![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
Detailed Description
A context is a workspace where the application builds an OpenNI production graph. It contains methods for managing the production graph.
In order to use any of the OpenNI functionality you must first construct a Context object and initialize it. Prior to this, the application cannot do anything with OpenNI.
For a comprehensive overview to the Context class and its members, see Overview to Contexts.
Event: 'Global Error State Change'
Signals that the error state of any of the nodes has changed.
This event can be used by the application to get a signal as soon as any error occurs in one of the production nodes. The global error state aggregates error state from all the production nodes in the context.
Use RegisterToErrorStateChange() and UnregisterFromErrorStateChange() for using this event.
Event: 'Node Creation'
Signals that a new node was created in the context production graph.
Use RegisterToNodeCreation() and UnregisterFromNodeCreation() for using this event.
Event: 'Node Destruction'
Signals that a node was removed from the context production graph, and destroyed.
Use RegisterToNodeDestruction() and UnregisterFromNodeDestruction() for using this event.
Member Typedef Documentation
typedef void(* xn::Context::NodeCreationHandler)(Context &context, ProductionNode &createdNode, void *pCookie) |
Callback prototype for the 'Node Creation' event handler.
- Parameters:
-
[in] context The context that raised this event. [in] createdNode The id of the hand that disappeared. [in] pCookie A user-provided cookie that was given when registering to this event.
Example for such a handler:
void XN_CALLBACK_TYPE OnNodeCreation(Context& context, ProductionNode& createdNode, void* pCookie) {}
typedef void(* xn::Context::NodeDestructionHandler)(Context &context, const XnChar *strDestroyedNodeName, void *pCookie) |
Callback prototype for the 'Node Destruction' event handler.
- Parameters:
-
[in] context The context that raised this event. [in] strDestroyedNodeName The name of the node that was destroyed. [in] pCookie A user-provided cookie that was given when registering to this event.
Example for such a handler:
void XN_CALLBACK_TYPE OnNodeDestruction(Context& context, const XnChar* strDestroyedNodeName, void* pCookie) {}
Constructor & Destructor Documentation
xn::Context::Context | ( | ) | [inline] |
Ctor
xn::Context::Context | ( | XnContext * | pContext | ) | [inline] |
Ctor
- Parameters:
-
[in] pContext Underlying C object
xn::Context::Context | ( | const Context & | other | ) | [inline] |
Copy Ctor
- Parameters:
-
[in] other Another context. Note that the context will only be destroyed when the original object is destroyed.
xn::Context::~Context | ( | ) | [inline] |
Dtor
Member Function Documentation
XnStatus xn::Context::AddRef | ( | ) | [inline] |
Adds a reference to the context object.
For full details and usage, see xnContextAddRefXnStatus xn::Context::AutoEnumerateOverSingleInput | ( | NodeInfoList & | List, |
XnProductionNodeDescription & | description, | ||
const XnChar * | strCreationInfo, | ||
XnProductionNodeType | InputType, | ||
EnumerationErrors * | pErrors, | ||
Query * | pQuery = NULL |
||
) | const [inline] |
XnStatus xn::Context::CreateAnyProductionTree | ( | XnProductionNodeType | type, |
Query * | pQuery, | ||
ProductionNode & | node, | ||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Enumerates for production nodes of a specific node type, and creates the first production node found of that type.
- Parameters:
-
[in] type Requested node type. [in] pQuery Optional. A query object that can be used to filter results. [out] node Handle to the newly created node. [in,out] pErrors Optional. To contain enumeration errors.
Remarks:
This method is a 'shortcut' version of using the EnumerateProductionTrees() method and then passing the first result to CreateProductionTree(). Thus, this method is exactly like the Create() method for nodes.
Other ways of creating a production graph let the application retrieve a complete list of alternatives and then choose the most appropriate alternative.
This method is the method used by the above XML script methods to create the ProductionNode objects. The XML method runs the XML script, and for each node description the XML method runs this function to create that node.
Using this function is called 'creating a node from the context'. You can also create a node by invoking the Create method of the node itself, e.g., the Create() method of a HandsGenerator node.
By default, this method does not enable the production nodes to start generating data immediately on creation.
- See also:
- Understanding the Create() method for more detail.
XnStatus xn::Context::CreateCodec | ( | XnCodecID | codecID, |
ProductionNode & | initializerNode, | ||
Codec & | codec | ||
) | [inline] |
XnStatus xn::Context::CreateMockNode | ( | XnProductionNodeType | type, |
const XnChar * | strName, | ||
ProductionNode & | mockNode | ||
) | [inline] |
Creates a production node which is only a mock. This node does not represent an actual node, but only keeps a state and implements an interface above it. Mock nodes are useful when simulating nodes for playing recordings, or for use in tests. See also xnCreateMockNodeBasedOn().
