRegisterToUserReEnter(GetHandle(), UserSingleCallback, pUserCookie, &pUserCookie->hCallback); 07953 if (nRetVal != XN_STATUS_OK) 07954 { 07955 xnOSFree(pUserCookie); 07956 return (nRetVal); 07957 } 07958 07959 hCallback = pUserCookie; 07960 07961 return (XN_STATUS_OK); 07962 } 07963 07971 inline void UnregisterFromUserReEnter(XnCallbackHandle hCallback) 07972 { 07973 UserSingleCookie* pUserCookie = (UserSingleCookie*)hCallback; 07974 xnUnregisterFromUserReEnter(GetHandle(), pUserCookie->hCallback); 07975 xnOSFree(pUserCookie); 07976 } 07977 07978 private: 07979 typedef struct UserCookie 07980 { 07981 UserHandler newHandler; 07982 UserHandler lostHandler; 07983 void* pUserCookie; 07984 XnCallbackHandle hCallback; 07985 } UserCookie; 07986 07987 static void XN_CALLBACK_TYPE NewUserCallback(XnNodeHandle hNode, XnUserID user, void* pCookie) 07988 { 07989 UserCookie* pUserCookie = (UserCookie*)pCookie; 07990 UserGenerator gen(hNode); 07991 if (pUserCookie->newHandler != NULL) 07992 { 07993 pUserCookie->newHandler(gen, user, pUserCookie->pUserCookie); 07994 } 07995 } 07996 07997 static void XN_CALLBACK_TYPE LostUserCallback(XnNodeHandle hNode, XnUserID user, void* pCookie) 07998 { 07999 UserCookie* pUserCookie = (UserCookie*)pCookie; 08000 UserGenerator gen(hNode); 08001 if (pUserCookie->lostHandler != NULL) 08002 { 08003 pUserCookie->lostHandler(gen, user, pUserCookie->pUserCookie); 08004 } 08005 } 08006 08007 typedef struct UserSingleCookie 08008 { 08009 UserHandler handler; 08010 void* pUserCookie; 08011 XnCallbackHandle hCallback; 08012 } UserSingleCookie; 08013 08014 static void XN_CALLBACK_TYPE UserSingleCallback(XnNodeHandle hNode, XnUserID user, void* pCookie) 08015 { 08016 UserSingleCookie* pUserCookie = (UserSingleCookie*)pCookie; 08017 UserGenerator gen(hNode); 08018 if (pUserCookie->handler != NULL) 08019 { 08020 pUserCookie->handler(gen, user, pUserCookie->pUserCookie); 08021 } 08022 } 08023 }; 08024 08038 class AudioGenerator : public Generator 08039 { 08040 public: 08046 inline AudioGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 08047 inline AudioGenerator(const NodeWrapper& other) : Generator(other) {} 08048 08056 inline XnStatus Create(Context& context, Query* pQuery = NULL, EnumerationErrors* pErrors = NULL); 08057 08074 inline void GetMetaData(AudioMetaData& metaData) const 08075 { 08076 xnGetAudioMetaData(GetHandle(), metaData.GetUnderlying()); 08077 } 08078 08083 inline const XnUChar* GetAudioBuffer() const 08084 { 08085 return xnGetAudioBuffer(GetHandle()); 08086 } 08087 08091 inline XnUInt32 GetSupportedWaveOutputModesCount() const 08092 { 08093 return xnGetSupportedWaveOutputModesCount(GetHandle()); 08094 } 08095 08102 inline XnStatus GetSupportedWaveOutputModes(XnWaveOutputMode* aSupportedModes, XnUInt32& nCount) const 08103 { 08104 return xnGetSupportedWaveOutputModes(GetHandle(), aSupportedModes, &nCount); 08105 } 08106 08118 inline XnStatus SetWaveOutputMode(const XnWaveOutputMode& OutputMode) 08119 { 08120 return xnSetWaveOutputMode(GetHandle(), &OutputMode); 08121 } 08122 08134 inline XnStatus GetWaveOutputMode(XnWaveOutputMode& OutputMode) const 08135 { 08136 return xnGetWaveOutputMode(GetHandle(), &OutputMode); 08137 } 08138 08148 inline XnStatus RegisterToWaveOutputModeChanges(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 08149 { 08150 return _RegisterToStateChange(xnRegisterToWaveOutputModeChanges, GetHandle(), handler, pCookie, hCallback); 08151 } 08152 08160 inline void UnregisterFromWaveOutputModeChanges(XnCallbackHandle hCallback) 08161 { 08162 _UnregisterFromStateChange(xnUnregisterFromWaveOutputModeChanges, GetHandle(), hCallback); 08163 } 08164 }; 08165 08170 class MockAudioGenerator : public AudioGenerator 08171 { 08172 public: 08178 inline MockAudioGenerator(XnNodeHandle hNode = NULL) : AudioGenerator(hNode) {} 08179 inline MockAudioGenerator(const NodeWrapper& other) : AudioGenerator(other) {} 08180 08187 XnStatus Create(Context& context, const XnChar* strName = NULL); 08188 08196 XnStatus CreateBasedOn(AudioGenerator& other, const XnChar* strName = NULL); 08197 08202 inline XnStatus SetData(XnUInt32 nFrameID, XnUInt64 nTimestamp, XnUInt32 nDataSize, const XnUInt8* pAudioBuffer) 08203 { 08204 return xnMockAudioSetData(GetHandle(), nFrameID, nTimestamp, nDataSize, pAudioBuffer); 08205 } 08206 08215 inline XnStatus SetData(const AudioMetaData& audioMD, XnUInt32 nFrameID, XnUInt64 nTimestamp) 08216 { 08217 return SetData(nFrameID, nTimestamp, audioMD.DataSize(), audioMD.Data()); 08218 } 08219 08225 inline XnStatus SetData(const AudioMetaData& audioMD) 08226 { 08227 return SetData(audioMD, audioMD.FrameID(), audioMD.Timestamp()); 08228 } 08229 }; 08230 08234 class MockRawGenerator : public Generator 08235 { 08236 public: 08237 MockRawGenerator(XnNodeHandle hNode = NULL) : Generator(hNode) {} 08238 MockRawGenerator(const NodeWrapper& other) : Generator(other) {} 08239 08240 inline XnStatus Create(Context& context, const XnChar* strName = NULL); 08241 08242 inline XnStatus SetData(XnUInt32 nFrameID, XnUInt64 nTimestamp, XnUInt32 nDataSize, const void* pData) 08243 { 08244 return xnMockRawSetData(GetHandle(), nFrameID, nTimestamp, nDataSize, pData); 08245 } 08246 08247 }; 08248 08253 class Codec : public ProductionNode 08254 { 08255 public: 08261 inline Codec(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 