![]() |
OpenNI 1.5.4
|
XnTypes.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_TYPES_H__ 00023 #define __XN_TYPES_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnStatus.h> 00029 #include <XnOS.h> 00030 00031 //--------------------------------------------------------------------------- 00032 // Defines 00033 //--------------------------------------------------------------------------- 00035 #define XN_MAX_NAME_LENGTH 80 00036 00038 #define XN_MAX_CREATION_INFO_LENGTH 255 00039 00041 #define XN_MAX_LICENSE_LENGTH 255 00042 00044 #define XN_NODE_WAIT_FOR_DATA_TIMEOUT 2000 00045 00047 #define XN_VENDOR_OPEN_NI "OpenNI" 00048 00050 #define XN_FORMAT_NAME_ONI "oni" 00051 00053 #define XN_SCRIPT_FORMAT_XML "xml" 00054 00056 #define XN_PLAYBACK_SPEED_FASTEST 0.0 00057 00059 #define XN_AUTO_CONTROL XN_MIN_INT32 00060 00061 //--------------------------------------------------------------------------- 00062 // Forward Declarations 00063 //--------------------------------------------------------------------------- 00064 struct XnInternalNodeData; 00065 00066 //--------------------------------------------------------------------------- 00067 // Types 00068 //--------------------------------------------------------------------------- 00069 00070 #if XN_PLATFORM != XN_PLATFORM_ARC 00071 #pragma pack (push, 1) 00072 #endif 00073 00077 typedef struct XnContext XnContext; 00078 00082 typedef struct XnInternalNodeData* XnNodeHandle; 00083 00087 typedef XnUInt32 XnLockHandle; 00088 00092 typedef XnInt32 XnProductionNodeType; 00093 00097 typedef enum XnPredefinedProductionNodeType 00098 { 00100 XN_NODE_TYPE_INVALID = -1, 00101 00103 XN_NODE_TYPE_DEVICE = 1, 00104 00106 XN_NODE_TYPE_DEPTH = 2, 00107 00109 XN_NODE_TYPE_IMAGE = 3, 00110 00112 XN_NODE_TYPE_AUDIO = 4, 00113 00115 XN_NODE_TYPE_IR = 5, 00116 00118 XN_NODE_TYPE_USER = 6, 00119 00121 XN_NODE_TYPE_RECORDER = 7, 00122 00124 XN_NODE_TYPE_PLAYER = 8, 00125 00127 XN_NODE_TYPE_GESTURE = 9, 00128 00130 XN_NODE_TYPE_SCENE = 10, 00131 00133 XN_NODE_TYPE_HANDS = 11, 00134 00136 XN_NODE_TYPE_CODEC = 12, 00137 00139 XN_NODE_TYPE_PRODUCTION_NODE = 13, 00140 XN_NODE_TYPE_GENERATOR = 14, 00141 XN_NODE_TYPE_MAP_GENERATOR = 15, 00142 XN_NODE_TYPE_SCRIPT = 16, 00143 00144 XN_NODE_TYPE_FIRST_EXTENSION, 00145 00146 } XnPredefinedProductionNodeType; 00147 00151 typedef struct XnVersion 00152 { 00153 XnUInt8 nMajor; 00154 XnUInt8 nMinor; 00155 XnUInt16 nMaintenance; 00156 XnUInt32 nBuild; 00157 } XnVersion; 00158 00162 typedef struct XnProductionNodeDescription 00163 { 00165 XnProductionNodeType Type; 00167 XnChar strVendor[XN_MAX_NAME_LENGTH]; 00169 XnChar strName[XN_MAX_NAME_LENGTH]; 00171 XnVersion Version; 00172 } XnProductionNodeDescription; 00173 00177 typedef struct XnNodeInfo XnNodeInfo; 00178 00182 typedef struct XnNodeInfoListNode XnNodeInfoListNode; 00183 00187 typedef struct XnNodeInfoList XnNodeInfoList; 00188 00192 typedef struct XnNodeInfoListIterator 00193 { 00194 XnNodeInfoListNode* pCurrent; 00195 } XnNodeInfoListIterator; 00196 00197 typedef struct