![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
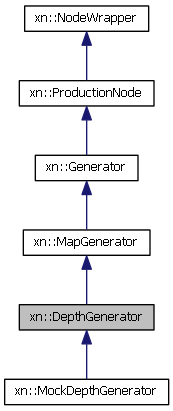
Public Member Functions | |
DepthGenerator (XnNodeHandle hNode=NULL) | |
DepthGenerator (const NodeWrapper &other) | |
XnStatus | Create (Context &context, Query *pQuery=NULL, EnumerationErrors *pErrors=NULL) |
void | GetMetaData (DepthMetaData &metaData) const |
const XnDepthPixel * | GetDepthMap () const |
XnDepthPixel | GetDeviceMaxDepth () const |
XnStatus | GetFieldOfView (XnFieldOfView &FOV) const |
XnStatus | RegisterToFieldOfViewChange (StateChangedHandler handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromFieldOfViewChange (XnCallbackHandle hCallback) |
XnStatus | ConvertProjectiveToRealWorld (XnUInt32 nCount, const XnPoint3D aProjective[], XnPoint3D aRealWorld[]) const |
XnStatus | ConvertRealWorldToProjective (XnUInt32 nCount, const XnPoint3D aRealWorld[], XnPoint3D aProjective[]) const |
const UserPositionCapability | GetUserPositionCap () const |
UserPositionCapability | GetUserPositionCap () |
Detailed Description
Purpose: A DepthGenerator node generates depth maps. Each pixel value of a depth map represents a distance from the sensor's plane in millimeters.
Usage: Instantiate the class and call Create() to create a DepthGenerator node.
This class is at the first level of classes in the OpenNI class hierarchy that is typically instantiated to create an actual node. (Examples of other classes at the first level are xn::ImageGenerator and xn::SceneAnalyzer).
Data output: Depth maps and associated configuration data. Access via the node's xn::DepthMetaData object.
Overview:
A DepthGenerator node generates a depth map as an array of pixels, where each pixel is a depth value representing a distance from the sensor's plane in millimeters. This is the Z-coordinate of the X-Y-Z coordinate of each user pixel. A smaller depth value indicates a scene point that is closer to the hardware sensor device; and a larger depth value indicates a scene point that is a further away from the sensor. A zero depth value indicates that the DepthGenerator node did not succeed in obtaining a valid depth reading.
Typically, the process of generating 3D data begins by using a sensor device that produces raw output data. Dedicated middleware is then used to process this raw output, and produce a higher-level output, which can then be understood and used by the application.
In a typical OpenNI production graph, a DepthGenerator node takes input directly from a sensor device and generates a depth map. Other generator nodes further along the production graph (dependant on the DepthGenerator node) take input from the DepthGenerator node's output. However, alternative dependency configurations are also possible.
The DepthGenerator node's data and associated configuration are accessed through its frame object (i.e., its xn::DepthMetaData object).
Summary of Main Features:
- Depth map: Provides the data of the depth map
- Sensor device's maximum depth: Provides the maximum distance available for this depth generator
- Field of View: Provides the sensor's maximum horizontal and vertical angles
- User Position capability (optional)
Constructor & Destructor Documentation
xn::DepthGenerator::DepthGenerator | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::DepthGenerator::DepthGenerator | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
XnStatus xn::DepthGenerator::ConvertProjectiveToRealWorld | ( | XnUInt32 | nCount, |
const XnPoint3D | aProjective[], | ||
XnPoint3D | aRealWorld[] | ||
) | const [inline] |
Converts a list of points from projective coordinates to real world coordinates.
- Parameters:
-
[in] nCount Number of points to convert. [in] aProjective Array of projective point coordinates. [in,out] aRealWorld Array to be filled with real-world point coordinates.
See Coordinate Systems for futher information.
XnStatus xn::DepthGenerator::ConvertRealWorldToProjective | ( | XnUInt32 | nCount, |
const XnPoint3D | aRealWorld[], | ||
XnPoint3D | aProjective[] | ||
) | const [inline] |
Converts a list of points from real world coordinates to projective coordinates.
- Parameters:
-
[in] nCount Number of points to convert. [in] aRealWorld Array of projective point coordinates. [in,out] aProjective Array to be filled with real-world point coordinates.
See Coordinate Systems for futher information.
XnStatus xn::DepthGenerator::Create | ( | Context & | context, |
Query * | pQuery = NULL , |
||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Creates a DepthGenerator node from available production node alternatives.
Remarks:
For full details and usage, see Understanding the Create() method.
const XnDepthPixel* xn::DepthGenerator::GetDepthMap | ( | ) | const [inline] |
Gets the current depth-map. This map is updated after a call to xnWaitAndUpdateData().
For full details and usage, see xnGetDepthMapXnDepthPixel xn::DepthGenerator::GetDeviceMaxDepth | ( | ) | const [inline] |
Gets the maximum depth value that the DepthGenerator node can generate. The maximum depth value is a hardware specification of the sensor.
Remarks:
A typical maximum depth supports up to 10 meters of depth. Units are in millimeters, so the maximum depth value for 10 meters of depth would range from 0 to 10,000.
XnStatus xn::DepthGenerator::GetFieldOfView | ( | XnFieldOfView & | FOV | ) | const [inline] |
Gets the dimensions of the field of view (FOV) of the hardware sensor.
- Parameters:
-
[out] FOV A struct to be filled with the field of view of the hardware sensor.
Remarks:
This method gets the dimensions of the field of view in radians. Radians are used for the output form since they are a spherical measurement and the FOV is defined in terms of the angle of width and height that the sensor sees, e.g., 0.524 radians (30 degrees) above and below, and 1.047 radians (60 degrees) left and right.
This method is useful for developers building algorithms to convert between real world and productive coordinate systems. Although OpenNI offers its own functionality for converting real-world and productive coordinate systems, some developers may wish to build their own conversion functions for reasons of optimization.
void xn::DepthGenerator::GetMetaData | ( | DepthMetaData & | metaData | ) | const [inline] |
Gets the depth generator node's latest frame object, saving it in the xn::DepthMetaData object. This frame object is a snapshot of the generated depth map data and its associated configuration information at a certain point in time. This saved frame object provides fast and easy access to the depth generator node's data and configuration information.
- Parameters:
-
[out] metaData Structure to be filled with the frame object.
Remarks:
This method is central in the use of the DepthGenerator node. Once the DepthGenerator node is generating data, after each 'Update Data' call, the application calls this method to get the node's frame object, which contains the data and all its associated properties.
const UserPositionCapability xn::DepthGenerator::GetUserPositionCap | ( | ) | const [inline] |
Gets an UserPositionCapability object for accessing User Position functionality. It is the application responsibility to check first if XN_CAPABILITY_USER_POSITION is supported by calling IsCapabilitySupported().
UserPositionCapability xn::DepthGenerator::GetUserPositionCap | ( | ) | [inline] |
Gets an UserPositionCapability object for accessing User Position functionality. It is the application responsibility to check first if XN_CAPABILITY_USER_POSITION is supported by calling IsCapabilitySupported().
XnStatus xn::DepthGenerator::RegisterToFieldOfViewChange | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Field-Of-View Change' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
void xn::DepthGenerator::UnregisterFromFieldOfViewChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the event handler for the 'Field-Of-View Change' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
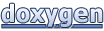