08262 inline Codec(const NodeWrapper& other) : ProductionNode(other) {} 08263 08268 inline XnStatus Create(Context& context, XnCodecID codecID, ProductionNode& initializerNode); 08269 08274 inline XnCodecID GetCodecID() const 08275 { 08276 return xnGetCodecID(GetHandle()); 08277 } 08278 08283 inline XnStatus EncodeData(const void* pSrc, XnUInt32 nSrcSize, void* pDst, XnUInt32 nDstSize, XnUInt* pnBytesWritten) const 08284 { 08285 return xnEncodeData(GetHandle(), pSrc, nSrcSize, pDst, nDstSize, pnBytesWritten); 08286 } 08287 08292 inline XnStatus DecodeData(const void* pSrc, XnUInt32 nSrcSize, void* pDst, XnUInt32 nDstSize, XnUInt* pnBytesWritten) const 08293 { 08294 return xnDecodeData(GetHandle(), pSrc, nSrcSize, pDst, nDstSize, pnBytesWritten); 08295 } 08296 }; 08297 08331 class ScriptNode : public ProductionNode 08332 { 08333 public: 08339 inline ScriptNode(XnNodeHandle hNode = NULL) : ProductionNode(hNode) {} 08340 inline ScriptNode(const NodeWrapper& other) : ProductionNode(other) {} 08341 08342 inline XnStatus Create(Context& context, const XnChar* strFormat); 08343 08344 inline const XnChar* GetSupportedFormat() 08345 { 08346 return xnScriptNodeGetSupportedFormat(GetHandle()); 08347 } 08348 08354 inline XnStatus LoadScriptFromFile(const XnChar* strFileName) 08355 { 08356 return xnLoadScriptFromFile(GetHandle(), strFileName); 08357 } 08358 08364 inline XnStatus LoadScriptFromString(const XnChar* strScript) 08365 { 08366 return xnLoadScriptFromString(GetHandle(), strScript); 08367 } 08368 08380 inline XnStatus Run(EnumerationErrors* pErrors); 08381 }; 08382 08383 //--------------------------------------------------------------------------- 08384 // EnumerationErrors 08385 //--------------------------------------------------------------------------- 08406 class EnumerationErrors 08407 { 08408 public: 08412 inline EnumerationErrors() : m_bAllocated(TRUE), m_pErrors(NULL) { xnEnumerationErrorsAllocate(&m_pErrors); } 08413 08421 inline EnumerationErrors(XnEnumerationErrors* pErrors, XnBool bOwn = FALSE) : m_bAllocated(bOwn), m_pErrors(pErrors) {} 08422 08426 ~EnumerationErrors() { Free(); } 08427 08431 class Iterator 08432 { 08433 public: 08434 friend class EnumerationErrors; 08435 08441 XnBool operator==(const Iterator& other) const 08442 { 08443 return m_it == other.m_it; 08444 } 08445 08451 XnBool operator!=(const Iterator& other) const 08452 { 08453 return m_it != other.m_it; 08454 } 08455 08460 inline Iterator& operator++() 08461 { 08462 m_it = xnEnumerationErrorsGetNext(m_it); 08463 return *this; 08464 } 08465 08470 inline Iterator operator++(int) 08471 { 08472 return Iterator(xnEnumerationErrorsGetNext(m_it)); 08473 } 08474 08483 inline const XnProductionNodeDescription& Description() { return *xnEnumerationErrorsGetCurrentDescription(m_it); } 08484 08489 inline XnStatus Error() { return xnEnumerationErrorsGetCurrentError(m_it); } 08490 08491 private: 08492 inline Iterator(XnEnumerationErrorsIterator it) : m_it(it) {} 08493 08494 XnEnumerationErrorsIterator m_it; 08495 }; 08496 08505 inline Iterator Begin() const { return Iterator(xnEnumerationErrorsGetFirst(m_pErrors)); } 08506 08515 inline Iterator End() const { return Iterator(NULL); } 08516 08525 inline XnStatus ToString(XnChar* csBuffer, XnUInt32 nSize) 08526 { 08527 return xnEnumerationErrorsToString(m_pErrors, csBuffer, nSize); 08528 } 08529 08534 inline void Free() 08535 { 08536 if (m_bAllocated) 08537 { 08538 xnEnumerationErrorsFree(m_pErrors); 08539 m_pErrors = NULL; 08540 m_bAllocated = FALSE; 08541 } 08542 } 08543 08547 inline XnEnumerationErrors* GetUnderlying() { return m_pErrors; } 08548 08549 private: 08550 XnEnumerationErrors* m_pErrors; 08551 XnBool m_bAllocated; 08552 }; 08553 08554 //--------------------------------------------------------------------------- 08555 // Context 08556 //--------------------------------------------------------------------------- 08557 08593 class Context 08594 { 08595 public: 08610 typedef void (XN_CALLBACK_TYPE* NodeCreationHandler)(Context& context, ProductionNode& createdNode, void* pCookie); 08611 08626 typedef void (XN_CALLBACK_TYPE* NodeDestructionHandler)(Context& context, const XnChar* strDestroyedNodeName, void* pCookie); 08627 08631 inline Context() : m_pContext(NULL), m_bUsingDeprecatedAPI(FALSE), m_bAllocated(FALSE), m_hShuttingDownCallback(NULL) {} 08632 08638 inline Context(XnContext* pContext) : m_pContext(NULL), m_bUsingDeprecatedAPI(FALSE), m_bAllocated(FALSE), m_hShuttingDownCallback(NULL) 08639 { 08640 SetHandle(pContext); 08641 } 08642 08649 inline Context(const Context& other) : m_pContext(NULL), m_bUsingDeprecatedAPI(FALSE), m_bAllocated(FALSE), m_hShuttingDownCallback(NULL) 08650 { 08651 SetHandle(other.m_pContext); 08652 } 08653 08657 ~Context() 08658 { 08659 SetHandle(NULL); 08660 } 08661 08662 inline Context& operator=(const Context& other) 08663 { 08664 SetHandle(other.m_pContext); 08665 return *this; 08666 } 08667 08671 inline XnContext* GetUnderlyingObject() const { return m_pContext; } 08672 08678 inline XnBool operator==(const Context& other) 08679 { 08680 return (GetUnderlyingObject() == other.GetUnderlyingObject()); 08681 } 08682 08688 inline XnBool operator!