![]() |
OpenNI 1.5.4
|
XnModuleInterface.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_MODULE_INTERFACE_H__ 00023 #define __XN_MODULE_INTERFACE_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include <XnTypes.h> 00029 00030 //--------------------------------------------------------------------------- 00031 // Defines 00032 //--------------------------------------------------------------------------- 00033 #define XN_MODULE_LOAD xnModuleLoad 00034 #define XN_MODULE_UNLOAD xnModuleUnload 00035 #define XN_MODULE_GET_EXPORTED_NODES_COUNT xnModuleGetExportedNodesCount 00036 #define XN_MODULE_GET_EXPORTED_NODES_ENTRY_POINTS xnModuleGetExportedNodesEntryPoints 00037 #define XN_MODULE_GET_OPEN_NI_VERSION xnModuleGetOpenNIVersion 00038 00039 //--------------------------------------------------------------------------- 00040 // Forward Declarations 00041 //--------------------------------------------------------------------------- 00042 struct XnModuleProductionNodeInterface; 00043 struct XnModuleDeviceInterface; 00044 struct XnModuleDepthGeneratorInterface; 00045 struct XnModuleImageGeneratorInterface; 00046 struct XnModuleIRGeneratorInterface; 00047 struct XnModuleGestureGeneratorInterface; 00048 struct XnModuleUserGeneratorInterface; 00049 struct XnModuleHandsGeneratorInterface; 00050 struct XnModuleSceneAnalyzerInterface; 00051 struct XnModuleAudioGeneratorInterface; 00052 struct XnModuleRecorderInterface; 00053 struct XnModulePlayerInterface; 00054 struct XnModuleGeneratorInterface; 00055 struct XnModuleCodecInterface; 00056 struct XnModuleScriptNodeInterface; 00057 struct XnModuleMapGeneratorInterface; 00058 00059 //--------------------------------------------------------------------------- 00060 // Types 00061 //--------------------------------------------------------------------------- 00062 00063 typedef void (XN_CALLBACK_TYPE* XnModuleGetExportedInterfacePtr)(XnModuleExportedProductionNodeInterface* pInterface); 00064 typedef XnStatus (XN_C_DECL* XnModuleLoadPtr)(); 00065 typedef void (XN_C_DECL* XnModuleUnloadPtr)(); 00066 typedef XnUInt32 (XN_C_DECL* XnModuleGetExportedNodesCountPtr)(); 00067 typedef XnStatus (XN_C_DECL* XnModuleGetExportedNodesEntryPointsPtr)(XnModuleGetExportedInterfacePtr* aEntryPoints, XnUInt32 nCount); 00068 typedef void (XN_C_DECL* XnModuleGetOpenNIVersionPtr)(XnVersion* pVersion); 00069 00070 typedef struct XnOpenNIModuleInterface 00071 { 00072 XnModuleLoadPtr pLoadFunc; 00073 XnModuleUnloadPtr pUnloadFunc; 00074 XnModuleGetExportedNodesCountPtr pGetCountFunc; 00075 XnModuleGetExportedNodesEntryPointsPtr pGetEntryPointsFunc; 00076 XnModuleGetOpenNIVersionPtr pGetVersionFunc; 00077 } XnOpenNIModuleInterface; 00078 00080 typedef void (XN_CALLBACK_TYPE* XnModuleStateChangedHandler)(void* pCookie); 00081 00082 // User 00083 typedef void (XN_CALLBACK_TYPE* XnModuleUserHandler)(XnUserID user, void* pCookie); 00084 00085 // Hand touching FOV edge 00086 typedef void (XN_CALLBACK_TYPE* XnModuleHandTouchingFOVEdge)(XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, XnDirection eDir, void* pCookie); 00087 00088 // UI 00089 typedef void (XN_CALLBACK_TYPE* XnModuleHandCreate)(XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 00090 typedef void (XN_CALLBACK_TYPE* XnModuleHandUpdate)(XnUserID user, const XnPoint3D* pPosition, XnFloat fTime, void* pCookie); 00091 typedef void (XN_CALLBACK_TYPE* XnModuleHandDestroy)(XnUserID user, XnFloat fTime, void* pCookie); 00092 00093 // Gesture Module 00094 typedef void (XN_CALLBACK_TYPE* XnModuleGestureRecognized)(const XnChar* strGesture, const XnPoint3D* pIDPosition, const XnPoint3D* pEndPosition, void* pCookie); 00095 typedef void (XN_CALLBACK_TYPE* XnModuleGestureProgress)(const XnChar* strGesture, const XnPoint3D* pPosition, XnFloat fProgress, void* pCookie); 00096 typedef void (XN_CALLBACK_TYPE* XnModuleGestureIntermediateStageCompleted)(const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 00097 typedef void (XN_CALLBACK_TYPE* XnModuleGestureReadyForNextIntermediateStage)(const XnChar* strGesture, const XnPoint3D* pPosition, void* pCookie); 00098 00099 // Skeleton 00100 typedef void (XN_CALLBACK_TYPE* XnModuleCalibrationStart)(XnUserID user, void* pCookie); 00101 typedef void (XN_CALLBACK_TYPE* XnModuleCalibrationEnd)(XnUserID user, XnBool bSuccess, void* pCookie); 00102 typedef void (XN_CALLBACK_TYPE* XnModuleCalibrationInProgress)(XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 00103 typedef void (XN_CALLBACK_TYPE* XnModuleCalibrationComplete)(XnUserID user, XnCalibrationStatus calibrationError, void* pCookie); 00104 00105 // Pose Detection 00106 typedef void (XN_CALLBACK_TYPE* XnModulePoseDetectionCallback)(const XnChar* strPose, XnUserID user, void* pCookie); 00107 typedef void (XN_CALLBACK_TYPE* XnModulePoseDetectionInProgressCallback)(const XnChar* strPose, XnUserID user, XnPoseDetectionStatus poseError, void* pCookie); 00108 00109 typedef struct XnModuleExportedProductionNodeInterface 00110 { 00116 void (XN_CALLBACK_TYPE* GetDescription) 00117 (XnProductionNodeDescription* pDescription); 00118 00126 XnStatus (XN_CALLBACK_TYPE* EnumerateProductionTrees) 00127 (XnContext* pContext, 00128 XnNodeInfoList* pNodesList, 00129 XnEnumerationErrors* pErrors); 00130 00141 XnStatus (XN_CALLBACK_TYPE* Create) 00142 (XnContext* pContext, 00143 const XnChar* strInstanceName, 00144 const XnChar* strCreationInfo, 00145 XnNodeInfoList* pNeededNodes, 00146 const XnChar* strConfigurationDir, 00147 XnModuleNodeHandle* phInstance); 00148 00154 void (XN_CALLBACK_TYPE* Destroy) 00155 (XnModuleNodeHandle hInstance); 00156 00164 union 00165 { 00166 void (XN_CALLBACK_TYPE* ProductionNode)(struct XnModuleProductionNodeInterface* pInterface); 00167 void (XN_CALLBACK_TYPE* Device)(struct XnModuleDeviceInterface* pInterface); 00168 void (XN_CALLBACK_TYPE* Generator)(struct XnModuleGeneratorInterface* pInterface); 00169 void (XN_CALLBACK_TYPE* MapGenerator)(struct XnModuleMapGeneratorInterface* pInterface); 00170 void (XN_CALLBACK_TYPE* Depth)(struct XnModuleDepthGeneratorInterface* pInterface); 00171 void (XN_CALLBACK_TYPE* Image)(struct XnModuleImageGeneratorInterface* pInterface); 00172 void (XN_CALLBACK_TYPE* IR)(struct XnModuleIRGeneratorInterface* pInterface); 00173 void (XN_CALLBACK_TYPE* User)(struct XnModuleUserGeneratorInterface* pInterface); 00174 void (XN_CALLBACK_TYPE* Hands)(struct XnModuleHandsGeneratorInterface* pInterace); 00175 void (XN_CALLBACK_TYPE* Gesture)(struct XnModuleGestureGeneratorInterface* pInterface); 00176 void (XN_CALLBACK_TYPE* Scene)(struct XnModuleSceneAnalyzerInterface* pInterface); 00177 void (XN_CALLBACK_TYPE* Audio)(struct XnModuleAudioGeneratorInterface* pInterface); 00178 void (XN_CALLBACK_TYPE* Recorder)(struct XnModuleRecorderInterface* pInterface); 00179 void (XN_CALLBACK_TYPE* Player)(struct XnModulePlayerInterface* pInterface); 00180 void (XN_CALLBACK_TYPE* Codec)(struct XnModuleCodecInterface* pInterface); 00181 void (XN_CALLBACK_TYPE* Script)(struct XnModuleScriptNodeInterface* pInterface); 00182 00183 void (XN_CALLBACK_TYPE* General)(void* pInterface); 00184 } GetInterface; 00185 00186 } XnModuleExportedProductionNodeInterface; 00187 00188 typedef struct XnModuleExtendedSerializationInterface 00189 { 00190 XnStatus (XN_CALLBACK_TYPE* InitNotifications)(XnModuleNodeHandle hInstance, XnNodeNotifications* pNotifications, void* pCookie); 00191 void (XN_CALLBACK_TYPE* StopNotifications)(XnModuleNodeHandle hInstance); 00192 00193 } XnModuleExtendedSerializationInterface; 00194 00195 typedef struct XnModuleLockAwareInterface 00196 { 00203 XnStatus (XN_CALLBACK_TYPE* SetLockState)(XnModuleNodeHandle hInstance, XnBool bLocked); 00204 00210 XnBool (XN_CALLBACK_TYPE* GetLockState)(XnModuleNodeHandle hInstance); 00211 00220 XnStatus (XN_CALLBACK_TYPE* RegisterToLockChange) 00221 (XnModuleNodeHandle hInstance, XnModuleStateChangedHandler handler, 00222 void* pCookie, XnCallbackHandle* phCallback); 00223 00230 void (XN_CALLBACK_TYPE* UnregisterFromLockChange) 00231 (XnModuleNodeHandle hInstance, XnCallbackHandle hCallback); 00232 00233 } XnModuleLockAwareInterface; 00234 00235 typedef struct XnModuleErrorStateInterface 00236 { 00242 XnStatus (XN_CALLBACK_TYPE* GetErrorState)(XnModuleNodeHandle hInstance); 00243 00252 XnStatus (XN_CALLBACK_TYPE* RegisterToErrorStateChange) 00253 (XnModuleNodeHandle hInstance, XnModuleStateChangedHandler handler, 00254 void* pCookie, XnCallbackHandle* phCallback); 00255 00262 void (XN_CALLBACK_TYPE* UnregisterFromErrorStateChange) 00263 (XnModuleNodeHandle hInstance, XnCallbackHandle hCallback); 00264 00265 } XnModuleErrorStateInterface; 00266 00267 typedef struct XnModuleGeneralIntInterface 00268 { 00280 XnStatus (XN_CALLBACK_TYPE* GetRange)(XnModuleNodeHandle hGenerator, const XnChar* strCap, XnInt32* pnMin, XnInt32* pnMax, XnInt32* pnStep, XnInt32* pnDefault, XnBool* pbIsAutoSupported); 00281 00289 XnStatus (XN_CALLBACK_TYPE* Get)(XnModuleNodeHandle hGenerator, const XnChar* strCap, XnInt32* pnValue); 00290 00298 XnStatus (XN_CALLBACK_TYPE* Set)(XnModuleNodeHandle hGenerator, const XnChar* strCap, XnInt32 nValue); 00299 00309 XnStatus (XN_CALLBACK_TYPE* RegisterToValueChange) 00310 (XnModuleNodeHandle hGenerator, const XnChar* strCap, XnModuleStateChangedHandler handler, 00311 void* pCookie, XnCallbackHandle* phCallback); 00312 00320 void (XN_CALLBACK_TYPE* UnregisterFromValueChange) 00321 (XnModuleNodeHandle hGenerator, const XnChar* strCap, XnCallbackHandle hCallback); 00322 00323 } XnModuleGeneralIntInterface; 00324 00325 typedef struct XnModuleProductionNodeInterface 00326 { 00333 XnBool (XN_CALLBACK_TYPE* IsCapabilitySupported)( 00334 XnModuleNodeHandle hInstance, 00335 const XnChar* strCapabilityName 00336 ); 00337 00345 XnStatus (XN_CALLBACK_TYPE* SetIntProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnUInt64 nValue); 00346 XnStatus (XN_CALLBACK_TYPE* SetRealProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnDouble dValue); 00347 XnStatus (XN_CALLBACK_TYPE* SetStringProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, const XnChar* strValue); 