XnNodeQuery XnNodeQuery; 00198 00202 typedef struct XnLicense 00203 { 00205 XnChar strVendor[XN_MAX_NAME_LENGTH]; 00207 XnChar strKey[XN_MAX_LICENSE_LENGTH]; 00208 } XnLicense; 00209 00214 typedef struct XnEnumerationErrors XnEnumerationErrors; 00215 00219 typedef void* XnModuleNodeHandle; 00220 00227 typedef void (XN_CALLBACK_TYPE* XnStateChangedHandler)(XnNodeHandle hNode, void* pCookie); 00228 00235 typedef void (XN_CALLBACK_TYPE* XnErrorStateChangedHandler)(XnStatus errorState, void* pCookie); 00236 00242 typedef void (XN_CALLBACK_TYPE* XnFreeHandler)(const void* pData); 00243 00244 typedef void (XN_CALLBACK_TYPE* XnContextShuttingDownHandler)(XnContext* pContext, void* pCookie); 00245 00256 typedef void (XN_CALLBACK_TYPE* XnNodeCreationHandler)(XnContext* pContext, XnNodeHandle hCreatedNode, void* pCookie); 00257 00265 typedef void (XN_CALLBACK_TYPE* XnNodeDestructionHandler)(XnContext* pContext, const XnChar* strDestroyedNodeName, void* pCookie); 00266 00268 typedef void* XnCallbackHandle; 00269 00270 typedef struct XnModuleExportedProductionNodeInterface XnModuleExportedProductionNodeInterface; // Forward Declaration 00271 00272 //--------------------------------------------------------------------------- 00273 // 3D Vision Types 00274 //--------------------------------------------------------------------------- 00276 typedef XnUInt16 XnDepthPixel; 00277 00279 #define XN_DEPTH_NO_SAMPLE_VALUE ((XnDepthPixel)0) 00280 00282 typedef struct XnRGB24Pixel 00283 { 00284 XnUInt8 nRed; 00285 XnUInt8 nGreen; 00286 XnUInt8 nBlue; 00287 } XnRGB24Pixel; 00288 00290 typedef struct XnYUV422DoublePixel 00291 { 00292 XnUInt8 nU; 00293 XnUInt8 nY1; 00294 XnUInt8 nV; 00295 XnUInt8 nY2; 00296 } XnYUV422DoublePixel; 00297 00299 typedef XnUInt8 XnGrayscale8Pixel; 00300 00302 typedef XnUInt16 XnGrayscale16Pixel; 00303 00305 typedef XnGrayscale16Pixel XnIRPixel; 00306 00308 typedef XnUInt16 XnLabel; 00309 00310 //--------------------------------------------------------------------------- 00311 // Generators Capabilities 00312 //--------------------------------------------------------------------------- 00313 #define XN_CAPABILITY_EXTENDED_SERIALIZATION "ExtendedSerialization" 00314 #define XN_CAPABILITY_MIRROR "Mirror" 00315 #define XN_CAPABILITY_ALTERNATIVE_VIEW_POINT "AlternativeViewPoint" 00316 #define XN_CAPABILITY_CROPPING "Cropping" 00317 #define XN_CAPABILITY_USER_POSITION "UserPosition" 00318 #define XN_CAPABILITY_SKELETON "User::Skeleton" 00319 #define XN_CAPABILITY_POSE_DETECTION "User::PoseDetection" 00320 #define XN_CAPABILITY_LOCK_AWARE "LockAware" 00321 #define XN_CAPABILITY_ERROR_STATE "ErrorState" 00322 #define XN_CAPABILITY_FRAME_SYNC "FrameSync" 00323 #define XN_CAPABILITY_DEVICE_IDENTIFICATION "DeviceIdentification" 00324 #define XN_CAPABILITY_BRIGHTNESS "Brightness" 00325 #define XN_CAPABILITY_CONTRAST "Contrast" 00326 #define XN_CAPABILITY_HUE "Hue" 00327 #define XN_CAPABILITY_SATURATION "Saturation" 00328 #define XN_CAPABILITY_SHARPNESS "Sharpness" 00329 #define XN_CAPABILITY_GAMMA "Gamma" 00330 #define XN_CAPABILITY_COLOR_TEMPERATURE "ColorTemperature" 00331 #define XN_CAPABILITY_BACKLIGHT_COMPENSATION "BacklightCompensation" 00332 #define XN_CAPABILITY_GAIN "Gain" 00333 #define XN_CAPABILITY_PAN "Pan" 00334 #define XN_CAPABILITY_TILT "Tilt" 00335 #define XN_CAPABILITY_ROLL "Roll" 00336 #define XN_CAPABILITY_ZOOM "Zoom" 00337 #define XN_CAPABILITY_EXPOSURE "Exposure" 00338 #define XN_CAPABILITY_IRIS "Iris" 00339 #define XN_CAPABILITY_FOCUS "Focus" 00340 #define XN_CAPABILITY_LOW_LIGHT_COMPENSATION "LowLightCompensation" 00341 #define XN_CAPABILITY_ANTI_FLICKER "AntiFlicker" 00342 #define XN_CAPABILITY_HAND_TOUCHING_FOV_EDGE "Hands::HandTouchingFOVEdge" 00343 00344 // Backwards compatibility - typo was fixed 00345 #define XN_CAPABILITY_ANTI_FILCKER XN_CAPABILITY_ANTI_FLICKER 00346 00347 // deprecated pragma is only supported in Visual Studio 00348 #if (XN_PLATFORM == XN_PLATFORM_WIN32) 00349 #pragma deprecated("XN_CAPABILITY_ANTI_FILCKER") 00350 #endif 00351 00352 //--------------------------------------------------------------------------- 00353 // Generators API Structs 00354 //--------------------------------------------------------------------------- 00355 00356 #define XN_QQVGA_X_RES 160 00357 #define XN_QQVGA_Y_RES 120 00358 00359 #define XN_CGA_X_RES 320 00360 #define XN_CGA_Y_RES 200 00361 00362 #define XN_QVGA_X_RES 320 00363 #define XN_QVGA_Y_RES 240 00364 00365 #define XN_VGA_X_RES 640 00366 #define XN_VGA_Y_RES 480 00367 00368 #define XN_SVGA_X_RES 800 00369 #define XN_SVGA_Y_RES 600 00370 00371 #define XN_XGA_X_RES 1024 00372 #define XN_XGA_Y_RES 768 00373 00374 #define XN_720P_X_RES 1280 00375 #define XN_720P_Y_RES 720 00376 00377 #define XN_SXGA_X_RES 1280 00378 #define XN_SXGA_Y_RES 1024 00379 00380 #define XN_UXGA_X_RES 1600 00381 #define XN_UXGA_Y_RES 1200 00382 00383 #define XN_1080P_X_RES 1920 00384 #define XN_1080P_Y_RES 1080 00385 00386 #define XN_QCIF_X_RES 176 00387 #define XN_QCIF_Y_RES 144 00388 00389 #define XN_240P_X_RES 423 00390 #define XN_240P_Y_RES 240 00391 00392 #define XN_CIF_X_RES 352 00393 #define XN_CIF_Y_RES 288 00394 00395 #define XN_WVGA_X_RES 640 00396 #define XN_WVGA_Y_RES 360 00397 00398 #define XN_480P_X_RES 864 00399 #define XN_480P_Y_RES 480 00400 00401 #define XN_576P_X_RES 1024 00402 #define XN_576P_Y_RES 576 00403 00404 #define XN_DV_X_RES 960 00405 #define XN_DV_Y_RES 720 00406 00407 typedef enum XnResolution 00408 { 00409 XN_RES_CUSTOM = 0, 00410 XN_RES_QQVGA = 1, 00411 XN_RES_CGA = 2, 00412 XN_RES_QVGA = 3, 00413 XN_RES_VGA = 4, 00414 XN_RES_SVGA = 5, 00415 XN_RES_XGA = 6, 00416 XN_RES_720P = 7, 00417 XN_RES_SXGA = 8, 00418 XN_RES_UXGA = 9, 00419 XN_RES_1080P = 10, 00420 XN_RES_QCIF = 11, 00421 XN_RES_240P = 12, 00422 XN_RES_CIF = 13, 00423 XN_RES_WVGA = 14, 00424 XN_RES_480P = 15, 00425 XN_RES_576P = 16, 00426 XN_RES_DV = 17, 00427 } XnResolution; 00428 00432 typedef struct XnMapOutputMode 00433 { 00435 XnUInt32 nXRes; 00437 XnUInt32 nYRes; 00439 XnUInt32 nFPS; 00440 } XnMapOutputMode; 00441 00442 typedef enum XnSampleRate 00443 { 00444 