=(const Context& other) 08689 { 08690 return (GetUnderlyingObject() != other.GetUnderlyingObject()); 08691 } 08692 08707 inline XnStatus Init() 08708 { 08709 XnContext* pContext = NULL; 08710 XnStatus nRetVal = xnInit(&pContext); 08711 XN_IS_STATUS_OK(nRetVal); 08712 08713 TakeOwnership(pContext); 08714 m_bAllocated = TRUE; 08715 08716 return (XN_STATUS_OK); 08717 } 08718 08734 inline XnStatus RunXmlScript(const XnChar* strScript, ScriptNode& scriptNode, EnumerationErrors* pErrors = NULL) 08735 { 08736 XnStatus nRetVal = XN_STATUS_OK; 08737 08738 XnNodeHandle hScriptNode; 08739 nRetVal = xnContextRunXmlScriptEx(m_pContext, strScript, pErrors == NULL ? NULL : pErrors->GetUnderlying(), &hScriptNode); 08740 XN_IS_STATUS_OK(nRetVal); 08741 08742 scriptNode.TakeOwnership(hScriptNode); 08743 08744 return (XN_STATUS_OK); 08745 } 08746 08762 inline XnStatus RunXmlScriptFromFile(const XnChar* strFileName, ScriptNode& scriptNode, EnumerationErrors* pErrors = NULL) 08763 { 08764 XnStatus nRetVal = XN_STATUS_OK; 08765 08766 XnNodeHandle hScriptNode; 08767 nRetVal = xnContextRunXmlScriptFromFileEx(m_pContext, strFileName, pErrors == NULL ? NULL : pErrors->GetUnderlying(), &hScriptNode); 08768 XN_IS_STATUS_OK(nRetVal); 08769 08770 scriptNode.TakeOwnership(hScriptNode); 08771 08772 return (XN_STATUS_OK); 08773 } 08774 08790 inline XnStatus InitFromXmlFile(const XnChar* strFileName, ScriptNode& scriptNode, EnumerationErrors* pErrors = NULL) 08791 { 08792 XnContext* pContext = NULL; 08793 08794 XnNodeHandle hScriptNode; 08795 XnStatus nRetVal = xnInitFromXmlFileEx(strFileName, &pContext, pErrors == NULL ? NULL : pErrors->GetUnderlying(), &hScriptNode); 08796 XN_IS_STATUS_OK(nRetVal); 08797 08798 scriptNode.TakeOwnership(hScriptNode); 08799 TakeOwnership(pContext); 08800 m_bAllocated = TRUE; 08801 08802 return (XN_STATUS_OK); 08803 } 08804 08821 inline XnStatus OpenFileRecording(const XnChar* strFileName, ProductionNode& playerNode) 08822 { 08823 XnStatus nRetVal = XN_STATUS_OK; 08824 08825 XnNodeHandle hPlayer; 08826 nRetVal = xnContextOpenFileRecordingEx(m_pContext, strFileName, &hPlayer); 08827 XN_IS_STATUS_OK(nRetVal); 08828 08829 playerNode.TakeOwnership(hPlayer); 08830 08831 return (XN_STATUS_OK); 08832 } 08833 08838 inline XnStatus CreateMockNode(XnProductionNodeType type, const XnChar* strName, ProductionNode& mockNode) 08839 { 08840 XnStatus nRetVal = XN_STATUS_OK; 08841 08842 XnNodeHandle hMockNode; 08843 nRetVal = xnCreateMockNode(m_pContext, type, strName, &hMockNode); 08844 XN_IS_STATUS_OK(nRetVal); 08845 08846 mockNode.TakeOwnership(hMockNode); 08847 08848 return (XN_STATUS_OK); 08849 } 08850 08855 inline XnStatus CreateMockNodeBasedOn(ProductionNode& originalNode, const XnChar* strName, ProductionNode& mockNode) 08856 { 08857 XnStatus nRetVal = XN_STATUS_OK; 08858 08859 XnNodeHandle hMockNode; 08860 nRetVal = xnCreateMockNodeBasedOn(m_pContext, originalNode, strName, &hMockNode); 08861 XN_IS_STATUS_OK(nRetVal); 08862 08863 mockNode.TakeOwnership(hMockNode); 08864 08865 return (XN_STATUS_OK); 08866 } 08867 08872 inline XnStatus CreateCodec(XnCodecID codecID, ProductionNode& initializerNode, Codec& codec) 08873 { 08874 XnStatus nRetVal = XN_STATUS_OK; 08875 08876 XnNodeHandle hCodec; 08877 nRetVal = xnCreateCodec(m_pContext, codecID, initializerNode.GetHandle(), &hCodec); 08878 XN_IS_STATUS_OK(nRetVal); 08879 08880 codec.TakeOwnership(hCodec); 08881 08882 return (XN_STATUS_OK); 08883 } 08884 08889 inline XnStatus AddRef() 08890 { 08891 return xnContextAddRef(m_pContext); 08892 } 08893 08898 inline void Release() 08899 { 08900 SetHandle(NULL); 08901 } 08902 08903 08908 inline XnStatus AddLicense(const XnLicense& License) 08909 { 08910 return xnAddLicense(m_pContext, &License); 08911 } 08912 08917 inline XnStatus EnumerateLicenses(XnLicense*& aLicenses, XnUInt32& nCount) const 08918 { 08919 return xnEnumerateLicenses(m_pContext, &aLicenses, &nCount); 08920 } 08921 08926 inline static void FreeLicensesList(XnLicense aLicenses[]) 08927 { 08928 xnFreeLicensesList(aLicenses); 08929 } 08930 08951 XnStatus EnumerateProductionTrees(XnProductionNodeType Type, const Query* pQuery, NodeInfoList& TreesList, EnumerationErrors* pErrors = NULL) const 08952 { 08953 XnStatus nRetVal = XN_STATUS_OK; 08954 08955 const XnNodeQuery* pInternalQuery = (pQuery != NULL) ? pQuery->GetUnderlyingObject() : NULL; 08956 08957 XnNodeInfoList* pList = NULL; 08958 nRetVal = xnEnumerateProductionTrees(m_pContext, Type, pInternalQuery, &pList, pErrors == NULL ? NULL : pErrors->GetUnderlying()); 08959 XN_IS_STATUS_OK(nRetVal); 08960 08961 TreesList.ReplaceUnderlyingObject(pList); 08962 08963 return (XN_STATUS_OK); 08964 } 08965 08998 XnStatus CreateAnyProductionTree(XnProductionNodeType type, Query* pQuery, ProductionNode& node, EnumerationErrors* pErrors = NULL) 08999 { 09000 XnStatus nRetVal = XN_STATUS_OK; 09001 09002 XnNodeQuery* pInternalQuery = (pQuery != NULL) ? pQuery->GetUnderlyingObject() : NULL; 09003 09004 XnNodeHandle hNode; 09005 nRetVal = xnCreateAnyProductionTree(m_pContext, type, pInternalQuery, &hNode, pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09006 XN_IS_STATUS_OK(nRetVal); 09007 09008 node.TakeOwnership(hNode); 09009 09010 return (XN_STATUS_OK); 09011 } 09012 09029 XnStatus CreateProductionTree(NodeInfo& Tree, ProductionNode& node) 09030 { 09031 XnStatus nRetVal = XN_STATUS_OK; 09032 09033 XnNodeHandle hNode; 09034 nRetVal = xnCreateProductionTree(m_pContext, Tree, &hNode); 09035 XN_IS_STATUS_OK(nRetVal); 