00348 XnStatus (XN_CALLBACK_TYPE* SetGeneralProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnUInt32 nBufferSize, const void* pBuffer); 00349 00357 XnStatus (XN_CALLBACK_TYPE* GetIntProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnUInt64* pnValue); 00358 XnStatus (XN_CALLBACK_TYPE* GetRealProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnDouble* pdValue); 00359 XnStatus (XN_CALLBACK_TYPE* GetStringProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnChar* csValue, XnUInt32 nBufSize); 00360 XnStatus (XN_CALLBACK_TYPE* GetGeneralProperty)(XnModuleNodeHandle hInstance, const XnChar* strName, XnUInt32 nBufferSize, void* pBuffer); 00361 00362 XnModuleExtendedSerializationInterface* pExtendedSerializationInterface; 00363 XnModuleLockAwareInterface* pLockAwareInterface; 00364 XnModuleErrorStateInterface* pErrorStateInterface; 00365 XnModuleGeneralIntInterface* pGeneralIntInterface; 00366 00367 } XnModuleProductionNodeInterface; 00368 00369 typedef struct XnModuleDeviceIdentificationInterface 00370 { 00382 XnStatus (XN_CALLBACK_TYPE* GetDeviceName)(XnModuleNodeHandle hInstance, XnChar* strBuffer, XnUInt32* pnBufferSize); 00383 00395 XnStatus (XN_CALLBACK_TYPE* GetVendorSpecificData)(XnModuleNodeHandle hInstance, XnChar* strBuffer, XnUInt32* pnBufferSize); 00396 00408 XnStatus (XN_CALLBACK_TYPE* GetSerialNumber)(XnModuleNodeHandle hInstance, XnChar* strBuffer, XnUInt32* pnBufferSize); 00409 00410 } XnModuleDeviceIdentificationInterface; 00411 00412 typedef struct XnModuleDeviceInterface 00413 { 00414 XnModuleProductionNodeInterface* pProductionNode; 00415 00416 XnModuleDeviceIdentificationInterface* pDeviceIdentificationInterface; 00417 00418 } XnModuleDeviceInterface; 00419 00420 typedef struct XnModuleMirrorInterface 00421 { 00428 XnStatus (XN_CALLBACK_TYPE* SetMirror)(XnModuleNodeHandle hInstance, XnBool bMirror); 00429 00435 XnBool (XN_CALLBACK_TYPE* IsMirrored)(XnModuleNodeHandle hInstance); 00436 00445 XnStatus (XN_CALLBACK_TYPE* RegisterToMirrorChange) 00446 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00447 void* pCookie, XnCallbackHandle* phCallback); 00448 00455 void (XN_CALLBACK_TYPE* UnregisterFromMirrorChange) 00456 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00457 00458 } XnModuleMirrorInterface; 00459 00460 typedef struct XnModuleAlternativeViewPointInterface 00461 { 00471 XnBool (XN_CALLBACK_TYPE* IsViewPointSupported)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00472 00479 XnStatus (XN_CALLBACK_TYPE* SetViewPoint)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00480 00487 XnBool (XN_CALLBACK_TYPE* IsViewPointAs)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00488 00494 XnStatus (XN_CALLBACK_TYPE* ResetViewPoint)(XnModuleNodeHandle hGenerator); 00495 00504 XnStatus (XN_CALLBACK_TYPE* RegisterToViewPointChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, void* pCookie, XnCallbackHandle* phCallback); 00505 00512 void (XN_CALLBACK_TYPE* UnregisterFromViewPointChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00513 00514 } XnModuleAlternativeViewPointInterface; 00515 00516 typedef struct XnModuleFrameSyncInterface 00517 { 00526 XnBool (XN_CALLBACK_TYPE* CanFrameSyncWith)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00527 00534 XnStatus (XN_CALLBACK_TYPE* FrameSyncWith)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00535 00542 XnStatus (XN_CALLBACK_TYPE* StopFrameSyncWith)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00543 00550 XnBool (XN_CALLBACK_TYPE* IsFrameSyncedWith)(XnModuleNodeHandle hGenerator, XnNodeHandle hOther); 00551 00560 XnStatus (XN_CALLBACK_TYPE* RegisterToFrameSyncChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, void* pCookie, XnCallbackHandle* phCallback); 00561 00568 void (XN_CALLBACK_TYPE* UnregisterFromFrameSyncChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00569 00570 } XnModuleFrameSyncInterface; 00571 00573 typedef struct XnModuleGeneratorInterface 00574 { 00578 XnModuleProductionNodeInterface* pProductionNodeInterface; 00579 00585 XnStatus (XN_CALLBACK_TYPE* StartGenerating) 00586 (XnModuleNodeHandle hGenerator); 00587 00593 XnBool (XN_CALLBACK_TYPE* IsGenerating) 00594 (XnModuleNodeHandle hGenerator); 00595 00601 void (XN_CALLBACK_TYPE* StopGenerating) 00602 (XnModuleNodeHandle hGenerator); 00603 00612 XnStatus (XN_CALLBACK_TYPE* RegisterToGenerationRunningChange) 00613 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00614 void* pCookie, XnCallbackHandle* phCallback); 00615 00622 void (XN_CALLBACK_TYPE* UnregisterFromGenerationRunningChange) 00623 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00624 00632 XnStatus (XN_CALLBACK_TYPE* RegisterToNewDataAvailable) 00633 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00634 void* pCookie, XnCallbackHandle* phCallback); 00635 00642 void (XN_CALLBACK_TYPE* UnregisterFromNewDataAvailable) 00643 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00644 00651 XnBool (XN_CALLBACK_TYPE* IsNewDataAvailable) 00652 (XnModuleNodeHandle hGenerator, XnUInt64* pnTimestamp); 