![]() |
OpenNI 1.5.4
|
XnQueueT.h
Go to the documentation of this file.
00001 #ifndef _XN_QUEUE_T_H_ 00002 #define _XN_QUEUE_T_H_ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include <XnListT.h> 00008 00009 //--------------------------------------------------------------------------- 00010 // Code 00011 //--------------------------------------------------------------------------- 00012 template<class T, class TAlloc = XnLinkedNodeDefaultAllocatorT<T> > 00013 class XnQueueT : protected XnListT<T, TAlloc> 00014 { 00015 public: 00016 typedef XnListT<T, TAlloc> Base; 00017 00018 XnQueueT() : Base() {} 00019 00020 XnQueueT(const XnQueueT& other) : Base() 00021 { 00022 *this = other; 00023 } 00024 00025 XnQueueT& operator=(const XnQueueT& other) 00026 { 00027 Base::operator=(other); 00028 // no other members 00029 return *this; 00030 } 00031 00032 ~XnQueueT() {} 00033 00034 using Base::ConstIterator; 00035 using Base::IsEmpty; 00036 00037 XnStatus Push(T const& value) 00038 { 00039 return Base::AddLast(value); 00040 } 00041 00042 XnStatus Pop(T& value) 00043 { 00044 Iterator it = Begin(); 00045 if (it == End()) 00046 { 00047 return XN_STATUS_IS_EMPTY; 00048 } 00049 value = *it; 00050 return Base::Remove(it); 00051 } 00052 00053 T const& Top() const { return *Begin(); } 00054 T& Top() { return *Begin(); } 00055 00056 using Base::Begin; 00057 using Base::End; 00058 using Base::Size; 00059 }; 00060 00061 00062 00063 #endif // _XN_QUEUE_T_H_
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
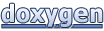