09036 09037 node.TakeOwnership(hNode); 09038 09039 return (XN_STATUS_OK); 09040 } 09041 09050 XnStatus EnumerateExistingNodes(NodeInfoList& list) const 09051 { 09052 XnNodeInfoList* pList; 09053 XnStatus nRetVal = xnEnumerateExistingNodes(m_pContext, &pList); 09054 XN_IS_STATUS_OK(nRetVal); 09055 09056 list.ReplaceUnderlyingObject(pList); 09057 09058 return (XN_STATUS_OK); 09059 } 09060 09079 XnStatus EnumerateExistingNodes(NodeInfoList& list, XnProductionNodeType type) const 09080 { 09081 XnNodeInfoList* pList; 09082 XnStatus nRetVal = xnEnumerateExistingNodesByType(m_pContext, type, &pList); 09083 XN_IS_STATUS_OK(nRetVal); 09084 09085 list.ReplaceUnderlyingObject(pList); 09086 09087 return (XN_STATUS_OK); 09088 } 09089 09108 XnStatus FindExistingNode(XnProductionNodeType type, ProductionNode& node) const 09109 { 09110 XnStatus nRetVal = XN_STATUS_OK; 09111 09112 XnNodeHandle hNode; 09113 nRetVal = xnFindExistingRefNodeByType(m_pContext, type, &hNode); 09114 XN_IS_STATUS_OK(nRetVal); 09115 09116 node.TakeOwnership(hNode); 09117 09118 return (XN_STATUS_OK); 09119 } 09120 09125 XnStatus GetProductionNodeByName(const XnChar* strInstanceName, ProductionNode& node) const 09126 { 09127 XnStatus nRetVal = XN_STATUS_OK; 09128 09129 XnNodeHandle hNode; 09130 nRetVal = xnGetRefNodeHandleByName(m_pContext, strInstanceName, &hNode); 09131 XN_IS_STATUS_OK(nRetVal); 09132 09133 node.TakeOwnership(hNode); 09134 09135 return (XN_STATUS_OK); 09136 } 09137 09142 XnStatus GetProductionNodeInfoByName(const XnChar* strInstanceName, NodeInfo& nodeInfo) const 09143 { 09144 XnStatus nRetVal = XN_STATUS_OK; 09145 09146 XnNodeHandle hNode; 09147 nRetVal = xnGetRefNodeHandleByName(m_pContext, strInstanceName, &hNode); 09148 XN_IS_STATUS_OK(nRetVal); 09149 09150 xnProductionNodeRelease(hNode); 09151 09152 nodeInfo = NodeInfo(xnGetNodeInfo(hNode)); 09153 09154 return (XN_STATUS_OK); 09155 } 09156 09161 inline XnStatus StartGeneratingAll() 09162 { 09163 return xnStartGeneratingAll(m_pContext); 09164 } 09165 09169 inline XnStatus StopGeneratingAll() 09170 { 09171 return xnStopGeneratingAll(m_pContext); 09172 } 09173 09183 inline XnStatus SetGlobalMirror(XnBool bMirror) 09184 { 09185 return xnSetGlobalMirror(m_pContext, bMirror); 09186 } 09187 09192 inline XnBool GetGlobalMirror() 09193 { 09194 return xnGetGlobalMirror(m_pContext); 09195 } 09196 09201 inline XnStatus GetGlobalErrorState() 09202 { 09203 return xnGetGlobalErrorState(m_pContext); 09204 } 09205 09215 inline XnStatus RegisterToErrorStateChange(XnErrorStateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 09216 { 09217 return xnRegisterToGlobalErrorStateChange(m_pContext, handler, pCookie, &hCallback); 09218 } 09219 09227 inline void UnregisterFromErrorStateChange(XnCallbackHandle hCallback) 09228 { 09229 xnUnregisterFromGlobalErrorStateChange(m_pContext, hCallback); 09230 } 09231 09241 inline XnStatus RegisterToNodeCreation(NodeCreationHandler handler, void* pCookie, XnCallbackHandle& hCallback) 09242 { 09243 XnStatus nRetVal = XN_STATUS_OK; 09244 09245 NodeCreationCookie* pCreationCookie; 09246 XN_VALIDATE_ALLOC(pCreationCookie, NodeCreationCookie); 09247 pCreationCookie->pFunc = handler; 09248 pCreationCookie->pCookie = pCookie; 09249 09250 nRetVal = xnRegisterToNodeCreation(m_pContext, NodeCreationCallback, pCreationCookie, &pCreationCookie->hUnderlyingCallback); 09251 XN_IS_STATUS_OK(nRetVal); 09252 09253 hCallback = pCreationCookie; 09254 09255 return XN_STATUS_OK; 09256 } 09257 09265 inline void UnregisterFromNodeCreation(XnCallbackHandle hCallback) 09266 { 09267 NodeCreationCookie* pCreationCookie = (NodeCreationCookie*)hCallback; 09268 xnUnregisterFromNodeCreation(m_pContext, pCreationCookie->hUnderlyingCallback); 09269 xnOSFree(pCreationCookie); 09270 } 09271 09281 inline XnStatus RegisterToNodeDestruction(NodeDestructionHandler handler, void* pCookie, XnCallbackHandle& hCallback) 09282 { 09283 XnStatus nRetVal = XN_STATUS_OK; 09284 09285 NodeDestructionCookie* pDestructionCookie; 09286 XN_VALIDATE_ALLOC(pDestructionCookie, NodeDestructionCookie); 09287 pDestructionCookie->pFunc = handler; 09288 pDestructionCookie->pCookie = pCookie; 09289 09290 nRetVal = xnRegisterToNodeDestruction(m_pContext, NodeDestructionCallback, pDestructionCookie, &pDestructionCookie->hUnderlyingCallback); 09291 XN_IS_STATUS_OK(nRetVal); 09292 09293 hCallback = pDestructionCookie; 09294 09295 return XN_STATUS_OK; 09296 } 09297 09305 inline void UnregisterFromNodeDestruction(XnCallbackHandle hCallback) 09306 { 09307 NodeDestructionCookie* pDestructionCookie = (NodeDestructionCookie*)hCallback; 09308 xnUnregisterFromNodeDestruction(m_pContext, pDestructionCookie->hUnderlyingCallback); 09309 xnOSFree(pDestructionCookie); 09310 } 09311 09345 inline XnStatus WaitAndUpdateAll() 09346 { 09347 return xnWaitAndUpdateAll(m_pContext); 09348 } 09349 09384 inline XnStatus WaitAnyUpdateAll() 09385 { 09386 return xnWaitAnyUpdateAll(m_pContext); 09387 } 09388 09419 inline XnStatus WaitOneUpdateAll(ProductionNode& node) 09420 { 09421 return xnWaitOneUpdateAll(m_pContext, node.GetHandle()); 09422 } 09423 09445 inline XnStatus WaitNoneUpdateAll() 09446 { 09447 return xnWaitNoneUpdateAll(m_pContext); 09448 } 09449 09454 inline XnStatus AutoEnumerateOverSingleInput(NodeInfoList& List, XnProductionNodeDescription& description, const