![]() |
OpenNI 1.5.4
|
XnDump.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_DUMP_H__ 00023 #define __XN_DUMP_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include "XnPlatform.h" 00029 #include "XnStatus.h" 00030 00031 //--------------------------------------------------------------------------- 00032 // Types 00033 //--------------------------------------------------------------------------- 00034 struct XnDumpFile; 00035 typedef struct XnDumpFile XnDumpFile; 00036 00037 //--------------------------------------------------------------------------- 00038 // Functions 00039 //--------------------------------------------------------------------------- 00040 00047 XN_C_API XnStatus XN_C_DECL xnDumpSetMaskState(const XnChar* strMask, XnBool bEnabled); 00048 00054 XN_C_API XnBool XN_C_DECL xnLogIsDumpMaskEnabled(const XnChar* strDumpMask); 00055 00064 XN_C_API XnDumpFile* XN_C_DECL xnDumpFileOpen(const XnChar* strDumpName, const XnChar* strNameFormat, ...); 00065 00078 XN_C_API XnDumpFile* XN_C_DECL xnDumpFileOpenEx(const XnChar* strDumpName, XnBool bForce, XnBool bSessionDump, const XnChar* strNameFormat, ...); 00079 00087 XN_C_API void XN_C_DECL _xnDumpFileWriteBuffer(XnDumpFile* pFile, const void* pBuffer, XnUInt32 nBufferSize); 00088 00097 XN_C_API void XN_C_DECL _xnDumpFileWriteString(XnDumpFile* pFile, const XnChar* strFormat, ...); 00098 00104 XN_C_API void XN_C_DECL _xnDumpFileClose(XnDumpFile* pFile); 00105 00106 #define xnDumpFileWriteBuffer(pFile, pBuffer, nBufferSize) \ 00107 if ((pFile) != NULL) \ 00108 { \ 00109 _xnDumpFileWriteBuffer(pFile, pBuffer, nBufferSize); \ 00110 } \ 00111 00112 #define xnDumpFileClose(pFile) \ 00113 if ((pFile) != NULL) \ 00114 { \ 00115 _xnDumpFileClose(pFile); \ 00116 pFile = NULL; \ 00117 } \ 00118 00119 #if XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_WIN32_VAARGS_STYLE 00120 #define xnDumpFileWriteString(pFile, strFormat, ...) \ 00121 if ((pFile) != NULL) \ 00122 { \ 00123 _xnDumpFileWriteString(pFile, strFormat, __VA_ARGS__); \ 00124 } 00125 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_GCC_VAARGS_STYLE 00126 #define xnDumpFileWriteString(pFile, strFormat, ...) \ 00127 if ((pFile) != NULL) \ 00128 { \ 00129 _xnDumpFileWriteString(pFile, strFormat, ##__VA_ARGS__);\ 00130 } 00131 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_ARC_VAARGS_STYLE 00132 #define xnDumpFileWriteString(pFile, strFormat, ...) \ 00133 if ((pFile) != NULL) \ 00134 { \ 00135 _xnDumpFileWriteString(pFile, strFormat); \ 00136 } 00137 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_NO_VAARGS 00138 #define xnDumpFileWriteString(pFile, strFormat, arg) \ 00139 if ((pFile) != NULL) \ 00140 { \ 00141 _xnDumpFileWriteString(pFile, strFormat,arg); \ 00142 } 00143 #else 00144 #error Xiron Log - Unknown VAARGS type! 00145 #endif 00146 00147 00148 //--------------------------------------------------------------------------- 00149 // Backwards Compatibility Stuff 00150 //--------------------------------------------------------------------------- 00151 00152 #ifndef __XN_NO_BC__ 00153 00154 #include "XnOS.h" 00155 00156 typedef struct XnDump 00157 { 00158 XN_FILE_HANDLE hFile; 00159 } XnDump; 00160 00161 const XnDump XN_DUMP_CLOSED = { XN_INVALID_FILE_HANDLE }; 00162 00163 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpInit(XnDump* pDump, const XnChar* csDumpMask, const XnChar* csHeader, const XnChar* csFileNameFormat, ...); 00164 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpForceInit(XnDump* pDump, const XnChar* csHeader, const XnChar* csFileNameFormat, ...); 00165 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpClose(XnDump* pDump); 00166 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpWriteBufferImpl(XnDump dump, const void* pBuffer, XnUInt32 nBufferSize); 00167 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpWriteStringImpl(XnDump dump, const XnChar* csFormat, ...); 00168 XN_C_API void XN_API_DEPRECATED("Use xnDumpFileX methods instead") XN_C_DECL xnDumpFlush(XnDump dump); 00169 00170 #define xnDumpWriteBuffer(dump, pBuffer, nBufferSize) \ 00171 if (dump.hFile != XN_INVALID_FILE_HANDLE) \ 00172 { \ 00173 xnDumpWriteBufferImpl(dump, pBuffer, nBufferSize); \ 00174 } 00175 00176 #if XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_WIN32_VAARGS_STYLE 00177 #define xnDumpWriteString(dump, csFormat, ...) \ 00178 if ((dump).hFile != XN_INVALID_FILE_HANDLE) { \ 00179 xnDumpWriteStringImpl((dump), csFormat, __VA_ARGS__); \ 00180 } 00181 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_GCC_VAARGS_STYLE 00182 #define xnDumpWriteString(dump, csFormat, ...) \ 00183 if ((dump).hFile != XN_INVALID_FILE_HANDLE) { \ 00184 xnDumpWriteStringImpl((dump), csFormat, ##__VA_ARGS__); \ 00185 } 00186 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_ARC_VAARGS_STYLE 00187 #define xnDumpWriteString(dump, csFormat...) \ 00188 if ((dump).hFile != XN_INVALID_FILE_HANDLE) { \ 00189 xnDumpWriteStringImpl((dump), csFormat); \ 00190 } 00191 #elif XN_PLATFORM_VAARGS_TYPE == XN_PLATFORM_USE_NO_VAARGS 00192 #define xnDumpWriteString(dump, csFormat, arg) \ 00193 if ((dump).hFile != XN_INVALID_FILE_HANDLE) { \ 00194 xnDumpWriteStringImpl((dump), csFormat, arg); \ 00195 } 00196 #else 00197 #error Xiron Log - Unknown VAARGS type! 00198 #endif 00199 00200 #endif // #ifndef __XN_NO_BC__ 00201 00202 #endif // __XN_DUMP_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
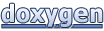