![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
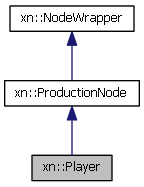
Public Member Functions | |
Player (XnNodeHandle hNode=NULL) | |
Player (const NodeWrapper &other) | |
XnStatus | Create (Context &context, const XnChar *strFormatName) |
XnStatus | SetRepeat (XnBool bRepeat) |
XnStatus | SetSource (XnRecordMedium sourceType, const XnChar *strSource) |
XnStatus | GetSource (XnRecordMedium &sourceType, XnChar *strSource, XnUInt32 nBufSize) const |
XnStatus | ReadNext () |
XnStatus | SeekToTimeStamp (XnInt64 nTimeOffset, XnPlayerSeekOrigin origin) |
XnStatus | SeekToFrame (const XnChar *strNodeName, XnInt32 nFrameOffset, XnPlayerSeekOrigin origin) |
XnStatus | TellTimestamp (XnUInt64 &nTimestamp) const |
XnStatus | TellFrame (const XnChar *strNodeName, XnUInt32 &nFrame) const |
XnStatus | GetNumFrames (const XnChar *strNodeName, XnUInt32 &nFrames) const |
const XnChar * | GetSupportedFormat () const |
XnStatus | EnumerateNodes (NodeInfoList &list) const |
XnBool | IsEOF () const |
XnStatus | RegisterToEndOfFileReached (StateChangedHandler handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromEndOfFileReached (XnCallbackHandle hCallback) |
XnStatus | SetPlaybackSpeed (XnDouble dSpeed) |
XnDouble | GetPlaybackSpeed () const |
Detailed Description
Purpose: The Player class is used for creating and managing a Player node. This node plays a saved recording of an OpenNI data generation session.
Remarks:
To play a file recording, use the xn::Context::OpenFileRecording() function. OpenNI will open the file, create a mock node for each node in the file, and populate it with the recorded configuration.
An application may take the nodes it needs by calling the xn::Context::FindExistingNode() method, and use them normally.
- Note:
- Nodes created by the player are locked, and cannot be changed, as the configuration must remain according to the recorded configuration.
Applications that initialize OpenNI using an XML file can easily replace their input. This means that instead of reading from a physical device, which generates data in real-time, they read from a recording by replacing the nodes in the XML file with a recording element (see Xml Scripts).
Constructor & Destructor Documentation
xn::Player::Player | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Player node handle
xn::Player::Player | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
Creates a player node.
Remarks
See Concepts: the Create() Method for a detailed description about the operation of this method.
XnStatus xn::Player::EnumerateNodes | ( | NodeInfoList & | list | ) | const [inline] |
Retrieves a list of the nodes played by a player.
For full details and usage, see xnEnumeratePlayerNodesXnStatus xn::Player::GetNumFrames | ( | const XnChar * | strNodeName, |
XnUInt32 & | nFrames | ||
) | const [inline] |
Gets the total number of frames a specific node has in the recording.
- Parameters:
-
[in] strNodeName Name of the node for which to retrieve the number of frames. [out] nFrames Retrieved number of frames.
XnDouble xn::Player::GetPlaybackSpeed | ( | ) | const [inline] |
Gets the playback speed.
- Returns:
- Speed ratio, or -1.0 if this node is not a player.
XnStatus xn::Player::GetSource | ( | XnRecordMedium & | sourceType, |
XnChar * | strSource, | ||
XnUInt32 | nBufSize | ||
) | const [inline] |
Gets the player's source, that is, the type and name of the medium that the recording is played back from.
- Parameters:
-
[out] sourceType Player's source type. [in] strSource Player's source. [in] nBufSize Size of the buffer specified by strSource
.
const XnChar* xn::Player::GetSupportedFormat | ( | ) | const [inline] |
Gets the name of the format supported by a player.
For full details and usage, see xnGetPlayerSupportedFormatXnBool xn::Player::IsEOF | ( | ) | const [inline] |
Returns whether the player is at the end-of-file marker.
Remarks
When Repeat mode is on, this function never returns TRUE. However, the 'End of File Reached' event will be raised during rewind.
XnStatus xn::Player::ReadNext | ( | ) | [inline] |
Reads the next data element from the player.