XnChar* strCreationInfo, XnProductionNodeType InputType, EnumerationErrors* pErrors, Query* pQuery = NULL) const 09455 { 09456 return xnAutoEnumerateOverSingleInput(m_pContext, List.GetUnderlyingObject(), &description, strCreationInfo, InputType, pErrors == NULL ? NULL : pErrors->GetUnderlying(), pQuery == NULL ? NULL : pQuery->GetUnderlyingObject()); 09457 } 09458 09462 inline void SetHandle(XnContext* pContext) 09463 { 09464 if (m_pContext == pContext) 09465 { 09466 return; 09467 } 09468 09469 if (m_pContext != NULL) 09470 { 09471 if (m_bUsingDeprecatedAPI && m_bAllocated) 09472 { 09473 // Backwards compatibility: call shutdown instead of release, to make old programs get the 09474 // exact same behavior they used to have. 09475 xnForceShutdown(m_pContext); 09476 } 09477 else 09478 { 09479 xnContextUnregisterFromShutdown(m_pContext, m_hShuttingDownCallback); 09480 xnContextRelease(m_pContext); 09481 } 09482 } 09483 09484 if (pContext != NULL) 09485 { 09486 XnStatus nRetVal = xnContextAddRef(pContext); 09487 XN_ASSERT(nRetVal == XN_STATUS_OK); 09488 XN_REFERENCE_VARIABLE(nRetVal); 09489 09490 nRetVal = xnContextRegisterForShutdown(pContext, ContextShuttingDownCallback, this, &m_hShuttingDownCallback); 09491 XN_ASSERT(nRetVal == XN_STATUS_OK); 09492 } 09493 09494 m_pContext = pContext; 09495 } 09496 09497 inline void TakeOwnership(XnContext* pContext) 09498 { 09499 SetHandle(pContext); 09500 09501 if (pContext != NULL) 09502 { 09503 xnContextRelease(pContext); 09504 } 09505 } 09506 09508 inline XnStatus XN_API_DEPRECATED("Use other overload!") RunXmlScript(const XnChar* strScript, EnumerationErrors* pErrors = NULL) 09509 { 09510 m_bUsingDeprecatedAPI = TRUE; 09511 #pragma warning (push) 09512 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 09513 return xnContextRunXmlScript(m_pContext, strScript, pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09514 #pragma warning (pop) 09515 } 09516 09517 inline XnStatus XN_API_DEPRECATED("Use other overload!") RunXmlScriptFromFile(const XnChar* strFileName, EnumerationErrors* pErrors = NULL) 09518 { 09519 m_bUsingDeprecatedAPI = TRUE; 09520 #pragma warning (push) 09521 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 09522 return xnContextRunXmlScriptFromFile(m_pContext, strFileName, pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09523 #pragma warning (pop) 09524 } 09525 09526 inline XnStatus XN_API_DEPRECATED("Use other overload!") InitFromXmlFile(const XnChar* strFileName, EnumerationErrors* pErrors = NULL) 09527 { 09528 XnContext* pContext = NULL; 09529 m_bUsingDeprecatedAPI = TRUE; 09530 09531 #pragma warning (push) 09532 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 09533 XnStatus nRetVal = xnInitFromXmlFile(strFileName, &pContext, pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09534 #pragma warning (pop) 09535 XN_IS_STATUS_OK(nRetVal); 09536 09537 TakeOwnership(pContext); 09538 m_bAllocated = TRUE; 09539 09540 return (XN_STATUS_OK); 09541 } 09542 09543 inline XnStatus XN_API_DEPRECATED("Use other overload!") OpenFileRecording(const XnChar* strFileName) 09544 { 09545 m_bUsingDeprecatedAPI = TRUE; 09546 #pragma warning (push) 09547 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 09548 return xnContextOpenFileRecording(m_pContext, strFileName); 09549 #pragma warning (pop) 09550 } 09551 09552 inline void XN_API_DEPRECATED("You may use Release() instead, or count on dtor") Shutdown() 09553 { 09554 if (m_pContext != NULL) 09555 { 09556 #pragma warning (push) 09557 #pragma warning (disable: XN_DEPRECATED_WARNING_IDS) 09558 xnShutdown(m_pContext); 09559 #pragma warning (pop) 09560 m_pContext = NULL; 09561 } 09562 } 09563 09564 XnStatus XN_API_DEPRECATED("Please use other overload") CreateProductionTree(NodeInfo& Tree) 09565 { 09566 XnStatus nRetVal = XN_STATUS_OK; 09567 09568 XnNodeHandle hNode; 09569 nRetVal = xnCreateProductionTree(m_pContext, Tree, &hNode); 09570 XN_IS_STATUS_OK(nRetVal); 09571 09572 Tree.m_bOwnerOfNode = TRUE; 09573 09574 return (XN_STATUS_OK); 09575 } 09578 private: 09579 typedef struct NodeCreationCookie 09580 { 09581 NodeCreationHandler pFunc; 09582 void* pCookie; 09583 XnCallbackHandle hUnderlyingCallback; 09584 } NodeCreationCookie; 09585 09586 typedef struct NodeDestructionCookie 09587 { 09588 NodeDestructionHandler pFunc; 09589 void* pCookie; 09590 XnCallbackHandle hUnderlyingCallback; 09591 } NodeDestructionCookie; 09592 09593 static void XN_CALLBACK_TYPE NodeCreationCallback(XnContext* pContext, XnNodeHandle hCreatedNode, void* pCookie) 09594 { 09595 NodeCreationCookie* pNodeCreationCookie = (NodeCreationCookie*)pCookie; 09596 Context context(pContext); 09597 ProductionNode createdNode(hCreatedNode); 09598 pNodeCreationCookie->pFunc(context, createdNode, pNodeCreationCookie->pCookie); 09599 } 09600 09601 static void XN_CALLBACK_TYPE NodeDestructionCallback(XnContext* pContext, const XnChar* strDestroyedNodeName, void* pCookie) 09602 { 09603 NodeDestructionCookie* pNodeCreationCookie = (NodeDestructionCookie*)pCookie; 09604 Context context(pContext); 09605 pNodeCreationCookie->pFunc(context, strDestroyedNodeName, pNodeCreationCookie->pCookie); 09606 } 09607 09608 static void XN_CALLBACK_TYPE ContextShuttingDownCallback(XnContext* /*pContext*/, void* pCookie) 09609 { 09610 Context* pThis = (Context*)pCookie; 09611 pThis->m_pContext = NULL; 09612 } 09613 09614 