![]() |
OpenNI 1.5.4
|
XnCyclicStackT.h
Go to the documentation of this file.
00001 #ifndef _XN_CYCLIC_STACK_T_H_ 00002 #define _XN_CYCLIC_STACK_T_H_ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include "XnStackT.h" 00008 00009 //--------------------------------------------------------------------------- 00010 // Code 00011 //--------------------------------------------------------------------------- 00012 00013 template<class T, XnUInt32 TDefaultMaxDepth, class TAlloc = XnLinkedNodeDefaultAllocatorT<T> > 00014 class XnCyclicStackT : protected XnStackT<T, TAlloc> 00015 { 00016 public: 00017 typedef XnStackT<T, TAlloc> Base; 00018 00019 XnCyclicStackT(XnUInt32 nMaxDepth = TDefaultMaxDepth) : Base(), m_nMaxDepth(nMaxDepth) {} 00020 00021 XnCyclicStackT(const XnCyclicStackT& other) : Base(other) 00022 { 00023 *this = other; 00024 } 00025 00026 XnCyclicStackT& operator=(const XnCyclicStackT& other) 00027 { 00028 Base::operator=(other); 00029 m_nMaxDepth = other.m_nMaxDepth; 00030 return *this; 00031 } 00032 00033 ~XnCyclicStackT() {} 00034 00035 using typename Base::ConstIterator; 00036 using Base::Remove; 00037 using Base::IsEmpty; 00038 using Base::Size; 00039 00040 XnStatus SetMaxSize(XnUInt32 nMaxSize) 00041 { 00042 XnStatus nRetVal = XN_STATUS_OK; 00043 00044 while (Size() > nMaxSize) 00045 { 00046 nRetVal = Remove(this->ReverseBegin()); 00047 XN_IS_STATUS_OK(nRetVal); 00048 } 00049 00050 m_nMaxDepth = nMaxSize; 00051 00052 return (XN_STATUS_OK); 00053 } 00054 00055 XnStatus Push(T const& value) 00056 { 00057 XnStatus nRetVal = XN_STATUS_OK; 00058 if (Size() == m_nMaxDepth) 00059 { 00060 nRetVal = Remove(this->ReverseBegin()); 00061 XN_IS_STATUS_OK(nRetVal); 00062 } 00063 00064 nRetVal = Base::Push(value); 00065 XN_IS_STATUS_OK(nRetVal); 00066 00067 return (XN_STATUS_OK); 00068 } 00069 00070 using Base::Pop; 00071 using Base::Top; 00072 using Base::Begin; 00073 using Base::End; 00074 00075 protected: 00076 XnUInt32 m_nMaxDepth; 00077 }; 00078 00079 00080 #endif // _XN_CYCLIC_STACK_T_H_
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
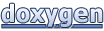