XN_SAMPLE_RATE_8K = 8000, 00445 XN_SAMPLE_RATE_11K = 11025, 00446 XN_SAMPLE_RATE_12K = 12000, 00447 XN_SAMPLE_RATE_16K = 16000, 00448 XN_SAMPLE_RATE_22K = 22050, 00449 XN_SAMPLE_RATE_24K = 24000, 00450 XN_SAMPLE_RATE_32K = 32000, 00451 XN_SAMPLE_RATE_44K = 44100, 00452 XN_SAMPLE_RATE_48K = 48000, 00453 } XnSampleRate; 00454 00455 typedef struct XnWaveOutputMode 00456 { 00457 XnUInt32 nSampleRate; 00458 XnUInt16 nBitsPerSample; 00459 XnUInt8 nChannels; 00460 } XnWaveOutputMode; 00461 00465 typedef struct XnVector3D 00466 { 00467 XnFloat X; 00468 XnFloat Y; 00469 XnFloat Z; 00470 } XnVector3D; 00471 00472 typedef XnVector3D XnPoint3D; 00473 00477 typedef struct XnBoundingBox3D 00478 { 00479 XnPoint3D LeftBottomNear; 00480 XnPoint3D RightTopFar; 00481 } XnBoundingBox3D; 00482 00486 typedef struct XnCropping 00487 { 00489 XnBool bEnabled; 00491 XnUInt16 nXOffset; 00493 XnUInt16 nYOffset; 00495 XnUInt16 nXSize; 00497 XnUInt16 nYSize; 00498 } XnCropping; 00499 00503 typedef struct XnFieldOfView 00504 { 00506 XnDouble fHFOV; 00508 XnDouble fVFOV; 00509 } XnFieldOfView; 00510 00511 typedef enum XnPixelFormat 00512 { 00513 XN_PIXEL_FORMAT_RGB24 = 1, 00514 XN_PIXEL_FORMAT_YUV422 = 2, 00515 XN_PIXEL_FORMAT_GRAYSCALE_8_BIT = 3, 00516 XN_PIXEL_FORMAT_GRAYSCALE_16_BIT = 4, 00517 XN_PIXEL_FORMAT_MJPEG = 5, 00518 } XnPixelFormat; 00519 00520 typedef struct XnSupportedPixelFormats 00521 { 00522 XnBool m_bRGB24 : 1; 00523 XnBool m_bYUV422 : 1; 00524 XnBool m_bGrayscale8Bit : 1; 00525 XnBool m_bGrayscale16Bit : 1; 00526 XnBool m_bMJPEG : 1; 00527 XnUInt m_nPadding : 3; 00528 XnUInt m_nReserved : 24; 00529 } XnSupportedPixelFormats; 00530 00531 typedef enum XnPlayerSeekOrigin 00532 { 00533 XN_PLAYER_SEEK_SET = 0, 00534 XN_PLAYER_SEEK_CUR = 1, 00535 XN_PLAYER_SEEK_END = 2, 00536 } XnPlayerSeekOrigin; 00537 00538 typedef enum XnPowerLineFrequency 00539 { 00540 XN_POWER_LINE_FREQUENCY_OFF = 0, 00541 XN_POWER_LINE_FREQUENCY_50_HZ = 50, 00542 XN_POWER_LINE_FREQUENCY_60_HZ = 60, 00543 } XnPowerLineFrequency; 00544 00545 // User 00546 typedef XnUInt32 XnUserID; 00547 typedef XnFloat XnConfidence; 00548 00550 typedef struct XnMatrix3X3 00551 { 00553 XnFloat elements[9]; 00554 } XnMatrix3X3; 00555 00560 typedef struct XnPlane3D 00561 { 00563 XnVector3D vNormal; 00564 00566 XnPoint3D ptPoint; 00567 } XnPlane3D; 00568 00573 typedef struct XnSkeletonJointPosition 00574 { 00576 XnVector3D position; 00577 00579 XnConfidence fConfidence; 00580 } XnSkeletonJointPosition; 00581 00589 typedef struct XnSkeletonJointOrientation 00590 { 00592 XnMatrix3X3 orientation; 00594 XnConfidence fConfidence; 00595 } XnSkeletonJointOrientation; 00596 00600 typedef struct XnSkeletonJointTransformation 00601 { 00603 XnSkeletonJointPosition position; 00605 XnSkeletonJointOrientation orientation; 00606 } XnSkeletonJointTransformation; 00607 00611 typedef enum XnSkeletonJoint 00612 { 00613 XN_SKEL_HEAD = 1, 00614 XN_SKEL_NECK = 2, 00615 XN_SKEL_TORSO = 3, 00616 XN_SKEL_WAIST = 4, 00617 00618 XN_SKEL_LEFT_COLLAR = 5, 00619 XN_SKEL_LEFT_SHOULDER = 6, 00620 