![]() |
OpenNI 1.5.4
|
XnUSBDevice.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef _XN_USB_DEVICE_H_ 00023 #define _XN_USB_DEVICE_H_ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include "XnPlatform.h" 00029 #include "XnStatus.h" 00030 00031 #if (XN_PLATFORM == XN_PLATFORM_WIN32) 00032 00033 #include <Win32/usb100.h> 00034 typedef USB_ENDPOINT_DESCRIPTOR XnUSBEndpointDescriptor; 00035 typedef USB_INTERFACE_DESCRIPTOR XnUSBInterfaceDescriptor; 00036 typedef USB_CONFIGURATION_DESCRIPTOR XnUSBConfigDescriptor; 00037 typedef USB_DEVICE_DESCRIPTOR XnUSBDeviceDescriptor; 00038 00039 #define USB_DT_CONFIG_SIZE 0 00040 #define USB_DT_CONFIG 0 00041 #define USB_CONFIG_ATT_ONE 0 00042 #define USB_DT_ENDPOINT_SIZE 0 00043 #define USB_DT_ENDPOINT 0 00044 #define USB_ENDPOINT_XFER_BULK 0 00045 #define USB_DT_INTERFACE_SIZE 0 00046 #define USB_DT_INTERFACE 0 00047 #define USB_CLASS_VENDOR_SPEC 0 00048 #define USB_DT_DEVICE_SIZE 0 00049 #define USB_DT_DEVICE 0 00050 00051 #elif (XN_PLATFORM == XN_PLATFORM_LINUX_X86 || XN_PLATFORM == XN_PLATFORM_LINUX_ARM) 00052 #include <linux/usb/ch9.h> 00053 typedef struct usb_endpoint_descriptor XnUSBEndpointDescriptor; 00054 typedef struct usb_interface_descriptor XnUSBInterfaceDescriptor; 00055 typedef struct usb_config_descriptor XnUSBConfigDescriptor; 00056 typedef struct usb_device_descriptor XnUSBDeviceDescriptor; 00057 #else 00058 #error "Unsupported Platform!" 00059 #endif 00060 00061 //--------------------------------------------------------------------------- 00062 // Structures & Enums 00063 //--------------------------------------------------------------------------- 00064 typedef enum XnUSBDeviceConnectionState 00065 { 00066 XN_USB_DEVICE_DISCONNECTED, 00067 XN_USB_DEVICE_CONNECTED, 00068 XN_USB_DEVICE_SUSPENDED, 00069 } XnUSBDeviceConnectionState; 00070 00071 typedef struct XnUSBStringDescriptor 00072 { 00073 XnUInt8 nID; 00074 const XnChar* strString; 00075 } XnUSBStringDescriptor; 00076 00077 typedef struct XnUSBInterfaceDescriptorHolder 00078 { 00079 XnUSBInterfaceDescriptor descriptor; 00080 XnUSBEndpointDescriptor** aEndpoints; 00081 } XnUSBInterfaceDescriptorHolder; 00082 00083 typedef struct XnUSBConfigDescriptorHolder 00084 { 00085 XnUSBConfigDescriptor descriptor; 00086 XnUSBInterfaceDescriptorHolder** aInterfaces; 00087 } XnUSBConfigDescriptorHolder; 00088 00089 typedef struct XnUSBDeviceDescriptorHolder 00090 { 00091 XnUSBDeviceDescriptor descriptor; 00092 XnUSBConfigDescriptorHolder** aConfigurations; 00093 XnUSBStringDescriptor* aStrings; 00094 XnUInt8 nStrings; 00095 } XnUSBDeviceDescriptorHolder; 00096 00097 struct XnUSBDevice; 00098 typedef struct XnUSBDevice XnUSBDevice; 00099 00100 typedef void (*XnUSBDeviceNewControlRequestCallback)(XnUSBDevice* pDevice, void* pCookie); 00101 typedef void (*XnUSBDeviceConnectivityChangedCallback)(XnUSBDevice* pDevice, XnUSBDeviceConnectionState state, void* pCookie); 00102 00103 //--------------------------------------------------------------------------- 00104 // API 00105 //--------------------------------------------------------------------------- 00106 XN_C_API XnStatus XN_C_DECL xnUSBDeviceInit(const XnUSBDeviceDescriptorHolder* pDeviceDescriptor, XnUInt32 nControlMessageMaxSize, XnUSBDevice** ppDevice); 00107 XN_C_API void XN_C_DECL xnUSBDeviceShutdown(XnUSBDevice* pDevice); 00108 XN_C_API XnBool XN_C_DECL xnUSBDeviceIsControlRequestPending(XnUSBDevice* pDevice); 00109 00110 //pnRequestSize is max size on input, actual size on output 00111 XN_C_API XnStatus XN_C_DECL xnUSBDeviceReceiveControlRequest(XnUSBDevice* pDevice, XnUChar* pBuffer, XnUInt32* pnRequestSize); 00112 XN_C_API XnStatus XN_C_DECL xnUSBDeviceSendControlReply(XnUSBDevice* pDevice, const XnUChar* pBuffer, XnUInt32 nReplySize); 00113 XN_C_API XnStatus XN_C_DECL xnUSBDeviceSetNewControlRequestCallback(XnUSBDevice* pDevice, XnUSBDeviceNewControlRequestCallback pFunc, void* pCookie); 00114 XN_C_API XnStatus XN_C_DECL xnUSBDeviceSetConnectivityChangedCallback(XnUSBDevice* pDevice, XnUSBDeviceConnectivityChangedCallback pFunc, void* pCookie); 00115 XN_C_API XnStatus XN_C_DECL xnUSBDeviceWriteEndpoint(XnUSBDevice* pDevice, XnUInt8 nAddress, const XnUChar* pData, XnUInt32 nDataSize); 00116 00117 #endif
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
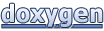