00653 00660 XnStatus (XN_CALLBACK_TYPE* UpdateData) 00661 (XnModuleNodeHandle hGenerator); 00662 00668 XnUInt32 (XN_CALLBACK_TYPE* GetDataSize)(XnModuleNodeHandle hGenerator); 00669 00675 XnUInt64 (XN_CALLBACK_TYPE* GetTimestamp)(XnModuleNodeHandle hGenerator); 00676 00682 XnUInt32 (XN_CALLBACK_TYPE* GetFrameID)(XnModuleNodeHandle hGenerator); 00683 00684 XnModuleMirrorInterface* pMirrorInterface; 00685 XnModuleAlternativeViewPointInterface* pAlternativeViewPointInterface; 00686 void* pObsolete1; // used to be pSeekingInterface (removed in 1.0.0.28) 00687 XnModuleFrameSyncInterface* pFrameSyncInterface; 00688 00689 //Note: The GetData() function was added in version 1.0.0.28 00695 const void* (XN_CALLBACK_TYPE* GetData)(XnModuleNodeHandle hGenerator); 00696 00697 } XnModuleGeneratorInterface; 00698 00699 typedef struct XnModuleRecorderInterface 00700 { 00701 /* 00702 * Sets the output stream for the recorder module. 00703 * 00704 * @param hInstance [in] A handle to the instance. 00705 * @param pStreamToken [in] A token that the recorder module must save for passing to later stream calls. 00706 * @param pStream [in] The stream interface the recorder module must save for later stream calls. 00707 */ 00708 XnStatus (XN_CALLBACK_TYPE* SetOutputStream) 00709 (XnModuleNodeHandle hInstance, void *pStreamToken, XnRecorderOutputStreamInterface *pStream); 00710 00711 XnModuleProductionNodeInterface* pProductionNode; 00712 XnNodeNotifications* pNodeNotifications; 00713 } XnModuleRecorderInterface; 00714 00715 typedef struct XnModulePlayerInterface 00716 { 00717 /* 00718 * Sets the input stream for the player module 00719 * 00720 * @param hInstance [in] A handle to the instance. 00721 * @param pStreamCookie [in] A cookie that the player module must save for passing to later stream calls. 00722 * @param pStream [in] The stream interface the player module must save for later stream calls. 00723 */ 00724 XnStatus (XN_CALLBACK_TYPE* SetInputStream) 00725 (XnModuleNodeHandle hInstance, void *pStreamCookie, XnPlayerInputStreamInterface *pStream); 00726 00732 XnStatus (XN_CALLBACK_TYPE* ReadNext)(XnModuleNodeHandle hInstance); 00733 00734 /* 00735 * Sets the node notifications object to be used by the player. The player will use this object to 00736 * notify about events it encounters in the stream that was set with @ref SetInputStream(). 00737 * 00738 * @param hInstance [in] A handle to the instance. 00739 * @param pNodeNotificationsCookie [in] A cookie that the player module must save for passing to node notification calls. 00740 * @param pNodeNotifications [in] The node notifications interface that the player module will use to raise node notifications it finds in the stream. 00741 */ 00742 XnStatus (XN_CALLBACK_TYPE* SetNodeNotifications) 00743 (XnModuleNodeHandle hInstance, void *pNodeNotificationsCookie, XnNodeNotifications *pNodeNotifications); 00744 00745 XnStatus (XN_CALLBACK_TYPE* SetRawNodeNotifications) 00746 (XnModuleNodeHandle hInstance, void *pRawNodeNotificationsCookie, XnNodeNotifications *pRawNodeNotifications); 00747 00748 00749 /* 00750 * Determines whether the player repeats the played stream or not. 00751 * 00752 * @param bRepeat [in] If TRUE, repeat is set to be ON. If FALSE, repeat is set to be OFF. 00753 */ 00754 XnStatus (XN_CALLBACK_TYPE* SetRepeat) 00755 (XnModuleNodeHandle hInstance, XnBool bRepeat); 00756 00757 XnStatus (XN_CALLBACK_TYPE* SeekToTimeStamp) 00758 (XnModuleNodeHandle hInstance, XnInt64 nTimeOffset, XnPlayerSeekOrigin origin); 00759 00760 XnStatus (XN_CALLBACK_TYPE* SeekToFrame) 00761 (XnModuleNodeHandle hInstance, const XnChar* strNodeName, XnInt32 nFrameOffset, XnPlayerSeekOrigin origin); 00762 00763 XnStatus (XN_CALLBACK_TYPE* TellTimestamp) 00764 (XnModuleNodeHandle hInstance, XnUInt64* pnTimestamp); 00765 00766 XnStatus (XN_CALLBACK_TYPE* TellFrame) 00767 (XnModuleNodeHandle hInstance, const XnChar* strNodeName, XnUInt32* pnFrame); 00768 00769 XnStatus (XN_CALLBACK_TYPE* GetNumFrames) 00770 (XnModuleNodeHandle hInstance, const XnChar* strNodeName, XnUInt32* pnFrames); 00771 00772 const XnChar* (XN_CALLBACK_TYPE* GetSupportedFormat) 00773 (XnModuleNodeHandle hInstance); 00774 00775 XnBool (XN_CALLBACK_TYPE* IsEOF) 00776 (XnModuleNodeHandle hInstance); 00777 00786 XnStatus (XN_CALLBACK_TYPE* RegisterToEndOfFileReached) 00787 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00788 void* pCookie, XnCallbackHandle* phCallback); 00789 00796 void (XN_CALLBACK_TYPE* UnregisterFromEndOfFileReached) 00797 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00798 00799 XnModuleProductionNodeInterface* pProductionNode; 00800 void* pObsolete1; // used to be pSeekingInterface (removed in 1.0.0.28) 00801 00802 } XnModulePlayerInterface; 00803 00804 typedef struct XnModuleCroppingInterface 00805 { 00812 XnStatus (XN_CALLBACK_TYPE* SetCropping)(XnModuleNodeHandle hGenerator, const XnCropping* pCropping); 00813 00820 XnStatus (XN_CALLBACK_TYPE* GetCropping)(XnModuleNodeHandle hGenerator, XnCropping* pCropping); 00821 