![]() |
OpenNI 1.5.4
|
#include <XnArray.h>
Public Types | |
enum | { BASE_SIZE = 8 } |
typedef T * | Iterator |
typedef const T * | ConstIterator |
Public Member Functions | |
XnArray (XnUInt32 nBaseSize=BASE_SIZE) | |
XnArray (const XnArray &other) | |
virtual | ~XnArray () |
XnArray & | operator= (const XnArray &other) |
XnStatus | CopyFrom (const XnArray &other) |
XnStatus | SetData (const T *pData, XnUInt32 nSize) |
const T * | GetData () const |
T * | GetData () |
XnStatus | Reserve (XnUInt32 nReservedSize) |
XnBool | IsEmpty () const |
XnUInt32 | GetSize () const |
XnStatus | SetSize (XnUInt32 nSize) |
XnStatus | SetSize (XnUInt32 nSize, const T &fillVal) |
XnStatus | SetMinSize (XnUInt32 nSize) |
XnStatus | SetMinSize (XnUInt32 nSize, const T &fillVal) |
XnUInt32 | GetAllocatedSize () const |
XnStatus | Set (XnUInt32 nIndex, const T &val) |
XnStatus | Set (XnUInt32 nIndex, const T &val, const T &fillVal) |
XnStatus | AddLast (const T &val) |
XnStatus | AddLast (const T *aValues, XnUInt32 nCount) |
void | Clear () |
T & | operator[] (XnUInt32 nIndex) |
const T & | operator[] (XnUInt32 nIndex) const |
Iterator | begin () |
ConstIterator | begin () const |
Iterator | end () |
ConstIterator | end () const |
template<typename T>
class XnArray< T >
Member Typedef Documentation
typedef const T* XnArray< T >::ConstIterator |
A constant iterator object that allows you to iterate over members in the array as read-only values.
An iterator object that allows you to iterate over members in the array.
Member Enumeration Documentation
Constructor & Destructor Documentation
Default constructor. Initializes the array to BASE_SIZE.
Copy constructor. Initializes this array from another array of the same type.
Destructor. Deallocates data in this array.
Member Function Documentation
Adds one element to the end of this array.
Adds a range of values to the end of this array.
- Returns:
- a modifiable iterator pointing to the beginning of this array.
ConstIterator XnArray< T >::begin | ( | ) | const [inline] |
- Returns:
- a const iterator pointing to the beginning of this array.
void XnArray< T >::Clear | ( | ) | [inline] |
Resets the data of this array and sets its size to 0.
Copies data from another array of the same type.
- Returns:
- a modifiable itertor pointing to the end of this array.
ConstIterator XnArray< T >::end | ( | ) | const [inline] |
- Returns:
- a const itertor pointing to the end of this array.
XnUInt32 XnArray< T >::GetAllocatedSize | ( | ) | const [inline] |
- Returns:
- The allocated size of this array. This is the maximum number of elements that the array can hold without re-allocating and copying data.
const T* XnArray< T >::GetData | ( | ) | const [inline] |
- Returns:
- the raw data of this array as a read-only buffer.
T* XnArray< T >::GetData | ( | ) | [inline] |
- Returns:
- the raw data of this array as a modifiable buffer.
XnUInt32 XnArray< T >::GetSize | ( | ) | const [inline] |
- Returns:
- The number of elements in this array.
XnBool XnArray< T >::IsEmpty | ( | ) | const [inline] |
- Returns:
- TRUE if this array is empty, FALSE otherwise.
Assignment operator. Copies data from another array of the same type.
T& XnArray< T >::operator[] | ( | XnUInt32 | nIndex | ) | [inline] |
Element access operator. Returns a modifiable reference to the element at specified index.
const T& XnArray< T >::operator[] | ( | XnUInt32 | nIndex | ) | const [inline] |
Element access operator. Returns a const reference to the element at specified index.
Reserves space in this array for the specified number of elements. This saves you re-allocations and data copies if you know the size in advance.
Sets value at specified index, grows array if needed. Note that when dealing with primitive types and adding beyond the current size of the array, any added values that fill the gap are uninitialized
XnStatus XnArray< T >::Set | ( | XnUInt32 | nIndex, |
const T & | val, | ||
const T & | fillVal | ||
) | [inline] |
Sets value at specified index and grows array if needed, filling any resulting blank elements with fillVal.
Copies data from a raw buffer of the same type pointed to by pData, and sets size to nSize.
Makes sure the array has at least nSize elements. If nSize is less than the current size, there is no effect. Note that for primitive types, any added elements are uninitialized.
XnStatus XnArray< T >::SetMinSize | ( | XnUInt32 | nSize, |
const T & | fillVal | ||
) | [inline] |
Makes sure the array has at least nSize elements. If nSize is less than the current size, there is no effect. Any added elements are initialized by the value specified by fillVal.
Sets the size of this array to the specified number of elements. Note that for primitive types, the data is uninitialized.
Sets the size of this array to the specified number of elements, initializing all elements with the value specified by fillVal.
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:06 for OpenNI 1.5.4 by
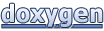