![]() |
OpenNI 1.5.4
|
Modules | |
Mirror Capability | |
Alternative View Point Capability | |
Frame-Sync Capability | |
Controlling Generation | |
The following functions allows controlling if a node is currently generating data or not. | |
XN_C_API XnStatus XN_C_DECL | xnStartGenerating (XnNodeHandle hInstance) |
XN_C_API XnBool XN_C_DECL | xnIsGenerating (XnNodeHandle hInstance) |
XN_C_API XnStatus XN_C_DECL | xnStopGenerating (XnNodeHandle hInstance) |
XN_C_API XnStatus XN_C_DECL | xnRegisterToGenerationRunningChange (XnNodeHandle hInstance, XnStateChangedHandler handler, void *pCookie, XnCallbackHandle *phCallback) |
XN_C_API void XN_C_DECL | xnUnregisterFromGenerationRunningChange (XnNodeHandle hInstance, XnCallbackHandle hCallback) |
Checking if new data is available | |
The following functions allows an application to know if and when a node has new data available. When new data is available, the node data is still not replaced, until explicitly done so using one of the update data functions. | |
XN_C_API XnStatus XN_C_DECL | xnRegisterToNewDataAvailable (XnNodeHandle hInstance, XnStateChangedHandler handler, void *pCookie, XnCallbackHandle *phCallback) |
XN_C_API void XN_C_DECL | xnUnregisterFromNewDataAvailable (XnNodeHandle hInstance, XnCallbackHandle hCallback) |
XN_C_API XnBool XN_C_DECL | xnIsNewDataAvailable (XnNodeHandle hInstance, XnUInt64 *pnTimestamp) |
Getting new data | |
XN_C_API XnStatus XN_C_DECL | xnWaitAndUpdateData (XnNodeHandle hInstance) |
Data properties | |
XN_C_API XnBool XN_C_DECL | xnIsDataNew (XnNodeHandle hInstance) |
XN_C_API const void *XN_C_DECL | xnGetData (XnNodeHandle hInstance) |
XN_C_API XnUInt32 XN_C_DECL | xnGetDataSize (XnNodeHandle hInstance) |
XN_C_API XnUInt64 XN_C_DECL | xnGetTimestamp (XnNodeHandle hInstance) |
XN_C_API XnUInt32 XN_C_DECL | xnGetFrameID (XnNodeHandle hInstance) |
Detailed Description
A Generator node is a production node that also generates continuous data. It supports all Production Node functions, and adds additional functions.
Function Documentation
XN_C_API const void* XN_C_DECL xnGetData | ( | XnNodeHandle | hInstance | ) |
Gets the current data.
- Parameters:
-
hInstance [in] A handle to the instance.
- Returns:
- NULL if this production node is not a generator.
XN_C_API XnUInt32 XN_C_DECL xnGetDataSize | ( | XnNodeHandle | hInstance | ) |
Gets the size of current data, in bytes.
- Parameters:
-
hInstance [in] A handle to the instance.
- Returns:
- (XnUInt32)-1 if this production node is not a generator.
XN_C_API XnUInt32 XN_C_DECL xnGetFrameID | ( | XnNodeHandle | hInstance | ) |
Gets the frame ID of current data.
- Parameters:
-
hInstance [in] A handle to the instance.
- Returns:
- (XnUInt32)-1 if this production node is not a generator.
XN_C_API XnUInt64 XN_C_DECL xnGetTimestamp | ( | XnNodeHandle | hInstance | ) |
Gets the timestamp of current data, in microseconds.
- Parameters:
-
hInstance [in] A handle to the instance.
- Returns:
- (XnUInt64)-1 if this production node is not a generator.
XN_C_API XnBool XN_C_DECL xnIsDataNew | ( | XnNodeHandle | hInstance | ) |
Checks whether current data is new. Meaning, did the data change on the last call to xnWaitAndUpdateAll(), xnWaitOneUpdateAll() or xnWaitAndUpdateData().
