![]() |
OpenNI 1.5.4
|
XnDumpWriters.h
Go to the documentation of this file.
00001 #ifndef __XN_DUMP_WRITERS_H__ 00002 #define __XN_DUMP_WRITERS_H__ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include "XnDump.h" 00008 00009 //--------------------------------------------------------------------------- 00010 // Types 00011 //--------------------------------------------------------------------------- 00012 typedef struct XnDumpWriterFileHandle 00013 { 00014 void* pInternal; 00015 } XnDumpWriterFileHandle; 00016 00017 typedef struct XnDumpWriter 00018 { 00019 void* pCookie; 00020 XnDumpWriterFileHandle (XN_CALLBACK_TYPE* OpenFile)(void* pCookie, const XnChar* strDumpName, XnBool bSessionDump, const XnChar* strFileName); 00021 void (XN_CALLBACK_TYPE* Write)(void* pCookie, XnDumpWriterFileHandle hFile, const void* pBuffer, XnUInt32 nBufferSize); 00022 void (XN_CALLBACK_TYPE* CloseFile)(void* pCookie, XnDumpWriterFileHandle hFile); 00023 } XnDumpWriter; 00024 00025 //--------------------------------------------------------------------------- 00026 // Functions 00027 //--------------------------------------------------------------------------- 00028 XN_C_API XnStatus XN_C_DECL xnDumpRegisterWriter(XnDumpWriter* pWriter); 00029 00030 XN_C_API void XN_C_DECL xnDumpUnregisterWriter(XnDumpWriter* pWriter); 00031 00032 XN_C_API XnStatus XN_C_DECL xnDumpSetFilesOutput(XnBool bOn); 00033 00034 //--------------------------------------------------------------------------- 00035 // Helpers 00036 //--------------------------------------------------------------------------- 00037 #ifdef __cplusplus 00038 00039 class XnDumpWriterBase 00040 { 00041 public: 00042 XnDumpWriterBase() : m_bRegistered(FALSE) 00043 { 00044 m_cObject.pCookie = this; 00045 m_cObject.OpenFile = OpenFileCallback; 00046 m_cObject.Write = WriteCallback; 00047 m_cObject.CloseFile = CloseFileCallback; 00048 } 00049 00050 virtual ~XnDumpWriterBase() 00051 { 00052 Unregister(); 00053 } 00054 00055 XnStatus Register() 00056 { 00057 XnStatus nRetVal = XN_STATUS_OK; 00058 00059 if (!m_bRegistered) 00060 { 00061 OnRegister(); 00062 00063 nRetVal = xnDumpRegisterWriter(&m_cObject); 00064 if (nRetVal != XN_STATUS_OK) 00065 { 00066 OnUnregister(); 00067 return (nRetVal); 00068 } 00069 00070 m_bRegistered = TRUE; 00071 } 00072 00073 return (XN_STATUS_OK); 00074 } 00075 00076 void Unregister() 00077 { 00078 if (m_bRegistered) 00079 { 00080 xnDumpUnregisterWriter(&m_cObject); 00081 m_bRegistered = FALSE; 00082 00083 OnUnregister(); 00084 } 00085 } 00086 00087 inline XnBool IsRegistered() { return m_bRegistered; } 00088 00089 virtual XnDumpWriterFileHandle OpenFile(const XnChar* strDumpName, XnBool bSessionDump, const XnChar* strFileName) = 0; 00090 virtual void Write(XnDumpWriterFileHandle hFile, const void* pBuffer, XnUInt32 nBufferSize) = 0; 00091 virtual void CloseFile(XnDumpWriterFileHandle hFile) = 0; 00092 00093 operator const XnDumpWriter*() const 00094 { 00095 return &m_cObject; 00096 } 00097 00098 protected: 00099 virtual void OnRegister() {} 00100 virtual void OnUnregister() {} 00101 00102 private: 00103 static XnDumpWriterFileHandle XN_CALLBACK_TYPE OpenFileCallback(void* pCookie, const XnChar* strDumpName, XnBool bSessionDump, const XnChar* strFileName) 00104 { 00105 XnDumpWriterBase* pThis = (XnDumpWriterBase*)pCookie; 00106 return pThis->OpenFile(strDumpName, bSessionDump, strFileName); 00107 } 00108 00109 static void XN_CALLBACK_TYPE WriteCallback(void* pCookie, XnDumpWriterFileHandle hFile, const void* pBuffer, XnUInt32 nBufferSize) 00110 { 00111 XnDumpWriterBase* pThis = (XnDumpWriterBase*)pCookie; 00112 return pThis->Write(hFile, pBuffer, nBufferSize); 00113 } 00114 00115 static void XN_CALLBACK_TYPE CloseFileCallback(void* pCookie, XnDumpWriterFileHandle hFile) 00116 { 00117 XnDumpWriterBase* pThis = (XnDumpWriterBase*)pCookie; 00118 return pThis->CloseFile(hFile); 00119 } 00120 00121 XnDumpWriter m_cObject; 00122 XnBool m_bRegistered; 00123 }; 00124 00125 #endif 00126 00127 #endif // __XN_DUMP_WRITERS_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
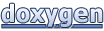