![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
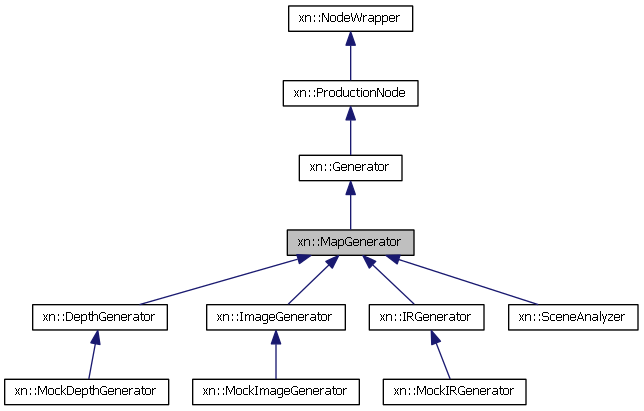
Detailed Description
Purpose: The MapGenerator class is the base class for every generator node that produces 2D map data, for example, depth maps, color image maps, IR maps, and scene analysis maps.
Usage: This class might be instantiated to reference any kind of map generator node (i.e., a node that derives from the MapGenerator class), however it is not intended to ever be instantiated to create any node of MapGenerator type itself.
Remarks:
Since the MapGenerator class is the base class for generator nodes that produce image maps, it provides the data structures common to all map generators: the current frame's X and Y dimensions and its frame rate.
MapGenerator's two principle functions are GetMapOutputMode() and SetMapOutputMode() for controlling and checking the node's map output mode. The map output mode is the combination of the node's scene resolution and frame rate. Correspondingly, these functions read and write an xn::XnMapOutputMode structure, comprising three parameters: the X and Y resolutions and the frame rate.
Constructor & Destructor Documentation
xn::MapGenerator::MapGenerator | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::MapGenerator::MapGenerator | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
AntiFlickerCapability xn::MapGenerator::GetAntiFlickerCap | ( | ) | [inline] |
Gets an AntiFlickerCapability object for accessing Anti Flicker functionality. It is the application responsibility to check first if XN_CAPABILITY_ANTI_FLICKER is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetBacklightCompensationCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing BacklightCompensation functionality. It is the application responsibility to check first if XN_CAPABILITY_BACKLIGHT_COMPENSATION is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetBrightnessCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Brightness functionality. It is the application responsibility to check first if XN_CAPABILITY_BRIGHTNESS is supported by calling IsCapabilitySupported().
XnUInt32 xn::MapGenerator::GetBytesPerPixel | ( | ) | const [inline] |
Gets the number of bytes per pixel for the node's map data. This mode is set by SetPixelFormat() for the next frame that the generator node generates.
Remarks
In ImageGenerator this can be set. In other generators, it's pre-defined.
GeneralIntCapability xn::MapGenerator::GetContrastCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Contrast functionality. It is the application responsibility to check first if XN_CAPABILITY_CONTRAST is supported by calling IsCapabilitySupported().
const CroppingCapability xn::MapGenerator::GetCroppingCap | ( | ) | const [inline] |
Gets a CroppingCapability object for accessing Cropping functionality.
Remarks
It is the application's responsibility to check first if XN_CAPABILITY_CROPPING is supported by calling IsCapabilitySupported().
CroppingCapability xn::MapGenerator::GetCroppingCap | ( | ) | [inline] |
Gets a CroppingCapability object for accessing Cropping functionality.
Remarks
It is the application's responsibility to check first if XN_CAPABILITY_CROPPING is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetExposureCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Exposure functionality. It is the application responsibility to check first if XN_CAPABILITY_EXPOSURE is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetFocusCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Focus functionality. It is the application responsibility to check first if XN_CAPABILITY_FOCUS is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetGainCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Gain functionality. It is the application responsibility to check first if XN_CAPABILITY_GAIN is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetGammaCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Gamma functionality. It is the application responsibility to check first if XN_CAPABILITY_GAMMA is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetHueCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Hue functionality. It is the application responsibility to check first if XN_CAPABILITY_HUE is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetIrisCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Iris functionality. It is the application responsibility to check first if XN_CAPABILITY_IRIS is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetLowLightCompensationCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Low Light Compensation functionality. It is the application responsibility to check first if XN_CAPABILITY_LOW_LIGHT_COMPENSATION is supported by calling IsCapabilitySupported().
XnStatus xn::MapGenerator::GetMapOutputMode | ( | XnMapOutputMode & | OutputMode | ) | const [inline] |
Gets the current map output mode of the generator node. This is the map output mode that the generator node will use to generate the next data frame.
Remarks
A map output mode is the combination of the scene resolution and frame rate. It is contained in an xn::XnMapOutputMode structure comprising the frame rate (in frames per second), and the X and Y dimensions (the number of elements in each of the X- and Y- axes).
For the map output mode of the most recent data frame available in the node's metadata object, see the xn::MapMetaData class (MapMetaData::Xres(), MapMetaData::YRes(), and MapMetaData::FPS() methods).
This map output mode is the mode set by SetMapOutputMode().
GeneralIntCapability xn::MapGenerator::GetPanCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Pan functionality. It is the application responsibility to check first if XN_CAPABILITY_PAN is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetRollCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Roll functionality. It is the application responsibility to check first if XN_CAPABILITY_ROLL is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetSaturationCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Saturation functionality. It is the application responsibility to check first if XN_CAPABILITY_SATURATION is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetSharpnessCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Sharpness functionality. It is the application responsibility to check first if XN_CAPABILITY_SHARPNESS is supported by calling IsCapabilitySupported().
XnStatus xn::MapGenerator::GetSupportedMapOutputModes | ( | XnMapOutputMode * | aModes, |
XnUInt32 & | nCount | ||
) | const [inline] |
Gets a list of all the output modes that the generator node supports.
Remarks
Each supported map output mode is represented by a XnMapOutputMode structure comprising resolution (nXRes
and nYRes
) and frames rate (nFPS
). The application then chooses one of the output modes. The size of the array that should be passed can be obtained by calling GetSupportedMapOutputModesCount().
XnUInt32 xn::MapGenerator::GetSupportedMapOutputModesCount | ( | ) | const [inline] |
Gets the number of output modes that the generator node supports.
Remarks
This is useful for allocating an array that will be passed to GetSupportedMapOutputModes().
GeneralIntCapability xn::MapGenerator::GetTiltCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Tilt functionality. It is the application responsibility to check first if XN_CAPABILITY_TILT is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetWhiteBalanceCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing WhiteBalance functionality. It is the application responsibility to check first if XN_CAPABILITY_COLOR_TEMPERATURE is supported by calling IsCapabilitySupported().
GeneralIntCapability xn::MapGenerator::GetZoomCap | ( | ) | [inline] |
Gets an GeneralIntCapability object for accessing Zoom functionality. It is the application responsibility to check first if XN_CAPABILITY_ZOOM is supported by calling IsCapabilitySupported().
XnStatus xn::MapGenerator::RegisterToMapOutputModeChange | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Map Output Mode Change' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::MapGenerator::SetMapOutputMode | ( | const XnMapOutputMode & | OutputMode | ) | [inline] |
Sets the current map output mode of the generator node.
Remarks
A map output mode is contained in a xn::XnMapOutputMode structure comprising the frame rate (in frames per second), and the X and Y dimensions (the number of elements in each of the X- and Y- axes).
It is the application programmer's responsibility to check first if the output mode is supported by calling the GetSupportedMapOutputModes() method.
void xn::MapGenerator::UnregisterFromMapOutputModeChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the event handler for the 'Map Output Mode Change' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
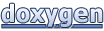