![]() |
OpenNI 1.5.4
|
XnStringsHashT.h
Go to the documentation of this file.
00001 #ifndef _XN_STRINGS_HASH_T_H_ 00002 #define _XN_STRINGS_HASH_T_H_ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include "XnHashT.h" 00008 00009 //--------------------------------------------------------------------------- 00010 // Code 00011 //--------------------------------------------------------------------------- 00012 class XnStringsHashKeyManager 00013 { 00014 public: 00015 static XnHashCode Hash(const XnChar* const& key) 00016 { 00017 XnUInt32 nCRC = 0; 00018 xnOSStrCRC32(key, &nCRC); 00019 00020 // convert from UINT32 to XnHashValue 00021 return nCRC % (1 << (sizeof(XnHashCode)*8)); 00022 } 00023 00024 static XnInt32 Compare(const XnChar* const& key1, const XnChar* const& key2) 00025 { 00026 return strcmp(key1, key2); 00027 } 00028 }; 00029 00030 template<class TValue> 00031 class XnStringsNodeAllocator 00032 { 00033 public: 00034 typedef XnKeyValuePair<const XnChar*, TValue> TPair; 00035 typedef XnLinkedNodeT<TPair> TLinkedNode; 00036 00037 static TLinkedNode* Allocate(TPair const& pair) 00038 { 00039 XnChar* pKeyCopy = xnOSStrDup(pair.Key()); 00040 if (pKeyCopy == NULL) 00041 { 00042 return NULL; 00043 } 00044 00045 return XN_NEW(TLinkedNode, TPair(pKeyCopy, pair.Value())); 00046 } 00047 00048 static void Deallocate(TLinkedNode* pNode) 00049 { 00050 XN_ASSERT(pNode != NULL); 00051 XN_ASSERT(pNode->value.Key() != NULL); 00052 00053 xnOSFree(pNode->value.Key()); 00054 XN_DELETE(pNode); 00055 } 00056 }; 00057 00058 template<class TValue> 00059 class XnStringsHashT : public XnHashT<const XnChar*, TValue, XnStringsHashKeyManager, XnStringsNodeAllocator<TValue> > 00060 { 00061 typedef XnHashT<const XnChar*, TValue, XnStringsHashKeyManager, XnStringsNodeAllocator<TValue> > Base; 00062 00063 public: 00064 XnStringsHashT() : Base() {} 00065 00066 XnStringsHashT(const XnStringsHashT& other) : Base() 00067 { 00068 *this = other; 00069 } 00070 00071 XnStringsHashT& operator=(const XnStringsHashT& other) 00072 { 00073 Base::operator=(other); 00074 // no other members 00075 return *this; 00076 } 00077 }; 00078 00079 class XnStringsSet : public XnStringsHashT<void*> 00080 { 00081 typedef XnStringsHashT<void*> Base; 00082 00083 public: 00084 XnStatus Set(const XnChar* key) 00085 { 00086 return Base::Set(key, NULL); 00087 } 00088 }; 00089 00090 #endif // _XN_STRINGS_HASH_T_H_
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
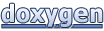