![]() |
OpenNI 1.5.4
|
#include <XnCppWrapper.h>
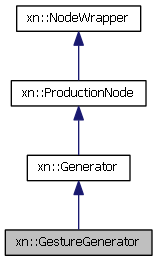
Classes | |
struct | GestureCookie |
struct | GestureIntermediateStageCompletedCookie |
struct | GestureReadyForNextIntermediateStageCookie |
Public Types | |
typedef void(* | GestureRecognized )(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pIDPosition, const XnPoint3D *pEndPosition, void *pCookie) |
typedef void(* | GestureProgress )(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, XnFloat fProgress, void *pCookie) |
typedef void(* | GestureIntermediateStageCompleted )(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie) |
typedef void(* | GestureReadyForNextIntermediateStage )(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie) |
Public Member Functions | |
GestureGenerator (XnNodeHandle hNode=NULL) | |
GestureGenerator (const NodeWrapper &other) | |
XnStatus | Create (Context &context, Query *pQuery=NULL, EnumerationErrors *pErrors=NULL) |
XnStatus | AddGesture (const XnChar *strGesture, XnBoundingBox3D *pArea) |
XnStatus | RemoveGesture (const XnChar *strGesture) |
XnStatus | GetAllActiveGestures (XnChar **astrGestures, XnUInt32 nNameLength, XnUInt16 &nGestures) const |
XnUInt16 | GetNumberOfAvailableGestures () const |
XnStatus | EnumerateAllGestures (XnChar **astrGestures, XnUInt32 nNameLength, XnUInt16 &nGestures) const |
XnBool | IsGestureAvailable (const XnChar *strGesture) const |
XnBool | IsGestureProgressSupported (const XnChar *strGesture) const |
XnStatus | RegisterGestureCallbacks (GestureRecognized RecognizedCB, GestureProgress ProgressCB, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterGestureCallbacks (XnCallbackHandle hCallback) |
XnStatus | RegisterToGestureChange (StateChangedHandler handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromGestureChange (XnCallbackHandle hCallback) |
XnStatus | RegisterToGestureIntermediateStageCompleted (GestureIntermediateStageCompleted handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromGestureIntermediateStageCompleted (XnCallbackHandle hCallback) |
XnStatus | RegisterToGestureReadyForNextIntermediateStage (GestureReadyForNextIntermediateStage handler, void *pCookie, XnCallbackHandle &hCallback) |
void | UnregisterFromGestureReadyForNextIntermediateStageCallbacks (XnCallbackHandle hCallback) |
Detailed Description
Purpose:A GesturesGenerator node recognizes certain hand gestures and raise corresponding events accordingly.
A gesture is a specific hand movement. The gesture generator node scans the FOV to detect gestures and generates the gesture data.
Usage: Instantiate to create a gesture generator node.
An example of a gesture is a specific hand movement. For example, the developer who builds a gesture generator node can decide that waving a hand can be a gesture; pushing can be a gesture. The developer can build an OpenNI gesture generator to support any number of gestures.
Overview with Sample of a Usage Scenario:
- Note:
- Everything in this introduction is just an example of usage; there is no obligation to use the gesture generator node in the particular way described here.
An application might want to know who is the person they should interact with. For example, if currently there are three people in the room, which hand should be tracked?
The application can use a specific gesture (such as waving to the camera) as a focus gesture, meaning the person who performs this gesture will be the one interacting with the application.
To do that, the application can use a GestureGenerator to detect that specific gesture and then use the position of that gesture to initialize the HandsGenerator, asking it to track the hand located at that position. (There is no inherent connection between the GestureGenerator and the HandsGenerator. Detecting a gesture and using that position to initialize the HandsGenerator is just an example of how applications can use the two).
Sample Process: Setting up a Gesture Generator
1. Creating a Gesture Generator
Assuming there is a gesture generator module (plug-in) installed on the computer, the application can create a gesture generator node and configure it to recognize gestures. As for many other types of generator nodes, use the Create() method of the GestureGenerator class to create a gesture generator node.
2. Enumerating generators
Now, once the application has created a gesture generator node, the application can find out which gestures are supported by enumerating all its gestures. The gestures are identified by their names, e.g., "Wave" and "Click".
The gesture generator node maintains a list of active gestures. An active gesture is a gesture for which the gesture generator is scanning the FOV in an attempt to recognize the gesture. Thus, to cause the gesture generator node to start scanning for a gesture, the application must add it to its list of active gestures.
In a typical application, the application detects one gesture - the focus gesture - and can then send its location to the hand generator node. The application typically will then not want to recognize further gestures and so it must remove the gesture from the list; further gestures in the FOV may cause confusion. For example, if one particular human user started to control the TV, that user would not want someone else "taking the control".
3. Recognizing Gestures
Every gesture has an identification point (the position where the gesture was identified - perhaps in retrospect) and an end point (the position in which the hand currently is), although in most cases, both points will be the same. The application must know the position of the end of the gesture movement; because that's where the hand really is at the time the application gets the 'Gesture Recognized' event. The gesture generator sends both positions with the 'Gesture Recognized' event to the application. The application can then pass the end position to the hand generator for tracking that hand.