- Parameters:
-
hInstance [in] A handle to the instance.
XN_C_API XnBool XN_C_DECL xnIsGenerating | ( | XnNodeHandle | hInstance | ) |
Checks if this node is currently generating.
- Parameters:
-
hInstance [in] A handle to the instance.
- Returns:
- FALSE if this production node is not a generator.
XN_C_API XnBool XN_C_DECL xnIsNewDataAvailable | ( | XnNodeHandle | hInstance, |
XnUInt64 * | pnTimestamp | ||
) |
Checks whether this node has new data (and so a call to xnWaitAndUpdateData() will not block).
- Parameters:
-
hInstance [in] A handle to the instance. pnTimestamp [out] [Optional] If not NULL, will be filled with that data timestamp.
XN_C_API XnStatus XN_C_DECL xnRegisterToGenerationRunningChange | ( | XnNodeHandle | hInstance, |
XnStateChangedHandler | handler, | ||
void * | pCookie, | ||
XnCallbackHandle * | phCallback | ||
) |
Registers a callback function to be called when generation starts or stops.
- Parameters:
-
hInstance [in] A handle to the instance. handler [in] A pointer to a function that will be called when generation starts/stops. pCookie [in] A user cookie that will be passed to the callback function. phCallback [out] Optional. Will be filled with a handle to be passed to xnUnregisterFromGenerationRunningChange().
XN_C_API XnStatus XN_C_DECL xnRegisterToNewDataAvailable | ( | XnNodeHandle | hInstance, |
XnStateChangedHandler | handler, | ||
void * | pCookie, | ||
XnCallbackHandle * | phCallback | ||
) |
Registers a callback function to be called when new data is available.
- Parameters:
-
hInstance [in] A handle to the instance. handler [in] A pointer to a function that will be called when new data is available. pCookie [in] A user cookie that will be passed to the callback function. phCallback [out] Optional. Will be filled with a handle to be passed to xnUnregisterFromNewDataAvailable().
XN_C_API XnStatus XN_C_DECL xnStartGenerating | ( | XnNodeHandle | hInstance | ) |
Starts generation of the output. This will also cause all dependencies to start generating.
- Parameters:
-
hInstance [in] A handle to the instance to start generating.
- Returns:
- XN_STATUS_INVALID_OPERATION if this production node is not a generator.
XN_C_API XnStatus XN_C_DECL xnStopGenerating | ( | XnNodeHandle | hInstance | ) |
Stops generation of the output.
- Parameters:
-
hInstance [in] A handle to the instance to stop generating.
- Returns:
- XN_STATUS_INVALID_OPERATION if this production node is not a generator.
XN_C_API void XN_C_DECL xnUnregisterFromGenerationRunningChange | ( | XnNodeHandle | hInstance, |
XnCallbackHandle | hCallback | ||
) |
Unregisters a callback function which was registered using xnRegisterToGenerationRunningChange().
- Parameters:
-
hInstance [in] A handle to the instance. hCallback [in] The handle to the callback returned from xnRegisterToGenerationRunningChange().
XN_C_API void XN_C_DECL xnUnregisterFromNewDataAvailable | ( | XnNodeHandle | hInstance, |
XnCallbackHandle | hCallback | ||
) |
Unregisters a callback function which was registered using xnRegisterToNewDataAvailable().
- Parameters:
-
hInstance [in] A handle to the instance. hCallback [in] The handle to the callback returned from xnRegisterToNewDataAvailable().
XN_C_API XnStatus XN_C_DECL xnWaitAndUpdateData | ( | XnNodeHandle | hInstance | ) |
Updates the data to the latest available one. If needed, the call will block until new data is available.
- Parameters:
-
hInstance [in] A handle to the instance to be updated.
- Returns:
- XN_STATUS_INVALID_OPERATION if this production node is not a generator.
Generated on Wed May 16 2012 10:16:06 for OpenNI 1.5.4 by
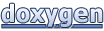