00830 XnStatus (XN_CALLBACK_TYPE* RegisterToCroppingChange) 00831 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00832 void* pCookie, XnCallbackHandle* phCallback); 00833 00840 void (XN_CALLBACK_TYPE* UnregisterFromCroppingChange) 00841 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00842 00843 } XnModuleCroppingInterface; 00844 00845 typedef struct XnModuleAntiFlickerInterface 00846 { 00853 XnStatus (XN_CALLBACK_TYPE* SetPowerLineFrequency)(XnModuleNodeHandle hGenerator, XnPowerLineFrequency nFrequency); 00854 00860 XnPowerLineFrequency (XN_CALLBACK_TYPE* GetPowerLineFrequency)(XnModuleNodeHandle hGenerator); 00861 00870 XnStatus (XN_CALLBACK_TYPE* RegisterToPowerLineFrequencyChange) 00871 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00872 void* pCookie, XnCallbackHandle* phCallback); 00873 00880 void (XN_CALLBACK_TYPE* UnregisterFromPowerLineFrequencyChange) 00881 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00882 00883 } XnModuleAntiFlickerInterface; 00884 00885 typedef struct XnModuleMapGeneratorInterface 00886 { 00890 XnModuleGeneratorInterface* pGeneratorInterface; 00891 00898 XnUInt32 (XN_CALLBACK_TYPE* GetSupportedMapOutputModesCount) 00899 (XnModuleNodeHandle hGenerator); 00900 00910 XnStatus (XN_CALLBACK_TYPE* GetSupportedMapOutputModes) 00911 (XnModuleNodeHandle hGenerator, 00912 XnMapOutputMode* aModes, XnUInt32* pnCount); 00913 00920 XnStatus (XN_CALLBACK_TYPE* SetMapOutputMode) 00921 (XnModuleNodeHandle hGenerator, const XnMapOutputMode* pOutputMode); 00922 00929 XnStatus (XN_CALLBACK_TYPE* GetMapOutputMode) 00930 (XnModuleNodeHandle hGenerator, XnMapOutputMode* pOutputMode); 00931 00940 XnStatus (XN_CALLBACK_TYPE* RegisterToMapOutputModeChange) 00941 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 00942 void* pCookie, XnCallbackHandle* phCallback); 00943 00950 void (XN_CALLBACK_TYPE* UnregisterFromMapOutputModeChange) 00951 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 00952 00953 XnModuleCroppingInterface* pCroppingInterface; 00954 00955 // NOTE: GetBytesPerPixel() was added in OpenNI 1.0.0.30 00961 XnUInt32 (XN_CALLBACK_TYPE* GetBytesPerPixel) 00962 (XnModuleNodeHandle hGenerator); 00963 00964 XnModuleAntiFlickerInterface* pAntiFlickerInterface; 00965 00966 } XnModuleMapGeneratorInterface; 00967 00971 typedef struct XnModuleUserPositionCapabilityInterface 00972 { 00978 XnUInt32 (XN_CALLBACK_TYPE* GetSupportedUserPositionsCount) 00979 (XnModuleNodeHandle hGenerator); 00980 00988 XnStatus (XN_CALLBACK_TYPE* SetUserPosition)( 00989 XnModuleNodeHandle hGenerator, 00990 XnUInt32 nIndex, 00991 const XnBoundingBox3D* pPosition); 00992 01000 XnStatus (XN_CALLBACK_TYPE* GetUserPosition) 01001 (XnModuleNodeHandle hGenerator, XnUInt32 nIndex, XnBoundingBox3D* pPosition); 01002 01011 XnStatus (XN_CALLBACK_TYPE* RegisterToUserPositionChange) 01012 (XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 01013 void* pCookie, XnCallbackHandle* phCallback); 01014 01021 void (XN_CALLBACK_TYPE* UnregisterFromUserPositionChange) 01022 (XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01023 01024 } XnModuleUserPositionCapabilityInterface; 01025 01027 typedef struct XnModuleDepthGeneratorInterface 01028 { 01032 XnModuleMapGeneratorInterface* pMapInterface; 01033 01039 XnDepthPixel* (XN_CALLBACK_TYPE* GetDepthMap) 01040 (XnModuleNodeHandle hGenerator); 01041 01047 XnDepthPixel (XN_CALLBACK_TYPE* GetDeviceMaxDepth) 01048 (XnModuleNodeHandle hGenerator); 01049 01058 void (XN_CALLBACK_TYPE* GetFieldOfView)(XnModuleNodeHandle hGenerator, XnFieldOfView* pFOV); 01059 01068 XnStatus (XN_CALLBACK_TYPE* RegisterToFieldOfViewChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 01069 void* pCookie, XnCallbackHandle* phCallback); 01070 01077 void (XN_CALLBACK_TYPE* UnregisterFromFieldOfViewChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01078 01082 XnModuleUserPositionCapabilityInterface* pUserPositionInterface; 01083 01084 } XnModuleDepthGeneratorInterface; 01085 01087 typedef struct XnModuleImageGeneratorInterface 01088 { 01092 XnModuleMapGeneratorInterface* pMapInterface; 01093 01095 XnUInt8* (XN_CALLBACK_TYPE* GetImageMap)( 01096 XnModuleNodeHandle hGenerator 01097 ); 01098 01105 XnBool (XN_CALLBACK_TYPE* IsPixelFormatSupported)(XnModuleNodeHandle hGenerator, XnPixelFormat Format); 01106 01113 XnStatus (XN_CALLBACK_TYPE* SetPixelFormat)(XnModuleNodeHandle hGenerator, XnPixelFormat Format); 01114 01120 XnPixelFormat (XN_CALLBACK_TYPE* GetPixelFormat)(XnModuleNodeHandle hGenerator); 01121 01130 XnStatus (XN_CALLBACK_TYPE* RegisterToPixelFormatChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 01131 void* pCookie, XnCallbackHandle* phCallback); 01132 01139 void (XN_CALLBACK_TYPE* UnregisterFromPixelFormatChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01140 01141 } XnModuleImageGeneratorInterface; 01142 01144 typedef struct XnModuleIRGeneratorInterface 01145 { 01149 XnModuleMapGeneratorInterface* pMapInterface; 01150 01152 XnIRPixel* (XN_CALLBACK_TYPE* GetIRMap)( 01153 XnModuleNodeHandle hGenerator 