Member Typedef Documentation
typedef void(* xn::GestureGenerator::GestureIntermediateStageCompleted)(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie) |
Callback for indication that an intermediate stage of a gesture has been completed.
Example of a callback function to handle the event:
void XN_CALLBACK_TYPE GestureIntermediateStageCompleted(GestureGenerator& generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie)
- Parameters:
-
[in] generator The node that raised the event. [in] strGesture The gesture that is on its way to being recognized. [in] pPosition The current position of the hand that is performing the gesture. [in] pCookie A user-provided cookie that was given when registering to this event.
Remarks
For different types of gestures there is a different point at which the GestureGenerator node can recognize that a gesture is in progress. For the 'Click' gesture, for example, the first step is the 'Push' movement (assuming the click gesture is defined as 'Push' and then 'Pull'). When the hand in the FOV completes the 'Push' the GestureGenerator node raises this event.
The difference between the 'Gesture Intermediate Stage Completed' event and the 'Gesture Progress' event is that the 'Gesture Intermediate Stage Completed' event does not refer to how much of the gesture is complete. It deals with breaking the gesture into smaller intermediate stages, and informing about them.
typedef void(* xn::GestureGenerator::GestureProgress)(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, XnFloat fProgress, void *pCookie) |
Callback for indication that a certain gesture is in progress.
Signals to the application that a gesture is expected to soon be recognized, that is, a gesture is in progress.
Example of a callback header to handle the event:
void XN_CALLBACK_TYPE GestureProgress (GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, XnFloat fProgress, void *pCookie)
- Parameters:
-
[in] generator The node that raised the event. [in] strGesture The gesture that is on its way to being recognized. [in] pPosition The current position of the hand that is performing the gesture. [in] fProgress The percentage of the gesture that was already performed. [in] pCookie A user-provided cookie that was given when registering to this event.
Gesture progress varies between different types of gestures. For the 'Click' gesture, for example, the click gesture's first step is the 'Push' movement (assuming the click gesture is defined as 'Push' and then 'Pull'). When the hand in the FOV completes the 'Push' the GestureGenerator node raises this event.
After the hand in the FOV completes the pull motion, the node sends the 'Gesture Recognized' event, as described above.
typedef void(* xn::GestureGenerator::GestureReadyForNextIntermediateStage)(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie) |
Callback for indication that the GestureGenerator node recognizes it is ready for the next intermediate stage. The next stage is specific to the gesture.
Example of a callback function to handle the event:
void XN_CALLBACK_TYPE GestureReadyForNextIntermediateStage(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pPosition, void *pCookie)
Remarks
The number of intermediate stages that there can be is implementation-dependent.
- Parameters:
-
[in] generator The node that raised the event. [in] strGesture The gesture that is on its way to being recognized. [in] pPosition The current position of the hand that is performing the gesture. [in] pCookie A user-provided cookie that was given when registering to this event.
typedef void(* xn::GestureGenerator::GestureRecognized)(GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pIDPosition, const XnPoint3D *pEndPosition, void *pCookie) |
Callback for the recognition of a gesture. Signals that the GestureGenerator node has recognized the named gesture in the scene.
Example of a callback header to handle the event:
void XN_CALLBACK_TYPE GestureRecognized (GestureGenerator &generator, const XnChar *strGesture, const XnPoint3D *pIDPosition, const XnPoint3D *pEndPosition, void *pCookie)
- Parameters:
-
[in] generator Node that raised the event. [in] strGesture Name of the gesture that was recognized. [in] pIDPosition X-Y-Z location coordinates of the hand point position in which the gesture was identified. [in] pEndPosition Position of the hand at the end of the gesture. This is the hand that performed the gesture. [in] pCookie User-provided cookie that was given when registering to this event.
Remarks
This event returns the identification point (the position where the gesture was identified - perhaps in retrospect) and an end point (the position in which the hand currently is), although in most cases, both points will be the same. The end position of the gesture movement is where the hand is located at the time the GestureGenerator recognized a complete gesture and raised the event.
Constructor & Destructor Documentation
xn::GestureGenerator::GestureGenerator | ( | XnNodeHandle | hNode = NULL | ) | [inline] |
Ctor
- Parameters:
-
[in] hNode Node handle
xn::GestureGenerator::GestureGenerator | ( | const NodeWrapper & | other | ) | [inline] |
Member Function Documentation
XnStatus xn::GestureGenerator::AddGesture | ( | const XnChar * | strGesture, |
XnBoundingBox3D * | pArea | ||
) | [inline] |
Activates the GestureGenerator node to start looking for the named gesture in the FOV. It does this by adding the gesture's name to the list of gestures that the GestureGenerator node actively scans for in the FOV. If an area is supplied, the gesture will only be recognized if it is detected in that area.
