![]() |
OpenNI 1.5.4
|
If you want your new node implementation to support any capabilities, it should both declare that and implement their interfaces.
Declaring the node implementation supported capabilities is done by implementing the IsCapabilitySupported() method. This method only declares if a certain capability is supported or not.
The actual implementation of the capability is done by implementing this capability interface. This implementation can be done in a different class, or in your node implementation class. In any case, your node implementation must implement the proper 'get' mechanism for this interface.
The following table summarizes all capabilities:
For example, let's say your hands generator supports the mirror capability as well as the error state capability. The HandsGenerator can implement the error state capability in the same class and the mirror capability in a different class.
Thus, in the class implementation below, the GetErrorStateInterface()
method returns this
, since the 'Error State Interface' is also implemented by this class. On the other hand, the GetMirrorInterface()
method has to return a reference to m_mirrorCap
, which is an instance of the helper class, MyHandsGeneratorMirror
.
class MyHandsGeneratorMirror : public virtual xn::ModuleMirrorInterface { ... }; class MyHandGenerator: public virtual xn::ModuleHandsGenerator, public virtual xn::ModuleErrorStateInterface { public: ... virtual XnBool IsCapabilitySupported(const XnChar* strCapabilityName) { return ( strcmp(strCapabilityName, XN_CAPABILITY_MIRROR) == 0 || strcmp(strCapabilityName, XN_CAPABILITY_ERROR_STATE) == 0 ); } ... virtual ModuleErrorStateInterface* GetErrorStateInterface() { return this; } ... virtual ModuleMirrorInterface* GetMirrorInterface() { return &m_mirrorCap; } private: MyHandsGeneratorMirror m_mirrorCap; };
Generated on Wed May 16 2012 10:16:06 for OpenNI 1.5.4 by
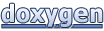