![]() |
OpenNI 1.5.4
|
XnStringsHash.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef _XN_STRINGS_HASH_H 00023 #define _XN_STRINGS_HASH_H 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include "XnHash.h" 00029 #include <XnOS.h> 00030 00031 //--------------------------------------------------------------------------- 00032 // Types 00033 //--------------------------------------------------------------------------- 00034 class XnStringsKeyManager 00035 { 00036 public: 00037 static XnHashValue Hash(const XnChar* const& key) 00038 { 00039 XnUInt32 nCRC = 0; 00040 xnOSStrCRC32(key, &nCRC); 00041 00042 // convert from UINT32 to XnHashValue 00043 return nCRC % (1 << (sizeof(XnHashValue)*8)); 00044 } 00045 00046 static XnInt32 Compare(const XnChar* const& key1, const XnChar* const& key2) 00047 { 00048 return strcmp(key1, key2); 00049 } 00050 }; 00051 00052 class XnStringsKeyTranslator 00053 { 00054 public: 00055 static XnValue CreateValueCopy(const XnChar* const& orig) 00056 { 00057 // we should copy string, so we can keep the key 00058 XnUInt32 nLen = xnOSStrLen(orig) + 1; // with null termination 00059 XnChar* pcKey = (XnChar*)xnOSMalloc(nLen); 00060 xnOSStrCopy(pcKey, orig, nLen); 00061 return (pcKey); 00062 } 00063 00064 static void FreeValue(XnValue& Value) 00065 { 00066 XnChar* pcKey = (XnChar*)Value; 00067 xnOSFree(pcKey); 00068 } 00069 00070 static XnValue GetAsValue(const XnChar* const& orig) 00071 { 00072 return (XnValue)orig; 00073 } 00074 00075 static const XnChar* const& GetFromValue(const XnValue& Value) 00076 { 00077 return (const XnChar* const&)Value; 00078 } 00079 00080 static const XnChar*& GetFromValue(XnValue& Value) 00081 { 00082 return (const XnChar*&)Value; 00083 } 00084 }; 00085 00090 #define XN_DECLARE_STRINGS_HASH_WITH_TRANSLATOR_DECL(decl, ValueType, ClassName, ValueTranslator) \ 00091 XN_DECLARE_HASH_DECL(decl, const XnChar*, ValueType, ClassName, XnStringsKeyTranslator, ValueTranslator, XnStringsKeyManager) \ 00092 00093 00097 #define XN_DECLARE_STRINGS_HASH_WITH_TRANSLATOR(ValueType, ClassName, ValueTranslator) \ 00098 XN_DECLARE_STRINGS_HASH_WITH_TRANSLATOR_DECL(, ValueType, ClassName, ValueTranslator) 00099 00104 #define XN_DECLARE_STRINGS_HASH_DECL(decl, ValueType, ClassName) \ 00105 XN_DECLARE_DEFAULT_VALUE_TRANSLATOR_DECL(decl, ValueType, XN_DEFAULT_TRANSLATOR_NAME(ClassName)) \ 00106 XN_DECLARE_STRINGS_HASH_WITH_TRANSLATOR_DECL(decl, ValueType, ClassName, XN_DEFAULT_TRANSLATOR_NAME(ClassName)) \ 00107 00108 00112 #define XN_DECLARE_STRINGS_HASH(ValueType, ClassName) \ 00113 XN_DECLARE_STRINGS_HASH_DECL(, ValueType, ClassName) 00114 00115 XN_DECLARE_STRINGS_HASH(XnValue, XnStringsHash) 00116 00117 00118 #endif //_XN_STRINGS_HASH_H
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
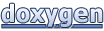