XnContext* m_pContext; 09615 XnBool m_bUsingDeprecatedAPI; 09616 XnBool m_bAllocated; 09617 XnCallbackHandle m_hShuttingDownCallback; 09618 }; 09619 09624 class Resolution 09625 { 09626 public: 09632 inline Resolution(XnResolution res) : m_Res(res) 09633 { 09634 m_nXRes = xnResolutionGetXRes(res); 09635 m_nYRes = xnResolutionGetYRes(res); 09636 m_strName = xnResolutionGetName(res); 09637 } 09638 09645 inline Resolution(XnUInt32 xRes, XnUInt32 yRes) : m_nXRes(xRes), m_nYRes(yRes) 09646 { 09647 m_Res = xnResolutionGetFromXYRes(xRes, yRes); 09648 m_strName = xnResolutionGetName(m_Res); 09649 } 09650 09656 inline Resolution(const XnChar* strName) 09657 { 09658 m_Res = xnResolutionGetFromName(strName); 09659 m_nXRes = xnResolutionGetXRes(m_Res); 09660 m_nYRes = xnResolutionGetYRes(m_Res); 09661 m_strName = xnResolutionGetName(m_Res); 09662 } 09663 09667 inline XnResolution GetResolution() const { return m_Res; } 09671 inline XnUInt32 GetXResolution() const { return m_nXRes; } 09675 inline XnUInt32 GetYResolution() const { return m_nYRes; } 09679 inline const XnChar* GetName() const { return m_strName; } 09680 09681 private: 09682 XnResolution m_Res; 09683 XnUInt32 m_nXRes; 09684 XnUInt32 m_nYRes; 09685 const XnChar* m_strName; 09686 }; 09687 09688 //--------------------------------------------------------------------------- 09689 // Functions Implementation 09690 //--------------------------------------------------------------------------- 09691 inline XnStatus NodeInfoList::FilterList(Context& context, Query& query) 09692 { 09693 return xnNodeQueryFilterList(context.GetUnderlyingObject(), query.GetUnderlyingObject(), m_pList); 09694 } 09695 09696 inline void ProductionNode::GetContext(Context& context) const 09697 { 09698 context.TakeOwnership(xnGetRefContextFromNodeHandle(GetHandle())); 09699 } 09700 09701 inline Context ProductionNode::GetContext() const 09702 { 09703 XnContext* pContext = xnGetRefContextFromNodeHandle(GetHandle()); 09704 Context result(pContext); 09705 xnContextRelease(pContext); 09706 return result; 09707 } 09708 09709 inline NodeInfoList& NodeInfo::GetNeededNodes() const 09710 { 09711 if (m_pNeededNodes == NULL) 09712 { 09713 XnNodeInfoList* pList = xnNodeInfoGetNeededNodes(m_pInfo); 09714 m_pNeededNodes = XN_NEW(NodeInfoList, pList); 09715 } 09716 09717 return *m_pNeededNodes; 09718 } 09719 09720 inline void NodeInfo::SetUnderlyingObject(XnNodeInfo* pInfo) 09721 { 09722 if (m_pNeededNodes != NULL) 09723 { 09724 XN_DELETE(m_pNeededNodes); 09725 } 09726 09727 m_bOwnerOfNode = FALSE; 09728 m_pInfo = pInfo; 09729 m_pNeededNodes = NULL; 09730 } 09731 09732 inline XnBool FrameSyncCapability::CanFrameSyncWith(Generator& other) const 09733 { 09734 return xnCanFrameSyncWith(GetHandle(), other.GetHandle()); 09735 } 09736 09737 inline XnStatus FrameSyncCapability::FrameSyncWith(Generator& other) 09738 { 09739 return xnFrameSyncWith(GetHandle(), other.GetHandle()); 09740 } 09741 09742 inline XnStatus FrameSyncCapability::StopFrameSyncWith(Generator& other) 09743 { 09744 return xnStopFrameSyncWith(GetHandle(), other.GetHandle()); 09745 } 09746 09747 inline XnBool FrameSyncCapability::IsFrameSyncedWith(Generator& other) const 09748 { 09749 return xnIsFrameSyncedWith(GetHandle(), other.GetHandle()); 09750 } 09751 09752 inline XnStatus NodeInfo::GetInstance(ProductionNode& node) const 09753 { 09754 if (m_pInfo == NULL) 09755 { 09756 return XN_STATUS_INVALID_OPERATION; 09757 } 09758 09759 XnNodeHandle hNode = xnNodeInfoGetRefHandle(m_pInfo); 09760 node.TakeOwnership(hNode); 09761 09762 if (m_bOwnerOfNode) 09763 { 09764 xnProductionNodeRelease(hNode); 09765 } 09766 09767 return (XN_STATUS_OK); 09768 } 09769 09770 //--------------------------------------------------------------------------- 09771 // Node creation functions 09772 //--------------------------------------------------------------------------- 09773 09774 inline XnStatus Device::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09775 { 09776 XnNodeHandle hNode; 09777 XnStatus nRetVal = xnCreateDevice(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09778 XN_IS_STATUS_OK(nRetVal); 09779 TakeOwnership(hNode); 09780 return (XN_STATUS_OK); 09781 } 09782 09783 inline XnStatus Recorder::Create(Context& context, const XnChar* strFormatName /*= NULL*/) 09784 { 09785 XnNodeHandle hNode; 09786 XnStatus nRetVal = xnCreateRecorder(context.GetUnderlyingObject(), strFormatName, &hNode); 09787 XN_IS_STATUS_OK(nRetVal); 09788 TakeOwnership(hNode); 09789 return (XN_STATUS_OK); 09790 } 09791 09792 inline XnStatus Player::Create(Context& context, const XnChar* strFormatName) 09793 { 09794 XnNodeHandle hNode; 09795 XnStatus nRetVal = xnCreatePlayer(context.GetUnderlyingObject(), strFormatName, &hNode); 09796 XN_IS_STATUS_OK(nRetVal); 09797 TakeOwnership(hNode); 09798 return (XN_STATUS_OK); 09799 } 09800 09801 inline XnStatus DepthGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09802 { 09803 XnNodeHandle hNode; 09804 XnStatus nRetVal = xnCreateDepthGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09805 XN_IS_STATUS_OK(nRetVal); 09806 TakeOwnership(hNode); 09807 return (XN_STATUS_OK); 09808 } 09809 09810 inline XnStatus MockDepthGenerator::Create(Context& context, const XnChar* strName /* = NULL */) 09811 { 09812 XnNodeHandle hNode; 09813 XnStatus nRetVal = xnCreateMockNode(context.GetUnderlyingObject(), XN_NODE_TYPE_DEPTH, strName, &hNode); 09814 XN_IS_STATUS_OK(nRetVal); 09815 TakeOwnership(hNode); 09816 return (XN_STATUS_OK); 09817 } 09818 09819 inline XnStatus MockDepthGenerator::CreateBasedOn(DepthGenerator& other, const XnChar* strName /* = NULL */) 09820 { 09821 Context context; 09822 other.GetContext(context); 09823 XnNodeHandle hNode; 09824 XnStatus nRetVal = xnCreateMockNodeBasedOn(context.GetUnderlyingObject(), other.GetHandle(), strName, &hNode); 09825 XN_IS_STATUS_OK(nRetVal); 09826 TakeOwnership(hNode); 09827 return (XN_STATUS_OK); 09828 } 09829 09830 inline XnStatus ImageGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09831 { 09832 XnNodeHandle hNode; 09833 XnStatus nRetVal = xnCreateImageGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09834 XN_IS_STATUS_OK(nRetVal); 09835 TakeOwnership(hNode); 09836 return (XN_STATUS_OK); 09837 } 09838 09839 inline XnStatus MockImageGenerator::Create(Context& context, const XnChar* strName /* = NULL */) 09840 { 09841 XnNodeHandle hNode; 09842 XnStatus nRetVal = xnCreateMockNode(context.GetUnderlyingObject(), XN_NODE_TYPE_IMAGE, strName, &hNode); 09843 XN_IS_STATUS_OK(nRetVal); 09844 TakeOwnership(hNode); 09845 return (XN_STATUS_OK); 09846 } 09847 09848 inline XnStatus MockImageGenerator::CreateBasedOn(ImageGenerator& other, const XnChar* strName /* = NULL */) 09849 { 09850 Context context; 09851 other.GetContext(context); 09852 XnNodeHandle hNode; 09853 XnStatus nRetVal = xnCreateMockNodeBasedOn(context.GetUnderlyingObject(), other.GetHandle(), strName, &hNode); 09854 XN_IS_STATUS_OK(nRetVal); 09855 TakeOwnership(hNode); 09856 return (XN_STATUS_OK); 09857 } 09858 09859 inline XnStatus IRGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09860 { 09861 XnNodeHandle hNode; 09862 XnStatus nRetVal = xnCreateIRGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09863 XN_IS_STATUS_OK(nRetVal); 09864 TakeOwnership(hNode); 09865 return (XN_STATUS_OK); 09866 } 09867 09868 inline XnStatus MockIRGenerator::Create(Context& context, const XnChar* strName /* = NULL */) 09869 { 09870 XnNodeHandle hNode; 09871 XnStatus nRetVal = xnCreateMockNode(context.GetUnderlyingObject(), XN_NODE_TYPE_IR, strName, &hNode); 09872 XN_IS_STATUS_OK(nRetVal); 09873 TakeOwnership(hNode); 09874 return (XN_STATUS_OK); 09875 } 09876 09877 inline XnStatus MockIRGenerator::CreateBasedOn(IRGenerator& other, const XnChar* strName /* = NULL */) 09878 { 09879 Context context; 09880 other.GetContext(context); 09881 XnNodeHandle hNode; 09882 XnStatus nRetVal = xnCreateMockNodeBasedOn(context.GetUnderlyingObject(), other.GetHandle(), strName, &hNode); 09883 XN_IS_STATUS_OK(nRetVal); 09884 TakeOwnership(hNode); 09885 return (XN_STATUS_OK); 09886 } 09887 09888 inline XnStatus GestureGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09889 { 09890 XnNodeHandle hNode; 09891 XnStatus nRetVal = xnCreateGestureGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09892 XN_IS_STATUS_OK(nRetVal); 09893 TakeOwnership(hNode); 09894 return (XN_STATUS_OK); 09895 } 09896 09897 inline XnStatus SceneAnalyzer::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09898 { 09899 //You're creating a scene! 09900 XnNodeHandle hNode; 09901 XnStatus nRetVal = xnCreateSceneAnalyzer(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09902 XN_IS_STATUS_OK(nRetVal); 09903 TakeOwnership(hNode); 09904 return (XN_STATUS_OK); 09905 } 09906 09907 inline XnStatus HandsGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09908 { 09909 XnNodeHandle hNode; 09910 XnStatus nRetVal = xnCreateHandsGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09911 XN_IS_STATUS_OK(nRetVal); 09912 TakeOwnership(hNode); 09913 return (XN_STATUS_OK); 09914 } 09915 09916 inline XnStatus UserGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09917 { 09918 XnNodeHandle hNode; 09919 XnStatus nRetVal = xnCreateUserGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09920 XN_IS_STATUS_OK(nRetVal); 09921 TakeOwnership(hNode); 09922 return (XN_STATUS_OK); 09923 } 09924 09925 inline XnStatus AudioGenerator::Create(Context& context, Query* pQuery/*=NULL*/, EnumerationErrors* pErrors/*=NULL*/) 09926 { 09927 XnNodeHandle hNode; 09928 XnStatus nRetVal = xnCreateAudioGenerator(context.GetUnderlyingObject(), &hNode, pQuery == NULL ? NULL : pQuery->GetUnderlyingObject(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09929 XN_IS_STATUS_OK(nRetVal); 09930 TakeOwnership(hNode); 09931 return (XN_STATUS_OK); 09932 } 09933 09934 inline XnStatus MockAudioGenerator::Create(Context& context, const XnChar* strName /* = NULL */) 09935 { 09936 XnNodeHandle hNode; 09937 XnStatus nRetVal = xnCreateMockNode(context.GetUnderlyingObject(), XN_NODE_TYPE_AUDIO, strName, &hNode); 09938 XN_IS_STATUS_OK(nRetVal); 09939 TakeOwnership(hNode); 09940 return (XN_STATUS_OK); 09941 } 09942 09943 inline XnStatus MockAudioGenerator::CreateBasedOn(AudioGenerator& other, const XnChar* strName /* = NULL */) 09944 { 09945 Context context; 09946 other.GetContext(context); 09947 XnNodeHandle hNode; 09948 XnStatus nRetVal = xnCreateMockNodeBasedOn(context.GetUnderlyingObject(), other.GetHandle(), strName, &hNode); 09949 XN_IS_STATUS_OK(nRetVal); 09950 TakeOwnership(hNode); 09951 return (XN_STATUS_OK); 09952 } 09953 09954 inline XnStatus MockRawGenerator::Create(Context& context, const XnChar* strName /*= NULL*/) 