![]() |
OpenNI 1.5.4
|
XnCyclicQueueT.h
Go to the documentation of this file.
00001 #ifndef _XN_CYCLIC_QUEUE_T_H_ 00002 #define _XN_CYCLIC_QUEUE_T_H_ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include "XnQueueT.h" 00008 00009 //--------------------------------------------------------------------------- 00010 // Code 00011 //--------------------------------------------------------------------------- 00012 00013 template<class T, XnUInt32 TDefaultMaxDepth, class TAlloc = XnLinkedNodeDefaultAllocatorT<T> > 00014 class XnCyclicQueueT : protected XnQueueT<T, TAlloc> 00015 { 00016 public: 00017 typedef XnQueueT<T, TAlloc> Base; 00018 00019 XnCyclicQueueT(XnUInt32 nMaxDepth = TDefaultMaxDepth) : Base(), m_nMaxDepth(nMaxDepth) {} 00020 00021 XnCyclicQueueT(const XnCyclicQueueT& other) : Base(other) 00022 { 00023 *this = other; 00024 } 00025 00026 XnCyclicQueueT& operator=(const XnCyclicQueueT& other) 00027 { 00028 Base::operator=(other); 00029 m_nMaxDepth = other.m_nMaxDepth; 00030 return *this; 00031 } 00032 00033 ~XnCyclicQueueT() {} 00034 00035 using Base::ConstIterator; 00036 using Base::IsEmpty; 00037 using Base::Size; 00038 00039 XnStatus SetMaxSize(XnUInt32 nMaxSize) 00040 { 00041 XnStatus nRetVal = XN_STATUS_OK; 00042 00043 while (Size() > nMaxSize) 00044 { 00045 nRetVal = Remove(this->Begin()); 00046 XN_IS_STATUS_OK(nRetVal); 00047 } 00048 00049 m_nMaxDepth = nMaxSize; 00050 00051 return (XN_STATUS_OK); 00052 } 00053 00054 XnStatus Push(T const& value) 00055 { 00056 XnStatus nRetVal = XN_STATUS_OK; 00057 if (Size() == m_nMaxDepth) 00058 { 00059 nRetVal = Remove(this->Begin()); 00060 XN_IS_STATUS_OK(nRetVal); 00061 } 00062 00063 nRetVal = Base::Push(value); 00064 XN_IS_STATUS_OK(nRetVal); 00065 00066 return (XN_STATUS_OK); 00067 } 00068 00069 using Base::Pop; 00070 using Base::Top; 00071 using Base::Begin; 00072 using Base::End; 00073 00074 protected: 00075 XnUInt32 m_nMaxDepth; 00076 }; 00077 00078 00079 #endif // _XN_CYCLIC_QUEUE_T_H_
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
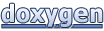