![]() |
OpenNI 1.5.4
|
XnThreadSafeQueue.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_THREAD_SAFE_QUEUE_H__ 00023 #define __XN_THREAD_SAFE_QUEUE_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnQueue.h> 00029 #include <XnOS.h> 00030 00031 //--------------------------------------------------------------------------- 00032 // Types 00033 //--------------------------------------------------------------------------- 00037 class XnThreadSafeQueue : public XnQueue 00038 { 00039 public: 00040 XnThreadSafeQueue() : m_hLock(NULL) {} 00041 00042 ~XnThreadSafeQueue() 00043 { 00044 xnOSCloseCriticalSection(&m_hLock); 00045 } 00046 00047 XnStatus Init() 00048 { 00049 XnStatus nRetVal = XN_STATUS_OK; 00050 00051 nRetVal = xnOSCreateCriticalSection(&m_hLock); 00052 XN_IS_STATUS_OK(nRetVal); 00053 00054 return (XN_STATUS_OK); 00055 } 00056 00057 XnStatus Push(XnValue const& value) 00058 { 00059 XnStatus nRetVal = XN_STATUS_OK; 00060 00061 nRetVal = xnOSEnterCriticalSection(&m_hLock); 00062 XN_IS_STATUS_OK(nRetVal); 00063 00064 nRetVal = XnQueue::Push(value); 00065 xnOSLeaveCriticalSection(&m_hLock); 00066 00067 return nRetVal; 00068 } 00069 00070 XnStatus Pop(XnValue& value) 00071 { 00072 XnStatus nRetVal = XN_STATUS_OK; 00073 00074 nRetVal = xnOSEnterCriticalSection(&m_hLock); 00075 XN_IS_STATUS_OK(nRetVal); 00076 00077 nRetVal = XnQueue::Pop(value); 00078 xnOSLeaveCriticalSection(&m_hLock); 00079 00080 return nRetVal; 00081 } 00082 00083 XnUInt32 Size() const 00084 { 00085 xnOSEnterCriticalSection(&m_hLock); 00086 XnUInt32 nSize = XnQueue::Size(); 00087 xnOSLeaveCriticalSection(&m_hLock); 00088 return (nSize); 00089 } 00090 00091 private: 00092 // NOTE: we declare the lock as mutable, as it may change on const methods. 00093 mutable XN_CRITICAL_SECTION_HANDLE m_hLock; 00094 }; 00095 00101 #define XN_DECLARE_THREAD_SAFE_QUEUE_WITH_TRANSLATOR_DECL(decl, Type, ClassName, Translator) \ 00102 class decl ClassName : public XnThreadSafeQueue \ 00103 { \ 00104 public: \ 00105 ~ClassName() \ 00106 { \ 00107 /* We do this using Pop() to make sure memory is freed. */ \ 00108 Type dummy; \ 00109 while (Size() != 0) \ 00110 Pop(dummy); \ 00111 } \ 00112 XnStatus Push(Type const& value) \ 00113 { \ 00114 XnValue val = Translator::CreateValueCopy(value); \ 00115 XnStatus nRetVal = XnThreadSafeQueue::Push(val); \ 00116 if (nRetVal != XN_STATUS_OK) \ 00117 { \ 00118 Translator::FreeValue(val); \ 00119 return (nRetVal); \ 00120 } \ 00121 return XN_STATUS_OK; \ 00122 } \ 00123 XnStatus Pop(Type& value) \ 00124 { \ 00125 XnValue val; \ 00126 XnStatus nRetVal = XnThreadSafeQueue::Pop(val); \ 00127 if (nRetVal != XN_STATUS_OK) return (nRetVal); \ 00128 value = Translator::GetFromValue(val); \ 00129 Translator::FreeValue(val); \ 00130 return XN_STATUS_OK; \ 00131 } \ 00132 }; 00133 00139 #define XN_DECLARE_THREAD_SAFE_QUEUE_WITH_TRANSLATOR(Type, ClassName, Translator) \ 00140 XN_DECLARE_THREAD_SAFE_QUEUE_WITH_TRANSLATOR_DECL(, Type, ClassName, Translator) 00141 00146 #define XN_DECLARE_THREAD_SAFE_QUEUE_DECL(decl, Type, ClassName) \ 00147 XN_DECLARE_DEFAULT_VALUE_TRANSLATOR_DECL(decl, Type, XN_DEFAULT_TRANSLATOR_NAME(ClassName)) \ 00148 XN_DECLARE_THREAD_SAFE_QUEUE_WITH_TRANSLATOR_DECL(decl, Type, ClassName, XN_DEFAULT_TRANSLATOR_NAME(ClassName)) 00149 00153 #define XN_DECLARE_THREAD_SAFE_QUEUE(Type, ClassName) \ 00154 XN_DECLARE_THREAD_SAFE_QUEUE_DECL(, Type, ClassName) 00155 00156 #endif //__XN_THREAD_SAFE_QUEUE_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
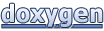