For full details and usage, see xnCreateMockNodeXnStatus xn::Context::CreateMockNodeBasedOn | ( | ProductionNode & | originalNode, |
const XnChar * | strName, | ||
ProductionNode & | mockNode | ||
) | [inline] |
Creates a production node which is only a mock, base on the type and properties of another node. This node does not represent an actual node, but only keeps a state and implements an interface above it. Mock nodes are useful when simulating nodes for playing recordings, or for use in tests. See also xnCreateMockNode().
For full details and usage, see xnCreateMockNodeBasedOnXnStatus xn::Context::CreateProductionTree | ( | NodeInfo & | Tree, |
ProductionNode & | node | ||
) | [inline] |
Creates a production node from the information supplied in a xn::NodeInfo object.
- Parameters:
-
[in] Tree Node properties for creating the new node. [out] node Reference to the new xn::ProductionNode object.
Remarks:
The application gets the NodeInfo object by choosing it from the list returned by the xn::Context::EnumerateProductionTrees() method.
If the new production node requires additional nodes for its input, and those nodes do not yet exist, then this method creates those additional nodes also and associates them with the "original" new production node.
XnStatus xn::Context::EnumerateExistingNodes | ( | NodeInfoList & | list | ) | const [inline] |
Returns a list of all the context's existing created nodes.
- Parameters:
-
[out] list List of the context's existing created nodes.
You can use the xn::NodeInfoList::FilterList() method to filter the returned list of existing nodes.
XnStatus xn::Context::EnumerateExistingNodes | ( | NodeInfoList & | list, |
XnProductionNodeType | type | ||
) | const [inline] |
Returns a list of the context's existing created nodes filtered by type.
- Parameters:
-
[in] list List of the context's existing created nodes. [in] type Type to enumerate for.
Remarks
Returns the list after filtering them by type, so it returns a list of the created nodes of the specific type only.
An example of a typical case of returning a list of two nodes of the same type is where there are two sensors in a 3D set-up and you need to retrieve the corresponding two depth nodes to generate data from them.
You can use the xn::NodeInfoList::FilterList() method to filter the returned list of existing nodes.
XnStatus xn::Context::EnumerateLicenses | ( | XnLicense *& | aLicenses, |
XnUInt32 & | nCount | ||
) | const [inline] |
XnStatus xn::Context::EnumerateProductionTrees | ( | XnProductionNodeType | Type, |
const Query * | pQuery, | ||
NodeInfoList & | TreesList, | ||
EnumerationErrors * | pErrors = NULL |
||
) | const [inline] |
Enumerates all available production nodes for a specific node type (e.g., the application wants to create a depth generator node) and returns a full list of matching production nodes.
- Parameters:
-
[in] Type Requested node type. [in] pQuery Optional. A query object that can be used to filter results. [out] TreesList List of possible production nodes. [out] pErrors Optional. To contain enumeration errors.
Remarks:
The application chooses one of the nodes from the node list returned by this method and uses it as input to the xn::Context::CreateProductionTree() method to create a new production node.
Using this method to help create a production node offers the greatest flexibility since it returns a complete list of matching production nodes and so the application can then choose the most suitable node.
By contrast, using CreateAnyProductionTree() or a node's Create() method creates a node from the first matching node that satisfies the query.
XnStatus xn::Context::FindExistingNode | ( | XnProductionNodeType | type, |
ProductionNode & | node | ||
) | const [inline] |
Searches for an existing created node of a specified type and returns a reference to it.
- Parameters:
-
[in] type Type of node to search for. [out] node Reference to an existing created node that was found.
Remarks
This method returns only the first node it finds; this method does not return the entire list of matching nodes. Compare this with the xn::Context::EnumerateExistingNodes() method, which returns the entire list of matching nodes. A typical usage is that the application already created all the nodes from XML. Then, when it needs to start generating data from a particular node, e.g., the skeleton data, it will call FindExistingNode specifying the skeleton type, and it will get it and start using it.
This method does not provide a query parameter (compare with xn::Context::EnumerateExistingNodes).
static void xn::Context::FreeLicensesList | ( | XnLicense | aLicenses[] | ) | [inline, static] |
XnStatus xn::Context::GetGlobalErrorState | ( | ) | [inline] |
Gets the global error state of the context. If one of the nodes in the context is in error state, that state will be returned. If more than one node is in error state, XN_STATUS_MULTIPLE_NODES_ERROR is returned. An application can query each node error state by calling xnGetNodeErrorState().
For full details and usage, see xnGetGlobalErrorStateXnBool xn::Context::GetGlobalMirror | ( | ) | [inline] |
Gets the current state of the GlobalMirror flag.
