![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
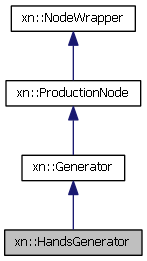
Classes | |
struct | HandCookie |
Public Types | |
typedef void(* | HandCreate )(HandsGenerator &generator, XnUserID user, const XnPoint3D *pPosition, XnFloat fTime, void *pCookie) |
typedef void(* | HandUpdate )(HandsGenerator &generator, XnUserID user, const XnPoint3D *pPosition, XnFloat fTime, void *pCookie) |
typedef void(* | HandDestroy )(HandsGenerator &generator, XnUserID user, XnFloat fTime, void *pCookie) |
Public Member Functions | |
HandsGenerator (XnNodeHandle hNode=NULL) | |
HandsGenerator (const NodeWrapper &other) | |
XnStatus | Create (Context &context, Query *pQuery=NULL, EnumerationErrors *pErrors=NULL) |
XnStatus | RegisterHandCallbacks (HandCreate CreateCB, HandUpdate UpdateCB, HandDestroy DestroyCB, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterHandCallbacks (XnCallbackHandle hCallback) |
XnStatus | StopTracking (XnUserID user) |
XnStatus | StopTrackingAll () |
XnStatus | StartTracking (const XnPoint3D &ptPosition) |
XnStatus | SetSmoothing (XnFloat fSmoothingFactor) |
const HandTouchingFOVEdgeCapability | GetHandTouchingFOVEdgeCap () const |
HandTouchingFOVEdgeCapability | GetHandTouchingFOVEdgeCap () |
Detailed Description
The HandsGenerator node generates tracking data about a single hand or multiple hands with persistent IDs.
The user interface (UI) in natural interaction applications is specifically implemented and performed through hand movements.
OpenNI's user interface (UI) ability is specifically through hand movements. Thus it is the HandsGenerator class that provides the main UI ability.
Using events, the HandsGenerator object notifies the application of various occurrences: a 'Hand Create' event is sent when an expected hand has been positively identified or when a new hand appears; a 'Hand Update' event is sent when a tracked hand appears again in a new frame; a 'Hand Destroy' event is sent when a tracked hand disappears.
NOTE: The HandsGenerator does not provide the mechanism for initially locating the hand. Initial location of the hand can be performed by the Gestures class (see further below). This is a typical scenario but not the only way to locate the hand.
The following figure illustrates a typical scenario for using the HandsGenerator class. After hand recognition the HandsGenerator class continues tracking the hand and sending HandUpdate events until the application sends calls HandsGenerator's StopTracking method.
1. Creating a HandsGenerator
As for many other types of generator nodes, HandsGenerator provides a Create method to create a hands generator node.
Assuming you have hands generator module (plug-in) installed on your computer, you can create a hands generator node. Then you can start tracking for an expected hand.
- Note:
- Creating a HandsGenerator node has nothing to do with the 'Hand Create' event that OpenNI sends to the app on starting to successfully track the hand in the FOV.
2. Starting Tracking
When starting to track a hand, the application already expects that a person's hand is already present in the field of view and is at a certain coordinate (x,y) position.
The source for this information, i.e., the presence of a hand object and its position, can be obtained, for example, from a gesture generator node. The application passes this location to the StartTracking() method of the HandsGenerator object, which starts tracking for the expected hand. When - and if - the StartTracking() method finds the hand at the expected position it sends a 'Hand Create' event to the application.
3. Tracking
While tracking a hand, 'Hand Update' events are sent to the application notifying that the hand has moved to such and such a position. A 'Hand Update' event is sent each time a new frame processed by the input sensors shows the hand is still present. A 'Hand Update' event is sent even if the hand is still in the same position as it was in the previous frame.
If the tracked hand disappears (e.g., when the person puts his hand behind his back) a 'Hand Destroy' event is sent to the application to notify that the hand has disappeared.
The 'Hand Create' event is also sent if an additional hand is found internally.
The tracking mechanism uses an algorithm that uses the depth to obtain points and track. This algorithm can be somewhat susceptible to jitter of the hand, so HandsGenerator provides a smoothing capability. Call the SetSmoothing() method to apply a smoothing factor of none (0) to maximum (1). The default is 0. Recommended is 0.1 when using the PrimeSense algorithm for hand generation.
4. Stopping Tracking
When the hand being tracked is no longer identified, the HandsGenerator object sends the 'Hand Destroy' event.
The application can also intentionally stop tracking a specific hand, e.g., when the hand is no longer of interest. The application does this by calling the StopTracking() method. When the node stops tracking the hand it sends a 'Hand Destroy' event to the application.
The application can also stop the tracking of all hands at once by calling the StopTrackingAll() method. In response, the node stops tracking all hands and for each hand it sends a 'Hand Destroy' event to the application. Even when there are no tracked hands, the HandsGenerator object remains functional for the next tracking request.
Events:
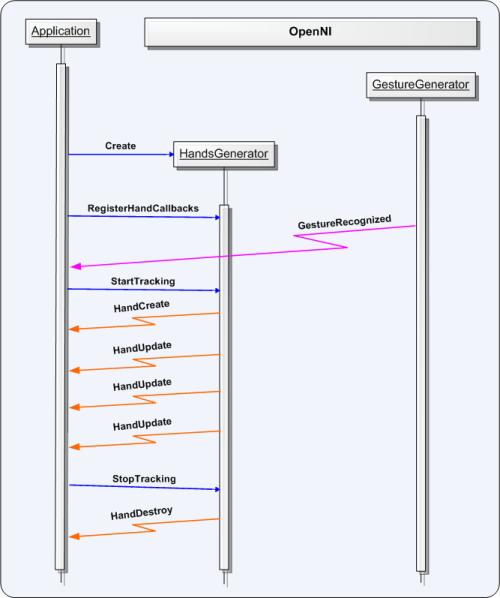
Member Typedef Documentation
typedef void(* xn::HandsGenerator::HandCreate)(HandsGenerator &generator, XnUserID user, const XnPoint3D *pPosition, XnFloat fTime, void *pCookie) |
Callback for the creation of a new hand.
