![]() |
OpenNI 1.5.4
|
XnOSCpp.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_OS_CPP_H__ 00023 #define __XN_OS_CPP_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnOS.h> 00029 00030 //--------------------------------------------------------------------------- 00031 // Types 00032 //--------------------------------------------------------------------------- 00033 class XnAutoCSLocker 00034 { 00035 public: 00036 inline XnAutoCSLocker(const XnAutoCSLocker& other) : m_hCS(other.m_hCS), m_bLocked(FALSE) 00037 { 00038 Lock(); 00039 } 00040 00041 inline XnAutoCSLocker& operator=(const XnAutoCSLocker& other) 00042 { 00043 Unlock(); 00044 m_hCS = other.m_hCS; 00045 Lock(); 00046 return *this; 00047 } 00048 00049 inline XnAutoCSLocker(XN_CRITICAL_SECTION_HANDLE hCS) : m_hCS(hCS), m_bLocked(FALSE) 00050 { 00051 Lock(); 00052 } 00053 00054 inline ~XnAutoCSLocker() 00055 { 00056 Unlock(); 00057 } 00058 00059 inline void Lock() 00060 { 00061 if (!m_bLocked) 00062 { 00063 xnOSEnterCriticalSection(&m_hCS); 00064 m_bLocked = TRUE; 00065 } 00066 } 00067 00068 inline void Unlock() 00069 { 00070 if (m_bLocked) 00071 { 00072 xnOSLeaveCriticalSection(&m_hCS); 00073 m_bLocked = FALSE; 00074 } 00075 } 00076 00077 private: 00078 XN_CRITICAL_SECTION_HANDLE m_hCS; 00079 XnBool m_bLocked; 00080 }; 00081 00082 class XnAutoMutexLocker 00083 { 00084 public: 00085 inline XnAutoMutexLocker(XN_MUTEX_HANDLE hMutex, XnUInt32 nMilliseconds) : m_hMutex(hMutex) 00086 { 00087 m_nStatus = xnOSLockMutex(m_hMutex, nMilliseconds); 00088 } 00089 00090 XnStatus GetStatus() const 00091 { 00092 return m_nStatus; 00093 } 00094 00095 inline ~XnAutoMutexLocker() 00096 { 00097 if (m_nStatus == XN_STATUS_OK) 00098 { 00099 //Only unlock if we managed to lock in the first place 00100 xnOSUnLockMutex(m_hMutex); 00101 } 00102 } 00103 00104 private: 00105 XN_MUTEX_HANDLE m_hMutex; 00106 XnStatus m_nStatus; 00107 }; 00108 00109 class XnOSEvent 00110 { 00111 public: 00112 XnOSEvent() : m_hEvent(NULL) {} 00113 00114 ~XnOSEvent() 00115 { 00116 Close(); 00117 } 00118 00119 operator XN_EVENT_HANDLE() const 00120 { 00121 return m_hEvent; 00122 } 00123 00124 XnStatus Create(XnBool bManualReset) 00125 { 00126 return xnOSCreateEvent(&m_hEvent, bManualReset); 00127 } 00128 00129 XnStatus Create(const XnChar* strName, XnBool bManualReset, XnBool bAllowOtherUsers = FALSE) 00130 { 00131 return xnOSCreateNamedEventEx(&m_hEvent, strName, bManualReset, bAllowOtherUsers); 00132 } 00133 00134 XnStatus Open(const XnChar* strName, XnBool bEnableOtherUsers = FALSE) 00135 { 00136 return xnOSOpenNamedEventEx(&m_hEvent, strName, bEnableOtherUsers); 00137 } 00138 00139 XnStatus Close() 00140 { 00141 return (m_hEvent != NULL) ? xnOSCloseEvent(&m_hEvent) : XN_STATUS_OK; 00142 } 00143 00144 XnStatus Set() 00145 { 00146 return xnOSSetEvent(m_hEvent); 00147 } 00148 00149 XnStatus Reset() 00150 { 00151 return xnOSResetEvent(m_hEvent); 00152 } 00153 00154 XnStatus Wait(XnUInt32 nMilliseconds) 00155 { 00156 return xnOSWaitEvent(m_hEvent, nMilliseconds); 00157 } 00158 00159 private: 00160 XN_EVENT_HANDLE m_hEvent; 00161 }; 00162 00163 #endif // __XN_OS_CPP_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
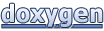