XnStatus xn::Context::GetProductionNodeByName | ( | const XnChar * | strInstanceName, |
ProductionNode & | node | ||
) | const [inline] |
XnStatus xn::Context::GetProductionNodeInfoByName | ( | const XnChar * | strInstanceName, |
NodeInfo & | nodeInfo | ||
) | const [inline] |
XnContext* xn::Context::GetUnderlyingObject | ( | ) | const [inline] |
Gets the underlying C object
XnStatus xn::Context::Init | ( | ) | [inline] |
Builds the context's general software environment.
This method initializes runtime variables and data structures, and examines all registered plug-ins to learn the purpose and specific capabilities of each. In particular, during initialization the context initialization examines all registered plug-ins to learn the purpose and specific capabilities of each. For example, one plug-in is for creating a skeleton node, and another plug-in is for creating a depth node. Thus an entire database is built of plug-ins that can be queried according to vendor, model, and capabilities of each.
Once you have completed the initialization you can create nodes that are based on the plug-ins that OpenNI has discovered by this initialization process.
XnStatus xn::Context::InitFromXmlFile | ( | const XnChar * | strFileName, |
ScriptNode & | scriptNode, | ||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Shorthand combination of two other initialization methods - Init() and then RunXmlScriptFromFile() - to initialize the context object and then create a production graph.
- Parameters:
-
[in] strFileName Name of file containing an XML script. [out] scriptNode ScriptNode object as the root to all the nodes created from the XML file. When no longer needed, the node can be released, causing all the created nodes to decrement their reference counts. [out] pErrors Optional. To be filled with enumeration errors.
Remarks:
The XML script file describes all the nodes you want to create. For each node description in the XML file, this method creates a xn::ProductionNode object.
XnStatus xn::Context::OpenFileRecording | ( | const XnChar * | strFileName, |
ProductionNode & | playerNode | ||
) | [inline] |
Recreates a production graph from a recorded ONI file and then replays the data generation exactly as it was recorded.
- Parameters:
-
[in] strFileName Name of the recorded file to be run. [out] playerNode Returns a xn::Player object through which playback can be controlled, e.g., seeking and setting playback speed.
Remarks:
OpenNI provides great flexibility of recording. You can decide you want to record only the final output, e.g., just a skeleton in movement; or record both the output skeleton and the depth input; or record only the depth input and then recreate the skeleton at run-time after reading the recorded raw depth data. You can also choose which nodes to record.
XnBool xn::Context::operator!= | ( | const Context & | other | ) | [inline] |
Checks if two context objects are not references to the same context.
- Parameters:
-
[in] other Another object
XnBool xn::Context::operator== | ( | const Context & | other | ) | [inline] |
Checks if two context objects are references to the same context.
- Parameters:
-
[in] other Another object
XnStatus xn::Context::RegisterToErrorStateChange | ( | XnErrorStateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Global Error State Change' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::Context::RegisterToNodeCreation | ( | NodeCreationHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Node Creation' event. see Event: 'Node Creation'.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::Context::RegisterToNodeDestruction | ( | NodeDestructionHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Node Destruction' event. see Event: 'Node Destruction'.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
void xn::Context::Release | ( | ) | [inline] |
Releases a context object, decreasing its ref count by 1. If reference count has reached 0, the context will be destroyed.
For full details and usage, see xnContextReleaseXnStatus xn::Context::RunXmlScript | ( | const XnChar * | strScript, |
ScriptNode & | scriptNode, | ||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Runs an XML script string to build a production graph.
- Parameters:
-
[in] strScript String containing an XML script. [out] scriptNode xn::ScriptNode object as the root to all the nodes created from the XML file. When no longer needed, the node can be released, causing all the created nodes to decrement their reference counts. [in,out] pErrors Optional. To be filled with enumeration errors.
Remarks:
For a full description of the XML structure, see Xml Scripts. Compare this method with initialization methods that run an XML script from a file.
XnStatus xn::Context::RunXmlScriptFromFile | ( | const XnChar * | strFileName, |
ScriptNode & | scriptNode, | ||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Runs an XML script file to build a production graph.
- Parameters:
-
[in] strFileName Name of file containing an XML script. [out] scriptNode scriptNode object as the root to all the nodes created from the XML file. When no longer needed, the node can be released, causing all the created nodes to decrement their reference counts. [in,out] pErrors Optional. To be filled with enumeration errors.
Remarks:
The XML script file describes all the nodes you want to create. For each node description in the XML file, this method creates a xn::ProductionNode object.
XnStatus xn::Context::SetGlobalMirror | ( | XnBool | bMirror | ) | [inline] |
Enables/disables the GlobalMirror flag.
- Parameters:
-
[in] bMirror New mirroring state.
Remarks:
See Global Mirror for understanding of the 'Global Mirror' concept.
void xn::Context::SetHandle | ( | XnContext * | pContext | ) | [inline] |
Replaces the underlying C object pointed to by this object.
XnStatus xn::Context::StartGeneratingAll | ( | ) | [inline] |
Ensures all created generator nodes are generating data.