09955 { 09956 XnNodeHandle hNode; 09957 XnStatus nRetVal = xnCreateMockNode(context.GetUnderlyingObject(), XN_NODE_TYPE_GENERATOR, strName, &hNode); 09958 XN_IS_STATUS_OK(nRetVal); 09959 TakeOwnership(hNode); 09960 return (XN_STATUS_OK); 09961 } 09962 09963 inline XnStatus Codec::Create(Context& context, XnCodecID codecID, ProductionNode& initializerNode) 09964 { 09965 XnNodeHandle hNode; 09966 XnStatus nRetVal = xnCreateCodec(context.GetUnderlyingObject(), codecID, initializerNode.GetHandle(), &hNode); 09967 XN_IS_STATUS_OK(nRetVal); 09968 TakeOwnership(hNode); 09969 return (XN_STATUS_OK); 09970 } 09971 09972 inline XnStatus ScriptNode::Run(EnumerationErrors* pErrors) 09973 { 09974 return xnScriptNodeRun(GetHandle(), pErrors == NULL ? NULL : pErrors->GetUnderlying()); 09975 } 09976 09977 inline XnStatus ScriptNode::Create(Context& context, const XnChar* strFormat) 09978 { 09979 XnNodeHandle hNode; 09980 XnStatus nRetVal = xnCreateScriptNode(context.GetUnderlyingObject(), strFormat, &hNode); 09981 XN_IS_STATUS_OK(nRetVal); 09982 TakeOwnership(hNode); 09983 return (XN_STATUS_OK); 09984 } 09985 09986 //--------------------------------------------------------------------------- 09987 // Global Helper Functions 09988 //--------------------------------------------------------------------------- 09989 09990 inline void XN_API_DEPRECATED("Use xn::Version::Current() instead") GetVersion(XnVersion& Version) 09991 { 09992 xnGetVersion(&Version); 09993 } 09994 09995 //--------------------------------------------------------------------------- 09996 // Internal Helper Classes and Functions 09997 //--------------------------------------------------------------------------- 09998 09999 class StateChangedCallbackTranslator 10000 { 10001 public: 10002 StateChangedCallbackTranslator(StateChangedHandler handler, void* pCookie) : m_UserHandler(handler), m_pUserCookie(pCookie), m_hCallback(NULL) {} 10003 10004 XnStatus Register(_XnRegisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode) 10005 { 10006 return xnFunc(hNode, StateChangedCallback, this, &m_hCallback); 10007 } 10008 10009 void Unregister(_XnUnregisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode) 10010 { 10011 xnFunc(hNode, m_hCallback); 10012 } 10013 10014 static XnStatus RegisterToUnderlying(_XnRegisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 10015 { 10016 XnStatus nRetVal = XN_STATUS_OK; 10017 10018 StateChangedCallbackTranslator* pTrans; 10019 XN_VALIDATE_NEW(pTrans, StateChangedCallbackTranslator, handler, pCookie); 10020 10021 nRetVal = pTrans->Register(xnFunc, hNode); 10022 if (nRetVal != XN_STATUS_OK) 10023 { 10024 XN_DELETE(pTrans); 10025 return (nRetVal); 10026 } 10027 10028 hCallback = pTrans; 10029 10030 return (XN_STATUS_OK); 10031 } 10032 10033 static XnStatus UnregisterFromUnderlying(_XnUnregisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, XnCallbackHandle hCallback) 10034 { 10035 StateChangedCallbackTranslator* pTrans = (StateChangedCallbackTranslator*)hCallback; 10036 pTrans->Unregister(xnFunc, hNode); 10037 XN_DELETE(pTrans); 10038 return XN_STATUS_OK; 10039 } 10040 10041 private: 10042 friend class GeneralIntCapability; 10043 10044 typedef struct StateChangeCookie 10045 { 10046 StateChangedHandler userHandler; 10047 void* pUserCookie; 10048 XnCallbackHandle hCallback; 10049 } StateChangeCookie; 10050 10051 static void XN_CALLBACK_TYPE StateChangedCallback(XnNodeHandle hNode, void* pCookie) 10052 { 10053 StateChangedCallbackTranslator* pTrans = (StateChangedCallbackTranslator*)pCookie; 10054 ProductionNode node(hNode); 10055 pTrans->m_UserHandler(node, pTrans->m_pUserCookie); 10056 } 10057 10058 StateChangedHandler m_UserHandler; 10059 void* m_pUserCookie; 10060 XnCallbackHandle m_hCallback; 10061 }; 10062 10063 static XnStatus _RegisterToStateChange(_XnRegisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 10064 { 10065 return StateChangedCallbackTranslator::RegisterToUnderlying(xnFunc, hNode, handler, pCookie, hCallback); 10066 } 10067 10068 static void _UnregisterFromStateChange(_XnUnregisterStateChangeFuncPtr xnFunc, XnNodeHandle hNode, XnCallbackHandle hCallback) 10069 { 10070 StateChangedCallbackTranslator::UnregisterFromUnderlying(xnFunc, hNode, hCallback); 10071 } 10072 10073 inline XnStatus GeneralIntCapability::RegisterToValueChange(StateChangedHandler handler, void* pCookie, XnCallbackHandle& hCallback) 10074 { 10075 XnStatus nRetVal = XN_STATUS_OK; 10076 10077 StateChangedCallbackTranslator* pTrans; 10078 XN_VALIDATE_NEW(pTrans, StateChangedCallbackTranslator, handler, pCookie); 10079 10080 nRetVal = xnRegisterToGeneralIntValueChange(GetHandle(), m_strCap, pTrans->StateChangedCallback, pTrans, &pTrans->m_hCallback); 10081 if (nRetVal != XN_STATUS_OK) 10082 { 10083 XN_DELETE(pTrans); 10084 return (nRetVal); 10085 } 10086 10087 hCallback = pTrans; 10088 10089 return (XN_STATUS_OK); 10090 } 10091 10092 inline void GeneralIntCapability::UnregisterFromValueChange(XnCallbackHandle hCallback) 10093 { 10094 StateChangedCallbackTranslator* pTrans = (StateChangedCallbackTranslator*)hCallback; 10095 xnUnregisterFromGeneralIntValueChange(GetHandle(), m_strCap, pTrans->m_hCallback); 10096 XN_DELETE(pTrans); 10097 } 10098 }; 10099 10100 #endif // __XN_CPP_WRAPPER_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
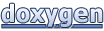