![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
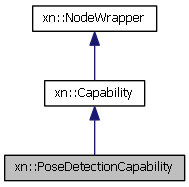
Classes | |
struct | PoseCookie |
struct | PoseDetectionCookie |
struct | PoseInProgressCookie |
Public Types | |
typedef void(* | PoseDetection )(PoseDetectionCapability &pose, const XnChar *strPose, XnUserID user, void *pCookie) |
typedef void(* | PoseInProgress )(PoseDetectionCapability &pose, const XnChar *strPose, XnUserID user, XnPoseDetectionStatus poseError, void *pCookie) |
Public Member Functions | |
PoseDetectionCapability (XnNodeHandle hNode) | |
PoseDetectionCapability (const NodeWrapper &node) | |
XnUInt32 | GetNumberOfPoses () const |
XnStatus | GetAllAvailablePoses (XnChar **pstrPoses, XnUInt32 nNameLength, XnUInt32 &nPoses) const |
XnBool | IsPoseSupported (const XnChar *strPose) |
XnStatus | GetPoseStatus (XnUserID userID, const XnChar *poseName, XnUInt64 &poseTime, XnPoseDetectionStatus &eStatus, XnPoseDetectionState &eState) |
XnStatus | StartPoseDetection (const XnChar *strPose, XnUserID user) |
XnStatus | StopPoseDetection (XnUserID user) |
XnStatus | StopSinglePoseDetection (XnUserID user, const XnChar *strPose) |
XnStatus | RegisterToPoseDetected (PoseDetection handler, void *pCookie, XnCallbackHandle &hCallback) |
XnStatus | RegisterToOutOfPose (PoseDetection handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromPoseDetected (XnCallbackHandle hCallback) |
void | UnregisterFromOutOfPose (XnCallbackHandle hCallback) |
XnStatus | RegisterToPoseInProgress (PoseInProgress handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromPoseInProgress (XnCallbackHandle hCallback) |
Detailed Description
Purpose: Provides a 'Pose Detection' functionality for a xn::UserGenerator node. This capability lets the node recognize when the user is posed in a specific position.
Usage: Do not instantiate directly. Instead, use UserGenerator::GetPoseDetectionCap() to obtain an instance.
Data output: Raises events.
Remarks:
A pose is a specific full body posture involving all the limbs. The PoseDetectionCapability object scans the FOV to detect users performing poses. The PoseDetectionCapability then generates the pose data.
When and if the PoseDetectionCapability object detects a pose it raises the 'Pose Detected' event.
Member Typedef Documentation
typedef void(* xn::PoseDetectionCapability::PoseDetection)(PoseDetectionCapability &pose, const XnChar *strPose, XnUserID user, void *pCookie) |
'Pose Detection' event
This event is raised to signal that a specific user has entered a pose.
- Parameters:
-
[in] pose Reference to the specific xn::UserGenerator node that raised the event. [in] strPose Name of the specific pose defined for this event handler. (The PoseDetectionCapability can define more than a single pose that can be detected.) [in] user ID of the user that entered the pose. [in] pCookie Returns any information ("cookie") that the application placed in this parameter at the time of registering the event..
typedef void(* xn::PoseDetectionCapability::PoseInProgress)(PoseDetectionCapability &pose, const XnChar *strPose, XnUserID user, XnPoseDetectionStatus poseError, void *pCookie) |
'Pose In Progress' event:
Raised at each frame time from the time StartPoseDetection was called until the pose is detected. This event returns the current status of pose detection of a user.
- Parameters:
-
[in] pose Reference to the specific UserGenerator node that raised the event. [in] strPose Name of the specific pose defined for this event handler. (The PoseDetectionCapability can define more than a single pose that can be detected. [in] user ID of the user that entered the pose. [in] poseError Current status of the pose detection. Defined statuses: XN_POSE_DETECTION_STATUS_OK XN_POSE_DETECTION_STATUS_NO_USER XN_POSE_DETECTION_STATUS_TOP_FOV XN_POSE_DETECTION_STATUS_SIDE_FOV XN_POSE_DETECTION_STATUS_ERROR [in] pCookie Returns any information ("cookie") that the application placed in this parameter at the time of registering the event.