XnStatus xn::Context::StopGeneratingAll | ( | ) | [inline] |
Ensures all generator nodes are not generating data.
void xn::Context::TakeOwnership | ( | XnContext * | pContext | ) | [inline] |
void xn::Context::UnregisterFromErrorStateChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Global Error State Change' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::Context::UnregisterFromNodeCreation | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Node Creation' event. see Event: 'Node Creation'.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events.
void xn::Context::UnregisterFromNodeDestruction | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Node Destruction' event. see Event: 'Node Destruction'.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events.
XnStatus xn::Context::WaitAndUpdateAll | ( | ) | [inline] |
Updates all generator nodes in the context to their latest available data, first waiting for all nodes to have new data available.
Remarks
This method requests from OpenNI to cause all nodes in the context's Production Graph that have new data available to update their application buffers with the new data, each node updating its own application buffer.
Before OpenNI causes the data updates, it waits until all the nodes have notified that they have new data available. On receiving all the 'new data available' notifications, OpenNI then causes all nodes with new data available to update their application buffers with their new data. At this stage the generator nodes have "generated" new data. This method then stops waiting and returns a success status. The application can now read the newly generated data.
- Note:
- Using this method is not recommended for most applications since it requires all the nodes to have new data. Consider instead using WaitAnyUpdateAll() or WaitOneUpdateAll(). Typical applications use the WaitAnyUpdateAll() method.
An error situation is defined as: after a preset timeout, not all nodes have yet notified OpenNI they have new data available. On error, the method stops waiting and returns an error status. None of the nodes update their application buffers.
See 'Wait and Update' Methods for an overview to the 'WaitXUpdateAll' methods and how to read the data from the nodes.
- Return values:
-
XN_STATUS_WAIT_DATA_TIMEOUT No new data available within 2 seconds.
XnStatus xn::Context::WaitAnyUpdateAll | ( | ) | [inline] |
Updates all generator nodes in the context to their latest available data, first waiting for any of the nodes to have new data available.
- Note:
- Typical applications use this method. Consider using this method for your application.
Remarks
This method requests from OpenNI to cause all nodes with new data available in the context's Production Graph to update their application buffers with the new data, each node updating its own application buffer.
Before OpenNI causes the data updates, it waits for any of the nodes to have notified that it has new data available. On receiving a 'new data available' notification, OpenNI then causes all nodes with new data available to update their application buffers with their new data. At this stage the generator nodes have "generated" new data. This method then stops waiting and returns a success status. The application can now read the newly generated data.
A node that does not have new data available does not update its application buffer.
An error situation is defined as: after a preset timeout, none of the nodes have yet notified OpenNI they have new data available. On error, the method stops waiting and returns an error status. None of the nodes update their application buffers.
See 'Wait and Update' Methods for an overview to the 'WaitXUpdateAll' methods and how to read the data from the nodes.
- Return values:
-
XN_STATUS_WAIT_DATA_TIMEOUT No new data available within 2 seconds.
XnStatus xn::Context::WaitNoneUpdateAll | ( | ) | [inline] |
Immediately updates all generator nodes in the context to their latest available data, without waiting for notification that any node has new data available.
Remarks
This method requests from OpenNI to cause all nodes in the context's Production Graph with new data available to update their application buffers with the new data, each node updating its own application buffer.
OpenNI causes all nodes with new data available to update their application buffers with their new data. At this stage the generator nodes have "generated" new data. This method returns a success status. The application can now read the newly generated data.
A node that does not have new data available does not update its application buffer.
See 'Wait and Update' Methods for an overview to the 'WaitXUpdateAll' methods and how to read the data from the nodes.
XnStatus xn::Context::WaitOneUpdateAll | ( | ProductionNode & | node | ) | [inline] |
Updates all generator nodes in the context to their latest available data, first waiting for a specified node to have new data available.
Remarks
This method requests from OpenNI to cause all nodes in the context's Production Graph with new data available to update their application buffers with the new data, each node updating its own application buffer.
Before OpenNI causes the data updates, it waits for a specified node to notify that it has new data available. On receiving the 'new data available' notification, OpenNI then causes all nodes with new data available to update their application buffers with their new data. At this stage the generator nodes have "generated" new data. This method then stops waiting and returns a success status. The application can now read the newly generated data.
A node that does not have new data available does not update its application buffer.
An error situation is defined as: after a preset timeout, none of the nodes have yet notified OpenNI they have has new data available. On error, the method stops waiting and returns an error status. None of the nodes update their application buffers.
See 'Wait and Update' Methods for an overview to the 'WaitXUpdateAll' methods and how to read the data from the nodes.
- Return values:
-
XN_STATUS_WAIT_DATA_TIMEOUT No new data available within 2 seconds.
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
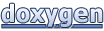