XN_SKEL_LEFT_ELBOW = 7, 00621 XN_SKEL_LEFT_WRIST = 8, 00622 XN_SKEL_LEFT_HAND = 9, 00623 XN_SKEL_LEFT_FINGERTIP =10, 00624 00625 XN_SKEL_RIGHT_COLLAR =11, 00626 XN_SKEL_RIGHT_SHOULDER =12, 00627 XN_SKEL_RIGHT_ELBOW =13, 00628 XN_SKEL_RIGHT_WRIST =14, 00629 XN_SKEL_RIGHT_HAND =15, 00630 XN_SKEL_RIGHT_FINGERTIP =16, 00631 00632 XN_SKEL_LEFT_HIP =17, 00633 XN_SKEL_LEFT_KNEE =18, 00634 XN_SKEL_LEFT_ANKLE =19, 00635 XN_SKEL_LEFT_FOOT =20, 00636 00637 XN_SKEL_RIGHT_HIP =21, 00638 XN_SKEL_RIGHT_KNEE =22, 00639 XN_SKEL_RIGHT_ANKLE =23, 00640 XN_SKEL_RIGHT_FOOT =24 00641 } XnSkeletonJoint; 00642 00644 typedef enum XnSkeletonProfile 00645 { 00647 XN_SKEL_PROFILE_NONE = 1, 00648 00650 XN_SKEL_PROFILE_ALL = 2, 00651 00653 XN_SKEL_PROFILE_UPPER = 3, 00654 00656 XN_SKEL_PROFILE_LOWER = 4, 00657 00659 XN_SKEL_PROFILE_HEAD_HANDS = 5, 00660 } XnSkeletonProfile; 00661 00663 typedef enum XnPoseDetectionStatus 00664 { 00665 XN_POSE_DETECTION_STATUS_OK = 0, 00666 XN_POSE_DETECTION_STATUS_NO_USER = 1, 00667 XN_POSE_DETECTION_STATUS_TOP_FOV = 2, 00668 XN_POSE_DETECTION_STATUS_SIDE_FOV = 3, 00669 XN_POSE_DETECTION_STATUS_ERROR = 4, 00670 XN_POSE_DETECTION_STATUS_NO_TRACKING = 5 00671 } XnPoseDetectionStatus; 00672 00673 00675 typedef enum XnPoseDetectionState 00676 { 00677 XN_POSE_DETECTION_STATE_IN_POSE =0, 00678 XN_POSE_DETECTION_STATE_OUT_OF_POSE =1, 00679 XN_POSE_DETECTION_STATE_UNDEFINED =2 00680 } XnPoseDetectionState; 00682 typedef enum XnCalibrationStatus 00683 { 00684 XN_CALIBRATION_STATUS_OK = 0, 00685 XN_CALIBRATION_STATUS_NO_USER = 1, 00686 XN_CALIBRATION_STATUS_ARM = 2, 00687 XN_CALIBRATION_STATUS_LEG = 3, 00688 XN_CALIBRATION_STATUS_HEAD = 4, 00689 XN_CALIBRATION_STATUS_TORSO = 5, 00690 XN_CALIBRATION_STATUS_TOP_FOV = 6, 00691 XN_CALIBRATION_STATUS_SIDE_FOV = 7, 00692 XN_CALIBRATION_STATUS_POSE = 8, 00693 XN_CALIBRATION_STATUS_MANUAL_ABORT = 9, 00694 XN_CALIBRATION_STATUS_MANUAL_RESET = 10, 00695 XN_CALIBRATION_STATUS_TIMEOUT_FAIL = 11 00696 } XnCalibrationStatus; 00697 00698 typedef enum XnDirection 00699 { 00700 XN_DIRECTION_ILLEGAL = 0, 00701 XN_DIRECTION_LEFT = 1, 00702 XN_DIRECTION_RIGHT = 2, 00703 XN_DIRECTION_UP = 3, 00704 XN_DIRECTION_DOWN = 4, 00705 XN_DIRECTION_FORWARD = 5, 00706 XN_DIRECTION_BACKWARD = 6, 00707 } XnDirection; 00708 00709 // User 00717 typedef void (XN_CALLBACK_TYPE* XnUserHandler)(XnNodeHandle hNode, XnUserID user, void* pCookie); 00718 00719 // Hands 00729 typedef void (XN_CALLBACK_TYPE* XnHandCreate)(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 00730 00740 typedef void (XN_CALLBACK_TYPE* XnHandUpdate)(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 00741 00750 typedef void (XN_CALLBACK_TYPE* XnHandDestroy)(XnNodeHandle hNode, XnUserID user, XnFloat fTime, void* pCookie); 00751 00762 typedef void (XN_CALLBACK_TYPE* XnHandTouchingFOVEdge)(XnNodeHandle hNode, XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, XnDirection eDir, void* pCookie); 00763 // Gesture Module 00773 typedef void (XN_CALLBACK_TYPE* XnGestureRecognized)(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pIDPosition, const