01154 ); 01155 01156 } XnModuleIRGeneratorInterface; 01157 01159 typedef struct XnModuleGestureGeneratorInterface 01160 { 01161 XnModuleGeneratorInterface* pGeneratorInterface; 01162 01163 XnStatus (XN_CALLBACK_TYPE* AddGesture)(XnModuleNodeHandle hGenerator, const XnChar* strGesture, XnBoundingBox3D* pArea); 01164 XnStatus (XN_CALLBACK_TYPE* RemoveGesture)(XnModuleNodeHandle hGenerator, const XnChar* strGesture); 01165 XnStatus (XN_CALLBACK_TYPE* GetActiveGestures)(XnModuleNodeHandle hGenerator, XnChar** pstrGestures, XnUInt16* nGestures); 01166 XnStatus (XN_CALLBACK_TYPE* EnumerateGestures)(XnModuleNodeHandle hGenerator, XnChar** pstrGestures, XnUInt16* nGestures); 01167 XnBool (XN_CALLBACK_TYPE* IsGestureAvailable)(XnModuleNodeHandle hInstance, const XnChar* strGesture); 01168 XnBool (XN_CALLBACK_TYPE* IsGestureProgressSupported)(XnModuleNodeHandle hGenerator, const XnChar* strGesture); 01169 XnStatus (XN_CALLBACK_TYPE* RegisterGestureCallbacks)(XnModuleNodeHandle hGenerator, XnModuleGestureRecognized RecognizedCB, XnModuleGestureProgress ProgressCB, void* pCookie, XnCallbackHandle* phCallback); 01170 void (XN_CALLBACK_TYPE* UnregisterGestureCallbacks)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01171 XnStatus (XN_CALLBACK_TYPE* RegisterToGestureChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, void* pCookie, XnCallbackHandle* phCallback); 01172 void (XN_CALLBACK_TYPE* UnregisterFromGestureChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01173 01174 XnStatus (XN_CALLBACK_TYPE* GetAllActiveGestures)(XnModuleNodeHandle hGenerator, XnChar** pstrGestures, XnUInt32 nNameLength, XnUInt16* nGestures); 01175 XnStatus (XN_CALLBACK_TYPE* EnumerateAllGestures)(XnModuleNodeHandle hGenerator, XnChar** pstrGestures, XnUInt32 nNameLength, XnUInt16* nGestures); 01176 01177 XnStatus (XN_CALLBACK_TYPE* RegisterToGestureIntermediateStageCompleted)(XnModuleNodeHandle hGenerator, XnModuleGestureIntermediateStageCompleted GestureIntermediateStageCompletedCB, void* pCookie, XnCallbackHandle* phCallback); 01178 void (XN_CALLBACK_TYPE* UnregisterFromGestureIntermediateStageCompleted)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01179 XnStatus (XN_CALLBACK_TYPE* RegisterToGestureReadyForNextIntermediateStage)(XnModuleNodeHandle hGenerator, XnModuleGestureReadyForNextIntermediateStage ReadyForNextIntermediateStageCB, void* pCookie, XnCallbackHandle* phCallback); 01180 void (XN_CALLBACK_TYPE* UnregisterFromGestureReadyForNextIntermediateStage)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01181 01182 } XnModuleGestureGeneratorInterface; 01183 01185 typedef struct XnModuleSceneAnalyzerInterface 01186 { 01187 XnModuleMapGeneratorInterface* pMapInterface; 01188 01189 const XnLabel* (XN_CALLBACK_TYPE* GetLabelMap)(XnModuleNodeHandle hGenerator); 01190 XnStatus (XN_CALLBACK_TYPE* GetFloor)(XnModuleNodeHandle hGenerator, XnPlane3D* pPlane); 01191 } XnModuleSceneAnalyzerInterface; 01192 01196 typedef struct XnModuleHandTouchingFOVEdgeCapabilityInterface 01197 { 01198 XnStatus (XN_CALLBACK_TYPE* RegisterToHandTouchingFOVEdge)(XnModuleNodeHandle hGenerator, XnModuleHandTouchingFOVEdge, void* pCookie, XnCallbackHandle* phCallback); 01199 void (XN_CALLBACK_TYPE* UnregisterFromHandTouchingFOVEdge)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01200 } XnModuleHandTouchingFOVEdgeCapabilityInterface; 01201 01202 typedef struct XnModuleHandsGeneratorInterface 01203 { 01204 XnModuleGeneratorInterface* pGeneratorInterface; 01205 01206 XnStatus (XN_CALLBACK_TYPE* RegisterHandCallbacks)(XnModuleNodeHandle hGenerator, XnModuleHandCreate CreateCB, XnModuleHandUpdate UpdateCB, XnModuleHandDestroy DestroyCB, void* pCookie, XnCallbackHandle* phCallback); 01207 void (XN_CALLBACK_TYPE* UnregisterHandCallbacks)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01208 XnStatus (XN_CALLBACK_TYPE* StopTracking)(XnModuleNodeHandle hGenerator, XnUserID user); 01209 XnStatus (XN_CALLBACK_TYPE* StopTrackingAll)(XnModuleNodeHandle hGenerator); 01210 XnStatus (XN_CALLBACK_TYPE* StartTracking)(XnModuleNodeHandle hGenerator, const XnPoint3D* pPosition); 01211 XnStatus (XN_CALLBACK_TYPE* SetSmoothing)(XnModuleNodeHandle hGenerator, XnFloat fSmoothingFactor); 01212 01213 XnModuleHandTouchingFOVEdgeCapabilityInterface* pHandTouchingFOVEdgeInterface; 01214 01215 } XnModuleHandsGeneratorInterface; 01216 01220 typedef struct XnModuleSkeletonCapabilityInterface 01221 { 01222 XnBool (XN_CALLBACK_TYPE* IsJointAvailable)(XnModuleNodeHandle hGenerator, XnSkeletonJoint eJoint); 01223 XnBool (XN_CALLBACK_TYPE* IsProfileAvailable)(XnModuleNodeHandle hGenerator, XnSkeletonProfile eProfile); 01224 XnStatus (XN_CALLBACK_TYPE* SetSkeletonProfile)(XnModuleNodeHandle hGenerator, XnSkeletonProfile eProfile); 01225 XnStatus (XN_CALLBACK_TYPE* SetJointActive)(XnModuleNodeHandle hGenerator, XnSkeletonJoint eJoint, XnBool bState); 01226 XnBool (XN_CALLBACK_TYPE* IsJointActive)(XnModuleNodeHandle hGenerator, XnSkeletonJoint eJoint); 01227 XnStatus (XN_CALLBACK_TYPE* RegisterToJointConfigurationChange)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, void* pCookie, XnCallbackHandle* phCallback); 01228 void (XN_CALLBACK_TYPE* UnregisterFromJointConfigurationChange)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01229 XnStatus (XN_CALLBACK_TYPE* EnumerateActiveJoints)(XnModuleNodeHandle hGenerator, XnSkeletonJoint* pJoints, XnUInt16* pnJoints); 01230 XnStatus (XN_CALLBACK_TYPE* GetSkeletonJoint)(XnModuleNodeHandle hGenerator, XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointTransformation* pJoint); 01231 XnStatus (XN_CALLBACK_TYPE* GetSkeletonJointPosition)(XnModuleNodeHandle hGenerator, XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointPosition* pJoint); 01232 XnStatus (XN_CALLBACK_TYPE* GetSkeletonJointOrientation)(XnModuleNodeHandle hGenerator, XnUserID user, XnSkeletonJoint eJoint, XnSkeletonJointOrientation* pJoint); 01233 XnBool (XN_CALLBACK_TYPE* IsTracking)(XnModuleNodeHandle hGenerator, XnUserID user); 01234 XnBool (XN_CALLBACK_TYPE* IsCalibrated)(XnModuleNodeHandle hGenerator, XnUserID user); 01235 XnBool (XN_CALLBACK_TYPE* IsCalibrating)(XnModuleNodeHandle hGenerator, XnUserID user); 01236 XnStatus (XN_CALLBACK_TYPE* RequestCalibration)(XnModuleNodeHandle hGenerator, XnUserID user, XnBool bForce); 01237 XnStatus (XN_CALLBACK_TYPE* AbortCalibration)(XnModuleNodeHandle hGenerator, XnUserID user); 01238 XnStatus (XN_CALLBACK_TYPE* SaveCalibrationData)(XnModuleNodeHandle hGenerator, XnUserID user, XnUInt32 nSlot); 01239 XnStatus (XN_CALLBACK_TYPE* LoadCalibrationData)(XnModuleNodeHandle hGenerator, XnUserID user, XnUInt32 nSlot); 01240 XnStatus (XN_CALLBACK_TYPE* ClearCalibrationData)(XnModuleNodeHandle hGenerator, XnUInt32 nSlot); 01241 XnBool (XN_CALLBACK_TYPE* IsCalibrationData)(XnModuleNodeHandle hGenerator, XnUInt32 nSlot); 01242 XnStatus (XN_CALLBACK_TYPE* StartTracking)(XnModuleNodeHandle hGenerator, XnUserID user); 01243 XnStatus (XN_CALLBACK_TYPE* StopTracking)(XnModuleNodeHandle hGenerator, XnUserID user); 01244 XnStatus (XN_CALLBACK_TYPE* Reset)(XnModuleNodeHandle hGenerator, XnUserID user); 01245 XnBool (XN_CALLBACK_TYPE* NeedPoseForCalibration)(XnModuleNodeHandle hGenerator); 01246 XnStatus (XN_CALLBACK_TYPE* GetCalibrationPose)(XnModuleNodeHandle hGenerator, XnChar* strPose); 01247 XnStatus (XN_CALLBACK_TYPE* SetSmoothing)(XnModuleNodeHandle hGenerator, XnFloat fSmoothingFactor); 01248 XnStatus (XN_CALLBACK_TYPE* RegisterCalibrationCallbacks)(XnModuleNodeHandle hGenerator, XnModuleCalibrationStart CalibrationStartCB, XnModuleCalibrationEnd CalibrationEndCB, void* pCookie, XnCallbackHandle* phCallback); 01249 void (XN_CALLBACK_TYPE* UnregisterCalibrationCallbacks)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01250 01251 XnStatus (XN_CALLBACK_TYPE* SaveCalibrationDataToFile)(XnModuleNodeHandle hGenerator, XnUserID user, const XnChar* strFileName); 01252 XnStatus (XN_CALLBACK_TYPE* LoadCalibrationDataFromFile)(XnModuleNodeHandle hGenerator, XnUserID user, const XnChar* strFileName); 01253 01254 XnStatus (XN_CALLBACK_TYPE* RegisterToCalibrationInProgress)(XnModuleNodeHandle hGenerator, XnModuleCalibrationInProgress CalibrationInProgressCB, void* pCookie, XnCallbackHandle* phCallback); 01255 void (XN_CALLBACK_TYPE* UnregisterFromCalibrationInProgress)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01256 XnStatus (XN_CALLBACK_TYPE* RegisterToCalibrationComplete)(XnModuleNodeHandle hGenerator, XnModuleCalibrationComplete CalibrationCompleteCB, void* pCookie, XnCallbackHandle* phCallback); 01257 void (XN_CALLBACK_TYPE* UnregisterFromCalibrationComplete)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01258 01259 XnStatus (XN_CALLBACK_TYPE* RegisterToCalibrationStart)(XnModuleNodeHandle hGenerator, XnModuleCalibrationStart handler, void* pCookie, XnCallbackHandle* phCallback); 01260 void (XN_CALLBACK_TYPE* UnregisterFromCalibrationStart)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01261 } XnModuleSkeletonCapabilityInterface; 01262 01263 typedef struct XnModulePoseDetectionCapabilityInterface 01264 { 01265 XnUInt32 (XN_CALLBACK_TYPE* GetNumberOfPoses)(XnModuleNodeHandle hGenerator); 01266 XnStatus (XN_CALLBACK_TYPE* GetAvailablePoses)(XnModuleNodeHandle hGenerator, XnChar** pstrPoses, XnUInt32* pnPoses); 01267 XnStatus (XN_CALLBACK_TYPE* StartPoseDetection)(XnModuleNodeHandle hGenerator, const XnChar* strPose, XnUserID user); 01268 XnStatus (XN_CALLBACK_TYPE* StopPoseDetection)(XnModuleNodeHandle hGenerator, XnUserID user); 01269 XnStatus (XN_CALLBACK_TYPE* RegisterToPoseCallbacks)(XnModuleNodeHandle hGenerator, XnModulePoseDetectionCallback StartPoseCB, XnModulePoseDetectionCallback EndCB, void* pCookie, XnCallbackHandle* phCallback); 01270 void (XN_CALLBACK_TYPE* UnregisterFromPoseCallbacks)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01271 01272 XnStatus (XN_CALLBACK_TYPE* GetAllAvailablePoses)(XnModuleNodeHandle hGenerator, XnChar** pstrPoses, XnUInt32 nNameLength, XnUInt32* pnPoses); 01273 01274 XnStatus (XN_CALLBACK_TYPE* RegisterToPoseDetectionInProgress)(XnModuleNodeHandle