- Parameters:
-
[in] strGesture Name of the gesture to look for [in] pArea Area in which to look for the gesture (in real-world coordinates)
Remarks
This gesture must be one of the built-in gestures of the GestureGenerator node.
XnStatus xn::GestureGenerator::Create | ( | Context & | context, |
Query * | pQuery = NULL , |
||
EnumerationErrors * | pErrors = NULL |
||
) | [inline] |
Creates a gesture generator node from available production node alternatives.
Remarks
The application then uses the AddGesture() method in order to activate the gesture.
Typical gestures are focus gestures. Examples of focus gestures are 'Wave' and 'Click'.
See Understanding the Create() method for a detailed description of this method.
XnStatus xn::GestureGenerator::EnumerateAllGestures | ( | XnChar ** | astrGestures, |
XnUInt32 | nNameLength, | ||
XnUInt16 & | nGestures | ||
) | const [inline] |
Gets the names of all active gestures in this generator node.
- Parameters:
-
[out] astrGestures Preallocated memory, for the gesture names [in] nNameLength Memory size for each gesture name [in,out] nGestures The size of the preallocated memory. Changed to number of gestures
XnStatus xn::GestureGenerator::GetAllActiveGestures | ( | XnChar ** | astrGestures, |
XnUInt32 | nNameLength, | ||
XnUInt16 & | nGestures | ||
) | const [inline] |
Get the names of all gestures that are currently active in this generator node.
- Parameters:
-
[out] astrGestures Preallocated memory, for the gesture names [in] nNameLength Memory size for each gesture name [in,out] nGestures Inputs the total size of the preallocated memory. Outputs the total number of gestures in the list.
XnUInt16 xn::GestureGenerator::GetNumberOfAvailableGestures | ( | ) | const [inline] |
Get the number of all gestures available.
For full details and usage, see xnGetNumberOfAvailableGesturesXnBool xn::GestureGenerator::IsGestureAvailable | ( | const XnChar * | strGesture | ) | const [inline] |
Returns whether a specific gesture is available in this generator node.
- Parameters:
-
[in] strGesture Name of the gesture to check
XnBool xn::GestureGenerator::IsGestureProgressSupported | ( | const XnChar * | strGesture | ) | const [inline] |
Check if the specific gesture supports 'in progress' callbacks.
For full details and usage, see xnIsGestureProgressSupportedXnStatus xn::GestureGenerator::RegisterGestureCallbacks | ( | GestureRecognized | RecognizedCB, |
GestureProgress | ProgressCB, | ||
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers event handlers for the 'Gesture Recognized' and 'Gesture Progress' events. Pass NULL for any event the application does not need to receive.
- Parameters:
-
[in] RecognizedCB [Optional] Callback function to be invoked when the 'Gesture Recognized' event is raised. [in] ProgressCB [Optional] Callback function to be invoked when the 'Gesture Progress' event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::GestureGenerator::RegisterToGestureChange | ( | StateChangedHandler | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Gesture Change' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::GestureGenerator::RegisterToGestureIntermediateStageCompleted | ( | GestureIntermediateStageCompleted | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Gesture Intermediate Stage Completed' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::GestureGenerator::RegisterToGestureReadyForNextIntermediateStage | ( | GestureReadyForNextIntermediateStage | handler, |
void * | pCookie, | ||
XnCallbackHandle & | hCallback | ||
) | [inline] |
Registers an event handler for the 'Gesture Ready For Next Intermediate Stage' event.
- Parameters:
-
[in] handler Callback function to be invoked when the event is raised. [in] pCookie User's cookie, to be delivered to the callback. [out] hCallback Handle to the callback to be used for unregistering it.
For full details and usage of the parameters, see Registering to Events.
XnStatus xn::GestureGenerator::RemoveGesture | ( | const XnChar * | strGesture | ) | [inline] |
Disables the GestureGenerator from looking for the named gesture in the FOV. It does this by removing the gesture's name from the list of gestures that the GestureGenerator node actively scans for in the FOV.
- Parameters:
-
[in] strGesture The name of the gesture to not look for anymore.
void xn::GestureGenerator::UnregisterFromGestureChange | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Gesture Change' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::GestureGenerator::UnregisterFromGestureIntermediateStageCompleted | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Gesture Intermediate Stage Completed' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::GestureGenerator::UnregisterFromGestureReadyForNextIntermediateStageCallbacks | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters an event handler for the 'Gesture Ready For Next Intermediate Stage' event.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
void xn::GestureGenerator::UnregisterGestureCallbacks | ( | XnCallbackHandle | hCallback | ) | [inline] |
Unregisters the event handlers for the 'Gesture Recognized' and 'Gesture Progress' events.
- Parameters:
-
[in] hCallback Handle received from registration.
For full details and usage of the parameter, see Unregistering from Events .
The documentation for this class was generated from the following file:
Generated on Wed May 16 2012 10:16:07 for OpenNI 1.5.4 by
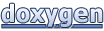