![]() |
OpenNI 1.5.4
|
XnArray.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XNARRAY_H__ 00023 #define __XNARRAY_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnOS.h> 00029 00030 //--------------------------------------------------------------------------- 00031 // Types 00032 //--------------------------------------------------------------------------- 00033 template <typename T> 00034 class XnArray 00035 { 00036 public: 00037 enum {BASE_SIZE = 8}; 00038 00040 typedef T* Iterator; 00041 00043 typedef const T* ConstIterator; 00044 00046 XnArray(XnUInt32 nBaseSize = BASE_SIZE) 00047 { 00048 Init(nBaseSize); 00049 } 00050 00052 XnArray(const XnArray& other) : m_pData(NULL), m_nSize(0), m_nAllocatedSize(0) 00053 { 00054 *this = other; 00055 } 00056 00058 virtual ~XnArray() 00059 { 00060 XN_DELETE_ARR(m_pData); 00061 } 00062 00064 XnArray& operator=(const XnArray& other) 00065 { 00066 CopyFrom(other); 00067 return *this; 00068 } 00069 00071 XnStatus CopyFrom(const XnArray& other) 00072 { 00073 if (this != &other) 00074 { 00075 XnStatus nRetVal = SetData(other.m_pData, other.m_nSize); 00076 XN_IS_STATUS_OK(nRetVal); 00077 } 00078 return XN_STATUS_OK; 00079 } 00080 00082 XnStatus SetData(const T* pData, XnUInt32 nSize) 00083 { 00084 Clear(); 00085 XnStatus nRetVal = SetSize(nSize); 00086 XN_IS_STATUS_OK(nRetVal); 00087 for (XnUInt32 i = 0; i < nSize; i++) 00088 { 00089 m_pData[i] = pData[i]; 00090 } 00091 return XN_STATUS_OK; 00092 } 00093 00095 const T* GetData() const 00096 { 00097 return m_pData; 00098 } 00099 00101 T* GetData() 00102 { 00103 return m_pData; 00104 } 00105 00108 XnStatus Reserve(XnUInt32 nReservedSize) 00109 { 00110 if (nReservedSize > m_nAllocatedSize) 00111 { 00112 //Calculate next power of 2 after nReservedSize 00113 nReservedSize--; 00114 nReservedSize = (nReservedSize >> 1) | nReservedSize; 00115 nReservedSize = (nReservedSize >> 2) | nReservedSize; 00116 nReservedSize = (nReservedSize >> 4) | nReservedSize; 00117 nReservedSize = (nReservedSize >> 8) | nReservedSize; 00118 nReservedSize = (nReservedSize >> 16) | nReservedSize; 00119 nReservedSize++; // nReservedSize is now the next power of 2. 00120 00121 //Allocate new data 00122 T* pNewData = XN_NEW_ARR(T, nReservedSize); 00123 XN_VALIDATE_ALLOC_PTR(pNewData); 00124 00125 //Copy old data into new data 00126 for (XnUInt32 i = 0; i < m_nSize; i++) 00127 { 00128 pNewData[i] = m_pData[i]; 00129 } 00130 00131 //Delete old data 00132 XN_DELETE_ARR(m_pData); 00133 00134 //Point to new data 00135 m_pData = pNewData; 00136 m_nAllocatedSize = nReservedSize; 00137 } 00138 return XN_STATUS_OK; 00139 } 00140 00142 XnBool IsEmpty() const 00143 { 00144 return (m_nSize == 0); 00145 } 00146 00148 XnUInt32 GetSize() const 00149 { 00150 return m_nSize; 00151 } 00152 00155 XnStatus SetSize(XnUInt32 nSize) 00156 { 00157 //TODO: Shrink allocated array if new size is smaller 00158 XnStatus nRetVal = SetMinSize(nSize); 00159 XN_IS_STATUS_OK(nRetVal); 