For full details and usage, see xnPlayerReadNextXnStatus xn::Player::RegisterToEndOfFileReached | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'End-Of-File Reached' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::Player::SeekToFrame | ( | const XnChar * | strNodeName, |
XnInt32 | nFrameOffset, | ||
XnPlayerSeekOrigin | origin | ||
) | [inline] |
Moves the player to a specific frame of a specific played node, e.g., an ImageGenerator node, so that playing will continue from that frame onwards.
- Parameters:
-
[in] strNodeName Name of the node. [in] nFrameOffset Number of frames to move, relative to the specified origin. See remarks below. [in] origin Origin to seek from. See remarks below.
The meaning of the nFrameOffset
parameter changes according to the origin parameter:
XN_PLAYER_SEEK_SET: nFrameOffset
specifies the total number of frames since the beginning of the node's recording. This must be a positive value.
XN_PLAYER_SEEK_CUR: nFrameOffset
specifies the number of frames to move, relative to the current frame of the specified node. A positive value means to move forward, and a negative value means to move backwards.
XN_PLAYER_SEEK_END: nFrameOffset
specifies the number of frames to move, relative to the end of the node's recording. This must be a negative value.
You can get different results using this function for different values of strNodeName, because the frame numbers of different nodes are not necessarily in sync.
XnStatus xn::Player::SeekToTimeStamp | ( | XnInt64 | nTimeOffset, |
XnPlayerSeekOrigin | origin | ||
) | [inline] |
Moves the player to a specific time, so that playback will continue from that point onwards.
- Parameters:
-
[in] nTimeOffset Offset to move, relative to the specified origin.
Units in microseconds.
See the remark below.[in] origin Origin to seek from. See the remarks below.
The meaning of the nTimeOffset
parameter changes according to the origin parameter:
XN_PLAYER_SEEK_SET: nTimeOffset
specifies the total time passed since the beginning of the recording. This must be a positive value.
XN_PLAYER_SEEK_CUR: nTimeOffset
specifies a period of time to move, relative to the current player position. A positive value means to move forward, and a negative value means to move backwards.
XN_PLAYER_SEEK_END: nTimeOffset
specifies a period of time to move, relative to the end of the recording. This must be a negative value.
Remarks
For the built-in ONI player, this function is not currently supported and always returns XN_STATUS_NOT_IMPLEMENTED.
XnStatus xn::Player::SetPlaybackSpeed | ( | XnDouble | dSpeed | ) | [inline] |
Sets the player's playback speed, as a ratio of the rate that the recording was made at.
- Parameters:
-
[in] dSpeed The speed ratio.
Values:
dSpeed
= 1.0 - Player will try to output frames at the same rate they were recorded (according to their timestamps).
dSpeed
> 1.0 - Fast-forward
0 < dSpeed
< 1.0 - Slow-motion.
dSpeed
= XN_PLAYBACK_SPEED_FASTEST (0.0) - There will be no delay, and frames will be returned immediately on demand.
XnStatus xn::Player::SetRepeat | ( | XnBool | bRepeat | ) | [inline] |
Specifies whether the player will automatically rewind to the beginning of the recording after reaching the end of the recording.
- Parameters:
-
[in] bRepeat Determines whether the player will repeat or not.
If bRepeat is set to TRUE, the player will automatically rewind when reaching the end.
If bRepeat is set to FALSE, the player will stop playing when reaching the end, and will raise the 'End-Of-File Reached' event. In this state, IsEOF() returns TRUE, and calls to ReadNext() will fail.
XnStatus xn::Player::SetSource | ( | XnRecordMedium | sourceType, |
const XnChar * | strSource | ||
) | [inline] |
Sets the source for the player, i.e. where the played events will come from.
For full details and usage, see xnSetPlayerSourceXnStatus xn::Player::TellFrame | ( | const XnChar * | strNodeName, |
XnUInt32 & | nFrame | ||
) | const [inline] |
Gets the current frame number of a specific node played by a player, i.e., the number of frames passed since the beginning of the recording.
- Parameters:
-
[in] strNodeName Name of the node. [out] nFrame Retrieved frame number.
Remarks
Different nodes that belong to the player can have different frame numbers, because the nodes are not necessarily in synchronization.
XnStatus xn::Player::TellTimestamp | ( | XnUInt64 & | nTimestamp | ) | const [inline] |
Gets the current time of a player, i.e., the time passed since the beginning of the recording.
- Parameters:
-
[out] nTimestamp Retrieved timestamp. Units are in microseconds.
void xn::Player::UnregisterFromEndOfFileReached | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the event handler for the 'End-Of-File Reached' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
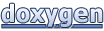