hGenerator, XnModulePoseDetectionInProgressCallback PoseProgressCB, void* pCookie, XnCallbackHandle* phCallback); 01275 void (XN_CALLBACK_TYPE* UnregisterFromPoseDetectionInProgress)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01276 01277 XnStatus (XN_CALLBACK_TYPE* RegisterToPoseDetected)(XnModuleNodeHandle hGenerator, XnModulePoseDetectionCallback handler, void* pCookie, XnCallbackHandle* phCallback); 01278 void (XN_CALLBACK_TYPE* UnregisterFromPoseDetected)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01279 XnStatus (XN_CALLBACK_TYPE* RegisterToOutOfPose)(XnModuleNodeHandle hGenerator, XnModulePoseDetectionCallback handler, void* pCookie, XnCallbackHandle* phCallback); 01280 void (XN_CALLBACK_TYPE* UnregisterFromOutOfPose)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01281 01282 XnStatus (XN_CALLBACK_TYPE* StopSinglePoseDetection)(XnModuleNodeHandle hGenerator, XnUserID user, const XnChar* strPose); 01283 } XnModulePoseDetectionCapabilityInterface; 01284 01286 typedef struct XnModuleUserGeneratorInterface 01287 { 01288 XnModuleGeneratorInterface* pGeneratorInterface; 01289 01290 XnUInt16 (XN_CALLBACK_TYPE* GetNumberOfUsers)(XnModuleNodeHandle hGenerator); 01291 XnStatus (XN_CALLBACK_TYPE* GetUsers)(XnModuleNodeHandle hGenerator, XnUserID* pUsers, XnUInt16* pnUsers); 01292 XnStatus (XN_CALLBACK_TYPE* GetCoM)(XnModuleNodeHandle hGenerator, XnUserID user, XnPoint3D* pCoM); 01293 XnStatus (XN_CALLBACK_TYPE* GetUserPixels)(XnModuleNodeHandle hGenerator, XnUserID user, XnSceneMetaData* pScene); 01294 XnStatus (XN_CALLBACK_TYPE* RegisterUserCallbacks)(XnModuleNodeHandle hGenerator, XnModuleUserHandler NewUserCB, XnModuleUserHandler LostUserCB, void* pCookie, XnCallbackHandle* phCallback); 01295 void (XN_CALLBACK_TYPE* UnregisterUserCallbacks)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01296 01300 XnModuleSkeletonCapabilityInterface* pSkeletonInterface; 01304 XnModulePoseDetectionCapabilityInterface* pPoseDetectionInterface; 01305 01306 XnStatus (XN_CALLBACK_TYPE* RegisterToUserExit)(XnModuleNodeHandle hGenerator, XnModuleUserHandler UserExitCB, void* pCookie, XnCallbackHandle* phCallback); 01307 void (XN_CALLBACK_TYPE* UnregisterFromUserExit)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01308 XnStatus (XN_CALLBACK_TYPE* RegisterToUserReEnter)(XnModuleNodeHandle hGenerator, XnModuleUserHandler UserReEnterCB, void* pCookie, XnCallbackHandle* phCallback); 01309 void (XN_CALLBACK_TYPE* UnregisterFromUserReEnter)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01310 01311 } XnModuleUserGeneratorInterface; 01312 01314 typedef struct XnModuleAudioGeneratorInterface 01315 { 01316 XnModuleGeneratorInterface* pGeneratorInterface; 01317 01318 XnUChar* (XN_CALLBACK_TYPE* GetAudioBuffer)(XnModuleNodeHandle hGenerator); 01319 XnUInt32 (XN_CALLBACK_TYPE* GetSupportedWaveOutputModesCount)(XnModuleNodeHandle hGenerator); 01320 XnStatus (XN_CALLBACK_TYPE* GetSupportedWaveOutputModes)(XnModuleNodeHandle hGenerator, XnWaveOutputMode* aSupportedModes, XnUInt32* pnCount); 01321 XnStatus (XN_CALLBACK_TYPE* SetWaveOutputMode)(XnModuleNodeHandle hGenerator, const XnWaveOutputMode* OutputMode); 01322 XnStatus (XN_CALLBACK_TYPE* GetWaveOutputMode)(XnModuleNodeHandle hGenerator, XnWaveOutputMode* OutputMode); 01323 XnStatus (XN_CALLBACK_TYPE* RegisterToWaveOutputModeChanges)(XnModuleNodeHandle hGenerator, XnModuleStateChangedHandler handler, 01324 void* pCookie, XnCallbackHandle* phCallback); 01325 void (XN_CALLBACK_TYPE* UnregisterFromWaveOutputModeChanges)(XnModuleNodeHandle hGenerator, XnCallbackHandle hCallback); 01326 01327 } XnModuleAudioGeneratorInterface; 01328 01329 typedef struct XnModuleCodecInterface 01330 { 01331 XnModuleProductionNodeInterface* pProductionNode; 01332 01333 XnCodecID (XN_CALLBACK_TYPE* GetCodecID)(XnModuleNodeHandle hCodec); 01334 XnStatus (XN_CALLBACK_TYPE* Init)(XnModuleNodeHandle hCodec, XnNodeHandle hNode); 01335 XnStatus (XN_CALLBACK_TYPE* CompressData)(XnModuleNodeHandle hCodec, const void* pSrc, XnUInt32 nSrcSize, void* pDst, XnUInt32 nDstSize, XnUInt* pnBytesWritten); 01336 XnStatus (XN_CALLBACK_TYPE* DecompressData)(XnModuleNodeHandle hCodec, const void* pSrc, XnUInt32 nSrcSize, void* pDst, XnUInt32 nDstSize, XnUInt* pnBytesWritten); 01337 01338 } XnModuleCodecInterface; 01339 01340 typedef struct XnModuleScriptNodeInterface 01341 { 01342 XnModuleProductionNodeInterface* pProductionNode; 01343 01344 const XnChar* (XN_CALLBACK_TYPE* GetSupportedFormat)(XnModuleNodeHandle hScript); 01345 XnStatus (XN_CALLBACK_TYPE* LoadScriptFromFile)(XnModuleNodeHandle hScript, const XnChar* strFileName); 01346 XnStatus (XN_CALLBACK_TYPE* LoadScriptFromString)(XnModuleNodeHandle hScript, const XnChar* strScript); 01347 XnStatus (XN_CALLBACK_TYPE* Run)(XnModuleNodeHandle hScript, XnNodeInfoList* pCreatedNodes, XnEnumerationErrors* pErrors); 01348 01349 } XnModuleScriptNodeInterface; 01350 01351 #endif // __XN_MODULE_INTERFACE_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
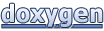