Signals that the hands generator has recognized and started tracking a new hand in response to the application calling the StartTracking() method. This event returns the ID of the new hand, the time of its recognition, and its position on recognition in the current frame.
- Parameters:
-
[in] generator Generator that raised this event. [in] user ID of the new hand. [in] pPosition Position at which the hand was created. [in] fTime Timestamp, in seconds. [in] pCookie User-provided cookie that was given when registering to this event.
typedef void(* xn::HandsGenerator::HandDestroy)(HandsGenerator &generator, XnUserID user, XnFloat fTime, void *pCookie) |
Callback for the disappearance of a hand.
Signals that an existing hand has disappeared from the frame for any reason. This event returns the user ID – still the same user ID as before – and the time that it disappeared.
- Parameters:
-
[in] generator the generator that raised this event. [in] user The id of the hand that disappeared. [in] fTime Timestamp, in seconds. [in] pCookie A user-provided cookie that was given when registering to this event.
typedef void(* xn::HandsGenerator::HandUpdate)(HandsGenerator &generator, XnUserID user, const XnPoint3D *pPosition, XnFloat fTime, void *pCookie) |
Signals that an already-existing hand has moved to a new position.
Signals that a currently tracked hand was recognized at a specific position in the new frame. OpenNI continues to send this event at each further frame that the hand is still present. This event returns the ID of the hand, which is the same ID returned by the HandCreate event, the hand's new position, and the time of the update.
- Parameters:
-
[in] generator the generator that raised this event. [in] user The id of the hand that moved. [in] pPosition The new position of the relevant hand. [in] fTime Timestamp, in seconds. [in] pCookie A user-provided cookie that was given when registering to this event.
Constructor & Destructor Documentation
xn::HandsGenerator::HandsGenerator | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::HandsGenerator::HandsGenerator | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
XnStatus xn::HandsGenerator::Create | ( | Context & | context, |
Query * | pQuery = NULL , |
||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Creates a HandsGenerator node from available production node alternatives.
Remarks:
See Understanding the Create() method for a detailed description of this method.
const HandTouchingFOVEdgeCapability xn::HandsGenerator::GetHandTouchingFOVEdgeCap | ( | ) | const [inline] |
Gets an HandTouchingFOVEdgeCapability object for accessing hand touching FOV edge callbacks functionality. It is the application responsibility to check first if XN_CAPABILITY_HAND_TOUCHING_FOV_EDGE is supported by calling IsCapabilitySupported().
HandTouchingFOVEdgeCapability xn::HandsGenerator::GetHandTouchingFOVEdgeCap | ( | ) | [inline] |
Gets an HandTouchingFOVEdgeCapability object for accessing hand touching FOV edge callbacks functionality. It is the application responsibility to check first if XN_CAPABILITY_HAND_TOUCHING_FOV_EDGE is supported by calling IsCapabilitySupported().
XnStatus xn::HandsGenerator::RegisterHandCallbacks | ( | HandCreate | CreateCB, |
HandUpdate | UpdateCB, | ||
HandDestroy | DestroyCB, | ||
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers event handlers for the 'Hand Create', 'Hand Update' and 'Hand Destroy' events. Pass NULL for any event you don't want your application to receive.
- Parameters:
-
[in] CreateCB [Optional] Callback function to be invoked when the 'Hand Create' event is raised. [in] UpdateCB [Optional] Callback function to be invoked when the 'Hand Update' event is raised. [in] DestroyCB [Optional] Callback function to be invoked when the 'Hand Destroy' event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::HandsGenerator::SetSmoothing | ( | XnFloat | fSmoothingFactor | ) | [inline] |
Changes the smoothing factor.
- Parameters:
-
[in] fSmoothingFactor Smoothing factor, in the range 0..1. 0 Means no smoothing, 1 means infinite smoothing. Inside the range is generator dependent.
Recommended: 0.1
Remarks
The hand is identified from its physical depth. Since the human hand is not absolutely steady, the hand point generated may be too jittery to be used for controlling the user interface, e.g., pressing buttons. This method provides an algorithm to smooth the jitter. You provide the desired smoothing factor as a parameter.
Starts tracking at the specific position where the application expects a hand.
- Parameters:
-
[in] ptPosition The position at which there is a new hand
Remarks
Use the ptPosition
parameter to tell the method what is the expected location (in X-Y-Z coordinates) of the hand.
This expected location can be obtained from a 'Gesture Recognized' event, but it is not the only way of getting the hand's expected location.
Calling this method causes the HandCreate event to be raised when OpenNI recognizes the hand.
Stops tracking an existing hand that you are no longer interested in.
- Parameters:
-
[in] user Hand to stop tracking. This parameter is the ID of the hand.
Remarks
Typically, the application will stop tracking an existing hand that it is no longer interested in.
Calling this method causes the 'Hand Destroy' event to be raised when OpenNI destroys the hand.
XnStatus xn::HandsGenerator::StopTrackingAll | ( | ) | [inline] |
Stops tracking all existing hands.
Remarks
Calling this method causes the 'Hand Destroy' event to be raised when OpenNI destroys the hand.
void xn::HandsGenerator::UnregisterHandCallbacks | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters event handlers for the 'Hand Create', 'Hand Update' and 'Hand Destroy' events.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
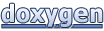