00160 m_nSize = nSize; 00161 return XN_STATUS_OK; 00162 } 00163 00166 XnStatus SetSize(XnUInt32 nSize, const T& fillVal) 00167 { 00168 //TODO: Shrink allocated array if new size is smaller 00169 XnStatus nRetVal = SetMinSize(nSize, fillVal); 00170 XN_IS_STATUS_OK(nRetVal); 00171 m_nSize = nSize; 00172 return XN_STATUS_OK; 00173 } 00174 00178 XnStatus SetMinSize(XnUInt32 nSize) 00179 { 00180 if (nSize > m_nSize) 00181 { 00182 XnStatus nRetVal = Reserve(nSize); 00183 XN_IS_STATUS_OK(nRetVal); 00184 m_nSize = nSize; 00185 } 00186 return XN_STATUS_OK; 00187 } 00188 00192 XnStatus SetMinSize(XnUInt32 nSize, const T& fillVal) 00193 { 00194 if (nSize > m_nSize) 00195 { 00196 XnStatus nRetVal = Reserve(nSize); 00197 XN_IS_STATUS_OK(nRetVal); 00198 for (XnUInt32 i = m_nSize; i < nSize; i++) 00199 { 00200 m_pData[i] = fillVal; 00201 } 00202 m_nSize = nSize; 00203 } 00204 00205 return XN_STATUS_OK; 00206 } 00207 00210 XnUInt32 GetAllocatedSize() const 00211 { 00212 return m_nAllocatedSize; 00213 } 00214 00218 XnStatus Set(XnUInt32 nIndex, const T& val) 00219 { 00220 XnStatus nRetVal = SetMinSize(nIndex+1); 00221 XN_IS_STATUS_OK(nRetVal); 00222 m_pData[nIndex] = val; 00223 return XN_STATUS_OK; 00224 } 00225 00227 XnStatus Set(XnUInt32 nIndex, const T& val, const T& fillVal) 00228 { 00229 XnStatus nRetVal = SetMinSize(nIndex+1, fillVal); 00230 XN_IS_STATUS_OK(nRetVal); 00231 m_pData[nIndex] = val; 00232 return XN_STATUS_OK; 00233 } 00234 00236 XnStatus AddLast(const T& val) 00237 { 00238 return Set(m_nSize, val); 00239 } 00240 00242 XnStatus AddLast(const T* aValues, XnUInt32 nCount) 00243 { 00244 XN_VALIDATE_INPUT_PTR(aValues); 00245 XnUInt32 nOffset = GetSize(); 00246 XnStatus nRetVal = SetMinSize(GetSize() + nCount); 00247 XN_IS_STATUS_OK(nRetVal); 00248 for (XnUInt32 i = 0; i < nCount; ++i) 00249 { 00250 m_pData[nOffset + i] = aValues[i]; 00251 } 00252 return XN_STATUS_OK; 00253 } 00254 00256 void Clear() 00257 { 00258 XN_DELETE_ARR(m_pData); 00259 Init(); 00260 } 00261 00263 T& operator[](XnUInt32 nIndex) 00264 { 00265 XN_ASSERT(nIndex < m_nSize); 00266 return m_pData[nIndex]; 00267 } 00268 00270 const T& operator[](XnUInt32 nIndex) const 00271 { 00272 XN_ASSERT(nIndex < m_nSize); 00273 return m_pData[nIndex]; 00274 } 00275 00277 Iterator begin() 00278 { 00279 return &m_pData[0]; 00280 } 00281 00283 ConstIterator begin() const 00284 { 00285 return &m_pData[0]; 00286 } 00287 00289 Iterator end() 00290 { 00291 return m_pData + m_nSize; 00292 } 00293 00295 ConstIterator end() const 00296 { 00297 return m_pData + m_nSize; 00298 } 00299 00300 private: 00301 void Init(XnUInt32 nBaseSize = BASE_SIZE) 00302 { 00303 m_pData = XN_NEW_ARR(T, nBaseSize); 00304 m_nAllocatedSize = nBaseSize; 00305 m_nSize = 0; 00306 } 00307 00308 T* m_pData; 00309 XnUInt32 m_nSize; 00310 XnUInt32 m_nAllocatedSize; 00311 }; 00312 00313 #endif // __XNARRAY_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
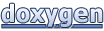