![]() |
OpenNI 1.5.4
|
XnGeneralBuffer.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_GENERAL_BUFFER_H__ 00023 #define __XN_GENERAL_BUFFER_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include "XnPlatform.h" 00029 #include "XnOS.h" 00030 #include "XnStatusCodes.h" 00031 00032 //--------------------------------------------------------------------------- 00033 // Types 00034 //--------------------------------------------------------------------------- 00035 00036 /* Describes a general buffer. */ 00037 typedef struct XnGeneralBuffer 00038 { 00039 /* A pointer to the actual data. */ 00040 void* pData; 00041 /* The size of the data in bytes. */ 00042 XnUInt32 nDataSize; 00043 } XnGeneralBuffer; 00044 00045 //--------------------------------------------------------------------------- 00046 // Exported Functions 00047 //--------------------------------------------------------------------------- 00048 00052 inline XnGeneralBuffer XnGeneralBufferPack(void* pData, XnUInt32 nDataSize) 00053 { 00054 XnGeneralBuffer result; 00055 result.pData = pData; 00056 result.nDataSize = nDataSize; 00057 return result; 00058 } 00059 00063 inline XnStatus XnGeneralBufferCopy(XnGeneralBuffer* pDest, const XnGeneralBuffer* pSrc) 00064 { 00065 XN_VALIDATE_INPUT_PTR(pDest); 00066 XN_VALIDATE_INPUT_PTR(pSrc); 00067 00068 if (pSrc->nDataSize > pDest->nDataSize) 00069 return XN_STATUS_OUTPUT_BUFFER_OVERFLOW; 00070 00071 xnOSMemCopy(pDest->pData, pSrc->pData, pSrc->nDataSize); 00072 pDest->nDataSize = pSrc->nDataSize; 00073 return XN_STATUS_OK; 00074 } 00075 00076 inline XnStatus XnGeneralBufferAlloc(XnGeneralBuffer* pDest, XnUInt32 nSize) 00077 { 00078 XN_VALIDATE_INPUT_PTR(pDest); 00079 00080 void* pData; 00081 pData = xnOSMalloc(nSize); 00082 XN_VALIDATE_ALLOC_PTR(pData); 00083 00084 pDest->pData = pData; 00085 pDest->nDataSize = nSize; 00086 return XN_STATUS_OK; 00087 } 00088 00089 inline XnStatus XnGeneralBufferRealloc(XnGeneralBuffer* pDest, XnUInt32 nSize) 00090 { 00091 XN_VALIDATE_INPUT_PTR(pDest); 00092 00093 void* pData; 00094 pData = xnOSRealloc(pDest, nSize); 00095 XN_VALIDATE_ALLOC_PTR(pData); 00096 00097 pDest->pData = pData; 00098 pDest->nDataSize = nSize; 00099 return XN_STATUS_OK; 00100 } 00101 00102 inline void XnGeneralBufferFree(XnGeneralBuffer* pDest) 00103 { 00104 XN_FREE_AND_NULL(pDest->pData); 00105 pDest->nDataSize = 0; 00106 } 00107 00108 //--------------------------------------------------------------------------- 00109 // Helper Macros 00110 //--------------------------------------------------------------------------- 00111 #define XN_PACK_GENERAL_BUFFER(x) XnGeneralBufferPack(&x, sizeof(x)) 00112 00113 #define XN_VALIDATE_GENERAL_BUFFER_TYPE(gb, t) \ 00114 if ((gb).nDataSize != sizeof(t)) \ 00115 { \ 00116 return XN_STATUS_INVALID_BUFFER_SIZE; \ 00117 } 00118 00119 #endif //__XN_GENERAL_BUFFER_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
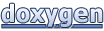