XnPoint3D* pEndPosition, void* pCookie); 00783 typedef void (XN_CALLBACK_TYPE* XnGestureProgress)(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, XnFloat fProgress, void* pCookie); 00784 00785 typedef void (XN_CALLBACK_TYPE* XnGestureIntermediateStageCompleted)(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 00786 typedef void (XN_CALLBACK_TYPE* XnGestureReadyForNextIntermediateStage)(XnNodeHandle hNode, const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 00787 00788 // Skeleton 00796 typedef void (XN_CALLBACK_TYPE* XnCalibrationStart)(XnNodeHandle hNode, XnUserID user, void* pCookie); 00805 typedef void (XN_CALLBACK_TYPE* XnCalibrationEnd)(XnNodeHandle hNode, XnUserID user, XnBool bSuccess, void* pCookie); 00806 00807 typedef void (XN_CALLBACK_TYPE* XnCalibrationInProgress)(XnNodeHandle hNode, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 00808 typedef void (XN_CALLBACK_TYPE* XnCalibrationComplete)(XnNodeHandle hNode, XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 00809 00810 // Pose Detection 00819 typedef void (XN_CALLBACK_TYPE* XnPoseDetectionCallback)(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, void* pCookie); 00820 00821 typedef void (XN_CALLBACK_TYPE* XnPoseDetectionInProgress)(XnNodeHandle hNode, const XnChar* strPose, XnUserID user, XnPoseDetectionStatus poseDetectionError, void* pCookie); 00822 00823 //--------------------------------------------------------------------------- 00824 // Recorder Types 00825 //--------------------------------------------------------------------------- 00826 00832 typedef enum XnRecordMedium 00833 { 00835 XN_RECORD_MEDIUM_FILE = 0, 00836 } XnRecordMedium; 00837 00839 typedef XnUInt32 XnCodecID; 00840 00842 #define XN_CODEC_ID(c1, c2, c3, c4) (XnCodecID)((c4 << 24) | (c3 << 16) | (c2 << 8) | c1) 00843 00849 typedef struct XnRecorderOutputStreamInterface 00850 { 00856 XnStatus (XN_CALLBACK_TYPE* Open)(void* pCookie); 00857 00866 XnStatus (XN_CALLBACK_TYPE* Write)(void* pCookie, const XnChar* strNodeName, 00867 const void* pData, XnUInt32 nSize); 00868 00876 XnStatus (XN_CALLBACK_TYPE* Seek)(void* pCookie, XnOSSeekType seekType, const XnInt32 nOffset); 00877 00886 XnUInt32 (XN_CALLBACK_TYPE* Tell)(void* pCookie); 00887 00893 void (XN_CALLBACK_TYPE* Close)(void* pCookie); 00894 00902 XnStatus (XN_CALLBACK_TYPE* Seek64)(void* pCookie, XnOSSeekType seekType, const XnInt64 nOffset); 00903 00912 XnUInt64 (XN_CALLBACK_TYPE* Tell64)(void* pCookie); 00913 00914 } XnRecorderOutputStreamInterface; 00915 00921 typedef struct XnPlayerInputStreamInterface 00922 { 00928 XnStatus (XN_CALLBACK_TYPE* Open)(void* pCookie); 00929 00939 XnStatus (XN_CALLBACK_TYPE* Read)(void* pCookie, void* pBuffer, XnUInt32 nSize, XnUInt32* pnBytesRead); 00940 00948 XnStatus (XN_CALLBACK_TYPE* Seek)(void* pCookie, XnOSSeekType seekType, const XnInt32 nOffset); 00949 00957 XnUInt32 (XN_CALLBACK_TYPE* Tell)(void* pCookie); 00958 00964 void (XN_CALLBACK_TYPE* Close)(void* pCookie); 00965 00973 XnStatus (XN_CALLBACK_TYPE* Seek64)(void* pCookie, XnOSSeekType seekType, const XnInt64 nOffset); 00974 00983 XnUInt64 (XN_CALLBACK_TYPE* Tell64)(void* pCookie); 00984 00985 } XnPlayerInputStreamInterface; 00986 00990 typedef struct XnNodeNotifications 00991 { 00998 XnStatus (XN_CALLBACK_TYPE* OnNodeAdded) 00999 (void* pCookie, const XnChar* strNodeName, XnProductionNodeType type, 01000 XnCodecID compression); 01001 01008 XnStatus (XN_CALLBACK_TYPE* OnNodeRemoved) 01009 (void* pCookie, const XnChar* strNodeName); 01010 01019 XnStatus (XN_CALLBACK_TYPE* OnNodeIntPropChanged) 01020 (void* pCookie, const XnChar* strNodeName, 01021 const XnChar* strPropName, XnUInt64 nValue); 01022 01031 XnStatus (XN_CALLBACK_TYPE* OnNodeRealPropChanged) 01032 (void* pCookie, const XnChar* strNodeName, 01033 const XnChar* strPropName, XnDouble dValue); 01034 01043 XnStatus (XN_CALLBACK_TYPE* OnNodeStringPropChanged) 01044 (void* pCookie, const XnChar* strNodeName, 01045 const XnChar* strPropName, const XnChar* strValue); 01046 01056 XnStatus (XN_CALLBACK_TYPE* OnNodeGeneralPropChanged) 01057 (void* pCookie, const XnChar* strNodeName, 01058 const XnChar* strPropName, XnUInt32 nBufferSize, const void* pBuffer); 01059 01066 XnStatus (XN_CALLBACK_TYPE* OnNodeStateReady) 01067 (void* pCookie, const XnChar* strNodeName); 01068 01078 XnStatus (XN_CALLBACK_TYPE* OnNodeNewData) 01079 (void* pCookie, const XnChar* strNodeName, 01080 XnUInt64 nTimeStamp, XnUInt32 nFrame, const void* pData, XnUInt32 nSize); 01081 01082 } XnNodeNotifications; 01083 01085 typedef struct XnUInt32XYPair 01086 { 01087 XnUInt32 X; 01088 XnUInt32 Y; 01089 } XnUInt32XYPair; 01090 01092 typedef struct XnOutputMetaData 01093 { 01095 XnUInt64 nTimestamp; 01096 01098 XnUInt32 nFrameID; 01099 01101 XnUInt32 nDataSize; 01102 01104 XnBool bIsNew; 01105 01106 } XnOutputMetaData; 01107 01109 typedef struct XnMapMetaData 01110 { 01112 XnOutputMetaData* pOutput; 01113 01115 XnUInt32XYPair Res; 01116 01118 XnUInt32XYPair Offset; 01119 01121 XnUInt32XYPair FullRes; 01122 01124 XnPixelFormat PixelFormat; 01125 01127 XnUInt32 nFPS; 01128 } XnMapMetaData; 01129 01131 typedef struct XnDepthMetaData 01132 { 01134 XnMapMetaData* pMap; 01135 01137 const XnDepthPixel* pData; 01138 01140 XnDepthPixel nZRes; 01141 } XnDepthMetaData; 01142 01144 typedef struct XnImageMetaData 01145 { 01147 XnMapMetaData* pMap; 01148 01150 const XnUInt8* pData; 01151 } XnImageMetaData; 01152 01154 typedef struct XnIRMetaData 01155 { 01157 XnMapMetaData* pMap; 01158 01160 const XnIRPixel* pData; 01161 } XnIRMetaData; 01162 01163 typedef struct XnAudioMetaData 01164 { 01166 XnOutputMetaData* pOutput; 01167 01169 XnWaveOutputMode Wave; 01170 01172 const XnUInt8* pData; 01173 } XnAudioMetaData; 01174 01175 typedef struct XnSceneMetaData 01176 { 01178 XnMapMetaData* pMap; 01179 01181 const XnLabel* pData; 01182 } XnSceneMetaData; 01183 01184 #if XN_PLATFORM != XN_PLATFORM_ARC 01185 #pragma pack (pop) 01186 #endif 01187 01188 #endif //__XN_TYPES_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
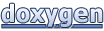