Constructor & Destructor Documentation
xn::PoseDetectionCapability::PoseDetectionCapability | ( | XnNodeHandle | hNode | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::PoseDetectionCapability::PoseDetectionCapability | ( | const NodeWrapper & | node | ) | [inline] |
Member Function Documentation
XnStatus xn::PoseDetectionCapability::GetAllAvailablePoses | ( | XnChar ** | pstrPoses, |
XnUInt32 | nNameLength, | ||
XnUInt32 & | nPoses | ||
) | const [inline] |
Gets the names of all poses supported by this capability.
- Parameters:
-
[out] pstrPoses Pre-allocated memory for the names of the supported poses. [in] nNameLength Memory size for each pose name. [in,out] nPoses In input - size of the preallocated memory, in output - the number of pose names.
Remarks
The names of the poses are preset in advance by the developer of this capability.
XnUInt32 xn::PoseDetectionCapability::GetNumberOfPoses | ( | ) | const [inline] |
Get the number of poses supported by this capability.
Remarks
The number of supported poses is defined by the developer of the capability and is common to all xn::UserGenerator nodes using this capability on this OpenNI deployment.
XnStatus xn::PoseDetectionCapability::GetPoseStatus | ( | XnUserID | userID, |
const XnChar * | poseName, | ||
XnUInt64 & | poseTime, | ||
XnPoseDetectionStatus & | eStatus, | ||
XnPoseDetectionState & | eState | ||
) | [inline] |
XnBool xn::PoseDetectionCapability::IsPoseSupported | ( | const XnChar * | strPose | ) | [inline] |
XnStatus xn::PoseDetectionCapability::RegisterToOutOfPose | ( | PoseDetection | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Out Of Pose' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::PoseDetectionCapability::RegisterToPoseDetected | ( | PoseDetection | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Pose Detected' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::PoseDetectionCapability::RegisterToPoseInProgress | ( | PoseInProgress | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Pose In Progress' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
Remarks
This event is raised at each frame time from the time StartPoseDetection() method was called until the pose is actually detected.
See the PoseDetection callback type declaration for how to write the callback (event handler).
XnStatus xn::PoseDetectionCapability::StartPoseDetection | ( | const XnChar * | strPose, |
XnUserID | user | ||
) | [inline] |
Starts attempting to detect a pose for a specific user.
- Parameters:
-
[in] strPose Name of the requested pose. Call the GetAvailablePoses() method to get the names of poses supported by the PoseDetectionCapability. [in] user ID of a user to detect a pose for.
Remarks
This method will typically be used after a new user has been recognized in the FOV. This is typically the next stage in the process of calibrating the new user -
- See also:
- skeleton_calib. When and if the PoseDetectionCapability object detects a Pose Detection Capability it raises the 'Pose Detected' event.
Stops attempting to detect a pose (for a specific user).
- Parameters:
-
[in] user ID of a user.
Remarks
The application will typically call this method when it has already received a 'Pose Detected' event. Once a pose has been detected it is no longer necessary to try to detect poses.
XnStatus xn::PoseDetectionCapability::StopSinglePoseDetection | ( | XnUserID | user, |
const XnChar * | strPose | ||
) | [inline] |
Stop detection of a specific pose for a specific user.
For full details and usage, see xnStopSinglePoseDetectionvoid xn::PoseDetectionCapability::UnregisterFromOutOfPose | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Out Of Pose' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::PoseDetectionCapability::UnregisterFromPoseDetected | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Pose Detected' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::PoseDetectionCapability::UnregisterFromPoseInProgress | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Pose In Progress' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
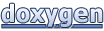